Understanding Computational Complexity Classes: A Comprehensive Guide
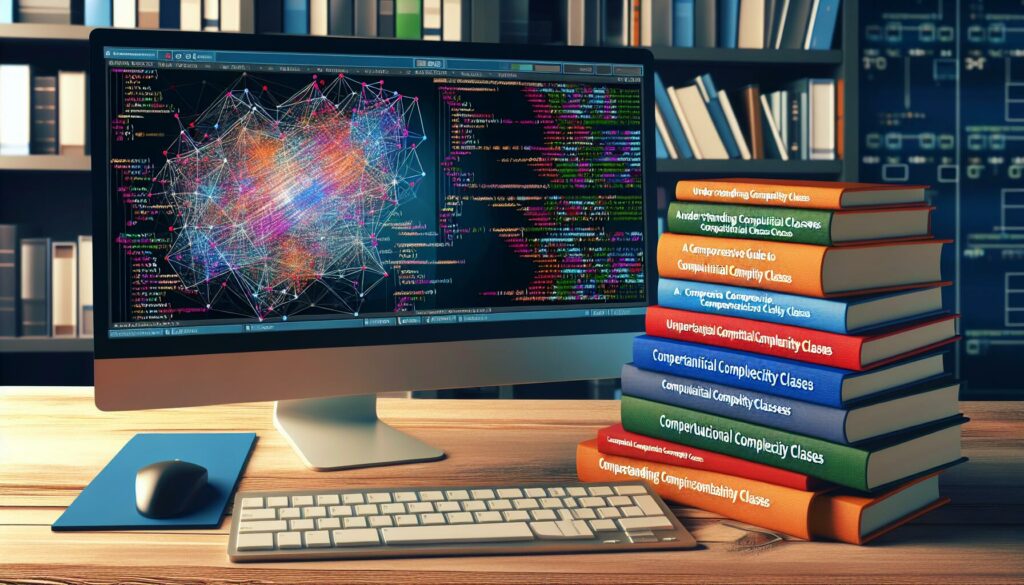
In the world of computer science and algorithm design, understanding computational complexity is crucial. It helps us analyze and compare the efficiency of different algorithms, allowing us to make informed decisions about which solutions to implement in various scenarios. This comprehensive guide will delve into the concept of computational complexity classes, exploring their significance, characteristics, and real-world applications.
What are Computational Complexity Classes?
Computational complexity classes are categories used to classify computational problems based on the resources (typically time or space) required to solve them. These classes help us understand the inherent difficulty of problems and the efficiency of algorithms designed to solve them.
The most common resource considered in complexity analysis is time, often expressed as the number of elementary operations an algorithm needs to perform. Space complexity, which refers to the amount of memory required, is also an important consideration in some contexts.
The Importance of Complexity Classes
Understanding complexity classes is vital for several reasons:
- Algorithm Efficiency: It allows us to compare different algorithms and choose the most efficient one for a given problem.
- Scalability: It helps predict how an algorithm’s performance will change as the input size grows.
- Problem Classification: It enables us to group problems based on their inherent difficulty, which is crucial in theoretical computer science and practical software development.
- Resource Allocation: It aids in estimating the computational resources needed to solve specific problems.
Common Complexity Classes
Let’s explore some of the most important complexity classes, starting from the most efficient and moving towards the more computationally intensive ones.
1. O(1) – Constant Time
Algorithms in this class perform a fixed number of operations, regardless of the input size. These are the most efficient algorithms.
Example: Accessing an array element by its index.
function getElement(arr, index) {
return arr[index];
}
2. O(log n) – Logarithmic Time
These algorithms reduce the problem size by a constant factor in each step. They are very efficient, especially for large inputs.
Example: Binary search in a sorted array.
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
3. O(n) – Linear Time
The running time of these algorithms increases linearly with the input size. They are considered efficient for many practical applications.
Example: Finding the maximum element in an unsorted array.
function findMax(arr) {
let max = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) max = arr[i];
}
return max;
}
4. O(n log n) – Linearithmic Time
Many efficient sorting algorithms fall into this category. These algorithms are more complex than linear time but still considered efficient for most purposes.
Example: Merge Sort algorithm.
function mergeSort(arr) {
if (arr.length <= 1) return arr;
const mid = Math.floor(arr.length / 2);
const left = arr.slice(0, mid);
const right = arr.slice(mid);
return merge(mergeSort(left), mergeSort(right));
}
function merge(left, right) {
let result = [];
let leftIndex = 0;
let rightIndex = 0;
while (leftIndex < left.length && rightIndex < right.length) {
if (left[leftIndex] < right[rightIndex]) {
result.push(left[leftIndex]);
leftIndex++;
} else {
result.push(right[rightIndex]);
rightIndex++;
}
}
return result.concat(left.slice(leftIndex)).concat(right.slice(rightIndex));
}
5. O(n^2) – Quadratic Time
These algorithms have a running time that is proportional to the square of the input size. They are less efficient and may become impractical for large inputs.
Example: Bubble Sort algorithm.
function bubbleSort(arr) {
const n = arr.length;
for (let i = 0; i < n; i++) {
for (let j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// Swap elements
[arr[j], arr[j + 1]] = [arr[j + 1], arr[j]];
}
}
}
return arr;
}
6. O(2^n) – Exponential Time
The running time doubles with each addition to the input size. These algorithms are typically impractical for all but small inputs.
Example: Recursive calculation of Fibonacci numbers (naive approach).
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
7. O(n!) – Factorial Time
These are among the least efficient algorithms, with running time growing faster than exponential algorithms as input size increases.
Example: Generating all permutations of a string.
function generatePermutations(str) {
if (str.length <= 1) return [str];
let permutations = [];
for (let i = 0; i < str.length; i++) {
let char = str[i];
let remainingChars = str.slice(0, i) + str.slice(i + 1);
for (let perm of generatePermutations(remainingChars)) {
permutations.push(char + perm);
}
}
return permutations;
}
The P, NP, and NP-Complete Classes
In computational complexity theory, there are several important classes of problems that are defined based on their solvability by different types of algorithms:
P (Polynomial Time)
Problems in P can be solved by a deterministic Turing machine in polynomial time. These are considered “efficiently solvable” problems.
Example: Sorting an array, finding the shortest path in a graph.
NP (Nondeterministic Polynomial Time)
Problems in NP can be verified in polynomial time. This means that if you’re given a solution, you can check its correctness quickly, even if finding the solution might be difficult.
Example: The Boolean Satisfiability Problem (SAT), where you need to determine if there exists an assignment of variables that makes a Boolean formula true.
NP-Complete
These are the hardest problems in NP. If an efficient algorithm is found for any NP-complete problem, it could be used to solve all problems in NP efficiently.
Example: The Traveling Salesman Problem, where you need to find the shortest possible route that visits each city exactly once and returns to the origin city.
Space Complexity
While time complexity is often the primary focus, space complexity is also crucial in many scenarios. Space complexity refers to the amount of memory an algorithm uses relative to the input size.
Common Space Complexity Classes
- O(1) – Constant Space: The algorithm uses a fixed amount of memory regardless of input size.
- O(n) – Linear Space: Memory usage grows linearly with input size.
- O(n^2) – Quadratic Space: Memory usage grows quadratically with input size.
Example: In-place vs. out-of-place sorting algorithms.
// In-place sorting (O(1) space complexity)
function bubbleSortInPlace(arr) {
const n = arr.length;
for (let i = 0; i < n; i++) {
for (let j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
[arr[j], arr[j + 1]] = [arr[j + 1], arr[j]];
}
}
}
return arr;
}
// Out-of-place sorting (O(n) space complexity)
function mergeSortOutOfPlace(arr) {
if (arr.length <= 1) return arr;
const mid = Math.floor(arr.length / 2);
const left = arr.slice(0, mid);
const right = arr.slice(mid);
return merge(mergeSortOutOfPlace(left), mergeSortOutOfPlace(right));
}
function merge(left, right) {
let result = [];
let leftIndex = 0;
let rightIndex = 0;
while (leftIndex < left.length && rightIndex < right.length) {
if (left[leftIndex] < right[rightIndex]) {
result.push(left[leftIndex]);
leftIndex++;
} else {
result.push(right[rightIndex]);
rightIndex++;
}
}
return result.concat(left.slice(leftIndex)).concat(right.slice(rightIndex));
}
Amortized Analysis
Amortized analysis is a method for analyzing the time complexity of algorithms that perform a sequence of operations. It provides a way to describe the worst-case performance of an algorithm over a sequence of operations, rather than for a single operation.
This type of analysis is particularly useful for data structures that occasionally require expensive operations but have a low average cost per operation when considered over a long sequence of operations.
Example: Dynamic Array (ArrayList in Java, List in Python)
Consider a dynamic array that doubles its size when it reaches capacity:
- Most insertions are O(1) – constant time.
- Occasionally, when the array is full, an insertion requires O(n) time to create a new array and copy all elements.
While the worst-case time for a single insertion is O(n), the amortized time per operation over a sequence of n insertions is O(1).
Best, Average, and Worst-Case Complexity
When analyzing algorithms, we often consider three scenarios:
- Best-case complexity: The minimum time/space an algorithm needs for any input of size n.
- Average-case complexity: The average time/space an algorithm needs over all possible inputs of size n.
- Worst-case complexity: The maximum time/space an algorithm needs for any input of size n.
Example: Quick Sort algorithm
- Best-case: O(n log n) – when the pivot always divides the array into two equal halves.
- Average-case: O(n log n)
- Worst-case: O(n^2) – when the pivot is always the smallest or largest element.
function quickSort(arr, low = 0, high = arr.length - 1) {
if (low < high) {
let pivotIndex = partition(arr, low, high);
quickSort(arr, low, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, high);
}
return arr;
}
function partition(arr, low, high) {
let pivot = arr[high];
let i = low - 1;
for (let j = low; j < high; j++) {
if (arr[j] <= pivot) {
i++;
[arr[i], arr[j]] = [arr[j], arr[i]];
}
}
[arr[i + 1], arr[high]] = [arr[high], arr[i + 1]];
return i + 1;
}
Practical Implications of Complexity Classes
Understanding complexity classes has several practical implications for software development:
- Algorithm Selection: Choose algorithms based on the expected input size and performance requirements of your application.
- Scalability Planning: Anticipate how your application will perform as data volume grows.
- Optimization Priorities: Focus optimization efforts on the most critical or frequently executed parts of your code.
- Resource Allocation: Estimate computational resources needed for different tasks or operations.
- Trade-offs: Make informed decisions about trade-offs between time and space complexity.
Techniques for Improving Algorithm Efficiency
Several techniques can be employed to improve algorithm efficiency:
- Dynamic Programming: Store and reuse solutions to subproblems to avoid redundant computations.
- Greedy Algorithms: Make locally optimal choices at each step to find a global optimum.
- Divide and Conquer: Break down a problem into smaller subproblems, solve them, and combine the results.
- Memoization: Cache results of expensive function calls to avoid repeated computations.
- Data Structure Selection: Choose appropriate data structures that offer efficient operations for your specific use case.
Conclusion
Computational complexity classes provide a powerful framework for understanding and comparing the efficiency of algorithms. By classifying problems and algorithms based on their time and space requirements, we can make informed decisions about algorithm selection, optimization strategies, and resource allocation in software development.
As you progress in your programming journey, particularly when preparing for technical interviews at major tech companies, a solid grasp of computational complexity will be invaluable. It will enable you to analyze and optimize your code effectively, making you a more proficient and sought-after developer.
Remember, while striving for efficiency is important, it’s equally crucial to balance it with code readability and maintainability. The most elegant solutions often find the sweet spot between theoretical efficiency and practical implementation.
Continue to practice analyzing and optimizing algorithms, and you’ll develop an intuition for complexity that will serve you well throughout your coding career. Happy coding!