Understanding APIs: Building Blocks of Modern Applications
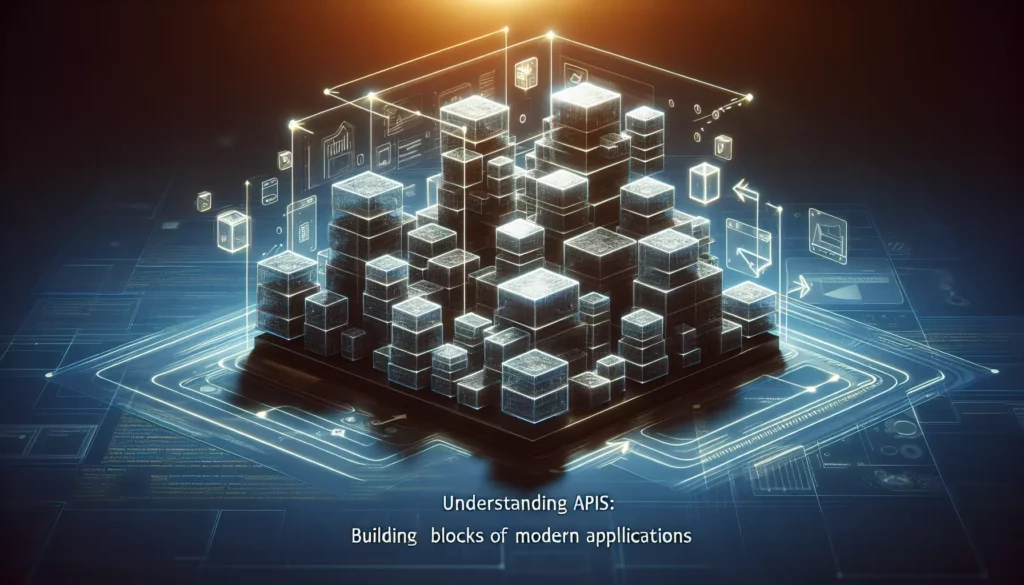
In today’s interconnected digital landscape, APIs (Application Programming Interfaces) have become the cornerstone of modern software development. They serve as the vital connectors that allow different applications, services, and systems to communicate and share data seamlessly. Whether you’re a beginner programmer or an experienced developer, understanding APIs is crucial for building robust, scalable, and efficient applications. In this comprehensive guide, we’ll dive deep into the world of APIs, exploring their importance, types, and how to work with them effectively.
What is an API?
An API, or Application Programming Interface, is a set of protocols, routines, and tools for building software applications. It specifies how software components should interact, allowing different applications to communicate with each other. Think of an API as a waiter in a restaurant – it takes your order (request), delivers it to the kitchen (server), and brings back your food (response).
APIs are used in various contexts, including:
- Web development
- Mobile app development
- Operating systems
- Database systems
- Hardware interactions
Why are APIs Important?
APIs play a crucial role in modern software development for several reasons:
- Integration: APIs allow different software systems to work together, enabling developers to integrate third-party services into their applications.
- Efficiency: By using APIs, developers can leverage existing functionality instead of building everything from scratch, saving time and resources.
- Scalability: APIs make it easier to scale applications by allowing modular development and efficient data exchange between components.
- Innovation: Open APIs encourage innovation by allowing developers to create new applications and services based on existing platforms.
- Consistency: APIs provide a standardized way for applications to interact, ensuring consistency across different implementations.
Types of APIs
There are several types of APIs, each serving different purposes and use cases:
1. Web APIs
Web APIs, also known as Web Services, are the most common type of APIs used in modern web development. They allow applications to communicate over the internet using standard web protocols. Some popular types of Web APIs include:
- REST (Representational State Transfer): A widely used architectural style for designing networked applications. RESTful APIs use HTTP methods like GET, POST, PUT, and DELETE to perform operations on resources.
- SOAP (Simple Object Access Protocol): A protocol that uses XML for exchanging structured data between systems. SOAP APIs are more rigid and formal compared to REST.
- GraphQL: A query language for APIs that allows clients to request specific data they need, reducing over-fetching and under-fetching of data.
2. Library-based APIs
These APIs are part of programming languages or software libraries. They provide a set of functions and procedures that developers can use to perform common tasks without writing the code from scratch. Examples include:
- Java API
- Python’s Standard Library
- C++ Standard Template Library (STL)
3. Operating System APIs
OS APIs provide a way for applications to interact with the operating system and hardware. They allow developers to access system resources, manage processes, and perform low-level operations. Examples include:
- Windows API
- POSIX (for Unix-like systems)
- macOS Cocoa API
4. Database APIs
Database APIs provide a way for applications to interact with databases, allowing them to perform operations like querying, inserting, updating, and deleting data. Examples include:
- SQL APIs (e.g., JDBC for Java, ADO.NET for .NET)
- NoSQL database APIs (e.g., MongoDB API, Cassandra API)
How APIs Work
To understand how APIs work, let’s break down the process into steps:
- Request: The client application sends a request to the API endpoint, specifying the desired action and any necessary parameters.
- Processing: The API receives the request and processes it, performing any required actions or calculations.
- Response: The API sends back a response to the client, typically in a standardized format like JSON or XML.
- Handling: The client application receives the response and processes the data as needed.
Let’s look at a simple example of how a REST API might work:
// Making a GET request to a REST API
fetch('https://api.example.com/users/123')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
In this example, we’re making a GET request to retrieve information about a user with ID 123. The API processes the request and returns the user data, which we then log to the console.
API Authentication and Security
Security is a crucial aspect of API design and usage. Most APIs require some form of authentication to ensure that only authorized users or applications can access the data. Common authentication methods include:
- API Keys: A unique identifier assigned to each client, typically included in the request header or as a query parameter.
- OAuth: An open standard for access delegation, commonly used for secure authorization in web applications.
- JWT (JSON Web Tokens): A compact, URL-safe means of representing claims to be transferred between two parties.
- Basic Auth: A simple authentication scheme built into the HTTP protocol, using a username and password.
Here’s an example of how you might include an API key in a request:
// Making a GET request with an API key
fetch('https://api.example.com/data', {
headers: {
'Authorization': 'Bearer YOUR_API_KEY_HERE'
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Best Practices for Working with APIs
When working with APIs, it’s important to follow best practices to ensure efficient and secure usage:
- Read the Documentation: Always start by thoroughly reading the API documentation to understand its capabilities, endpoints, and usage guidelines.
- Use HTTPS: Always use HTTPS to encrypt data transmitted between your application and the API.
- Handle Errors Gracefully: Implement proper error handling to manage API failures and unexpected responses.
- Implement Rate Limiting: Respect API rate limits and implement mechanisms to avoid exceeding them.
- Cache Responses: Implement caching strategies to reduce the number of API calls and improve performance.
- Validate Input: Always validate and sanitize user input before sending it to an API to prevent security vulnerabilities.
- Version Your APIs: If you’re building an API, use versioning to maintain backwards compatibility as you make changes.
Popular APIs and Their Uses
To give you a better idea of how APIs are used in real-world applications, let’s look at some popular APIs and their uses:
- Google Maps API: Allows developers to integrate Google Maps functionality into their applications, including features like geocoding, directions, and place searches.
- Twitter API: Enables developers to access Twitter data, post tweets, and interact with users programmatically.
- Stripe API: Provides functionality for processing payments, managing subscriptions, and handling other financial transactions in applications.
- Weather API (e.g., OpenWeatherMap): Offers access to weather data and forecasts for locations around the world.
- Spotify API: Allows developers to integrate Spotify’s music streaming functionality into their applications, including search, playback, and user data access.
Building Your Own API
As you become more comfortable with using APIs, you might want to create your own. Building an API involves several steps:
- Design: Plan your API’s structure, endpoints, and data models.
- Implementation: Write the code to handle requests and responses.
- Documentation: Create clear and comprehensive documentation for your API.
- Testing: Thoroughly test your API for functionality, performance, and security.
- Deployment: Host your API on a server or cloud platform.
- Maintenance: Regularly update and maintain your API to ensure its reliability and security.
Here’s a simple example of how you might create a basic REST API using Node.js and Express:
const express = require('express');
const app = express();
const port = 3000;
app.use(express.json());
let users = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' }
];
// GET all users
app.get('/users', (req, res) => {
res.json(users);
});
// GET a specific user
app.get('/users/:id', (req, res) => {
const user = users.find(u => u.id === parseInt(req.params.id));
if (!user) return res.status(404).send('User not found');
res.json(user);
});
// POST a new user
app.post('/users', (req, res) => {
const user = {
id: users.length + 1,
name: req.body.name
};
users.push(user);
res.status(201).json(user);
});
app.listen(port, () => {
console.log(`API running on port ${port}`);
});
This example creates a simple API with endpoints to get all users, get a specific user, and add a new user.
The Future of APIs
As technology continues to evolve, so do APIs. Some trends shaping the future of APIs include:
- Microservices Architecture: APIs are becoming more granular, with each microservice exposing its own API.
- Serverless Computing: APIs are increasingly being deployed as serverless functions, improving scalability and reducing costs.
- AI and Machine Learning Integration: APIs are being developed to provide easy access to AI and ML capabilities.
- IoT Integration: APIs are playing a crucial role in connecting and managing Internet of Things (IoT) devices.
- Real-time APIs: Technologies like WebSockets and Server-Sent Events are enabling real-time data exchange through APIs.
Conclusion
APIs are the unsung heroes of the digital world, powering the interconnected applications and services we use every day. As a developer, mastering the art of working with APIs is essential for building modern, efficient, and scalable applications. Whether you’re consuming third-party APIs or building your own, understanding the principles, best practices, and evolving trends in API development will set you up for success in your programming journey.
Remember, the world of APIs is vast and constantly evolving. Stay curious, keep learning, and don’t be afraid to experiment with different APIs to expand your skills and create innovative solutions. Happy coding!