Understanding API Security: Protecting Your Applications from Malicious Use
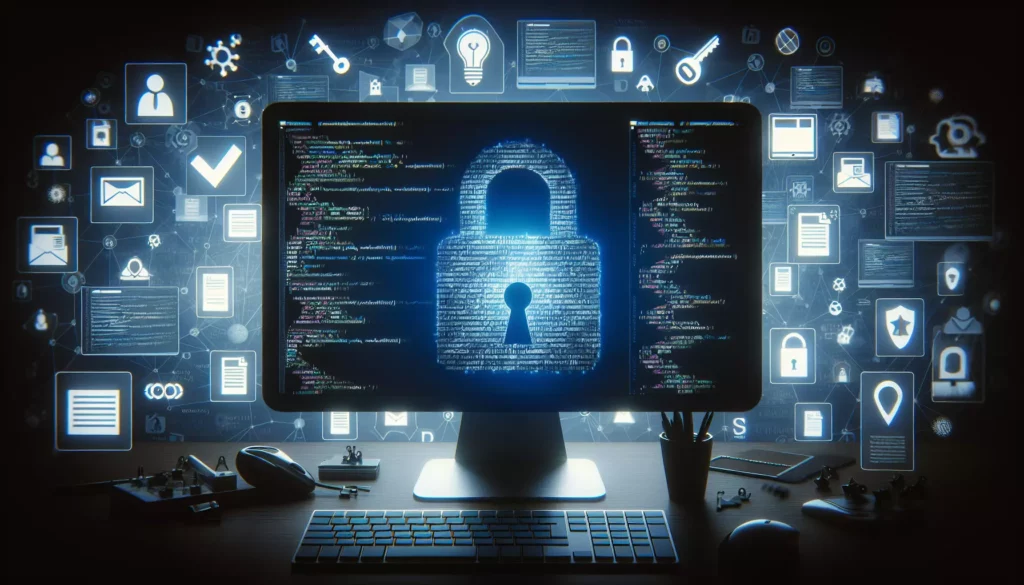
In today’s interconnected digital landscape, Application Programming Interfaces (APIs) have become the backbone of modern software development. They enable seamless communication between different applications, services, and systems, allowing developers to create robust and feature-rich software solutions. However, with the increasing reliance on APIs comes the critical need for robust security measures. In this comprehensive guide, we’ll dive deep into the world of API security, exploring why it’s crucial, common vulnerabilities, and best practices to protect your APIs from malicious use.
Table of Contents
- What is API Security?
- The Importance of API Security
- Common API Vulnerabilities
- Best Practices for API Security
- Authentication and Authorization
- Encryption and Data Protection
- Input Validation and Sanitization
- Rate Limiting and Throttling
- Monitoring and Logging
- API Security Testing
- Implementing API Gateways
- OAuth and OpenID Connect
- API Versioning and Deprecation
- Secure API Documentation
- Conclusion
What is API Security?
API security refers to the practices, protocols, and tools used to protect APIs from unauthorized access, data breaches, and other malicious activities. It encompasses a wide range of measures designed to ensure the confidentiality, integrity, and availability of the data and services exposed through APIs.
At its core, API security aims to:
- Prevent unauthorized access to sensitive data
- Protect against common attack vectors such as injection attacks and cross-site scripting
- Ensure the authenticity of API consumers
- Maintain the integrity of data transmitted through APIs
- Prevent API abuse and misuse
The Importance of API Security
As organizations increasingly rely on APIs to power their applications and services, the importance of API security cannot be overstated. Here are some key reasons why API security is crucial:
- Data Protection: APIs often handle sensitive data, including personal information, financial details, and proprietary business data. Securing APIs is essential to protect this valuable information from unauthorized access and potential breaches.
- Compliance: Many industries are subject to strict regulatory requirements, such as GDPR, HIPAA, or PCI DSS. Implementing robust API security measures is often necessary to comply with these regulations and avoid hefty fines.
- Reputation Management: A security breach can severely damage an organization’s reputation, leading to loss of customer trust and potential financial repercussions. Prioritizing API security helps maintain a positive brand image.
- Business Continuity: Secure APIs are less likely to be compromised or experience downtime due to attacks, ensuring uninterrupted service for users and maintaining business operations.
- Competitive Advantage: Organizations that prioritize API security can differentiate themselves in the market, attracting security-conscious customers and partners.
Common API Vulnerabilities
Before diving into best practices, it’s essential to understand the common vulnerabilities that can affect APIs. By recognizing these potential weak points, developers can better focus their security efforts:
- Broken Authentication: Weak or improperly implemented authentication mechanisms can allow attackers to gain unauthorized access to API endpoints.
- Lack of Rate Limiting: Without proper rate limiting, APIs can be vulnerable to brute-force attacks, denial-of-service (DoS) attacks, or excessive resource consumption.
- Insufficient Authorization: Even with proper authentication, inadequate authorization checks can lead to unauthorized access to sensitive data or functionality.
- Injection Attacks: SQL injection, XML injection, and other forms of injection attacks can exploit APIs that don’t properly sanitize user input.
- Sensitive Data Exposure: Improper handling of sensitive data, such as returning excessive information in API responses, can lead to data leaks.
- Cross-Site Scripting (XSS): APIs that don’t properly sanitize user input can be vulnerable to XSS attacks, especially in client-side applications consuming the API.
- Insufficient Logging and Monitoring: Lack of proper logging and monitoring can make it difficult to detect and respond to security incidents in a timely manner.
- Insecure Direct Object References (IDOR): Improper access controls can allow attackers to manipulate object references to access unauthorized resources.
Best Practices for API Security
Now that we’ve covered the importance of API security and common vulnerabilities, let’s explore best practices to protect your APIs from malicious use:
Authentication and Authorization
Implementing robust authentication and authorization mechanisms is crucial for API security:
- Use Strong Authentication: Implement multi-factor authentication (MFA) where possible, and use secure protocols like OAuth 2.0 or OpenID Connect.
- Implement Proper Authorization: Use role-based access control (RBAC) or attribute-based access control (ABAC) to ensure users can only access resources they’re authorized to use.
- Use Short-Lived Access Tokens: Implement token-based authentication with short expiration times to minimize the impact of token theft.
- Secure Token Storage: Store tokens securely on the client-side, using techniques like HTTP-only cookies or secure local storage.
Example of implementing JWT authentication in Node.js with Express:
const express = require('express');
const jwt = require('jsonwebtoken');
const app = express();
app.post('/login', (req, res) => {
// Authenticate user (not shown)
const user = { id: 1, username: 'example_user' };
const token = jwt.sign({ user }, 'your_jwt_secret', { expiresIn: '1h' });
res.json({ token });
});
function authenticateToken(req, res, next) {
const authHeader = req.headers['authorization'];
const token = authHeader && authHeader.split(' ')[1];
if (token == null) return res.sendStatus(401);
jwt.verify(token, 'your_jwt_secret', (err, user) => {
if (err) return res.sendStatus(403);
req.user = user;
next();
});
}
app.get('/protected', authenticateToken, (req, res) => {
res.json({ message: 'Protected resource accessed successfully' });
});
app.listen(3000, () => console.log('Server running on port 3000'));
Encryption and Data Protection
Protecting data in transit and at rest is essential for maintaining API security:
- Use HTTPS: Always use HTTPS to encrypt data in transit between clients and your API.
- Implement TLS: Use the latest version of TLS (currently TLS 1.3) to ensure secure communications.
- Encrypt Sensitive Data: Use strong encryption algorithms to protect sensitive data stored in databases or other storage systems.
- Use Secure Key Management: Implement proper key management practices, including regular key rotation and secure storage of encryption keys.
Example of setting up HTTPS in Node.js:
const https = require('https');
const fs = require('fs');
const express = require('express');
const app = express();
const options = {
key: fs.readFileSync('path/to/private-key.pem'),
cert: fs.readFileSync('path/to/certificate.pem')
};
https.createServer(options, app).listen(443, () => {
console.log('HTTPS server running on port 443');
});
Input Validation and Sanitization
Proper input validation and sanitization can prevent many common API vulnerabilities:
- Validate All Input: Implement strict input validation for all API parameters, including query strings, request bodies, and headers.
- Use Parameterized Queries: When interacting with databases, use parameterized queries to prevent SQL injection attacks.
- Sanitize Output: Properly encode or escape output to prevent XSS attacks, especially when returning user-generated content.
- Implement Content Security Policy (CSP): Use CSP headers to mitigate the risk of XSS and other injection attacks.
Example of input validation using Express-validator:
const express = require('express');
const { body, validationResult } = require('express-validator');
const app = express();
app.use(express.json());
app.post('/user',
body('username').isLength({ min: 5 }).trim().escape(),
body('email').isEmail().normalizeEmail(),
body('password').isLength({ min: 8 }),
(req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
// Process the validated input
res.json({ message: 'User created successfully' });
}
);
Rate Limiting and Throttling
Implementing rate limiting and throttling can protect your API from abuse and ensure fair usage:
- Set Rate Limits: Implement rate limiting to restrict the number of requests a client can make within a specific time frame.
- Use Throttling: Implement throttling to slow down requests from clients that are approaching their rate limits.
- Implement Backoff Algorithms: Use exponential backoff algorithms to handle retries and prevent overwhelming the API during high-load situations.
Example of implementing rate limiting in Express using the `express-rate-limit` middleware:
const express = require('express');
const rateLimit = require('express-rate-limit');
const app = express();
const limiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100 // limit each IP to 100 requests per windowMs
});
app.use(limiter);
app.get('/api', (req, res) => {
res.json({ message: 'Hello, World!' });
});
app.listen(3000, () => console.log('Server running on port 3000'));
Monitoring and Logging
Effective monitoring and logging are crucial for detecting and responding to security incidents:
- Implement Comprehensive Logging: Log all API access attempts, including successful and failed authentication attempts, as well as any suspicious activities.
- Use Secure Logging Practices: Ensure that logs are stored securely and cannot be tampered with.
- Implement Real-time Monitoring: Use monitoring tools to detect and alert on suspicious activities or potential security breaches in real-time.
- Regularly Review Logs: Establish a process for regularly reviewing logs to identify potential security issues or patterns of malicious behavior.
Example of implementing logging in Node.js using Winston:
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
defaultMeta: { service: 'user-service' },
transports: [
new winston.transports.File({ filename: 'error.log', level: 'error' }),
new winston.transports.File({ filename: 'combined.log' })
]
});
// Example usage in an Express route
app.post('/login', (req, res) => {
// ... authentication logic ...
if (authenticationSuccessful) {
logger.info('User logged in successfully', { userId: user.id });
res.json({ message: 'Login successful' });
} else {
logger.warn('Failed login attempt', { username: req.body.username });
res.status(401).json({ message: 'Authentication failed' });
}
});
API Security Testing
Regular security testing is essential to identify and address potential vulnerabilities in your API:
- Perform Regular Security Audits: Conduct thorough security audits of your API codebase and infrastructure.
- Implement Automated Security Testing: Use automated security testing tools to scan for common vulnerabilities regularly.
- Conduct Penetration Testing: Engage in regular penetration testing to identify potential weaknesses in your API security.
- Use Fuzzing Techniques: Implement fuzzing to test your API’s resilience against unexpected or malformed inputs.
Example of using OWASP ZAP for API security testing:
from zapv2 import ZAPv2
target = 'https://api.example.com'
api_key = 'your-zap-api-key'
# Initialize ZAP
zap = ZAPv2(apikey=api_key, proxies={'http': 'http://localhost:8080', 'https': 'http://localhost:8080'})
# Start the ZAP scanner
print('Starting ZAP scan on target: ' + target)
scan_id = zap.ascan.scan(target)
# Wait for the scan to complete
while int(zap.ascan.status(scan_id)) < 100:
print('Scan progress: ' + zap.ascan.status(scan_id) + '%')
time.sleep(5)
print('Scan completed')
# Retrieve and print the results
alerts = zap.core.alerts()
for alert in alerts:
print('Alert: ' + alert['alert'] + ' | Risk: ' + alert['risk'] + ' | URL: ' + alert['url'])
# Shutdown ZAP
zap.core.shutdown()
Implementing API Gateways
API gateways can provide an additional layer of security and management for your APIs:
- Centralize Authentication: Use API gateways to handle authentication and authorization, reducing the burden on individual API endpoints.
- Implement Traffic Management: Leverage API gateways for rate limiting, load balancing, and traffic shaping.
- Enable Monitoring and Analytics: Use API gateways to collect detailed metrics and logs for monitoring and analysis.
- Implement Security Policies: Apply security policies consistently across all APIs through the gateway.
Example of setting up an API gateway using Express Gateway:
const gateway = require('express-gateway');
gateway()
.load(path.join(__dirname, 'config'))
.run();
// In your config file (e.g., gateway.config.yml):
http:
port: 8080
apiEndpoints:
api:
host: localhost
paths: '/api/*'
serviceEndpoints:
backend:
url: 'http://localhost:3000'
policies:
- basic-auth
- cors
- expression
- key-auth
- log
- oauth2
- proxy
- rate-limit
pipelines:
default:
apiEndpoints:
- api
policies:
- key-auth:
- proxy:
- action:
serviceEndpoint: backend
changeOrigin: true
OAuth and OpenID Connect
Implementing OAuth 2.0 and OpenID Connect can significantly enhance API security:
- Use OAuth 2.0 for Authorization: Implement OAuth 2.0 to securely delegate access to resources without sharing credentials.
- Implement OpenID Connect: Use OpenID Connect on top of OAuth 2.0 to provide authentication and user information.
- Use Appropriate Grant Types: Choose the appropriate OAuth 2.0 grant type based on your application’s needs and security requirements.
- Implement PKCE: Use Proof Key for Code Exchange (PKCE) to enhance security for public clients using the Authorization Code flow.
Example of implementing OAuth 2.0 in Node.js using the `oauth2-server` package:
const express = require('express');
const OAuth2Server = require('oauth2-server');
const Request = OAuth2Server.Request;
const Response = OAuth2Server.Response;
const app = express();
const oauth = new OAuth2Server({
model: require('./model.js'),
accessTokenLifetime: 60 * 60,
allowBearerTokensInQueryString: true
});
app.use(express.json());
app.use(express.urlencoded({ extended: false }));
app.post('/oauth/token', (req, res) => {
const request = new Request(req);
const response = new Response(res);
oauth.token(request, response)
.then((token) => {
res.json(token);
})
.catch((err) => {
res.status(err.code || 500).json(err);
});
});
app.get('/secure', (req, res) => {
const request = new Request(req);
const response = new Response(res);
oauth.authenticate(request, response)
.then((token) => {
res.json({ message: 'Secure resource accessed successfully', user: token.user });
})
.catch((err) => {
res.status(err.code || 500).json(err);
});
});
app.listen(3000, () => console.log('Server running on port 3000'));
API Versioning and Deprecation
Proper API versioning and deprecation practices can help maintain security while evolving your API:
- Implement API Versioning: Use versioning to introduce changes without breaking existing integrations.
- Communicate Deprecations: Clearly communicate deprecation timelines and provide migration guides for users.
- Maintain Security for Older Versions: Ensure that security updates are applied to all supported API versions.
- Use Sunset Headers: Implement the `Sunset` HTTP header to indicate when an API version will be deprecated.
Example of implementing API versioning in Express:
const express = require('express');
const app = express();
const v1Router = express.Router();
const v2Router = express.Router();
v1Router.get('/users', (req, res) => {
res.json({ version: 'v1', users: ['Alice', 'Bob'] });
});
v2Router.get('/users', (req, res) => {
res.json({ version: 'v2', users: [{ name: 'Alice', id: 1 }, { name: 'Bob', id: 2 }] });
});
app.use('/api/v1', v1Router);
app.use('/api/v2', v2Router);
app.listen(3000, () => console.log('Server running on port 3000'));
Secure API Documentation
Providing secure and up-to-date API documentation is crucial for developers consuming your API:
- Use OpenAPI Specification: Document your API using the OpenAPI Specification (formerly Swagger) to provide clear and standardized documentation.
- Secure Documentation Access: Implement access controls for API documentation, especially for internal or partner-only APIs.
- Include Security Best Practices: Provide guidelines and best practices for securely consuming your API in the documentation.
- Keep Documentation Updated: Regularly update documentation to reflect changes in the API, including security-related updates.
Example of generating API documentation using Swagger UI Express:
const express = require('express');
const swaggerUi = require('swagger-ui-express');
const swaggerDocument = require('./swagger.json');
const app = express();
app.use('/api-docs', swaggerUi.serve, swaggerUi.setup(swaggerDocument));
// Your API routes go here
app.listen(3000, () => console.log('Server running on port 3000'));
Conclusion
Securing your APIs is a critical aspect of modern software development. By implementing the best practices outlined in this guide, you can significantly enhance the security of your APIs and protect them from malicious use. Remember that API security is an ongoing process that requires constant vigilance, regular updates, and a commitment to staying informed about the latest security threats and mitigation techniques.
As you continue to develop and maintain your APIs, keep these key takeaways in mind:
- Implement strong authentication and authorization mechanisms
- Use encryption to protect data in transit and at rest
- Validate and sanitize all input to prevent injection attacks
- Implement rate limiting and monitoring to detect and prevent abuse
- Regularly test and audit your APIs for security vulnerabilities
- Stay up-to-date with the latest security best practices and standards
By prioritizing API security, you not only protect your organization and users from potential threats but also build trust and reliability in your services. As the digital landscape continues to evolve, maintaining robust API security will remain a crucial factor in the success and longevity of your applications and services.