Understanding Big O Notation: A Comprehensive Guide for Beginners
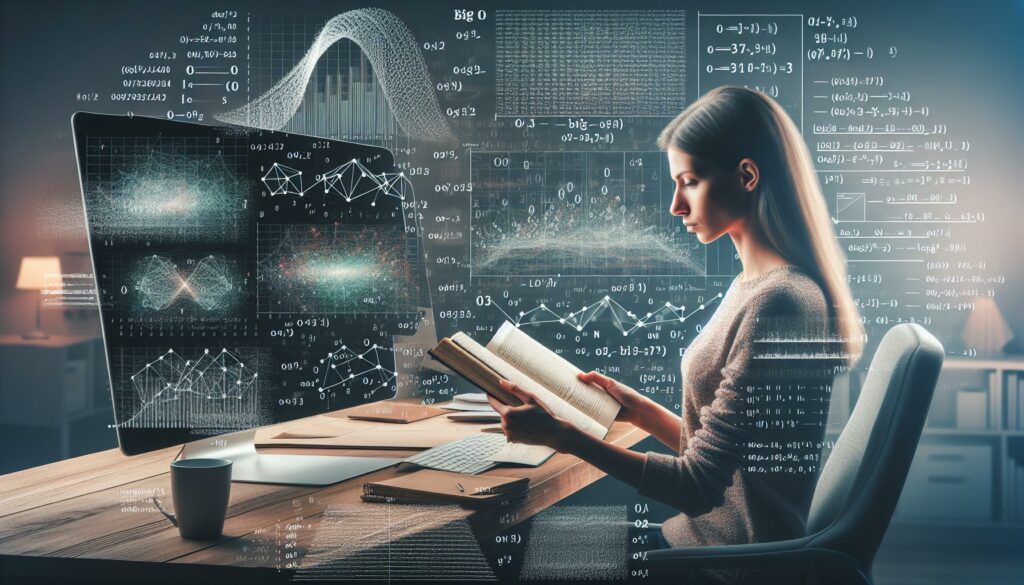
In the world of computer science and programming, efficiency is key. As you progress from writing simple programs to tackling complex algorithms, you’ll encounter the concept of Big O notation. This powerful tool is essential for analyzing and comparing the performance of different algorithms. In this comprehensive guide, we’ll demystify Big O notation, exploring its importance, common time complexities, and practical applications in coding interviews and real-world scenarios.
What is Big O Notation?
Big O notation is a mathematical notation used in computer science to describe the performance or complexity of an algorithm. Specifically, it describes the worst-case scenario, or the maximum time an algorithm will take to complete as the input size grows.
The “O” in Big O notation stands for “Order of,” which refers to the order of growth of the algorithm’s running time. It provides an upper bound on the growth rate of the algorithm’s time complexity in relation to the input size.
Why is Big O Notation Important?
Understanding Big O notation is crucial for several reasons:
- Algorithm Comparison: It allows developers to compare different algorithms and choose the most efficient one for a given problem.
- Scalability: It helps predict how an algorithm will perform as the input size increases, which is essential for building scalable applications.
- Optimization: By identifying the time complexity of an algorithm, developers can focus on optimizing the most critical parts of their code.
- Interview Preparation: Big O notation is a common topic in technical interviews, especially for positions at major tech companies.
Common Time Complexities
Let’s explore some of the most common time complexities you’ll encounter, ordered from best (most efficient) to worst (least efficient):
1. O(1) – Constant Time
An algorithm with O(1) complexity performs a fixed number of operations, regardless of the input size. This is the most efficient time complexity.
Example: Accessing an array element by index.
def get_element(arr, index):
return arr[index]
2. O(log n) – Logarithmic Time
Algorithms with O(log n) complexity divide the problem in half with each step. These are very efficient, especially for large datasets.
Example: Binary search in a sorted array.
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
3. O(n) – Linear Time
O(n) algorithms have a runtime directly proportional to the input size. They typically involve iterating through the input once.
Example: Finding the maximum element in an unsorted array.
def find_max(arr):
max_val = arr[0]
for num in arr:
if num > max_val:
max_val = num
return max_val
4. O(n log n) – Linearithmic Time
This complexity is common in efficient sorting algorithms. It’s more efficient than O(n^2) but less efficient than O(n).
Example: Merge sort algorithm.
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] <= right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
5. O(n^2) – Quadratic Time
Algorithms with O(n^2) complexity have nested iterations over the input. They become inefficient for large inputs.
Example: Bubble sort algorithm.
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
6. O(2^n) – Exponential Time
Exponential time algorithms have a runtime that doubles with each addition to the input. These are typically used for solving complex problems through recursion.
Example: Recursive calculation of Fibonacci numbers (naive approach).
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n - 1) + fibonacci(n - 2)
Analyzing Time Complexity
When analyzing the time complexity of an algorithm, follow these steps:
- Identify the input: Determine what variable represents the size of the input (usually denoted as n).
- Count the operations: Focus on the dominant operations that grow with the input size.
- Express in terms of n: Write the count of operations as a function of n.
- Simplify: Remove constants and lower-order terms, keeping only the highest-order term.
Let’s analyze the time complexity of a simple function:
def sum_and_product(arr):
total = 0
product = 1
for num in arr:
total += num
for num in arr:
product *= num
return total, product
Analysis:
- The input size is the length of the array, n.
- We have two separate loops, each iterating n times.
- The total number of operations is 2n + 2 (2 for the initializations).
- Simplifying, we remove the constant and lower-order terms, leaving us with O(n).
Therefore, the time complexity of this function is O(n).
Space Complexity
While we’ve focused on time complexity, it’s also important to consider space complexity. Space complexity refers to the amount of memory an algorithm uses relative to the input size.
The principles of Big O notation apply to space complexity as well. For example:
- O(1) space: The algorithm uses a constant amount of extra space.
- O(n) space: The algorithm uses extra space proportional to the input size.
- O(n^2) space: The algorithm uses extra space quadratic to the input size.
Example: Creating a matrix of size n x n
def create_matrix(n):
return [[0 for _ in range(n)] for _ in range(n)]
This function has a space complexity of O(n^2) because it creates a matrix with n^2 elements.
Best, Average, and Worst Case Scenarios
When discussing Big O notation, we typically focus on the worst-case scenario. However, it’s also valuable to understand best and average cases:
- Best Case: The minimum time an algorithm needs with the most optimal input.
- Average Case: The expected time an algorithm needs with a typical or random input.
- Worst Case: The maximum time an algorithm needs with the least optimal input.
For example, consider the linear search algorithm:
def linear_search(arr, target):
for i, num in enumerate(arr):
if num == target:
return i
return -1
- Best Case: O(1) – The target is the first element.
- Average Case: O(n) – The target is in the middle or distributed randomly.
- Worst Case: O(n) – The target is the last element or not in the array.
Common Pitfalls and Misconceptions
When working with Big O notation, be aware of these common pitfalls:
- Ignoring constants: While we drop constants in the final notation, they can be significant for smaller inputs.
- Overlooking space complexity: Always consider both time and space complexity when analyzing algorithms.
- Focusing only on the worst case: While worst-case analysis is important, understanding average-case performance can be crucial in practical scenarios.
- Premature optimization: Don’t always prioritize the algorithm with the best Big O notation without considering the specific context and requirements of your application.
Practical Applications in Coding Interviews
Understanding Big O notation is crucial for success in coding interviews, especially at major tech companies. Here are some tips for applying your knowledge during interviews:
- Analyze your solution: Always discuss the time and space complexity of your proposed solution.
- Optimize: If asked, try to optimize your initial solution and explain how it improves the time or space complexity.
- Compare alternatives: Discuss trade-offs between different approaches in terms of their Big O complexities.
- Understand the constraints: Consider the input size and any specific performance requirements mentioned in the problem statement.
Example Interview Question: “Find two numbers in an array that add up to a given target.”
Here are two possible solutions with different time complexities:
def two_sum_brute_force(arr, target):
n = len(arr)
for i in range(n):
for j in range(i + 1, n):
if arr[i] + arr[j] == target:
return [i, j]
return []
def two_sum_optimized(arr, target):
num_dict = {}
for i, num in enumerate(arr):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
The brute force approach has a time complexity of O(n^2), while the optimized solution using a hash table has a time complexity of O(n). In an interview, you’d be expected to explain these complexities and discuss the trade-offs between the two approaches.
Real-world Impact of Algorithm Efficiency
The efficiency of algorithms, as described by Big O notation, has significant real-world implications. Here are some examples:
- Search Engines: Efficient search algorithms are crucial for quickly finding relevant results among billions of web pages.
- Financial Systems: High-frequency trading systems require extremely fast algorithms to execute trades in microseconds.
- Social Media: Recommendation algorithms need to process vast amounts of data efficiently to provide personalized content.
- Navigation Apps: Routing algorithms must quickly find the optimal path among millions of possible routes.
Understanding Big O notation helps developers create scalable solutions that can handle growing datasets and user bases.
Conclusion
Big O notation is a fundamental concept in computer science that allows us to analyze and compare the efficiency of algorithms. By understanding time and space complexities, you can make informed decisions about algorithm selection and optimization in your software development projects.
As you continue your journey in programming and prepare for coding interviews, practice analyzing the time and space complexity of your solutions. This skill will not only help you in interviews but also in developing efficient, scalable software in your career.
Remember, while Big O notation is crucial, it’s just one aspect of algorithm analysis. Always consider the specific requirements of your project, including input sizes, hardware constraints, and the need for readability and maintainability in your code.
Keep practicing, analyzing, and optimizing your algorithms, and you’ll be well-prepared to tackle complex programming challenges and excel in your coding interviews!