Understanding Arrays and Their Applications in Coding Problems
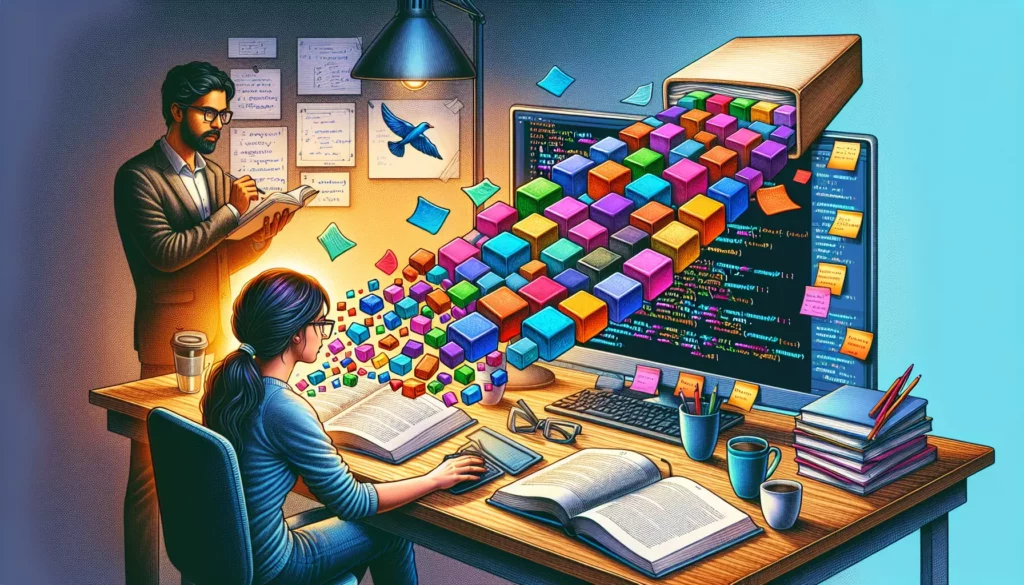
Arrays are fundamental data structures in computer programming that play a crucial role in solving various coding problems. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at major tech companies, having a solid understanding of arrays is essential. In this comprehensive guide, we’ll explore arrays in depth, discussing their properties, operations, and applications in solving common coding problems.
What are Arrays?
An array is a collection of elements of the same data type, stored in contiguous memory locations. Each element in an array can be accessed using an index, which typically starts from 0 in most programming languages. Arrays provide a way to store and organize multiple values under a single variable name, making it easier to manage and manipulate large sets of data.
Key Characteristics of Arrays:
- Fixed size (in most languages)
- Homogeneous elements (same data type)
- Random access (constant-time access to any element)
- Contiguous memory allocation
Array Declaration and Initialization
The syntax for declaring and initializing arrays varies slightly across programming languages. Let’s look at some examples in popular languages:
Java:
// Declaration
int[] numbers;
// Initialization
numbers = new int[5];
// Declaration and initialization in one line
int[] numbers = new int[5];
// Declaration and initialization with values
int[] numbers = {1, 2, 3, 4, 5};
Python:
# In Python, lists are used as dynamic arrays
numbers = [] # Empty list
numbers = [1, 2, 3, 4, 5] # List with initial values
JavaScript:
// Declaration and initialization
let numbers = [];
// Declaration and initialization with values
let numbers = [1, 2, 3, 4, 5];
C++:
// Declaration
int numbers[5];
// Declaration and initialization
int numbers[] = {1, 2, 3, 4, 5};
Basic Array Operations
Understanding how to perform basic operations on arrays is crucial for solving coding problems efficiently. Let’s explore some common operations:
1. Accessing Elements
Array elements are accessed using their index. Remember that array indices typically start at 0.
// Java
int[] numbers = {10, 20, 30, 40, 50};
int thirdElement = numbers[2]; // thirdElement = 30
// Python
numbers = [10, 20, 30, 40, 50]
third_element = numbers[2] # third_element = 30
// JavaScript
let numbers = [10, 20, 30, 40, 50];
let thirdElement = numbers[2]; // thirdElement = 30
2. Modifying Elements
You can change the value of an array element by assigning a new value to a specific index.
// Java
numbers[2] = 35; // {10, 20, 35, 40, 50}
// Python
numbers[2] = 35 # [10, 20, 35, 40, 50]
// JavaScript
numbers[2] = 35; // [10, 20, 35, 40, 50]
3. Finding Array Length
Knowing the length of an array is often necessary when working with loops or performing operations on all elements.
// Java
int length = numbers.length;
// Python
length = len(numbers)
// JavaScript
let length = numbers.length;
4. Iterating Through Arrays
Iterating through array elements is a common operation in many coding problems. Here are some ways to do it:
// Java
for (int i = 0; i < numbers.length; i++) {
System.out.println(numbers[i]);
}
// Enhanced for loop in Java
for (int num : numbers) {
System.out.println(num);
}
// Python
for num in numbers:
print(num)
// JavaScript
for (let i = 0; i < numbers.length; i++) {
console.log(numbers[i]);
}
// forEach method in JavaScript
numbers.forEach(num => console.log(num));
Common Array Problems and Solutions
Now that we’ve covered the basics, let’s explore some common coding problems involving arrays and how to solve them. These problems are often encountered in technical interviews and coding assessments.
1. Finding the Maximum Element in an Array
Problem: Given an array of integers, find the maximum element.
// Java
public static int findMax(int[] arr) {
if (arr == null || arr.length == 0) {
throw new IllegalArgumentException("Array is empty or null");
}
int max = arr[0];
for (int i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
// Python
def find_max(arr):
if not arr:
raise ValueError("Array is empty")
return max(arr)
// JavaScript
function findMax(arr) {
if (!arr || arr.length === 0) {
throw new Error("Array is empty or null");
}
return Math.max(...arr);
}
2. Reversing an Array
Problem: Reverse the elements of an array in-place.
// Java
public static void reverseArray(int[] arr) {
int left = 0;
int right = arr.length - 1;
while (left < right) {
int temp = arr[left];
arr[left] = arr[right];
arr[right] = temp;
left++;
right--;
}
}
// Python
def reverse_array(arr):
left, right = 0, len(arr) - 1
while left < right:
arr[left], arr[right] = arr[right], arr[left]
left += 1
right -= 1
// JavaScript
function reverseArray(arr) {
let left = 0;
let right = arr.length - 1;
while (left < right) {
[arr[left], arr[right]] = [arr[right], arr[left]];
left++;
right--;
}
}
3. Two Sum Problem
Problem: Given an array of integers and a target sum, find two numbers in the array that add up to the target sum.
// Java
public static int[] twoSum(int[] nums, int target) {
Map<Integer, Integer> map = new HashMap<>();
for (int i = 0; i < nums.length; i++) {
int complement = target - nums[i];
if (map.containsKey(complement)) {
return new int[] { map.get(complement), i };
}
map.put(nums[i], i);
}
throw new IllegalArgumentException("No two sum solution");
}
// Python
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
raise ValueError("No two sum solution")
// JavaScript
function twoSum(nums, target) {
const map = new Map();
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (map.has(complement)) {
return [map.get(complement), i];
}
map.set(nums[i], i);
}
throw new Error("No two sum solution");
}
Advanced Array Techniques
As you progress in your coding journey, you’ll encounter more complex array problems that require advanced techniques. Let’s explore some of these techniques:
1. Sliding Window Technique
The sliding window technique is used to perform operations on a specific window of elements in an array. It’s particularly useful for solving substring or subarray problems.
Example: Find the maximum sum of a subarray of size k
// Java
public static int maxSubarraySum(int[] arr, int k) {
if (arr == null || arr.length < k) {
throw new IllegalArgumentException("Invalid input");
}
int maxSum = 0;
int windowSum = 0;
// Calculate sum of first window
for (int i = 0; i < k; i++) {
windowSum += arr[i];
}
maxSum = windowSum;
// Slide the window and update maxSum
for (int i = k; i < arr.length; i++) {
windowSum = windowSum - arr[i - k] + arr[i];
maxSum = Math.max(maxSum, windowSum);
}
return maxSum;
}
2. Two Pointer Technique
The two pointer technique involves using two pointers to solve array problems efficiently. It’s often used in problems involving searching, reversing, or partitioning arrays.
Example: Remove duplicates from a sorted array
// Java
public static int removeDuplicates(int[] nums) {
if (nums == null || nums.length == 0) {
return 0;
}
int i = 0;
for (int j = 1; j < nums.length; j++) {
if (nums[j] != nums[i]) {
i++;
nums[i] = nums[j];
}
}
return i + 1;
}
3. Kadane’s Algorithm
Kadane’s algorithm is used to find the maximum subarray sum in an array. It’s an efficient solution to the maximum subarray problem.
// Java
public static int maxSubarraySum(int[] arr) {
int maxSoFar = arr[0];
int maxEndingHere = arr[0];
for (int i = 1; i < arr.length; i++) {
maxEndingHere = Math.max(arr[i], maxEndingHere + arr[i]);
maxSoFar = Math.max(maxSoFar, maxEndingHere);
}
return maxSoFar;
}
Multi-dimensional Arrays
Multi-dimensional arrays are arrays of arrays, allowing you to represent tables or matrices. They are commonly used in image processing, game development, and scientific computing.
Declaring and Initializing 2D Arrays
// Java
int[][] matrix = new int[3][4]; // 3 rows, 4 columns
// Initialize with values
int[][] matrix = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}
};
// Python
matrix = [
[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12]
]
// JavaScript
let matrix = [
[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12]
];
Accessing Elements in 2D Arrays
// Java
int element = matrix[1][2]; // Accesses the element in the 2nd row, 3rd column
// Python
element = matrix[1][2]
// JavaScript
let element = matrix[1][2];
Iterating Through 2D Arrays
// Java
for (int i = 0; i < matrix.length; i++) {
for (int j = 0; j < matrix[i].length; j++) {
System.out.print(matrix[i][j] + " ");
}
System.out.println();
}
// Python
for row in matrix:
for element in row:
print(element, end=" ")
print()
// JavaScript
for (let i = 0; i < matrix.length; i++) {
for (let j = 0; j < matrix[i].length; j++) {
console.log(matrix[i][j] + " ");
}
console.log();
}
Array-based Data Structures
Arrays serve as the foundation for many other data structures. Understanding arrays will help you grasp these more complex structures:
1. Dynamic Arrays (ArrayList in Java, List in Python)
Dynamic arrays automatically resize themselves when they reach capacity, providing more flexibility than fixed-size arrays.
// Java
ArrayList<Integer> dynamicArray = new ArrayList<>();
dynamicArray.add(1);
dynamicArray.add(2);
dynamicArray.add(3);
// Python
dynamic_array = []
dynamic_array.append(1)
dynamic_array.append(2)
dynamic_array.append(3)
// JavaScript
let dynamicArray = [];
dynamicArray.push(1);
dynamicArray.push(2);
dynamicArray.push(3);
2. Stacks
Stacks can be implemented using arrays, following the Last-In-First-Out (LIFO) principle.
// Java
class Stack {
private int[] array;
private int top;
private int capacity;
public Stack(int size) {
array = new int[size];
capacity = size;
top = -1;
}
public void push(int x) {
if (isFull()) {
throw new RuntimeException("Stack is full");
}
array[++top] = x;
}
public int pop() {
if (isEmpty()) {
throw new RuntimeException("Stack is empty");
}
return array[top--];
}
public boolean isEmpty() {
return top == -1;
}
public boolean isFull() {
return top == capacity - 1;
}
}
3. Queues
Queues can also be implemented using arrays, following the First-In-First-Out (FIFO) principle.
// Java
class Queue {
private int[] array;
private int front;
private int rear;
private int capacity;
private int count;
public Queue(int size) {
array = new int[size];
capacity = size;
front = 0;
rear = -1;
count = 0;
}
public void enqueue(int x) {
if (isFull()) {
throw new RuntimeException("Queue is full");
}
rear = (rear + 1) % capacity;
array[rear] = x;
count++;
}
public int dequeue() {
if (isEmpty()) {
throw new RuntimeException("Queue is empty");
}
int x = array[front];
front = (front + 1) % capacity;
count--;
return x;
}
public boolean isEmpty() {
return count == 0;
}
public boolean isFull() {
return count == capacity;
}
}
Array Performance and Time Complexity
Understanding the time complexity of array operations is crucial for writing efficient code and performing well in technical interviews. Here’s a quick overview of common array operations and their time complexities:
- Access by index: O(1)
- Insertion/deletion at the end: O(1)
- Insertion/deletion at the beginning: O(n)
- Searching in an unsorted array: O(n)
- Searching in a sorted array (binary search): O(log n)
Keep these time complexities in mind when choosing data structures and algorithms for your coding problems.
Conclusion
Arrays are fundamental data structures that play a crucial role in solving a wide range of coding problems. From basic operations to advanced techniques, understanding arrays is essential for any programmer, especially those preparing for technical interviews at major tech companies.
As you continue your coding journey, practice implementing various array operations and solving array-based problems. This will help you develop a strong foundation in algorithmic thinking and problem-solving skills. Remember that many complex data structures and algorithms build upon the concepts of arrays, so mastering arrays will give you a significant advantage in your programming career.
Keep practicing, exploring new problems, and challenging yourself with increasingly complex array manipulations. With time and dedication, you’ll become proficient in using arrays to solve a wide range of coding problems efficiently.