Understanding Conditionals: If, Else If, and Else Statements Explained
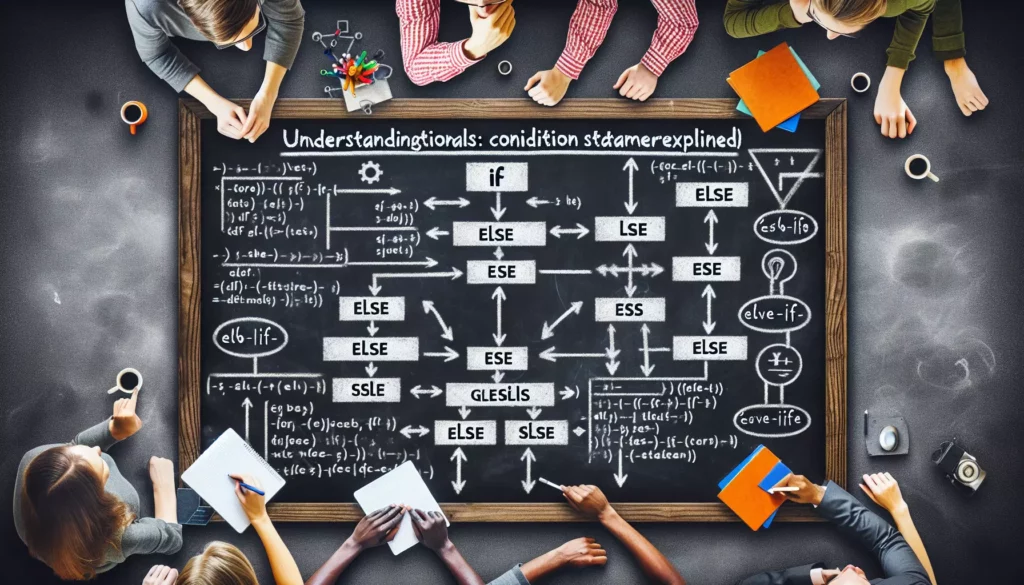
In the world of programming, decision-making is a crucial aspect of creating dynamic and responsive code. Conditional statements are the building blocks that allow programs to make choices based on different scenarios. Among these, the if, else if, and else statements are fundamental constructs that every programmer should master. In this comprehensive guide, we’ll dive deep into these conditional statements, exploring their syntax, usage, and best practices to help you become a more proficient coder.
What Are Conditional Statements?
Conditional statements are programming constructs that allow a program to execute different code blocks based on whether a specified condition is true or false. They enable developers to create branching logic in their code, making applications more flexible and capable of handling various scenarios.
The most common types of conditional statements are:
- If statements
- Else statements
- Else if statements
These statements can be used individually or in combination to create complex decision-making structures in your code.
The If Statement
The if statement is the most basic form of conditional statement. It allows you to execute a block of code only if a specified condition is true.
Syntax of an If Statement
The general syntax of an if statement is as follows:
if (condition) {
// Code to be executed if the condition is true
}
Let’s break down the components:
- if: This keyword initiates the conditional statement.
- condition: This is a boolean expression that evaluates to either true or false.
- { }: The curly braces contain the code block to be executed if the condition is true.
Example of an If Statement
Here’s a simple example of an if statement in JavaScript:
let age = 18;
if (age >= 18) {
console.log("You are eligible to vote.");
}
In this example, if the value of age
is 18 or greater, the message “You are eligible to vote.” will be printed to the console.
The Else Statement
The else statement is used in conjunction with an if statement to specify a block of code that should be executed when the if condition is false.
Syntax of an If-Else Statement
The syntax for an if-else statement is as follows:
if (condition) {
// Code to be executed if the condition is true
} else {
// Code to be executed if the condition is false
}
Example of an If-Else Statement
Let’s modify our previous example to include an else statement:
let age = 16;
if (age >= 18) {
console.log("You are eligible to vote.");
} else {
console.log("You are not eligible to vote yet.");
}
In this case, if age
is less than 18, the message “You are not eligible to vote yet.” will be displayed.
The Else If Statement
The else if statement allows you to check multiple conditions in sequence. It’s used when you have more than two possible outcomes.
Syntax of an If-Else If-Else Statement
The syntax for an if-else if-else statement is as follows:
if (condition1) {
// Code to be executed if condition1 is true
} else if (condition2) {
// Code to be executed if condition2 is true
} else if (condition3) {
// Code to be executed if condition3 is true
} else {
// Code to be executed if all conditions are false
}
You can have as many else if statements as needed, and the else statement at the end is optional.
Example of an If-Else If-Else Statement
Let’s look at an example that categorizes a person’s age group:
let age = 25;
if (age < 13) {
console.log("You are a child.");
} else if (age < 20) {
console.log("You are a teenager.");
} else if (age < 30) {
console.log("You are a young adult.");
} else {
console.log("You are an adult.");
}
In this example, the code will check each condition in order and execute the corresponding block for the first true condition it encounters.
Best Practices for Using Conditional Statements
To write clean, efficient, and maintainable code, consider the following best practices when using conditional statements:
1. Keep Conditions Simple
Try to keep your conditions as simple and clear as possible. If a condition becomes too complex, consider breaking it down into smaller, more manageable parts or using separate variables to store intermediate results.
2. Use Meaningful Variable Names
Choose descriptive variable names that clearly indicate what the condition is checking. This makes your code more readable and self-documenting.
3. Avoid Nested Conditionals When Possible
Excessive nesting of conditional statements can make your code hard to read and maintain. Consider using early returns or restructuring your logic to reduce nesting.
4. Use Switch Statements for Multiple Conditions
If you’re checking a single variable against multiple possible values, consider using a switch statement instead of multiple if-else statements.
5. Consider the Order of Conditions
When using else if statements, order your conditions from most specific to least specific. This can improve the efficiency of your code and prevent unintended behavior.
6. Use Proper Indentation
Consistent and proper indentation makes your code more readable and helps in identifying the scope of each code block.
Common Pitfalls and How to Avoid Them
While working with conditional statements, there are some common mistakes that programmers often make. Let’s explore these pitfalls and learn how to avoid them:
1. Using = Instead of ==
In many programming languages, the single equals sign (=) is used for assignment, while double equals (==) or triple equals (===) are used for comparison.
Incorrect:
if (x = 5) {
// This will always evaluate to true
}
Correct:
if (x == 5) {
// This correctly compares x to 5
}
2. Forgetting Curly Braces
While some languages allow you to omit curly braces for single-line statements, it’s generally a good practice to always use them to avoid potential errors and improve readability.
Potentially problematic:
if (condition)
doSomething();
doSomethingElse(); // This will always execute, regardless of the condition
Better practice:
if (condition) {
doSomething();
doSomethingElse(); // This will only execute if the condition is true
}
3. Not Considering All Possible Cases
When using multiple conditions, make sure you account for all possible scenarios, including edge cases.
let grade = 85;
if (grade >= 90) {
console.log("A");
} else if (grade >= 80) {
console.log("B");
} else if (grade >= 70) {
console.log("C");
}
// What about grades below 70?
It’s often a good idea to include a final else statement to handle any cases that don’t meet the specified conditions.
4. Overusing Else If
While else if statements are useful, overusing them can lead to code that’s hard to read and maintain. If you find yourself with a long chain of else if statements, consider using a switch statement or restructuring your logic.
Advanced Techniques with Conditional Statements
As you become more comfortable with basic conditional statements, you can explore more advanced techniques to make your code more efficient and expressive:
1. Ternary Operator
The ternary operator provides a concise way to write simple if-else statements in a single line.
let result = (condition) ? valueIfTrue : valueIfFalse;
Example:
let age = 20;
let canVote = (age >= 18) ? "Yes" : "No";
console.log(canVote); // Outputs: "Yes"
2. Short-Circuit Evaluation
Logical operators (&& and ||) can be used for short-circuit evaluation, allowing you to write more concise conditional statements.
// Using && for short-circuit evaluation
(condition) && doSomething();
// Using || for default values
let name = userInput || "Guest";
3. Switch Statements
For multiple conditions checking against a single variable, switch statements can be more readable and potentially more efficient than a series of if-else statements.
switch (variable) {
case value1:
// code block
break;
case value2:
// code block
break;
default:
// code block
}
4. Nullish Coalescing Operator (??)
In modern JavaScript, the nullish coalescing operator (??) provides a way to handle null or undefined values in conditional expressions.
let user = {
name: "John",
age: null
};
let userAge = user.age ?? "Age not provided";
console.log(userAge); // Outputs: "Age not provided"
Conditional Statements in Different Programming Languages
While the concepts behind conditional statements are similar across most programming languages, the syntax can vary. Let’s look at how if-else statements are implemented in a few popular languages:
Python
age = 20
if age >= 18:
print("You can vote")
elif age >= 16:
print("You can't vote, but you can drive")
else:
print("You can't vote or drive")
Java
int age = 20;
if (age >= 18) {
System.out.println("You can vote");
} else if (age >= 16) {
System.out.println("You can't vote, but you can drive");
} else {
System.out.println("You can't vote or drive");
}
C++
#include <iostream>
int main() {
int age = 20;
if (age >= 18) {
std::cout << "You can vote" << std::endl;
} else if (age >= 16) {
std::cout << "You can't vote, but you can drive" << std::endl;
} else {
std::cout << "You can't vote or drive" << std::endl;
}
return 0;
}
Practical Applications of Conditional Statements
Conditional statements are used extensively in real-world applications. Here are some common scenarios where you might use them:
1. Form Validation
When validating user input in forms, conditional statements are used to check if the input meets certain criteria.
function validateEmail(email) {
if (email.includes("@") && email.includes(".")) {
return "Valid email format";
} else {
return "Invalid email format";
}
}
2. Game Logic
In game development, conditionals are used to control game flow, check for collisions, manage scores, etc.
if (playerScore > highScore) {
highScore = playerScore;
console.log("New high score!");
}
3. User Authentication
Conditional statements are crucial in implementing user authentication systems.
if (username === storedUsername && password === storedPassword) {
allowAccess();
} else {
denyAccess();
}
4. Responsive Design
In web development, conditionals can be used to apply different styles or functionalities based on screen size or device type.
if (window.innerWidth < 768) {
// Apply mobile styles
} else {
// Apply desktop styles
}
Conclusion
Conditional statements are a fundamental concept in programming that allow for dynamic and responsive code. By mastering if, else if, and else statements, you’ll be able to create more complex and intelligent programs. Remember to keep your conditions clear, use appropriate syntax for your programming language, and consider readability and maintainability in your code structure.
As you continue to develop your programming skills, you’ll find that proficiency with conditional statements is crucial for solving algorithmic problems, implementing game logic, creating responsive user interfaces, and much more. Practice using these statements in various scenarios to become more comfortable with their application and to develop a intuitive understanding of when and how to use them effectively.
Whether you’re just starting out in coding or preparing for technical interviews with major tech companies, a solid grasp of conditional statements will serve you well throughout your programming journey. Keep practicing, exploring different use cases, and challenging yourself with more complex decision-making scenarios in your code.