Understanding Cache and Memory Optimization: Boosting Your Code’s Performance
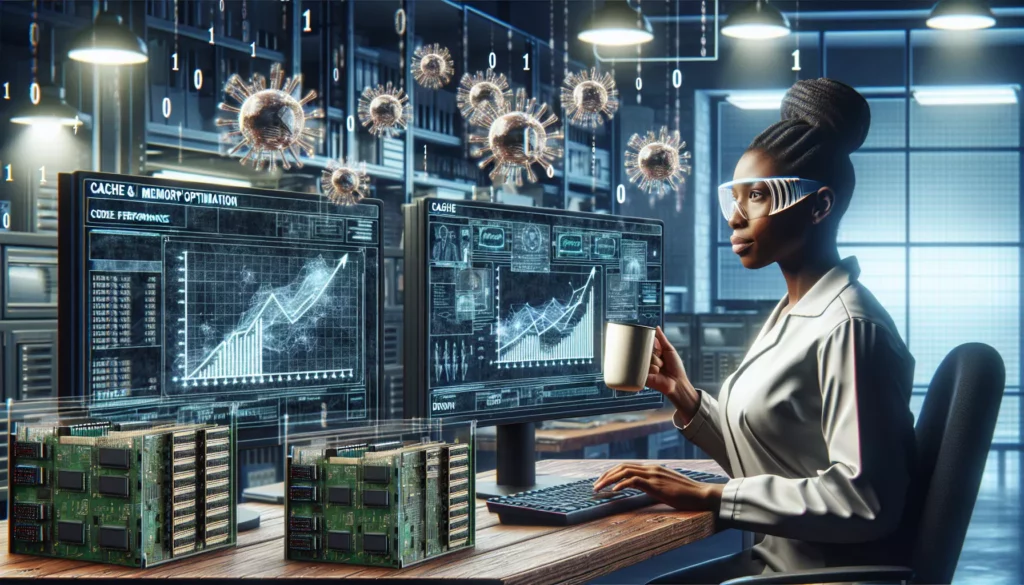
In the world of software development, performance is king. As applications grow in complexity and scale, the ability to optimize memory usage and leverage caching becomes increasingly crucial. Whether you’re preparing for technical interviews at top tech companies or simply aiming to write more efficient code, understanding cache and memory optimization is an essential skill. In this comprehensive guide, we’ll dive deep into the concepts, techniques, and best practices that will help you take your code’s performance to the next level.
Table of Contents
- Introduction to Cache and Memory Optimization
- Cache Basics: What You Need to Know
- Understanding the Memory Hierarchy
- Cache Optimization Techniques
- Effective Memory Management Strategies
- Data Structures and Algorithms for Optimization
- Profiling and Measuring Performance
- Cache and Memory Optimization in Distributed Systems
- Language-Specific Optimization Techniques
- Real-World Examples and Case Studies
- Future Trends in Cache and Memory Optimization
- Conclusion
1. Introduction to Cache and Memory Optimization
Cache and memory optimization are fundamental aspects of performance tuning in computer science. By efficiently managing how data is stored, accessed, and processed, developers can significantly improve the speed and responsiveness of their applications. This optimization process involves understanding the intricate relationship between different levels of memory, from high-speed caches to slower main memory and disk storage.
The importance of cache and memory optimization cannot be overstated. In today’s competitive tech landscape, where companies like Google, Amazon, and Facebook handle massive amounts of data and serve millions of users simultaneously, the ability to squeeze every ounce of performance from hardware resources is a critical skill. For developers aspiring to work at these tech giants or build scalable applications, mastering these concepts is essential.
2. Cache Basics: What You Need to Know
At its core, a cache is a high-speed data storage layer that stores a subset of data, typically transient in nature, so that future requests for that data can be served faster. The primary purpose of a cache is to increase data retrieval performance by reducing the need to access the underlying slower storage layer.
Types of Caches
- Hardware Caches: CPU caches (L1, L2, L3)
- Software Caches: Application-level caches, database query caches
- Distributed Caches: Used in multi-node systems to share cached data across servers
Cache Policies
Effective caching relies on well-defined policies for managing cached data:
- Eviction Policies: LRU (Least Recently Used), LFU (Least Frequently Used), FIFO (First In, First Out)
- Write Policies: Write-through, Write-back
- Consistency Policies: Ensuring cached data remains in sync with the source
Cache Hit vs. Cache Miss
A cache hit occurs when the requested data can be found in a cache, while a cache miss refers to the scenario where it cannot. Understanding and optimizing the cache hit ratio is crucial for performance tuning.
3. Understanding the Memory Hierarchy
The memory hierarchy in modern computer systems consists of multiple levels, each with different characteristics in terms of speed, size, and cost:
- Registers: Fastest, smallest, and most expensive
- CPU Caches (L1, L2, L3): Very fast, small, and expensive
- Main Memory (RAM): Slower than caches, larger, and less expensive
- Solid-State Drives (SSDs): Faster than HDDs, but slower than RAM
- Hard Disk Drives (HDDs): Slowest, largest, and least expensive
Understanding this hierarchy is crucial for optimizing data access patterns and storage strategies in your applications.
Locality of Reference
Two key principles govern efficient use of the memory hierarchy:
- Temporal Locality: Recently accessed items are likely to be accessed again soon
- Spatial Locality: Items physically stored close together tend to be accessed close together in time
Leveraging these principles can significantly improve cache utilization and overall performance.
4. Cache Optimization Techniques
Optimizing cache usage involves various techniques aimed at improving data access patterns and reducing cache misses:
Data Alignment and Padding
Properly aligning data structures can reduce cache misses and improve memory access efficiency. Consider the following C++ example:
// Unoptimized structure
struct UnoptimizedStruct {
char a;
int b;
char c;
};
// Optimized structure
struct OptimizedStruct {
int b;
char a;
char c;
char padding[2]; // Ensure 4-byte alignment
};
The optimized structure reduces padding and improves cache line utilization.
Loop Tiling
Loop tiling (or blocking) is a technique used to improve cache performance in nested loops, especially in matrix operations. Here’s a simple example in C:
// Original matrix multiplication
for (int i = 0; i < N; i++)
for (int j = 0; j < N; j++)
for (int k = 0; k < N; k++)
C[i][j] += A[i][k] * B[k][j];
// Tiled matrix multiplication
#define TILE_SIZE 32
for (int i = 0; i < N; i += TILE_SIZE)
for (int j = 0; j < N; j += TILE_SIZE)
for (int k = 0; k < N; k += TILE_SIZE)
for (int ii = i; ii < min(i + TILE_SIZE, N); ii++)
for (int jj = j; jj < min(j + TILE_SIZE, N); jj++)
for (int kk = k; kk < min(k + TILE_SIZE, N); kk++)
C[ii][jj] += A[ii][kk] * B[kk][jj];
The tiled version improves cache utilization by operating on smaller chunks of data that fit better in the cache.
Prefetching
Prefetching involves loading data into the cache before it’s actually needed. This can be done through hardware prefetching or software prefetching. Here’s an example of software prefetching in C++:
#include <xmmintrin.h>
void prefetch_example(int* data, int size) {
for (int i = 0; i < size; i += 16) {
_mm_prefetch((char*)&data[i + 16], _MM_HINT_T0);
// Process data[i]
}
}
This code uses SSE instructions to prefetch data 16 elements ahead of the current processing position.
5. Effective Memory Management Strategies
Efficient memory management is crucial for optimizing both performance and resource utilization. Here are some key strategies:
Memory Pools
Memory pools (or object pools) can significantly reduce the overhead of frequent allocations and deallocations. Here’s a simple implementation in C++:
template <typename T, size_t PoolSize>
class MemoryPool {
private:
T data[PoolSize];
bool used[PoolSize] = {false};
public:
T* allocate() {
for (size_t i = 0; i < PoolSize; ++i) {
if (!used[i]) {
used[i] = true;
return &data[i];
}
}
return nullptr; // Pool is full
}
void deallocate(T* ptr) {
size_t index = ptr - data;
if (index < PoolSize) {
used[index] = false;
}
}
};
Smart Pointers
Smart pointers help manage memory automatically, reducing the risk of memory leaks. In C++, std::unique_ptr and std::shared_ptr are commonly used:
#include <memory>
class Resource {
// Resource implementation
};
void smart_pointer_example() {
std::unique_ptr<Resource> uniqueResource = std::make_unique<Resource>();
std::shared_ptr<Resource> sharedResource = std::make_shared<Resource>();
// Use resources...
// No need to manually delete, smart pointers handle cleanup
}
Memory-Mapped Files
Memory-mapped files can provide efficient access to file data by mapping a file directly to memory. Here’s an example using the mmap function in C:
#include <sys/mman.h>
#include <fcntl.h>
#include <unistd.h>
void mmap_example(const char* filename) {
int fd = open(filename, O_RDONLY);
if (fd == -1) {
// Handle error
return;
}
// Get file size
off_t size = lseek(fd, 0, SEEK_END);
lseek(fd, 0, SEEK_SET);
// Map file to memory
void* mapped = mmap(NULL, size, PROT_READ, MAP_PRIVATE, fd, 0);
if (mapped == MAP_FAILED) {
// Handle error
close(fd);
return;
}
// Use mapped memory...
// Cleanup
munmap(mapped, size);
close(fd);
}
6. Data Structures and Algorithms for Optimization
Choosing the right data structures and algorithms can have a significant impact on cache and memory performance. Let’s explore some cache-friendly data structures and algorithms:
Cache-Friendly Data Structures
Array-based Structures
Arrays and array-based structures like vectors offer excellent cache performance due to their contiguous memory layout. For example, std::vector in C++ is often preferred over std::list for better cache utilization:
#include <vector>
#include <list>
void data_structure_comparison() {
std::vector<int> vec(10000);
std::list<int> lst(10000);
// Iterating over vector is typically faster due to better cache utilization
for (int &v : vec) {
// Process v
}
// List iteration may cause more cache misses
for (int &l : lst) {
// Process l
}
}
Flat Data Structures
Flat data structures, which store related data in contiguous memory, can improve cache performance. For instance, an array of structures (AoS) can be transformed into a structure of arrays (SoA) for better cache utilization:
// Array of Structures (AoS)
struct Particle {
float x, y, z;
float vx, vy, vz;
};
std::vector<Particle> particles_aos;
// Structure of Arrays (SoA)
struct ParticleSystem {
std::vector<float> x, y, z;
std::vector<float> vx, vy, vz;
};
ParticleSystem particles_soa;
The SoA approach can lead to better cache utilization when processing specific attributes across all particles.
Cache-Aware Algorithms
Cache-Oblivious Algorithms
Cache-oblivious algorithms are designed to perform well without explicit knowledge of cache parameters. The classic example is the cache-oblivious matrix multiplication algorithm:
void cache_oblivious_matrix_multiply(float* A, float* B, float* C, int n, int row_a, int col_a, int row_b, int col_b, int row_c, int col_c) {
if (n <= 32) { // Base case: small enough to multiply directly
for (int i = 0; i < n; i++)
for (int j = 0; j < n; j++)
for (int k = 0; k < n; k++)
C[row_c + i * n + col_c + j] += A[row_a + i * n + col_a + k] * B[row_b + k * n + col_b + j];
} else {
int new_n = n / 2;
// Recursive calls for subdivided matrices
cache_oblivious_matrix_multiply(A, B, C, new_n, row_a, col_a, row_b, col_b, row_c, col_c);
cache_oblivious_matrix_multiply(A, B, C, new_n, row_a, col_a + new_n, row_b + new_n, col_b, row_c, col_c);
cache_oblivious_matrix_multiply(A, B, C, new_n, row_a, col_a, row_b, col_b + new_n, row_c, col_c + new_n);
cache_oblivious_matrix_multiply(A, B, C, new_n, row_a, col_a + new_n, row_b + new_n, col_b + new_n, row_c, col_c + new_n);
cache_oblivious_matrix_multiply(A, B, C, new_n, row_a + new_n, col_a, row_b, col_b, row_c + new_n, col_c);
cache_oblivious_matrix_multiply(A, B, C, new_n, row_a + new_n, col_a + new_n, row_b + new_n, col_b, row_c + new_n, col_c);
cache_oblivious_matrix_multiply(A, B, C, new_n, row_a + new_n, col_a, row_b, col_b + new_n, row_c + new_n, col_c + new_n);
cache_oblivious_matrix_multiply(A, B, C, new_n, row_a + new_n, col_a + new_n, row_b + new_n, col_b + new_n, row_c + new_n, col_c + new_n);
}
}
This algorithm recursively divides the matrices into smaller submatrices, naturally adapting to different cache sizes without explicit tuning.
Cache-Conscious Sorting
Traditional sorting algorithms can be adapted to be more cache-friendly. For example, a cache-conscious quicksort implementation might use a small-size insertion sort for partitions that fit in the cache:
#define INSERTION_SORT_THRESHOLD 16
void insertion_sort(int* arr, int left, int right) {
for (int i = left + 1; i <= right; i++) {
int key = arr[i];
int j = i - 1;
while (j >= left && arr[j] > key) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key;
}
}
void cache_conscious_quicksort(int* arr, int left, int right) {
if (right - left + 1 <= INSERTION_SORT_THRESHOLD) {
insertion_sort(arr, left, right);
} else {
// Standard quicksort partitioning
int pivot = arr[(left + right) / 2];
int i = left, j = right;
while (i <= j) {
while (arr[i] < pivot) i++;
while (arr[j] > pivot) j--;
if (i <= j) {
std::swap(arr[i], arr[j]);
i++;
j--;
}
}
// Recursive calls
if (left < j) cache_conscious_quicksort(arr, left, j);
if (i < right) cache_conscious_quicksort(arr, i, right);
}
}
This approach reduces cache misses for small subarrays that fit entirely in the cache.
7. Profiling and Measuring Performance
Effective optimization requires accurate measurement and profiling of your code’s performance. Here are some tools and techniques for profiling cache and memory usage:
Profiling Tools
- Valgrind: A powerful tool suite for debugging and profiling
- perf: Linux profiling tool with CPU counter statistics
- Intel VTune Profiler: Advanced profiling tool for Intel processors
- gprof: GNU profiler for call graph profiling
Measuring Cache Performance
To measure cache performance, you can use hardware performance counters. Here’s an example using the PAPI library in C:
#include <papi.h>
void measure_cache_performance() {
int events[2] = { PAPI_L1_DCM, PAPI_L2_DCM }; // L1 and L2 data cache misses
long long values[2];
PAPI_start_counters(events, 2);
// Your code to be measured here
PAPI_stop_counters(values, 2);
printf("L1 data cache misses: %lld\n", values[0]);
printf("L2 data cache misses: %lld\n", values[1]);
}
Memory Profiling
For memory profiling, tools like Valgrind’s Massif can be invaluable. Here’s how to use Massif:
$ valgrind --tool=massif ./your_program
$ ms_print massif.out.<pid> > massif_report.txt
This will generate a detailed report of your program’s heap memory usage over time.
8. Cache and Memory Optimization in Distributed Systems
In distributed systems, cache and memory optimization takes on new dimensions. Here are some key considerations:
Distributed Caching
Distributed caching systems like Redis or Memcached can significantly improve performance in distributed applications. Here’s a simple example using Redis with Python:
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
def get_user_data(user_id):
# Try to get data from cache
cached_data = r.get(f"user:{user_id}")
if cached_data:
return cached_data.decode('utf-8')
# If not in cache, fetch from database
data = fetch_from_database(user_id)
# Store in cache for future requests
r.setex(f"user:{user_id}", 3600, data) # Cache for 1 hour
return data
Cache Coherence
Maintaining cache coherence across distributed nodes is crucial. Techniques like write-through, write-back, and cache invalidation protocols are commonly used. Here’s a simplified example of a distributed cache with invalidation:
class DistributedCache:
def __init__(self):
self.local_cache = {}
self.node_id = get_node_id()
def get(self, key):
if key in self.local_cache:
return self.local_cache[key]
return None
def set(self, key, value):
self.local_cache[key] = value
broadcast_invalidation(key, self.node_id)
def invalidate(self, key):
if key in self.local_cache:
del self.local_cache[key]
def broadcast_invalidation(key, source_node):
for node in get_all_nodes():
if node != source_node:
node.invalidate(key)
Memory-Centric Computing
In-memory computing frameworks like Apache Spark optimize performance by keeping data in memory across a cluster. Here’s a simple Spark example in Python:
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName("MemoryCentricComputing").getOrCreate()
# Create an RDD and cache it in memory
data = spark.sparkContext.parallelize(range(1, 1000001))
cached_data = data.cache()
# Perform operations on the cached data
result = cached_data.map(lambda x: x * 2).reduce(lambda a, b: a + b)
print(f"Result: {result}")
spark.stop()
This example demonstrates how Spark can cache data in memory across a cluster for faster repeated access.
9. Language-Specific Optimization Techniques
Different programming languages offer various tools and techniques for cache and memory optimization. Let’s look at some language-specific approaches:
C++
C++ provides low-level control over memory, making it powerful for optimization:
Custom Allocators
You can create custom allocators to optimize memory allocation for specific use cases:
template <typename T>
class PoolAllocator {
private:
std::vector<T*> free_list;
std::vector<std::vector<T>> storage;
public:
T* allocate() {
if (free_list.empty()) {
storage.emplace_back(1000); // Allocate in chunks
for (auto &item : storage.back()) {
free_list.push_back(&item);
}
}
T* result = free_list.back();
free_list.pop_back();
return result;
}
void deallocate(T* ptr) {
free_list.push_back(ptr);
}
};
// Usage
PoolAllocator<int> allocator;
int* ptr = allocator.allocate();
// Use ptr...
allocator.deallocate(ptr);
std::vector Reserve
Using reserve() can prevent unnecessary reallocations:
std::vector<int> vec;
vec.reserve(10000); // Preallocate space for 10000 elements
for (int i = 0; i < 10000; ++i) {
vec.push_back(i); // No reallocation occurs
}
Java
Java’s automatic memory management can be optimized in several ways:
JVM Tuning
Adjusting JVM parameters can significantly impact performance:
java -Xms4g -Xmx4g -XX:+UseG1GC YourApplication
This sets the initial and maximum heap size to 4GB and uses the G1 garbage collector.
Off-Heap Memory
For large data sets, using off-heap memory can improve performance:
import java.nio.ByteBuffer;
ByteBuffer buffer = ByteBuffer.allocateDirect(1024 * 1024);
// Use buffer for direct memory access
Python
While Python abstracts much of memory management, there are still optimization techniques available:
NumPy for Efficient Array Operations
Using NumPy can significantly improve performance for numerical operations:
import numpy as np
# Efficient array creation and operations
arr = np.arange(1000000)
result = np.sum(arr * 2)
Memoization
Caching function results can improve performance for expensive computations:
from functools import lru_cache
@lru_cache(maxsize=None)
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
# Subsequent calls to fibonacci will use cached results
10. Real-World Examples and Case Studies
Let’s examine some real-world examples of cache and memory optimization in action:
Google’s BigTable
Google’s BigTable, a distributed storage system, uses a multi-level caching strategy:
- Tablet Server Cache: Caches frequently accessed data in memory
- Block Cache: Caches SSTable blocks to reduce disk I/O
- Bloom Filters: Reduce unnecessary disk reads for non-existent rows
This multi-tiered approach significantly reduces latency and improves throughput for read operations.
Facebook’s Memcached at Scale
Facebook’s use of Memcached demonstrates large-scale distributed caching:
- Consistent Hashing: Distributes cache keys across a large number of servers
- UDP Protocol: Uses UDP for get operations to reduce latency
- Lease Mechanism: Prevents thundering herds when cache misses occur
These optimizations allow Facebook to handle billions of cache operations per second.
Linux Kernel Page Cache
The Linux kernel’s page cache optimizes file system operations:
- Read-Ahead: Prefetches data that’s likely to be needed soon
- Write-Back: Defers writes to improve performance
- Unified Page Cache: Shares memory between file I/O and anonymous mappings
These features significantly improve I/O performance across the entire operating system.
11. Future Trends in Cache and Memory Optimization
As technology evolves, new trends are emerging in cache and memory optimization:
Non-Volatile Memory (NVM)
Technologies like Intel’s Optane are blurring the lines between storage and memory, offering new possibilities for data persistence and caching strategies.
Machine Learning for Cache Prediction
AI-driven approaches are being explored to predict cache usage patterns and optimize prefetching strategies dynamically.
Quantum Computing
While still in its infancy, quantum computing may revolutionize how we think about memory and caching, potentially solving certain optimization problems exponentially faster than classical computers.
Edge Computing
As computation moves closer to data sources in edge computing scenarios, new caching strategies are being developed to optimize data processing at the network edge.
12. Conclusion
Cache and memory optimization is a critical skill in the world of high-performance computing and large-scale systems. From understanding the basics of cache hierarchies to implementing advanced distributed caching strategies, the techniques covered in this guide form the foundation of efficient software design.
As you prepare for technical interviews or work on optimizing your own projects, remember that cache and memory optimization is often about finding the right balance. It’s not just about using the fastest data structures or the most advanced algorithms; it’s about understanding your specific use case, profiling your application, and making informed decisions based on real-world performance data.
The field of cache and memory optimization is constantly evolving, with new hardware technologies and software techniques emerging regularly. Stay curious, keep learning, and don’t be afraid to experiment with different approaches. Whether you’re aiming for a position at a top tech company or building the next big application, mastering these concepts will give you a significant advantage in creating efficient, scalable, and high-performance software systems.
Remember, the journey to optimization is ongoing. Each new project brings its own challenges and opportunities for improvement. By applying the principles and techniques discussed in this guide, you’ll be well-equipped to tackle these challenges and push the boundaries of what’s possible in software performance.