Understanding and Using APIs in Your Projects: A Comprehensive Guide
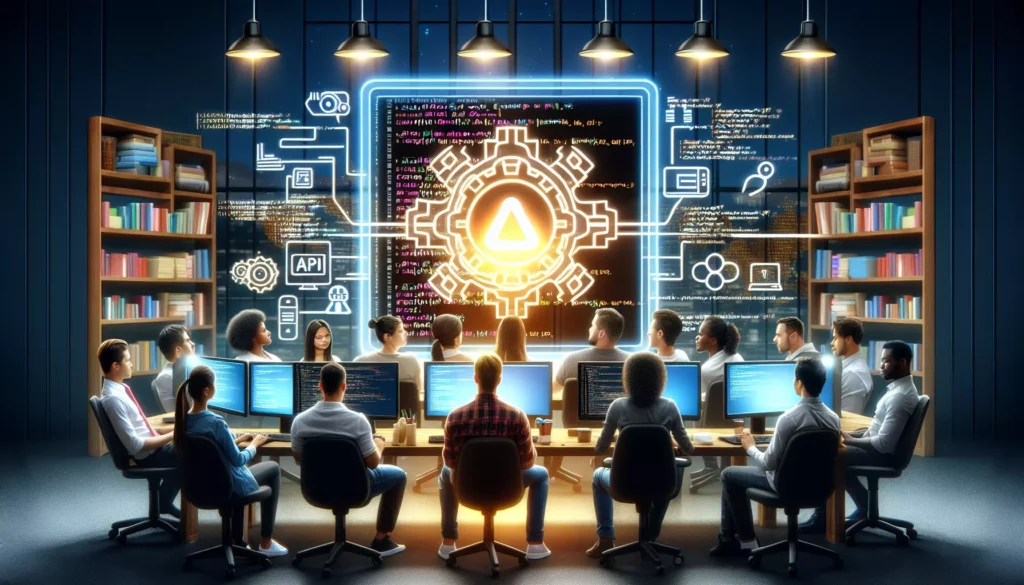
In today’s interconnected digital world, APIs (Application Programming Interfaces) have become an integral part of software development. Whether you’re a beginner just starting your coding journey or an experienced developer looking to enhance your skills, understanding and effectively using APIs can significantly boost your programming capabilities and open up new possibilities for your projects. In this comprehensive guide, we’ll dive deep into the world of APIs, exploring what they are, how they work, and how you can leverage them in your own projects.
Table of Contents
- What Are APIs?
- Types of APIs
- How APIs Work
- Benefits of Using APIs
- Getting Started with APIs
- Making API Requests
- Handling API Responses
- Authentication and Security
- Best Practices for Working with APIs
- Common Challenges and How to Overcome Them
- Popular APIs for Different Purposes
- Creating Your Own API
- Testing and Debugging API Integrations
- The Future of APIs
- Conclusion
1. What Are APIs?
An API, or Application Programming Interface, is a set of protocols, routines, and tools that define how software components should interact. In simpler terms, it’s a way for different software applications to communicate with each other. APIs act as intermediaries, allowing developers to access specific features or data from other applications or services without needing to understand the intricate details of their internal workings.
Think of an API as a waiter in a restaurant. You, the customer (or in this case, the developer), don’t need to know how the kitchen operates to order food. You simply tell the waiter (the API) what you want, and they bring it to you. The API handles the complexities of the request, communicates with the necessary systems, and returns the requested information or performs the desired action.
2. Types of APIs
There are several types of APIs, each serving different purposes and operating in various contexts:
- Web APIs: These are the most common type, accessible over the internet using HTTP protocols. Examples include RESTful APIs and SOAP APIs.
- Library-based APIs: These are collections of functions and procedures that can be used in software development. For instance, the Java API or the Python standard library.
- Operating System APIs: These allow applications to interact with the operating system, such as the Windows API or POSIX.
- Database APIs: These provide a way for applications to communicate with databases, like JDBC for Java or ADO.NET for .NET.
- Remote APIs: These allow interactions between different systems over a network, often used in distributed computing.
For most developers working on web and mobile applications, Web APIs are the most frequently encountered and utilized type.
3. How APIs Work
At a high level, APIs work through a series of requests and responses:
- Request: The client application sends a request to the API, typically specifying an endpoint (a URL) and including any necessary parameters or data.
- Processing: The API receives the request and processes it, often interacting with databases or other systems to fulfill the request.
- Response: The API sends back a response, usually in a standardized format like JSON or XML, containing the requested data or confirmation of the action performed.
- Client-side handling: The client application receives the response and processes it accordingly, often displaying the data to the user or using it for further operations.
This process is typically facilitated through HTTP methods such as GET (retrieve data), POST (send data), PUT (update data), and DELETE (remove data).
4. Benefits of Using APIs
Integrating APIs into your projects offers numerous advantages:
- Efficiency: APIs allow you to leverage existing services and functionalities without reinventing the wheel.
- Scalability: By using APIs, you can easily scale your application by integrating more powerful services as needed.
- Flexibility: APIs enable you to add or modify features without overhauling your entire application.
- Integration: They facilitate seamless integration between different systems and services.
- Specialization: You can focus on your core functionality while relying on specialized services for other features.
- Cost-effectiveness: Using APIs can be more cost-effective than developing and maintaining all functionalities in-house.
- Innovation: APIs open up possibilities for creative combinations of services, fostering innovation.
5. Getting Started with APIs
To begin working with APIs, follow these steps:
- Choose an API: Select an API that provides the functionality you need for your project. Popular choices for beginners include weather APIs, social media APIs, or public data APIs.
- Read the Documentation: Thoroughly review the API’s documentation to understand its endpoints, request formats, and authentication requirements.
- Get API Keys: Most APIs require authentication. Sign up for an account and obtain the necessary API keys or tokens.
- Choose a Programming Language: While you can work with APIs in almost any programming language, some popular choices include Python, JavaScript, and Ruby due to their simplicity and extensive libraries for HTTP requests.
- Set Up Your Development Environment: Install any necessary libraries or tools for making HTTP requests in your chosen language.
6. Making API Requests
Let’s look at an example of making a simple API request using Python and the popular requests
library:
import requests
# API endpoint URL
url = "https://api.example.com/data"
# Parameters for the request
params = {
"key": "your_api_key",
"query": "example"
}
# Make the GET request
response = requests.get(url, params=params)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON response
data = response.json()
print(data)
else:
print(f"Error: {response.status_code}")
This example demonstrates a basic GET request to an API. It includes parameters such as an API key and a query, which are common in many API requests.
7. Handling API Responses
API responses typically come in standardized formats, with JSON being the most common. Here’s how you might handle and process a JSON response:
import json
# Assuming 'response' is the API response from the previous example
if response.status_code == 200:
data = response.json()
# Access specific data from the response
if "results" in data:
for item in data["results"]:
print(f"Title: {item['title']}")
print(f"Description: {item['description']}")
print("---")
else:
print(f"Error: {response.status_code}")
print(response.text) # Print the error message if available
This code snippet demonstrates how to parse a JSON response, check for the presence of expected data, and iterate through results. It’s crucial to include error handling to manage cases where the API request fails or returns unexpected data.
8. Authentication and Security
Most APIs require some form of authentication to ensure secure access. Common authentication methods include:
- API Keys: A unique identifier sent with each request.
- OAuth: A protocol that allows secure authorization without sharing credentials.
- JWT (JSON Web Tokens): A compact, URL-safe means of representing claims to be transferred between two parties.
Here’s an example of using an API key in a request header:
import requests
url = "https://api.example.com/data"
headers = {
"Authorization": "Bearer YOUR_API_KEY"
}
response = requests.get(url, headers=headers)
Always keep your API keys and tokens secure. Never expose them in client-side code or public repositories.
9. Best Practices for Working with APIs
To effectively use APIs in your projects, consider these best practices:
- Read the Documentation Thoroughly: Understand the API’s capabilities, limitations, and any usage restrictions.
- Handle Rate Limits: Many APIs have rate limits. Implement proper handling to avoid exceeding these limits.
- Implement Error Handling: Always include robust error handling to manage API failures gracefully.
- Cache Responses: When appropriate, cache API responses to reduce the number of requests and improve performance.
- Use HTTPS: Always use HTTPS for secure communication with APIs.
- Versioning: Pay attention to API versions and update your code when necessary.
- Asynchronous Requests: For better performance, consider using asynchronous requests, especially when dealing with multiple API calls.
10. Common Challenges and How to Overcome Them
Working with APIs can present several challenges. Here are some common ones and strategies to address them:
- Rate Limiting: Implement backoff strategies and request queuing to manage rate limits effectively.
- API Changes: Stay updated with API documentation and implement version checking in your code.
- Data Inconsistencies: Implement robust data validation and error handling to manage unexpected data formats.
- Authentication Issues: Securely store and manage API keys and tokens, and implement proper error handling for authentication failures.
- Performance: Use caching, implement pagination for large datasets, and consider using asynchronous requests to improve performance.
11. Popular APIs for Different Purposes
Here are some popular APIs you might consider for various project types:
- Social Media: Twitter API, Facebook Graph API, Instagram API
- Payment Processing: Stripe API, PayPal API
- Maps and Geolocation: Google Maps API, Mapbox API
- Weather: OpenWeatherMap API, Dark Sky API
- E-commerce: Shopify API, WooCommerce API
- Machine Learning: Google Cloud ML API, IBM Watson API
- Data and Analytics: Google Analytics API, Mixpanel API
12. Creating Your Own API
As you become more comfortable with using APIs, you might want to create your own. Here’s a basic example of creating a simple API using Python and Flask:
from flask import Flask, jsonify, request
app = Flask(__name__)
# Sample data
books = [
{"id": 1, "title": "To Kill a Mockingbird", "author": "Harper Lee"},
{"id": 2, "title": "1984", "author": "George Orwell"}
]
@app.route('/api/books', methods=['GET'])
def get_books():
return jsonify({"books": books})
@app.route('/api/books/<int:book_id>', methods=['GET'])
def get_book(book_id):
book = next((book for book in books if book["id"] == book_id), None)
if book:
return jsonify(book)
return jsonify({"error": "Book not found"}), 404
@app.route('/api/books', methods=['POST'])
def add_book():
new_book = request.json
books.append(new_book)
return jsonify(new_book), 201
if __name__ == '__main__':
app.run(debug=True)
This example creates a simple API with endpoints to get all books, get a specific book by ID, and add a new book. Remember, this is a basic implementation and lacks important features like data validation, proper error handling, and authentication, which would be crucial in a production environment.
13. Testing and Debugging API Integrations
Effective testing and debugging are crucial when working with APIs. Here are some strategies:
- Use API Testing Tools: Tools like Postman or Insomnia can help you test API endpoints without writing code.
- Implement Unit Tests: Write unit tests for your API integration code to ensure it behaves correctly under various scenarios.
- Log API Requests and Responses: Implement logging to track API interactions, which can be invaluable for debugging.
- Use Mocking: For testing, consider mocking API responses to simulate various scenarios without making actual API calls.
- Monitor API Usage: Implement monitoring to track API usage, response times, and error rates in your application.
Here’s a simple example of logging API requests and responses:
import requests
import logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
def make_api_request(url, params=None):
logger.info(f"Making API request to {url}")
logger.info(f"Parameters: {params}")
response = requests.get(url, params=params)
logger.info(f"Response status code: {response.status_code}")
logger.info(f"Response content: {response.text[:100]}...") # Log first 100 characters
return response
# Usage
response = make_api_request("https://api.example.com/data", params={"key": "value"})
14. The Future of APIs
As technology continues to evolve, so do APIs. Here are some trends shaping the future of APIs:
- GraphQL: This query language for APIs is gaining popularity due to its flexibility and efficiency in data fetching.
- Serverless APIs: APIs that run on serverless architectures, reducing infrastructure management overhead.
- AI-powered APIs: Increasing integration of AI and machine learning capabilities into APIs.
- IoT APIs: As the Internet of Things grows, APIs specifically designed for IoT devices and applications are becoming more prevalent.
- API-first Design: More companies are adopting an API-first approach to software development, prioritizing API design from the outset.
- Increased Security Measures: With growing concerns about data privacy and security, APIs are implementing more advanced security protocols.
15. Conclusion
Understanding and effectively using APIs is a crucial skill for modern developers. APIs allow you to leverage the power of existing services, extend the functionality of your applications, and participate in the broader ecosystem of interconnected software. By mastering API integration, you open up a world of possibilities for your projects, from accessing vast datasets to incorporating complex functionalities with ease.
Remember, working with APIs is as much about understanding the technical aspects as it is about following best practices, maintaining security, and staying updated with the latest trends. As you continue your journey in software development, make API proficiency a key part of your skill set. Practice by integrating different APIs into your projects, experiment with creating your own APIs, and always strive to write clean, efficient, and secure code when working with APIs.
The world of APIs is vast and constantly evolving. By building a strong foundation in API usage and staying curious about new developments, you’ll be well-equipped to tackle a wide range of programming challenges and create innovative, powerful applications. Happy coding!