Understanding Backtracking Algorithms: A Comprehensive Guide
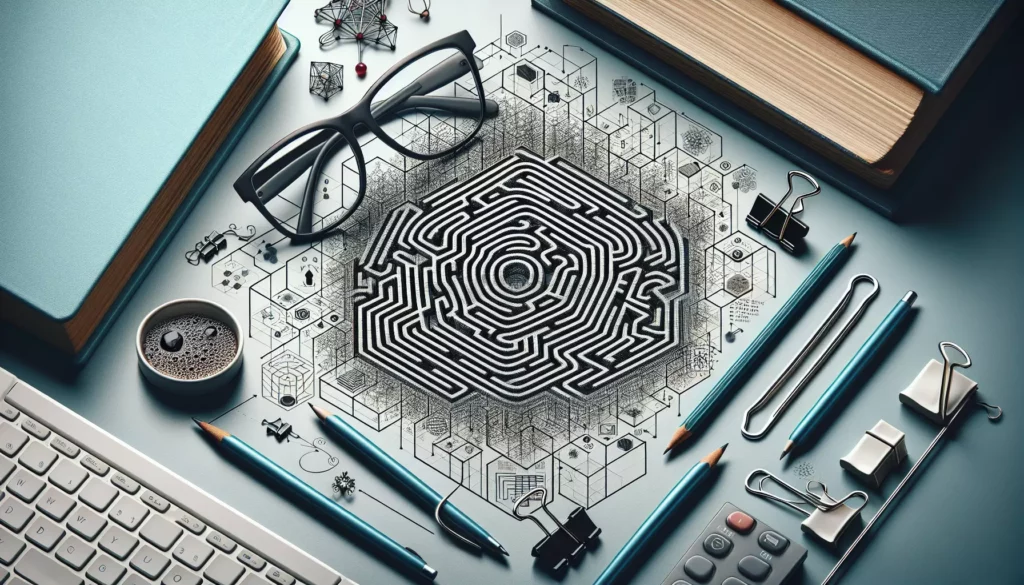
In the world of computer science and programming, algorithms play a crucial role in solving complex problems efficiently. Among the various algorithmic techniques, backtracking stands out as a powerful and versatile approach. This article will delve deep into the concept of backtracking algorithms, exploring their principles, applications, and implementation strategies.
What is Backtracking?
Backtracking is an algorithmic technique for solving problems recursively by trying to build a solution incrementally, one piece at a time, removing those solutions that fail to satisfy the constraints of the problem at any point of time (by time, here, is referred to the time elapsed till reaching any level of the search tree).
In simpler terms, backtracking can be thought of as a systematic way to try out different possibilities until you find a solution that works. It’s like solving a maze: you explore one path, and if it leads to a dead end, you backtrack and try another path.
Key Principles of Backtracking
- Choice: At each step, make a choice from the available options.
- Constraint: Check if the choice satisfies the problem constraints.
- Goal: Determine if the current state is a valid solution.
If a choice doesn’t work out, the algorithm “backtracks” to the previous state and tries a different choice. This process continues until a solution is found or all possibilities are exhausted.
The Backtracking Algorithm Template
While the specific implementation can vary depending on the problem, most backtracking algorithms follow a general template:
def backtrack(candidate):
if find_solution(candidate):
output(candidate)
return
for next_candidate in list_of_candidates:
if is_valid(next_candidate):
place(next_candidate)
backtrack(next_candidate)
remove(next_candidate)
This template encapsulates the core ideas of backtracking:
- Recursively explore candidates
- Check for validity before exploring further
- Place and remove candidates as you explore
Common Applications of Backtracking
Backtracking algorithms are used in a wide variety of problems. Some common applications include:
1. N-Queens Problem
The N-Queens problem involves placing N chess queens on an N×N chessboard so that no two queens threaten each other. This is a classic example of a constraint satisfaction problem solved using backtracking.
2. Sudoku Solver
Sudoku puzzles can be efficiently solved using backtracking. The algorithm tries filling digits sequentially and backtracks when it finds an invalid digit placement.
3. Maze Solving
Finding a path through a maze is another common application. The algorithm explores paths and backtracks when it hits a dead end.
4. Combinatorial Optimization
Many optimization problems, such as the Traveling Salesman Problem, can be approached using backtracking combined with other techniques.
Implementing a Backtracking Algorithm: The N-Queens Problem
Let’s implement a solution to the N-Queens problem to illustrate how backtracking works in practice.
def solve_n_queens(n):
def is_safe(board, row, col):
# Check if a queen can be placed on board[row][col]
# Check this row on left side
for i in range(col):
if board[row][i] == 1:
return False
# Check upper diagonal on left side
for i, j in zip(range(row, -1, -1), range(col, -1, -1)):
if board[i][j] == 1:
return False
# Check lower diagonal on left side
for i, j in zip(range(row, n, 1), range(col, -1, -1)):
if board[i][j] == 1:
return False
return True
def solve(board, col):
# Base case: If all queens are placed, return true
if col >= n:
return True
# Consider this column and try placing this queen in all rows one by one
for i in range(n):
if is_safe(board, i, col):
# Place this queen in board[i][col]
board[i][col] = 1
# Recur to place rest of the queens
if solve(board, col + 1):
return True
# If placing queen in board[i][col] doesn't lead to a solution,
# then remove queen from board[i][col]
board[i][col] = 0
# If the queen can't be placed in any row in this column col, return false
return False
# Initialize the board
board = [[0 for _ in range(n)] for _ in range(n)]
if solve(board, 0) == False:
print("Solution does not exist")
return False
# Print the solution
for i in range(n):
for j in range(n):
print(board[i][j], end=" ")
print()
return True
# Test the function
solve_n_queens(4)
This implementation demonstrates several key aspects of backtracking:
- The
is_safe
function checks if a queen can be safely placed in a given position. - The
solve
function recursively tries to place queens in each column. - If a placement doesn’t work, it backtracks by removing the queen and trying the next row.
Optimizing Backtracking Algorithms
While backtracking is powerful, it can be computationally expensive for large problem spaces. Here are some strategies to optimize backtracking algorithms:
1. Pruning
Pruning involves eliminating choices that are guaranteed not to lead to a solution. This can significantly reduce the search space.
2. Ordering
Choosing a good order to explore candidates can lead to finding solutions faster or failing quickly on invalid paths.
3. Constraint Propagation
In constraint satisfaction problems, propagating the effects of each choice can help identify invalid paths earlier.
4. Memoization
Storing and reusing the results of expensive computations can speed up the algorithm, especially when subproblems are repeated.
Backtracking vs. Other Algorithmic Techniques
It’s important to understand how backtracking compares to other problem-solving techniques:
Backtracking vs. Brute Force
While both explore all possibilities, backtracking is more efficient as it abandons paths that cannot lead to a solution.
Backtracking vs. Dynamic Programming
Dynamic programming is used when a problem has overlapping subproblems and an optimal substructure. Backtracking is more suitable for problems where you need to find all (or any) solutions.
Backtracking vs. Greedy Algorithms
Greedy algorithms make the locally optimal choice at each step. Backtracking, on the other hand, explores all possibilities and can find the globally optimal solution.
Common Pitfalls and How to Avoid Them
When implementing backtracking algorithms, be aware of these common issues:
1. Infinite Recursion
Ensure your base case is correctly defined and that you’re making progress towards it in each recursive call.
2. Inefficient State Management
Be careful about how you manage state changes. Inefficient state management can lead to incorrect results or poor performance.
3. Overlooking Constraints
Make sure you’re correctly checking all constraints. Missing a constraint can lead to invalid solutions.
4. Poor Choice of Data Structures
Choose appropriate data structures for your problem. The right data structure can significantly improve the efficiency of your algorithm.
Real-world Applications of Backtracking
Backtracking isn’t just a theoretical concept; it has numerous practical applications:
1. Artificial Intelligence
In game playing and puzzle-solving AI, backtracking is often used to explore possible moves and find optimal strategies.
2. Computer Networking
Routing algorithms in computer networks sometimes use backtracking to find optimal paths.
3. Bioinformatics
In DNA sequencing and protein folding problems, backtracking can be used to explore possible configurations.
4. Compiler Design
Backtracking is used in parsing and syntax analysis phases of compiler design.
Advanced Backtracking Techniques
As you become more comfortable with basic backtracking, consider these advanced techniques:
1. Iterative Deepening
This technique combines depth-first search’s space-efficiency with breadth-first search’s completeness.
2. Dance Links (Algorithm X)
Developed by Donald Knuth, this technique is particularly efficient for exact cover problems.
3. Backjumping
An optimization of backtracking that can jump back multiple levels when a conflict is detected.
Practicing Backtracking Problems
To master backtracking, practice is key. Here are some problems to try:
- Generate all permutations of a string
- Solve a Cryptarithmetic puzzle
- Find all possible words in a Boggle board
- Solve the Knight’s Tour problem
- Generate all possible valid parentheses pairs
These problems will help you develop a strong intuition for when and how to apply backtracking.
Conclusion
Backtracking is a powerful algorithmic technique that allows us to solve complex problems by systematically exploring all possible solutions. While it can be computationally expensive, various optimization techniques can make it highly efficient for many real-world applications.
As you continue your journey in algorithm design and problem-solving, backtracking will prove to be an invaluable tool in your arsenal. Remember, the key to mastering backtracking is practice and patience. Start with simple problems and gradually work your way up to more complex ones.
Happy coding, and may your algorithms always find their way back to the right solution!