Understanding Computational Complexity: Time and Space
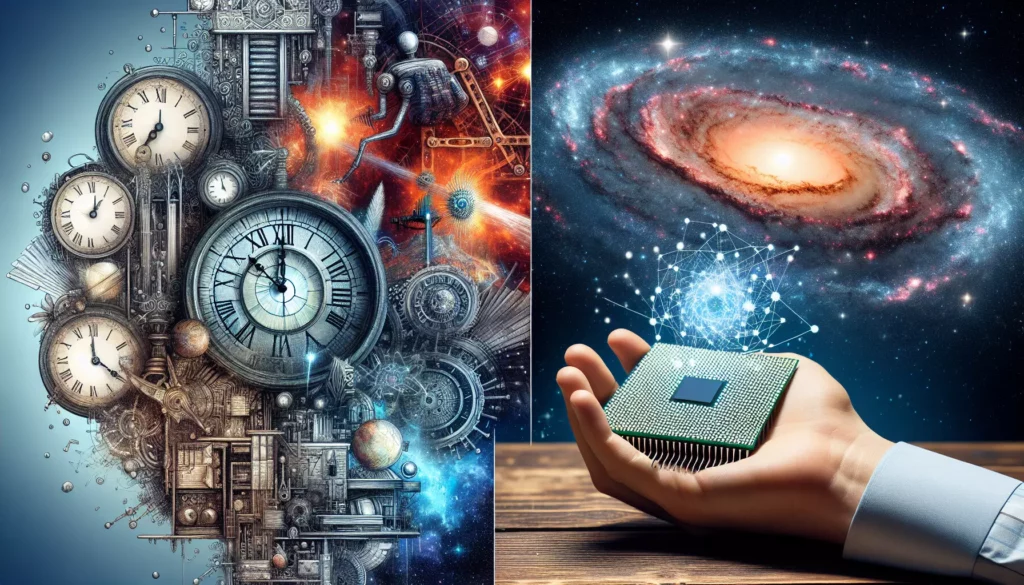
In the world of computer science and software engineering, efficiency is key. As programmers, we’re not just concerned with writing code that works; we want our code to work well, fast, and with minimal resource consumption. This is where the concept of computational complexity comes into play. Understanding computational complexity is crucial for developing efficient algorithms and optimizing code performance, especially when preparing for technical interviews at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google).
In this comprehensive guide, we’ll dive deep into the world of computational complexity, focusing on two primary aspects: time complexity and space complexity. We’ll explore what these concepts mean, how they’re measured, and why they’re essential for every programmer to understand.
What is Computational Complexity?
Computational complexity is a measure of the resources required by an algorithm to solve a problem. These resources typically include:
- Time: How long does the algorithm take to run?
- Space: How much memory does the algorithm use?
By analyzing these factors, we can compare different algorithms and determine which one is more efficient for a given problem. This analysis is crucial when dealing with large datasets or when working on systems with limited resources.
Time Complexity
Time complexity refers to the amount of time an algorithm takes to complete as a function of the input size. It’s typically expressed using Big O notation, which describes the upper bound of the growth rate of an algorithm’s running time.
Understanding Big O Notation
Big O notation provides a standardized way to describe the time complexity of an algorithm. It focuses on the worst-case scenario and gives us an idea of how the algorithm’s performance scales as the input size increases. Some common Big O notations include:
- O(1): Constant time
- O(log n): Logarithmic time
- O(n): Linear time
- O(n log n): Linearithmic time
- O(n²): Quadratic time
- O(2^n): Exponential time
Examples of Time Complexity
Let’s look at some examples to better understand time complexity:
1. Constant Time – O(1)
An algorithm with constant time complexity always takes the same amount of time to execute, regardless of the input size. For example, accessing an element in an array by its index:
def get_element(arr, index):
return arr[index]
This function will always take the same amount of time, no matter how large the array is.
2. Linear Time – O(n)
Linear time complexity means the execution time grows linearly with the input size. A common example is iterating through an array:
def find_element(arr, target):
for element in arr:
if element == target:
return True
return False
In the worst case, this function might need to check every element in the array, so its time complexity is O(n), where n is the number of elements in the array.
3. Quadratic Time – O(n²)
Quadratic time complexity occurs when the execution time is proportional to the square of the input size. Nested loops often result in quadratic time complexity:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
This bubble sort algorithm has two nested loops, resulting in O(n²) time complexity.
Space Complexity
Space complexity refers to the amount of memory an algorithm uses relative to the input size. Like time complexity, it’s typically expressed using Big O notation.
Types of Space Complexity
When analyzing space complexity, we consider two types of space usage:
- Auxiliary Space: The extra space used by the algorithm, not including the space taken by the inputs.
- Total Space: The sum of the auxiliary space and the space used by the input.
In most cases, we focus on the auxiliary space when discussing space complexity.
Examples of Space Complexity
Let’s examine some examples to illustrate different space complexities:
1. Constant Space – O(1)
An algorithm with constant space complexity uses the same amount of extra space regardless of the input size. For example:
def sum_array(arr):
total = 0
for num in arr:
total += num
return total
This function only uses a single variable (total) regardless of the array size, so its space complexity is O(1).
2. Linear Space – O(n)
Linear space complexity means the space usage grows linearly with the input size. Creating a new array based on the input is a common example:
def double_array(arr):
return [num * 2 for num in arr]
This function creates a new array with the same length as the input, resulting in O(n) space complexity.
3. Quadratic Space – O(n²)
Quadratic space complexity occurs when the space usage is proportional to the square of the input size. For example, creating a 2D matrix based on the input size:
def create_matrix(n):
return [[0 for _ in range(n)] for _ in range(n)]
This function creates an n x n matrix, resulting in O(n²) space complexity.
The Trade-off Between Time and Space
Often in algorithm design, there’s a trade-off between time complexity and space complexity. An algorithm that runs faster might require more memory, and vice versa. This trade-off is known as the time-space trade-off.
For example, consider the problem of finding the nth Fibonacci number:
Recursive Approach (High Time Complexity, Low Space Complexity)
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
This recursive approach has a time complexity of O(2^n) but a space complexity of O(n) due to the call stack.
Dynamic Programming Approach (Lower Time Complexity, Higher Space Complexity)
def fibonacci_dp(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
This dynamic programming approach has a time complexity of O(n) but a space complexity of O(n) due to the additional array.
Choosing between these approaches depends on the specific requirements of your application and the constraints of your system.
Analyzing Complexity in Practice
When analyzing the complexity of an algorithm, consider the following steps:
- Identify the input: Determine what variable(s) represent the input size.
- Count the operations: Identify the basic operations performed by the algorithm.
- Determine the growth rate: Analyze how the number of operations grows as the input size increases.
- Express in Big O notation: Use the simplest term that describes the upper bound of the growth rate.
Remember that Big O notation represents the worst-case scenario. In some cases, it’s also useful to consider average-case complexity (often denoted with Θ (Theta) notation) or best-case complexity (often denoted with Ω (Omega) notation).
Common Pitfalls in Complexity Analysis
When analyzing complexity, be aware of these common pitfalls:
- Ignoring constants: While O(2n) and O(n) are equivalent in Big O notation, the constant factor can make a significant difference in practice for small inputs.
- Overlooking hidden loops: Some operations, like string concatenation or certain built-in functions, may have hidden loops that affect complexity.
- Focusing only on time complexity: Don’t forget to consider space complexity, especially when working with limited memory resources.
- Assuming worst-case scenario always applies: While Big O represents the worst case, algorithms often perform better in practice.
Importance in Technical Interviews
Understanding computational complexity is crucial for technical interviews, especially at top tech companies like FAANG. Interviewers often ask candidates to:
- Analyze the time and space complexity of their solutions
- Optimize algorithms to improve efficiency
- Compare different approaches based on their complexities
- Discuss the trade-offs between time and space complexity
Being able to articulate your understanding of computational complexity demonstrates your ability to write efficient code and make informed decisions about algorithm selection.
Tools and Techniques for Improving Algorithm Efficiency
To improve the efficiency of your algorithms, consider these techniques:
- Use appropriate data structures: Choosing the right data structure can significantly impact your algorithm’s performance. For example, using a hash table for fast lookups instead of a list.
- Apply dynamic programming: For problems with overlapping subproblems, dynamic programming can reduce time complexity by storing and reusing intermediate results.
- Implement divide and conquer: Breaking a problem into smaller subproblems can lead to more efficient solutions, as seen in algorithms like merge sort.
- Utilize caching and memoization: Storing the results of expensive function calls can improve performance in recursive algorithms.
- Consider amortized analysis: Some data structures, like dynamic arrays, have operations that are occasionally expensive but cheap on average.
Conclusion
Understanding computational complexity is a fundamental skill for any programmer, especially those aiming to excel in technical interviews and build efficient, scalable systems. By mastering the concepts of time and space complexity, you’ll be better equipped to analyze, optimize, and compare algorithms.
Remember, the goal isn’t always to achieve the lowest possible time or space complexity. Instead, it’s about finding the right balance for your specific use case, considering factors like input size, system constraints, and the frequency of operations.
As you continue your journey in programming and algorithm design, make it a habit to analyze the complexity of your solutions. With practice, you’ll develop an intuition for efficiency that will serve you well throughout your career.
Keep coding, keep optimizing, and never stop learning!