Understanding Binary and Hexadecimal Number Systems: A Comprehensive Guide
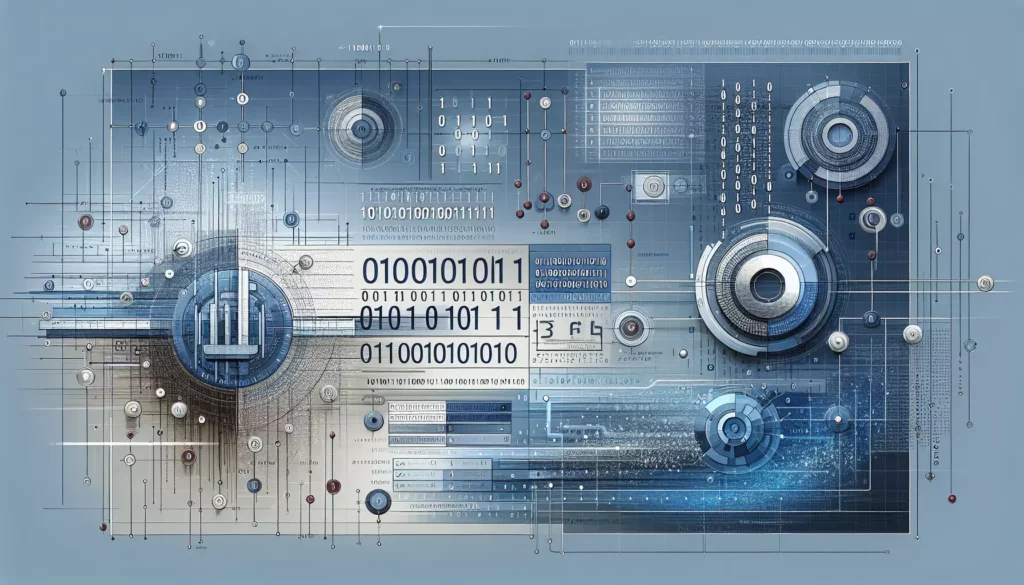
In the world of computer science and digital systems, understanding different number systems is crucial. While we’re accustomed to using the decimal system in our daily lives, computers operate on binary, and programmers often work with hexadecimal. This comprehensive guide will delve into the binary and hexadecimal number systems, exploring their fundamentals, applications, and importance in coding and computer science.
1. Introduction to Number Systems
Before we dive into binary and hexadecimal, let’s briefly review what a number system is. A number system is a way of representing numbers using a specific set of digits. The system we use in everyday life is the decimal system, which uses ten digits (0-9). However, computers and various areas of computer science utilize other number systems, primarily binary and hexadecimal.
2. The Binary Number System
2.1 What is Binary?
Binary is a base-2 number system that uses only two digits: 0 and 1. It’s the fundamental language of computers, as all data in computer systems is ultimately represented as sequences of these binary digits, or “bits”.
2.2 How Binary Works
In binary, each digit represents a power of 2, starting from the rightmost digit and increasing as we move left. For example:
1 0 1 1 0
^ ^ ^ ^ ^
| | | | |
| | | | 0 * 2^0 = 0
| | | 1 * 2^1 = 2
| | 1 * 2^2 = 4
| 0 * 2^3 = 0
1 * 2^4 = 16
Total: 16 + 0 + 4 + 2 + 0 = 22 (in decimal)
2.3 Converting Decimal to Binary
To convert a decimal number to binary, you can use the following steps:
- Divide the number by 2
- Keep track of the remainder (0 or 1)
- Repeat steps 1 and 2 with the quotient until the quotient becomes 0
- Read the remainders from bottom to top
Let’s convert the decimal number 22 to binary:
22 ÷ 2 = 11 remainder 0
11 ÷ 2 = 5 remainder 1
5 ÷ 2 = 2 remainder 1
2 ÷ 2 = 1 remainder 0
1 ÷ 2 = 0 remainder 1
Reading from bottom to top: 10110
2.4 Converting Binary to Decimal
To convert binary to decimal, multiply each digit by its corresponding power of 2 and sum the results, as shown in the earlier example.
2.5 Importance of Binary in Computing
Binary is crucial in computing for several reasons:
- Simplicity: Having only two states (on/off, true/false) makes it easy to implement in electronic circuits.
- Error detection: Binary makes it easier to detect and correct errors in data transmission.
- Efficiency: Binary calculations can be performed very quickly by computers.
3. The Hexadecimal Number System
3.1 What is Hexadecimal?
Hexadecimal is a base-16 number system that uses 16 distinct symbols. It uses the digits 0-9 and the letters A-F to represent values from 0 to 15.
3.2 How Hexadecimal Works
In hexadecimal, each digit represents a power of 16. The digits and their decimal equivalents are:
0 = 0 4 = 4 8 = 8 C = 12
1 = 1 5 = 5 9 = 9 D = 13
2 = 2 6 = 6 A = 10 E = 14
3 = 3 7 = 7 B = 11 F = 15
For example, the hexadecimal number 2A3 is calculated as:
2A3 (hex) = 2 * 16^2 + 10 * 16^1 + 3 * 16^0
= 512 + 160 + 3
= 675 (decimal)
3.3 Converting Decimal to Hexadecimal
To convert decimal to hexadecimal:
- Divide the number by 16
- Keep track of the remainder (0-15, using A-F for 10-15)
- Repeat steps 1 and 2 with the quotient until the quotient becomes 0
- Read the remainders from bottom to top
Let’s convert 675 to hexadecimal:
675 ÷ 16 = 42 remainder 3
42 ÷ 16 = 2 remainder 10 (A)
2 ÷ 16 = 0 remainder 2
Reading from bottom to top: 2A3
3.4 Converting Hexadecimal to Decimal
To convert hexadecimal to decimal, multiply each digit by its corresponding power of 16 and sum the results, as shown in the earlier example.
3.5 Importance of Hexadecimal in Computing
Hexadecimal is important in computing for several reasons:
- Compact representation: It can represent large numbers with fewer digits than decimal or binary.
- Easy conversion to binary: Each hexadecimal digit corresponds to exactly four binary digits.
- Common in programming: Used for color codes, memory addresses, and other computer-related contexts.
4. Relationship Between Binary and Hexadecimal
One of the main reasons hexadecimal is popular in computing is its easy conversion to and from binary. Each hexadecimal digit corresponds to exactly four binary digits (bits). This makes it a compact way to represent binary numbers.
Here’s a table showing the relationship:
Hex Binary Hex Binary
0 0000 8 1000
1 0001 9 1001
2 0010 A 1010
3 0011 B 1011
4 0100 C 1100
5 0101 D 1101
6 0110 E 1110
7 0111 F 1111
For example, the hexadecimal number 2A3 in binary is:
2 A 3
0010 1010 0011
5. Applications in Programming
5.1 Binary in Programming
Binary is fundamental to programming and is used in various ways:
- Bitwise operations: Manipulating individual bits in data.
- Data compression: Efficiently storing and transmitting data.
- Network protocols: Formatting data for transmission over networks.
Here’s an example of bitwise AND operation in Python:
a = 0b1010 # Binary representation of 10
b = 0b1100 # Binary representation of 12
c = a & b # Bitwise AND
print(bin(c)) # Output: 0b1000 (binary representation of 8)
5.2 Hexadecimal in Programming
Hexadecimal is widely used in programming for various purposes:
- Color codes: In web development, colors are often specified using hexadecimal.
- Memory addresses: In low-level programming, memory addresses are typically represented in hexadecimal.
- Debugging: Hexadecimal is often used in debugging output for its compactness.
Here’s an example of using hexadecimal color codes in CSS:
body {
background-color: #1A2B3C; /* Dark blue-gray */
color: #FFFFFF; /* White */
}
.highlight {
color: #FF4500; /* Orange-red */
}
6. Binary and Hexadecimal in Different Programming Languages
6.1 Python
Python provides built-in functions for working with binary and hexadecimal:
# Binary
binary_num = 0b1010 # Binary literal
print(bin(10)) # Convert to binary string
print(int('1010', 2)) # Convert binary string to int
# Hexadecimal
hex_num = 0xA3 # Hexadecimal literal
print(hex(163)) # Convert to hexadecimal string
print(int('A3', 16)) # Convert hexadecimal string to int
6.2 JavaScript
JavaScript also supports binary and hexadecimal literals and conversions:
// Binary
let binaryNum = 0b1010; // Binary literal
console.log(binaryNum.toString(2)); // Convert to binary string
console.log(parseInt('1010', 2)); // Convert binary string to int
// Hexadecimal
let hexNum = 0xA3; // Hexadecimal literal
console.log(hexNum.toString(16)); // Convert to hexadecimal string
console.log(parseInt('A3', 16)); // Convert hexadecimal string to int
6.3 Java
Java provides similar functionality:
// Binary
int binaryNum = 0b1010; // Binary literal
System.out.println(Integer.toBinaryString(10)); // Convert to binary string
System.out.println(Integer.parseInt("1010", 2)); // Convert binary string to int
// Hexadecimal
int hexNum = 0xA3; // Hexadecimal literal
System.out.println(Integer.toHexString(163)); // Convert to hexadecimal string
System.out.println(Integer.parseInt("A3", 16)); // Convert hexadecimal string to int
7. Practical Examples and Exercises
7.1 Binary to Decimal Converter
Here’s a Python function to convert binary to decimal:
def binary_to_decimal(binary_str):
decimal = 0
power = 0
for digit in binary_str[::-1]:
decimal += int(digit) * (2 ** power)
power += 1
return decimal
# Test the function
print(binary_to_decimal("1010")) # Should print 10
print(binary_to_decimal("11111111")) # Should print 255
7.2 Hexadecimal to RGB Converter
Here’s a JavaScript function to convert a hexadecimal color code to RGB:
function hexToRGB(hex) {
// Remove the # if present
hex = hex.replace(/^#/, '');
// Parse the hex values
let r = parseInt(hex.substr(0, 2), 16);
let g = parseInt(hex.substr(2, 2), 16);
let b = parseInt(hex.substr(4, 2), 16);
return `rgb(${r}, ${g}, ${b})`;
}
// Test the function
console.log(hexToRGB("#FF4500")); // Should print "rgb(255, 69, 0)"
8. Advanced Topics
8.1 Two’s Complement
Two’s complement is a method used to represent signed integers in binary. It allows for efficient addition and subtraction operations. To find the two’s complement of a binary number:
- Invert all the bits (change 0 to 1 and 1 to 0)
- Add 1 to the result
For example, to represent -5 in 8-bit two’s complement:
5 in binary: 00000101
Invert all bits: 11111010
Add 1: 11111011 (This is -5 in two's complement)
8.2 Floating-Point Representation
Computers use binary to represent floating-point numbers as well. The IEEE 754 standard defines how this is done. A floating-point number is represented using three components:
- Sign bit: Determines if the number is positive or negative
- Exponent: Stored in biased form
- Mantissa (or significand): Represents the precision bits of the number
Understanding this representation is crucial for dealing with precision issues in floating-point arithmetic.
9. Conclusion
Understanding binary and hexadecimal number systems is fundamental for anyone serious about computer science and programming. These systems form the backbone of how data is represented and manipulated in computers. From low-level operations to high-level programming concepts, a solid grasp of these number systems will enhance your ability to work effectively with computers and write efficient code.
As you continue your journey in programming and computer science, you’ll encounter these number systems repeatedly. Whether you’re debugging memory issues, working with color codes, or optimizing algorithms, the knowledge you’ve gained here will prove invaluable. Remember to practice converting between these systems and experiment with their applications in your preferred programming languages.
By mastering binary and hexadecimal, you’re not just learning about different ways to represent numbers – you’re gaining insight into the very language of computers. This understanding will serve as a strong foundation as you delve deeper into more advanced topics in computer science and software development.