Understanding Bitwise Operations and Their Uses in Programming
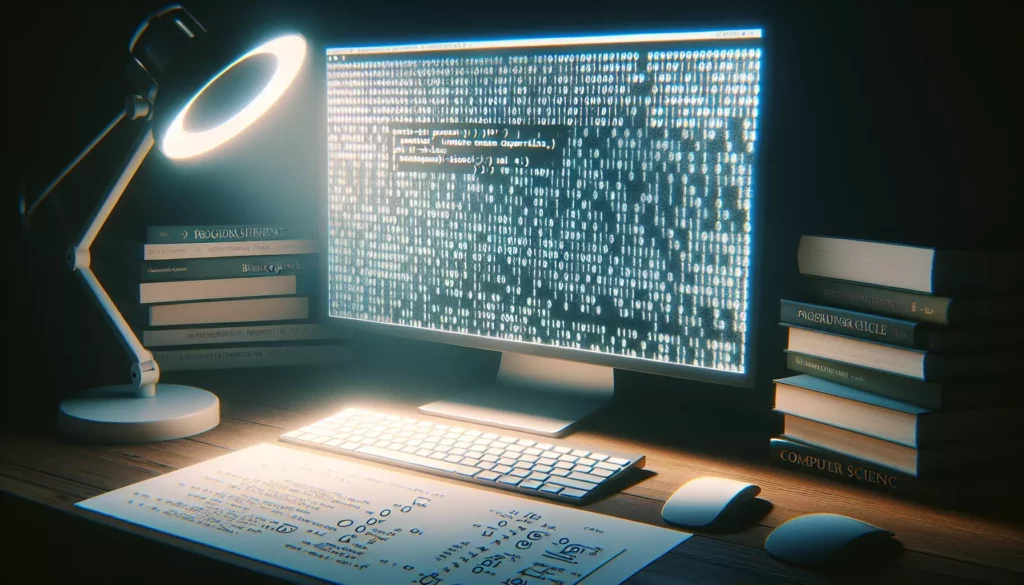
In the world of programming, bitwise operations are powerful tools that operate directly on the binary representations of numbers. While they may seem esoteric at first, mastering bitwise operations can lead to more efficient code and unlock solutions to complex problems. This article will delve into the intricacies of bitwise operations, their various uses, and how they can be applied in real-world programming scenarios.
What Are Bitwise Operations?
Bitwise operations are fundamental operations that work at the bit level of numbers. Instead of treating numbers as whole entities, these operations manipulate individual bits within the binary representation of those numbers. The most common bitwise operators are:
- AND (&)
- OR (|)
- XOR (^)
- NOT (~)
- Left Shift (<<)
- Right Shift (>>)
Let’s explore each of these operators in detail.
1. Bitwise AND (&)
The bitwise AND operator compares each bit of two numbers and returns 1 if both bits are 1, otherwise it returns 0. This operation is often used for masking or checking if a particular bit is set.
1010 (10 in decimal)
& 1100 (12 in decimal)
----
1000 (8 in decimal)
2. Bitwise OR (|)
The bitwise OR operator compares each bit of two numbers and returns 1 if at least one of the bits is 1, otherwise it returns 0. This operation is commonly used for combining flags or setting specific bits.
1010 (10 in decimal)
| 1100 (12 in decimal)
----
1110 (14 in decimal)
3. Bitwise XOR (^)
The bitwise XOR (exclusive OR) operator compares each bit of two numbers and returns 1 if the bits are different, otherwise it returns 0. XOR has interesting properties that make it useful for various operations, including simple encryption and toggling bits.
1010 (10 in decimal)
^ 1100 (12 in decimal)
----
0110 (6 in decimal)
4. Bitwise NOT (~)
The bitwise NOT operator inverts all the bits of a number, changing 0s to 1s and vice versa. It’s important to note that the result depends on the number of bits used to represent the number in your system.
~ 1010 (10 in decimal)
----
0101 (5 in decimal, assuming 4-bit representation)
5. Left Shift (<<)
The left shift operator moves all bits in a number to the left by a specified number of positions. This operation effectively multiplies the number by 2 raised to the power of the shift amount.
1010 << 1
----
10100 (20 in decimal)
6. Right Shift (>>)
The right shift operator moves all bits in a number to the right by a specified number of positions. This operation effectively divides the number by 2 raised to the power of the shift amount (for positive numbers).
1010 >> 1
----
0101 (5 in decimal)
Practical Applications of Bitwise Operations
Now that we’ve covered the basics of bitwise operations, let’s explore some practical applications where these operations shine.
1. Efficient Multiplication and Division
Left and right shift operations can be used for quick multiplication and division by powers of 2. This is often faster than traditional multiplication and division operations.
// Multiply by 2
int multiplyByTwo(int n) {
return n << 1;
}
// Divide by 2
int divideByTwo(int n) {
return n >> 1;
}
2. Checking Even/Odd Numbers
The bitwise AND operator can be used to efficiently check if a number is even or odd.
boolean isEven(int n) {
return (n & 1) == 0;
}
3. Swapping Variables Without a Temporary Variable
XOR can be used to swap two variables without using a temporary variable:
void swapWithoutTemp(int &a, int &b) {
a = a ^ b;
b = a ^ b;
a = a ^ b;
}
4. Setting, Clearing, and Toggling Bits
Bitwise operations are perfect for manipulating individual bits:
// Set the nth bit
int setBit(int num, int n) {
return num | (1 << n);
}
// Clear the nth bit
int clearBit(int num, int n) {
return num & ~(1 << n);
}
// Toggle the nth bit
int toggleBit(int num, int n) {
return num ^ (1 << n);
}
5. Bit Masking
Bit masking is a technique used to extract or manipulate specific bits within a number. It’s commonly used in graphics programming, network protocols, and embedded systems.
// Extract the last 4 bits of a number
int extractLastFourBits(int num) {
return num & 0xF; // 0xF is 1111 in binary
}
6. Efficient Power of 2 Checking
Bitwise operations can be used to efficiently check if a number is a power of 2:
boolean isPowerOfTwo(int n) {
return n > 0 && (n & (n - 1)) == 0;
}
Advanced Bitwise Techniques
As we delve deeper into bitwise operations, we encounter more advanced techniques that can significantly optimize certain algorithms and solve complex problems efficiently.
1. The Brian Kernighan Algorithm
This algorithm is used to count the number of set bits (1s) in a binary number efficiently:
int countSetBits(int n) {
int count = 0;
while (n) {
n &= (n - 1);
count++;
}
return count;
}
This algorithm works by repeatedly turning off the rightmost set bit until the number becomes zero.
2. Finding the Rightmost Set Bit
To find the position of the rightmost set bit in a number:
int findRightmostSetBit(int n) {
return log2(n & -n) + 1;
}
This uses the property that for any integer n, n & -n gives a number with only the rightmost set bit of n.
3. XOR Properties for Solving Unique Element Problems
XOR has some interesting properties that make it useful for solving problems involving finding unique elements:
- XOR of a number with itself is 0
- XOR of a number with 0 is the number itself
- XOR is associative and commutative
These properties can be used to solve problems like finding the single non-repeating element in an array where every other element appears twice:
int findSingleElement(int arr[], int n) {
int result = 0;
for (int i = 0; i < n; i++) {
result ^= arr[i];
}
return result;
}
4. Gray Code
Gray code is a sequence of numbers where adjacent numbers differ by only one bit. It can be generated using bitwise operations:
int grayCode(int n) {
return n ^ (n >> 1);
}
5. Bitwise Operations in Cryptography
Bitwise operations play a crucial role in many cryptographic algorithms. For example, the XOR operation is often used in simple encryption techniques:
string xorEncrypt(string text, char key) {
string result = text;
for (int i = 0; i < text.length(); i++) {
result[i] = text[i] ^ key;
}
return result;
}
// Decryption is the same operation
string xorDecrypt(string encryptedText, char key) {
return xorEncrypt(encryptedText, key);
}
Bitwise Operations in Different Programming Languages
While the concepts of bitwise operations remain the same across programming languages, the syntax and some specific behaviors may vary. Let’s look at how bitwise operations are implemented in some popular programming languages:
C/C++
C and C++ provide direct support for all bitwise operators:
#include <iostream>
using namespace std;
int main() {
int a = 5, b = 3;
cout << "AND: " << (a & b) << endl;
cout << "OR: " << (a | b) << endl;
cout << "XOR: " << (a ^ b) << endl;
cout << "NOT: " << (~a) << endl;
cout << "Left Shift: " << (a << 1) << endl;
cout << "Right Shift: " << (a >> 1) << endl;
return 0;
}
Python
Python also supports all bitwise operators, but uses different symbols for some operations:
a, b = 5, 3
print(f"AND: {a & b}")
print(f"OR: {a | b}")
print(f"XOR: {a ^ b}")
print(f"NOT: {~a}")
print(f"Left Shift: {a << 1}")
print(f"Right Shift: {a >> 1}")
Java
Java’s bitwise operators are similar to C/C++:
public class BitwiseDemo {
public static void main(String[] args) {
int a = 5, b = 3;
System.out.println("AND: " + (a & b));
System.out.println("OR: " + (a | b));
System.out.println("XOR: " + (a ^ b));
System.out.println("NOT: " + (~a));
System.out.println("Left Shift: " + (a << 1));
System.out.println("Right Shift: " + (a >> 1));
}
}
JavaScript
JavaScript supports bitwise operations, but it’s important to note that all numbers in JavaScript are floating-point, so bitwise operations convert them to 32-bit integers:
let a = 5, b = 3;
console.log("AND:", a & b);
console.log("OR:", a | b);
console.log("XOR:", a ^ b);
console.log("NOT:", ~a);
console.log("Left Shift:", a << 1);
console.log("Right Shift:", a >> 1);
console.log("Unsigned Right Shift:", a >>> 1);
Note that JavaScript has an additional unsigned right shift operator (>>>) that always fills with zeros.
Performance Considerations
While bitwise operations can lead to more efficient code in many cases, it’s important to consider the context and potential trade-offs:
Advantages:
- Speed: Bitwise operations are typically faster than their arithmetic counterparts, especially for simple operations like multiplication or division by powers of 2.
- Memory efficiency: Bitwise operations can be used to pack multiple boolean flags into a single integer, saving memory.
- Low-level control: They provide fine-grained control over individual bits, which is crucial in areas like embedded systems and device drivers.
Considerations:
- Readability: Code using bitwise operations can be less intuitive and harder to maintain if not well-documented.
- Portability: The behavior of bitwise operations, especially with signed integers, can vary across different systems and languages.
- Optimization: Modern compilers are often capable of optimizing arithmetic operations to use bitwise operations where appropriate, so manual optimization may not always be necessary.
Common Pitfalls and Best Practices
When working with bitwise operations, be aware of these common pitfalls and follow these best practices:
Pitfalls:
- Sign extension: Be careful with right shifts on signed integers, as the behavior can be platform-dependent.
- Overflow: Left shifts can easily cause overflow, especially when shifting by large amounts.
- Precedence: Bitwise operators often have lower precedence than arithmetic operators. Use parentheses to ensure correct order of operations.
Best Practices:
- Use constants: Define named constants for bit masks to improve readability.
- Comment your code: Clearly explain the purpose and logic behind complex bitwise operations.
- Test thoroughly: Bitwise operations can be tricky, so ensure comprehensive testing, especially for edge cases.
- Consider alternatives: Sometimes, using standard arithmetic or boolean operations might be clearer and just as efficient.
Conclusion
Bitwise operations are powerful tools in a programmer’s arsenal. They offer efficiency and elegance in solving certain types of problems, particularly in areas like low-level system programming, optimization, and algorithm design. While they may seem daunting at first, with practice and understanding, bitwise operations can become an invaluable part of your coding toolkit.
As you continue to develop your programming skills, remember that mastering bitwise operations is just one aspect of becoming a proficient coder. Platforms like AlgoCademy offer comprehensive resources and interactive tutorials to help you build a strong foundation in algorithmic thinking and problem-solving, which are crucial for excelling in technical interviews and real-world programming challenges.
Whether you’re preparing for interviews with major tech companies or simply looking to enhance your coding skills, understanding and effectively using bitwise operations can give you an edge in tackling complex problems efficiently. Keep practicing, exploring different applications, and don’t hesitate to incorporate these powerful operations into your code when appropriate.