Understanding Boolean Logic and Its Application in Coding
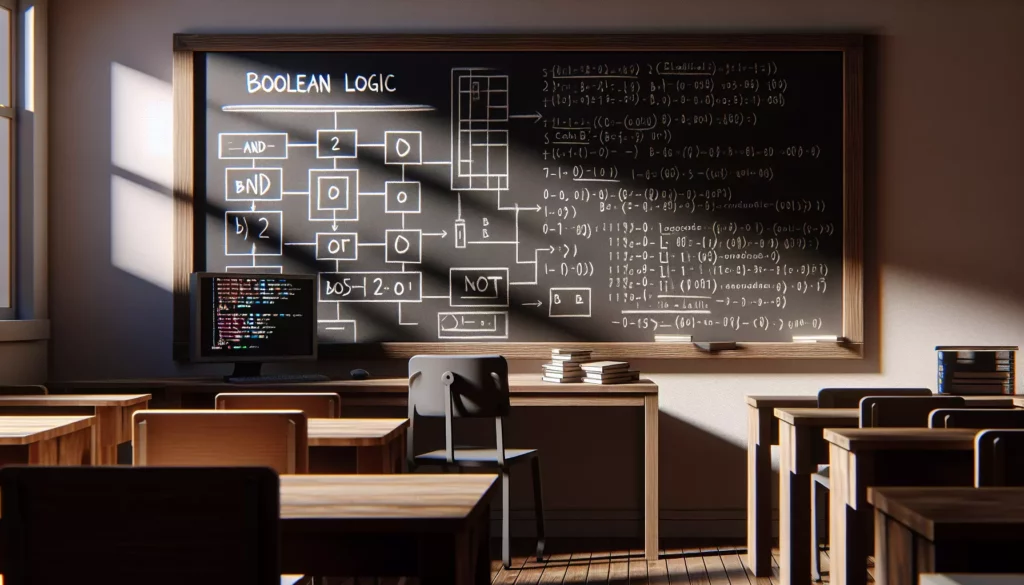
In the world of computer science and programming, Boolean logic forms the foundation of decision-making processes and conditional statements. Named after mathematician George Boole, Boolean logic is a branch of algebra that deals with true/false or yes/no values. Understanding Boolean logic is crucial for aspiring programmers and seasoned developers alike, as it plays a vital role in creating efficient and effective code.
In this comprehensive guide, we’ll explore the fundamentals of Boolean logic, its operations, and how it’s applied in various programming scenarios. We’ll also delve into more advanced topics like Boolean algebra and its relevance in modern computing.
Table of Contents
- Fundamentals of Boolean Logic
- Boolean Operations
- Truth Tables
- Boolean Logic in Programming
- Advanced Boolean Concepts
- Real-World Applications
- Boolean Logic and Code Optimization
- Boolean Logic in Technical Interviews
- Conclusion
1. Fundamentals of Boolean Logic
At its core, Boolean logic deals with two possible values: true and false. These values are often represented as 1 (true) and 0 (false) in computer systems. The simplicity of this binary system makes it incredibly powerful and efficient for computational processes.
In programming languages, Boolean values are typically represented by keywords such as true
and false
(in languages like Java, Python, and JavaScript) or True
and False
(in Python).
Boolean logic is based on several fundamental principles:
- Law of Excluded Middle: A proposition is either true or false, with no middle ground.
- Law of Noncontradiction: A proposition cannot be both true and false at the same time.
- Law of Identity: If a proposition is true, then it is true.
These principles form the basis for more complex Boolean operations and expressions.
2. Boolean Operations
Boolean logic involves several basic operations that can be combined to create more complex logical expressions. The three fundamental Boolean operations are:
2.1 AND Operation
The AND operation returns true only if both of its operands are true. It is often represented by the symbol “∧” or by the keyword “AND” in programming languages.
A AND B is true if and only if both A and B are true.
2.2 OR Operation
The OR operation returns true if at least one of its operands is true. It is represented by the symbol “∨” or by the keyword “OR” in programming languages.
A OR B is true if either A or B (or both) are true.
2.3 NOT Operation
The NOT operation is a unary operation that negates the value of its operand. It is represented by the symbol “¬” or by keywords like “NOT” or “!” in programming languages.
NOT A is true if A is false, and false if A is true.
These basic operations can be combined to create more complex logical expressions, which form the basis of decision-making in computer programs.
3. Truth Tables
Truth tables are a fundamental tool in Boolean logic for visualizing the outcomes of logical operations. They show all possible combinations of input values and their corresponding output values for a given logical expression.
3.1 AND Truth Table
A | B | A AND B |
---|---|---|
false | false | false |
false | true | false |
true | false | false |
true | true | true |
3.2 OR Truth Table
A | B | A OR B |
---|---|---|
false | false | false |
false | true | true |
true | false | true |
true | true | true |
3.3 NOT Truth Table
A | NOT A |
---|---|
false | true |
true | false |
Understanding truth tables is crucial for analyzing and designing logical circuits and for writing complex conditional statements in programming.
4. Boolean Logic in Programming
Boolean logic is extensively used in programming for decision-making, control flow, and data manipulation. Let’s explore some common applications:
4.1 Conditional Statements
Conditional statements like if-else use Boolean expressions to determine which code block should be executed.
if (age >= 18 AND hasValidID) {
allowPurchase = true;
} else {
allowPurchase = false;
}
4.2 Loops
Boolean conditions are often used to control loop execution:
while (isRunning AND health > 0) {
// Game loop code
}
4.3 Logical Operators
Most programming languages provide logical operators that correspond to Boolean operations:
- AND: usually represented by
&&
orand
- OR: usually represented by
||
oror
- NOT: usually represented by
!
ornot
if (isLoggedIn && (isAdmin || hasPermission)) {
// Allow access
}
4.4 Short-Circuit Evaluation
Many programming languages use short-circuit evaluation for Boolean expressions. This means that in an AND operation, if the first operand is false, the second operand is not evaluated because the result will always be false. Similarly, in an OR operation, if the first operand is true, the second operand is not evaluated.
if (checkCondition() && performExpensiveOperation()) {
// This code block is executed only if both conditions are true
}
In this example, performExpensiveOperation()
is only called if checkCondition()
returns true.
5. Advanced Boolean Concepts
As we delve deeper into Boolean logic, we encounter more advanced concepts that are particularly relevant in computer science and digital circuit design.
5.1 Boolean Algebra
Boolean algebra is a mathematical system that extends the basic principles of Boolean logic. It includes several laws and theorems that allow for the simplification and manipulation of Boolean expressions:
- Commutative Laws: A ∧ B = B ∧ A; A ∨ B = B ∨ A
- Associative Laws: (A ∧ B) ∧ C = A ∧ (B ∧ C); (A ∨ B) ∨ C = A ∨ (B ∨ C)
- Distributive Laws: A ∧ (B ∨ C) = (A ∧ B) ∨ (A ∧ C); A ∨ (B ∧ C) = (A ∨ B) ∧ (A ∨ C)
- Identity Laws: A ∧ 1 = A; A ∨ 0 = A
- Complement Laws: A ∧ ¬A = 0; A ∨ ¬A = 1
5.2 De Morgan’s Laws
De Morgan’s laws are particularly important in Boolean logic and digital circuit design. They state:
- ¬(A ∧ B) = ¬A ∨ ¬B
- ¬(A ∨ B) = ¬A ∧ ¬B
These laws are useful for simplifying complex Boolean expressions and are often used in circuit design and optimization.
5.3 Karnaugh Maps
Karnaugh maps (K-maps) are a graphical method for simplifying Boolean algebra expressions. They are particularly useful for expressions with up to four variables. K-maps allow for visual identification of patterns that can lead to simpler expressions.
5.4 Boolean Functions
Boolean functions are functions that take Boolean inputs and produce Boolean outputs. They can be represented using truth tables, Boolean expressions, or logic circuits. Understanding Boolean functions is crucial for designing digital circuits and optimizing logical expressions in programming.
6. Real-World Applications
Boolean logic finds applications in various fields beyond just programming:
6.1 Digital Circuit Design
Boolean logic is the foundation of digital circuit design. Logic gates like AND, OR, and NOT are physical implementations of Boolean operations. Complex digital systems, including the CPU in your computer, are built using combinations of these basic logic gates.
6.2 Database Queries
SQL and other database query languages use Boolean logic extensively. For example:
SELECT * FROM users WHERE age >= 18 AND (country = 'USA' OR country = 'Canada');
6.3 Search Engines
Boolean logic is used in search engines to refine and filter search results. Many search engines allow users to use operators like AND, OR, and NOT to create more precise search queries.
6.4 Artificial Intelligence
In AI and machine learning, Boolean logic is used in decision trees, rule-based systems, and certain types of neural network architectures.
7. Boolean Logic and Code Optimization
Understanding Boolean logic can lead to more efficient and optimized code. Here are some optimization techniques:
7.1 Simplifying Boolean Expressions
Complex Boolean expressions can often be simplified, leading to more efficient code. For example:
// Before optimization
if (a && b && c || a && b && !c) {
// Do something
}
// After optimization
if (a && b) {
// Do something
}
7.2 Short-Circuit Evaluation
As mentioned earlier, short-circuit evaluation can be used to optimize code by avoiding unnecessary computations:
if (quickCheck() && expensiveCheck()) {
// This is more efficient than checking both conditions always
}
7.3 De Morgan’s Laws in Practice
De Morgan’s laws can be used to simplify complex conditions:
// Before
if (!(a < 5 || b > 10)) {
// Do something
}
// After applying De Morgan's law
if (a >= 5 && b <= 10) {
// Do something
}
8. Boolean Logic in Technical Interviews
Boolean logic is a common topic in technical interviews, especially for roles involving software development or digital systems design. Here are some areas where Boolean logic might come up:
8.1 Logical Puzzles
Interviewers might present logical puzzles that require Boolean reasoning to solve. For example:
“You have three boxes labeled ‘Apples’, ‘Oranges’, and ‘Apples and Oranges’. All labels are incorrect. You can open only one box to look inside. How can you label all boxes correctly?”
Solving this puzzle requires applying Boolean logic to eliminate possibilities.
8.2 Truth Table Problems
You might be asked to construct truth tables for complex Boolean expressions or to simplify Boolean functions using truth tables.
8.3 Bitwise Operations
Boolean logic is closely related to bitwise operations, which are often tested in coding interviews. Understanding how to use AND, OR, and XOR at the bit level can be crucial for certain optimization problems.
8.4 Circuit Design Questions
For hardware-related roles, you might encounter questions about designing simple logic circuits or optimizing Boolean expressions for hardware implementation.
8.5 Conditional Logic in Coding Problems
Many coding problems implicitly test your understanding of Boolean logic through the use of complex conditional statements or loop conditions.
9. Conclusion
Boolean logic is a fundamental concept in computer science and programming that extends far beyond simple true/false statements. Its applications range from basic conditional statements in code to complex circuit design in hardware.
Understanding Boolean logic and its operations allows programmers to write more efficient and effective code, optimize algorithms, and solve complex logical problems. It’s a crucial skill for anyone looking to excel in software development, especially when preparing for technical interviews at major tech companies.
As you continue your journey in programming and computer science, remember that Boolean logic is not just a theoretical concept but a practical tool that you’ll use daily in your coding practice. Whether you’re designing a user interface, optimizing a database query, or developing a complex algorithm, the principles of Boolean logic will be your constant companions.
Keep practicing, experimenting with Boolean expressions, and challenging yourself with logical puzzles. The more you engage with these concepts, the more intuitive they’ll become, and the stronger your programming skills will grow.