Understanding Cache Replacement Policies: A Deep Dive into LRU
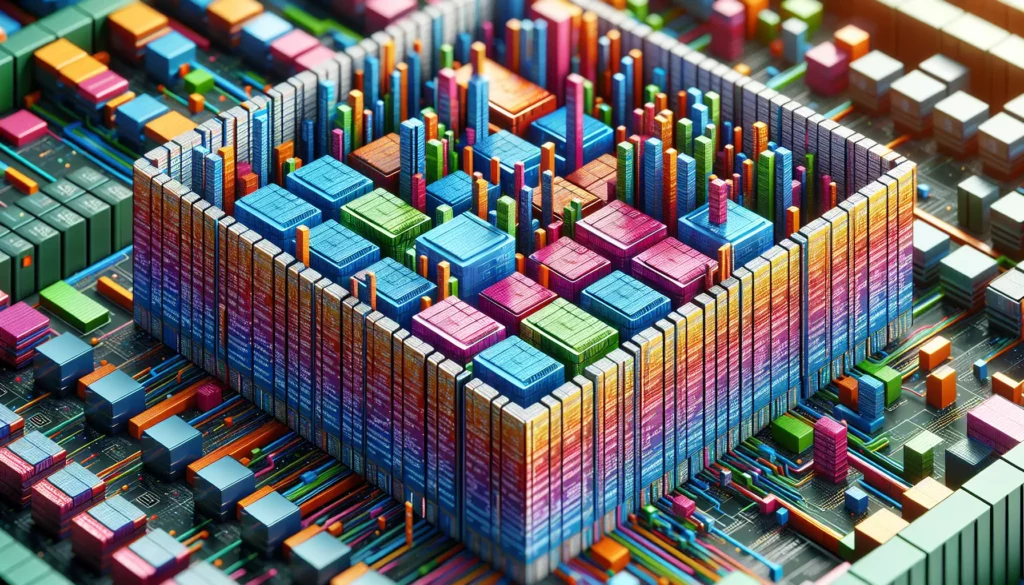
In the world of computer science and system design, caching is a crucial technique used to improve performance and reduce latency. At the heart of any caching system lies the cache replacement policy, which determines how to manage the limited cache space when it becomes full. One of the most popular and effective cache replacement policies is the Least Recently Used (LRU) algorithm. In this comprehensive guide, we’ll explore how LRU works, its advantages and disadvantages, and how it compares to other cache replacement policies.
What is Caching?
Before we dive into the specifics of LRU, let’s briefly review what caching is and why it’s important. Caching is a technique used to store frequently accessed data in a faster, more easily accessible location. This helps reduce the time and resources required to fetch data from slower, primary storage systems.
Caches are used in various contexts, including:
- CPU caches
- Web browser caches
- Content Delivery Networks (CDNs)
- Database query caches
- Application-level caches
In all these scenarios, the cache has a limited size, which means that at some point, it will become full. When this happens, the system needs to decide which items to keep and which to remove to make room for new data. This is where cache replacement policies come into play.
Cache Replacement Policies: An Overview
Cache replacement policies are algorithms that determine which items should be evicted from the cache when it reaches its capacity limit. The goal is to maximize the cache hit rate, which is the percentage of requests that can be served directly from the cache without accessing the slower primary storage.
Some common cache replacement policies include:
- Least Recently Used (LRU)
- First-In-First-Out (FIFO)
- Least Frequently Used (LFU)
- Random Replacement
- Most Recently Used (MRU)
Among these, LRU is one of the most widely used and effective policies due to its simplicity and good performance in many real-world scenarios.
How LRU Works: The Basics
The Least Recently Used (LRU) cache replacement policy is based on the principle that items that have been accessed recently are more likely to be accessed again in the near future. This principle is known as temporal locality, which is a common pattern in many computing workloads.
Here’s how LRU works at a high level:
- When a new item is added to the cache, it’s placed at the “most recently used” end of the cache.
- Whenever an existing item in the cache is accessed, it’s moved to the “most recently used” end.
- When the cache is full and a new item needs to be added, the item at the “least recently used” end is evicted to make room for the new item.
This simple mechanism ensures that the cache always contains the most recently accessed items, which are presumed to be the most likely to be accessed again soon.
Implementing LRU: Data Structures and Algorithms
To implement an efficient LRU cache, we need a combination of data structures that allow for fast lookup, insertion, and deletion operations. A common approach is to use a hash table in conjunction with a doubly linked list.
The Hash Table and Doubly Linked List Approach
Here’s how this implementation works:
- Use a hash table to store key-value pairs, where the key is the cache item’s identifier, and the value is a pointer to a node in the doubly linked list.
- Maintain a doubly linked list to keep track of the order of item usage. The head of the list represents the most recently used item, while the tail represents the least recently used item.
- When an item is accessed or added:
- If it’s already in the cache, move it to the head of the list.
- If it’s not in the cache and the cache is full, remove the tail of the list (least recently used item) and add the new item to the head.
- If it’s not in the cache and there’s space available, simply add it to the head of the list.
This approach allows for O(1) time complexity for both get and put operations, making it very efficient.
Example Implementation in Python
Here’s a simple implementation of an LRU cache in Python:
class Node:
def __init__(self, key, value):
self.key = key
self.value = value
self.prev = None
self.next = None
class LRUCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = {}
self.head = Node(0, 0)
self.tail = Node(0, 0)
self.head.next = self.tail
self.tail.prev = self.head
def _add_node(self, node):
node.prev = self.head
node.next = self.head.next
self.head.next.prev = node
self.head.next = node
def _remove_node(self, node):
prev = node.prev
new = node.next
prev.next = new
new.prev = prev
def _move_to_head(self, node):
self._remove_node(node)
self._add_node(node)
def get(self, key):
if key in self.cache:
node = self.cache[key]
self._move_to_head(node)
return node.value
return -1
def put(self, key, value):
if key in self.cache:
node = self.cache[key]
node.value = value
self._move_to_head(node)
else:
new_node = Node(key, value)
self.cache[key] = new_node
self._add_node(new_node)
if len(self.cache) > self.capacity:
lru = self.tail.prev
self._remove_node(lru)
del self.cache[lru.key]
This implementation uses a hash map (dictionary in Python) for O(1) lookup and a doubly linked list to maintain the order of item usage.
Advantages of LRU
LRU has several advantages that make it a popular choice for cache replacement:
- Simplicity: LRU is easy to understand and implement, making it a good choice for many applications.
- Adaptability: It automatically adapts to changing access patterns by keeping recently used items in the cache.
- Good performance: LRU often performs well in practice, especially for workloads with high temporal locality.
- Constant-time operations: With the right implementation, both get and put operations can be performed in O(1) time.
Disadvantages and Limitations of LRU
Despite its advantages, LRU also has some limitations:
- Scan resistance: LRU can perform poorly when faced with a scanning workload, where a large number of items are accessed once and never again. This can potentially flush the entire cache of useful items.
- No consideration of frequency: LRU only considers recency, not frequency of access. This can lead to suboptimal decisions in some cases.
- Memory overhead: The need for additional data structures (like the doubly linked list) increases memory usage compared to simpler policies.
- Concurrency challenges: In multi-threaded environments, maintaining the LRU order can become a bottleneck and require careful synchronization.
Variations and Improvements on LRU
To address some of the limitations of LRU, several variations and improvements have been developed:
1. LRU-K
LRU-K is an extension of LRU that considers the K most recent references to each item. This helps to better distinguish between frequently and infrequently used items.
2. 2Q
The 2Q algorithm uses two queues: a short-term queue for items accessed only once, and a long-term queue for items accessed multiple times. This helps to protect against scanning workloads.
3. CLOCK
The CLOCK algorithm approximates LRU behavior using a circular buffer and a “clock hand.” It reduces the overhead of maintaining a strict LRU order.
4. ARC (Adaptive Replacement Cache)
ARC combines recency and frequency information and dynamically adjusts the balance between the two based on the observed workload.
Comparing LRU to Other Cache Replacement Policies
To better understand LRU’s strengths and weaknesses, let’s compare it to some other common cache replacement policies:
LRU vs. FIFO (First-In-First-Out)
FIFO is simpler than LRU, as it just maintains items in the order they were added to the cache. However, it doesn’t consider usage patterns, which can lead to poor performance in many scenarios. LRU generally outperforms FIFO for workloads with temporal locality.
LRU vs. LFU (Least Frequently Used)
LFU evicts the item with the lowest access frequency. It can perform better than LRU for some workloads, especially those with stable access patterns. However, LFU can be slow to adapt to changing patterns and may keep items in the cache long after they’ve become irrelevant.
LRU vs. Random Replacement
Random replacement simply chooses a random item to evict when the cache is full. While this policy is very simple and can work surprisingly well in some cases, LRU typically performs better for workloads with temporal locality.
Real-World Applications of LRU
LRU and its variants are widely used in various real-world applications:
1. Operating Systems
Many operating systems use LRU or LRU-like algorithms for page replacement in virtual memory management.
2. Database Management Systems
Database systems often use LRU for buffer pool management to cache frequently accessed data pages.
3. Web Browsers
Web browsers typically use LRU or similar policies to manage their cache of web pages and resources.
4. Content Delivery Networks (CDNs)
CDNs may use LRU or more advanced variants to decide which content to keep in edge caches.
5. In-Memory Caches
Popular in-memory caching systems like Memcached and Redis offer LRU-based eviction policies.
Implementing LRU in Different Programming Languages
While we’ve seen a Python implementation earlier, let’s look at how LRU can be implemented in a few other popular programming languages:
Java Implementation
In Java, we can use the LinkedHashMap class, which provides an LRU-like ordering:
import java.util.LinkedHashMap;
import java.util.Map;
public class LRUCache<K, V> extends LinkedHashMap<K, V> {
private final int capacity;
public LRUCache(int capacity) {
super(capacity, 0.75f, true);
this.capacity = capacity;
}
@Override
protected boolean removeEldestEntry(Map.Entry<K, V> eldest) {
return size() > capacity;
}
}
C++ Implementation
In C++, we can use a combination of std::unordered_map and std::list:
#include <unordered_map>
#include <list>
template<typename K, typename V>
class LRUCache {
private:
int capacity;
std::list<std::pair<K, V>> items;
std::unordered_map<K, typename std::list<std::pair<K, V>>::iterator> cache;
public:
LRUCache(int cap) : capacity(cap) {}
V get(K key) {
auto it = cache.find(key);
if (it == cache.end()) {
return V();
}
items.splice(items.begin(), items, it->second);
return it->second->second;
}
void put(K key, V value) {
auto it = cache.find(key);
if (it != cache.end()) {
items.erase(it->second);
}
items.push_front({key, value});
cache[key] = items.begin();
if (cache.size() > capacity) {
auto last = items.back();
cache.erase(last.first);
items.pop_back();
}
}
};
Best Practices for Using LRU Caches
When implementing or using LRU caches in your applications, consider the following best practices:
- Choose the right capacity: The cache size should be large enough to hold a significant portion of your working set, but not so large that it consumes too much memory.
- Monitor cache performance: Keep track of hit rates and miss rates to ensure your cache is effective.
- Consider your workload: If your workload doesn’t exhibit strong temporal locality, consider alternative policies or LRU variants.
- Use thread-safe implementations: In multi-threaded environments, ensure your LRU implementation is thread-safe or use appropriate synchronization mechanisms.
- Implement expiration policies: Consider combining LRU with time-based expiration to ensure cache freshness.
- Be aware of memory usage: LRU caches can consume significant memory, so monitor and adjust as needed.
Conclusion
The Least Recently Used (LRU) cache replacement policy is a powerful and widely-used algorithm in computer science and system design. Its simplicity, adaptability, and good performance make it an excellent choice for many caching scenarios. By understanding how LRU works, its strengths and limitations, and how to implement it effectively, you can make informed decisions about cache management in your applications.
While LRU is not a one-size-fits-all solution, it provides a solid foundation for cache management. For more complex or specific use cases, consider exploring LRU variants or other cache replacement policies. Remember that the effectiveness of any caching strategy depends on the specific characteristics of your workload and system requirements.
As you design and implement caching solutions, keep in mind the trade-offs between simplicity, performance, and adaptability. With careful consideration and proper implementation, LRU and its variants can significantly improve the performance and efficiency of your systems.