Understanding API Development and Integration: A Comprehensive Guide
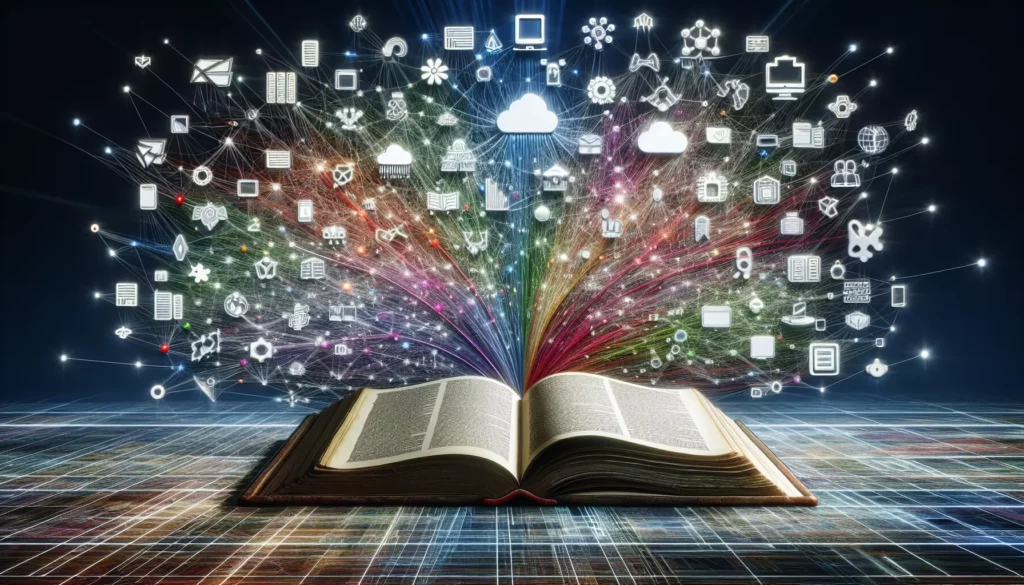
In today’s interconnected digital landscape, Application Programming Interfaces (APIs) play a crucial role in enabling seamless communication between different software systems. As a fundamental component of modern software development, understanding API development and integration is essential for aspiring programmers and seasoned developers alike. This comprehensive guide will delve into the world of APIs, exploring their importance, development process, and integration techniques.
Table of Contents
- What is an API?
- The Importance of APIs in Modern Software Development
- Types of APIs
- API Development Process
- API Design Best Practices
- API Security Considerations
- API Testing and Documentation
- API Integration Techniques
- API Management and Monitoring
- The Future of APIs
- Conclusion
What is an API?
An API, or Application Programming Interface, is a set of protocols, routines, and tools for building software applications. It specifies how software components should interact, allowing different applications to communicate with each other. In essence, an API acts as a messenger that takes requests, translates them, and returns responses.
APIs can be thought of as contracts between two applications, stipulating the kinds of requests and data exchanges that can be made between them. They abstract the underlying implementation and expose only the objects or actions the developer needs, simplifying programming by providing building blocks that can be easily assembled.
The Importance of APIs in Modern Software Development
APIs have become indispensable in modern software development for several reasons:
- Interoperability: APIs enable different software systems to work together, regardless of their underlying technologies or programming languages.
- Modularity: By using APIs, developers can build applications in a modular fashion, making it easier to update and maintain individual components without affecting the entire system.
- Efficiency: APIs allow developers to leverage existing functionalities instead of building everything from scratch, saving time and resources.
- Scalability: Well-designed APIs can handle growing amounts of work and users, making it easier for applications to scale.
- Innovation: By providing access to data and functionality, APIs foster innovation and enable developers to create new applications and services.
Types of APIs
There are several types of APIs, each serving different purposes and use cases:
1. Web APIs
Web APIs, also known as Web Services, are the most common type of APIs. They use HTTP protocols for communication and are designed to be consumed over the internet. Examples include REST (Representational State Transfer) and SOAP (Simple Object Access Protocol) APIs.
2. Library-based APIs
These APIs are collections of functions and procedures that can be used by other software. They are typically specific to a programming language or platform, such as the Java API or the .NET Framework API.
3. Operating System APIs
OS APIs allow applications to interact with the operating system’s services and resources. Examples include the Windows API and the POSIX API for Unix-based systems.
4. Database APIs
These APIs enable communication between an application and a database management system. Examples include JDBC for Java and ADO.NET for .NET applications.
API Development Process
Developing an API involves several key steps:
1. Planning and Design
Before writing any code, it’s crucial to plan the API’s structure, endpoints, and data models. This involves:
- Identifying the API’s purpose and target users
- Defining the resources and operations the API will support
- Choosing an appropriate architectural style (e.g., REST, GraphQL)
- Designing the API’s endpoints and request/response formats
2. Implementation
Once the design is finalized, the next step is to implement the API. This typically involves:
- Setting up the development environment
- Implementing the API’s endpoints and logic
- Handling data storage and retrieval
- Implementing error handling and validation
3. Testing
Thorough testing is crucial to ensure the API functions as intended. This includes:
- Unit testing individual components
- Integration testing to ensure different parts work together
- Performance testing to verify the API can handle expected loads
- Security testing to identify vulnerabilities
4. Documentation
Comprehensive documentation is essential for API adoption and usage. This should include:
- Detailed descriptions of all endpoints and their parameters
- Example requests and responses
- Authentication and authorization information
- Rate limiting and usage guidelines
5. Deployment and Maintenance
The final steps involve deploying the API to a production environment and maintaining it over time. This includes:
- Setting up hosting and infrastructure
- Implementing monitoring and logging
- Handling versioning and updates
- Providing support to API consumers
API Design Best Practices
Designing a good API is crucial for its success and adoption. Here are some best practices to follow:
1. Use Clear and Consistent Naming Conventions
Choose descriptive and intuitive names for your API endpoints, parameters, and response fields. Consistency in naming across your API will make it easier for developers to understand and use.
2. Follow REST Principles (for RESTful APIs)
If you’re designing a RESTful API, adhere to REST principles such as using appropriate HTTP methods (GET, POST, PUT, DELETE) and organizing resources hierarchically.
3. Use Proper HTTP Status Codes
Return appropriate HTTP status codes to indicate the result of API requests. For example, use 200 for successful requests, 201 for resource creation, 400 for bad requests, and 404 for not found resources.
4. Implement Versioning
Include versioning in your API to allow for future changes without breaking existing integrations. This can be done through URL versioning (e.g., /v1/users) or using headers.
5. Provide Comprehensive Error Handling
Return detailed error messages that help developers understand what went wrong and how to fix it. Include error codes, descriptions, and possibly links to further documentation.
6. Use Pagination for Large Data Sets
Implement pagination for endpoints that return large amounts of data to improve performance and reduce load on both the server and client.
7. Support HATEOAS (Hypermedia as the Engine of Application State)
For truly RESTful APIs, consider implementing HATEOAS to provide links to related resources in API responses, making the API more discoverable and self-documenting.
API Security Considerations
Security is a critical aspect of API development. Here are some key security considerations:
1. Authentication and Authorization
Implement robust authentication mechanisms such as OAuth 2.0 or JWT (JSON Web Tokens) to verify the identity of API consumers. Use authorization to control access to specific resources and operations.
2. Use HTTPS
Always use HTTPS to encrypt data in transit between the client and server, protecting against man-in-the-middle attacks and data interception.
3. Input Validation
Validate and sanitize all input data to prevent injection attacks and other security vulnerabilities. This includes checking data types, formats, and ranges.
4. Rate Limiting
Implement rate limiting to prevent abuse and ensure fair usage of your API. This can help protect against denial-of-service attacks and excessive resource consumption.
5. API Keys
Use API keys to identify and track API usage. While not a replacement for proper authentication, API keys can help with monitoring and controlling access.
6. Security Headers
Implement security headers such as Content Security Policy (CSP), X-XSS-Protection, and X-Frame-Options to enhance the security of your API and prevent common web vulnerabilities.
API Testing and Documentation
Thorough testing and comprehensive documentation are crucial for the success of any API. Let’s explore these aspects in more detail:
API Testing
API testing involves verifying the functionality, reliability, performance, and security of an API. Here are some key types of API tests:
- Functional Testing: Verifies that each API endpoint works as expected, returning the correct responses for various inputs.
- Integration Testing: Ensures that different components of the API work together correctly and that the API integrates properly with other systems.
- Performance Testing: Measures the API’s response times, throughput, and resource usage under various load conditions.
- Security Testing: Identifies vulnerabilities in the API, such as authentication bypasses, injection flaws, or data exposure.
- Usability Testing: Evaluates the API from a developer’s perspective, ensuring it’s easy to understand and integrate.
Popular tools for API testing include Postman, SoapUI, and JMeter. Additionally, many developers write automated tests using frameworks like Jest for JavaScript or pytest for Python.
API Documentation
Good API documentation is crucial for developer adoption and efficient use of your API. Here are key elements to include in your documentation:
- Overview: A high-level description of what the API does and its key features.
- Authentication: Detailed instructions on how to authenticate with the API.
- Endpoints: A list of all available endpoints, including their HTTP methods, parameters, and expected responses.
- Request/Response Examples: Sample requests and responses for each endpoint, preferably in multiple programming languages.
- Error Codes: A comprehensive list of possible error codes and their meanings.
- Rate Limits: Information about any rate limiting policies.
- Changelog: A record of changes and updates to the API.
- SDKs and Libraries: Links to any official SDKs or client libraries.
Tools like Swagger (OpenAPI) can help generate interactive API documentation that allows developers to test endpoints directly from the documentation page.
API Integration Techniques
Integrating an API into your application involves making HTTP requests to the API’s endpoints and handling the responses. Here are some common techniques and best practices for API integration:
1. Use HTTP Libraries
Most programming languages have libraries that simplify making HTTP requests. For example:
- JavaScript: Axios or Fetch API
- Python: Requests library
- Java: HttpClient or OkHttp
- C#: HttpClient class
2. Handle Authentication
Implement the API’s required authentication method, whether it’s API keys, OAuth tokens, or another mechanism. Store credentials securely and refresh tokens when necessary.
3. Implement Error Handling
Always handle potential errors from API calls. This includes network errors, API errors (e.g., 4xx and 5xx status codes), and parsing errors for the response data.
4. Use Asynchronous Calls
To prevent blocking the main thread of your application, use asynchronous API calls. Most modern programming languages and frameworks support asynchronous programming, such as Promises in JavaScript or async/await in Python and C#.
5. Implement Caching
To improve performance and reduce the number of API calls, implement caching for frequently accessed and relatively static data.
6. Rate Limiting and Throttling
Respect the API’s rate limits by implementing client-side throttling. This can involve tracking your request rate and pausing or queueing requests when approaching the limit.
7. Data Transformation
Often, you’ll need to transform the data received from an API to fit your application’s needs. Consider creating a layer that maps API responses to your internal data models.
Example: Making an API Request in JavaScript
Here’s a simple example of making an API request using the Fetch API in JavaScript:
async function fetchUserData(userId) {
try {
const response = await fetch(`https://api.example.com/users/${userId}`, {
headers: {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN'
}
});
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
const data = await response.json();
return data;
} catch (error) {
console.error('Error fetching user data:', error);
}
}
// Usage
fetchUserData(123).then(userData => {
console.log(userData);
}).catch(error => {
console.error('Error:', error);
});
API Management and Monitoring
As your API grows in usage and complexity, proper management and monitoring become crucial. Here are key aspects to consider:
1. API Gateways
An API gateway acts as a single entry point for all API calls, handling tasks like authentication, rate limiting, and request routing. Popular API gateway solutions include Amazon API Gateway, Kong, and Apigee.
2. Analytics and Monitoring
Implement robust logging and monitoring to track API usage, performance metrics, and errors. Tools like Prometheus, Grafana, or cloud-based solutions like AWS CloudWatch can help visualize and alert on these metrics.
3. Version Management
As your API evolves, manage different versions to prevent breaking changes for existing consumers. This might involve maintaining multiple versions of your API simultaneously or implementing a deprecation policy.
4. Developer Portal
Provide a dedicated portal for developers to access API documentation, test the API, manage their API keys, and get support. Platforms like Swagger Hub or ReadMe.io can help create comprehensive developer portals.
5. SLA Management
If you’re offering your API as a service, define and monitor Service Level Agreements (SLAs) to ensure you’re meeting promised uptime and performance metrics.
The Future of APIs
As technology continues to evolve, so do APIs. Here are some trends shaping the future of API development:
1. GraphQL
GraphQL is gaining popularity as an alternative to REST, offering more flexibility in data querying and reducing over-fetching of data.
2. Serverless APIs
Serverless architectures are becoming more common for API development, allowing for easier scaling and potentially lower costs.
3. AI-Enhanced APIs
APIs are increasingly incorporating AI capabilities, from natural language processing to predictive analytics.
4. IoT and APIs
As the Internet of Things (IoT) grows, APIs play a crucial role in enabling communication between devices and systems.
5. API-First Design
More organizations are adopting an API-first approach, designing APIs before implementing the underlying systems.
Conclusion
APIs have become the backbone of modern software development, enabling seamless integration between diverse systems and fostering innovation. Understanding API development and integration is crucial for any developer looking to build scalable, efficient, and interconnected applications.
As we’ve explored in this comprehensive guide, API development involves careful planning, design, implementation, and ongoing management. By following best practices in API design, prioritizing security, and leveraging appropriate tools for testing and documentation, developers can create robust and user-friendly APIs.
The world of APIs continues to evolve, with new technologies and paradigms emerging regularly. Staying informed about these trends and continuously improving your API development skills will be key to success in the ever-changing landscape of software development.
Remember, the journey of mastering API development and integration is ongoing. As you apply these concepts in real-world projects, you’ll gain valuable experience and insights that will further enhance your skills. Keep exploring, experimenting, and learning to stay at the forefront of this exciting field.