Understanding and Implementing Web Scraping Algorithms
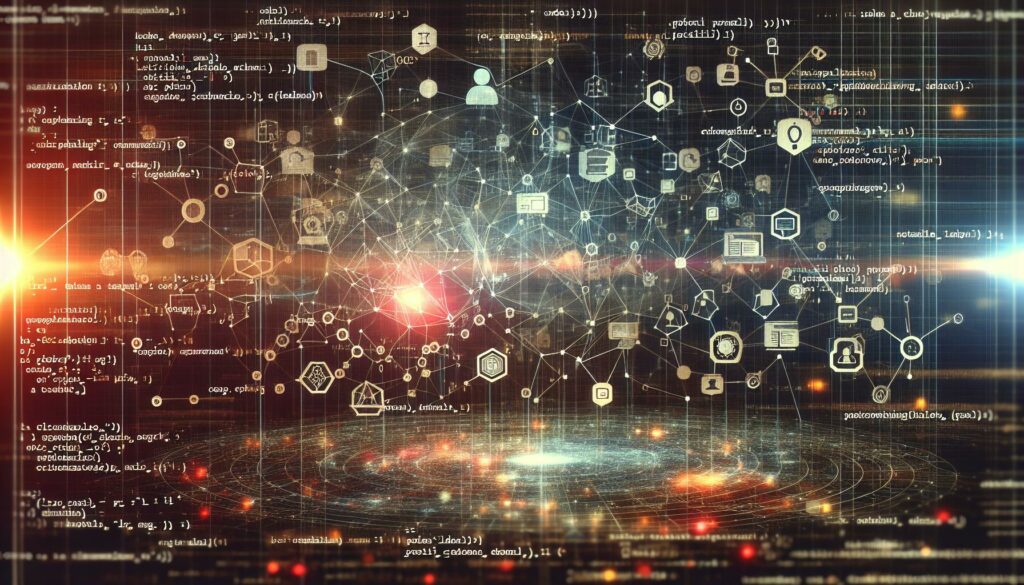
In today’s data-driven world, web scraping has become an essential skill for developers, data scientists, and businesses alike. Web scraping algorithms allow us to extract valuable information from websites automatically, enabling data analysis, market research, and various other applications. This comprehensive guide will delve into the intricacies of web scraping algorithms, their implementation, and best practices to help you master this powerful technique.
What is Web Scraping?
Web scraping, also known as web harvesting or web data extraction, is the process of automatically collecting information from websites. It involves writing programs that send HTTP requests to web servers, download the HTML content of web pages, and then parse that content to extract specific data points.
Web scraping can be used for various purposes, including:
- Price monitoring and comparison
- Lead generation
- Market research and competitor analysis
- News and content aggregation
- Academic research
- Social media sentiment analysis
The Basics of Web Scraping Algorithms
At its core, a web scraping algorithm typically follows these steps:
- Send an HTTP request to the target website
- Download the HTML content of the page
- Parse the HTML to extract desired information
- Store the extracted data in a structured format
- Repeat the process for multiple pages or websites if needed
Let’s explore each of these steps in detail and look at some common algorithms and techniques used in web scraping.
1. Sending HTTP Requests
The first step in web scraping is to send an HTTP request to the target website. This is typically done using libraries like Requests in Python or HttpClient in C#. Here’s a simple example using Python’s Requests library:
import requests
url = "https://example.com"
response = requests.get(url)
if response.status_code == 200:
print("Successfully retrieved the webpage")
html_content = response.text
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
In this example, we send a GET request to the specified URL and check if the response status code is 200 (indicating a successful request). If successful, we store the HTML content of the page in the html_content
variable.
2. Downloading HTML Content
Once we’ve sent the HTTP request and received a response, we need to download the HTML content of the page. This is usually straightforward, as most HTTP libraries automatically handle this process. In the previous example, we’ve already stored the HTML content in the html_content
variable.
3. Parsing HTML
Parsing HTML is a crucial step in web scraping, as it allows us to extract specific information from the webpage. There are several popular libraries and techniques for parsing HTML:
Beautiful Soup
Beautiful Soup is a widely used Python library for parsing HTML and XML documents. It creates a parse tree from the HTML content, which can be navigated easily to find and extract data. Here’s an example of using Beautiful Soup to extract all the links from a webpage:
from bs4 import BeautifulSoup
# Assuming we have the html_content from the previous step
soup = BeautifulSoup(html_content, 'html.parser')
# Find all <a> tags and extract their href attributes
links = [a['href'] for a in soup.find_all('a', href=True)]
print(f"Found {len(links)} links on the page")
for link in links[:5]: # Print the first 5 links
print(link)
XPath
XPath is a query language for selecting nodes from XML documents, which can also be used with HTML. Many web scraping libraries, such as lxml in Python, support XPath queries. Here’s an example of using XPath with lxml to extract all paragraph text from a webpage:
from lxml import html
# Assuming we have the html_content from the previous step
tree = html.fromstring(html_content)
# Use XPath to select all <p> elements
paragraphs = tree.xpath('//p/text()')
print(f"Found {len(paragraphs)} paragraphs on the page")
for paragraph in paragraphs[:3]: # Print the first 3 paragraphs
print(paragraph.strip())
Regular Expressions
While not recommended for parsing HTML in general (due to the complexity and potential inconsistencies of HTML structure), regular expressions can be useful for extracting specific patterns from HTML content. Here’s an example of using regex to extract all email addresses from a webpage:
import re
# Assuming we have the html_content from the previous step
email_pattern = r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b'
emails = re.findall(email_pattern, html_content)
print(f"Found {len(emails)} email addresses on the page")
for email in emails[:5]: # Print the first 5 email addresses
print(email)
4. Storing Extracted Data
After extracting the desired information, it’s important to store it in a structured format for further analysis or processing. Common formats include CSV, JSON, and databases. Here’s an example of storing extracted data in a CSV file using Python’s built-in csv module:
import csv
# Assuming we have extracted some data
data = [
{'name': 'John Doe', 'email': 'john@example.com', 'age': 30},
{'name': 'Jane Smith', 'email': 'jane@example.com', 'age': 28},
{'name': 'Bob Johnson', 'email': 'bob@example.com', 'age': 35}
]
# Write the data to a CSV file
with open('extracted_data.csv', 'w', newline='') as csvfile:
fieldnames = ['name', 'email', 'age']
writer = csv.DictWriter(csvfile, fieldnames=fieldnames)
writer.writeheader()
for row in data:
writer.writerow(row)
print("Data has been written to extracted_data.csv")
5. Handling Multiple Pages
Often, you’ll need to scrape data from multiple pages or navigate through pagination. This can be achieved by implementing a crawling algorithm. Here’s a simple example of scraping multiple pages using Python:
import requests
from bs4 import BeautifulSoup
base_url = "https://example.com/page/"
max_pages = 5
all_data = []
for page_num in range(1, max_pages + 1):
url = f"{base_url}{page_num}"
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
# Extract data from the current page
# This is a placeholder; replace with your actual data extraction logic
page_data = [item.text for item in soup.find_all('div', class_='item')]
all_data.extend(page_data)
print(f"Scraped page {page_num}")
else:
print(f"Failed to retrieve page {page_num}")
print(f"Total items scraped: {len(all_data)}")
Advanced Web Scraping Techniques
As you become more proficient in web scraping, you’ll encounter more complex scenarios that require advanced techniques. Let’s explore some of these techniques and the algorithms behind them.
1. Handling Dynamic Content
Many modern websites use JavaScript to load content dynamically. This can pose a challenge for traditional web scraping techniques that only work with static HTML. To scrape dynamic content, you can use one of the following approaches:
Selenium WebDriver
Selenium WebDriver allows you to automate web browsers, making it possible to interact with dynamic content. Here’s an example of using Selenium with Python to scrape a dynamic website:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# Initialize the WebDriver (make sure you have the appropriate driver installed)
driver = webdriver.Chrome()
url = "https://example.com/dynamic-page"
driver.get(url)
try:
# Wait for a specific element to be present (adjust the timeout as needed)
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, "dynamic-content"))
)
# Extract the text from the dynamic element
dynamic_text = element.text
print(f"Dynamic content: {dynamic_text}")
finally:
driver.quit()
API Requests
Some websites load dynamic content through API calls. By inspecting the network traffic, you can identify these API endpoints and make direct requests to them. Here’s an example using Python’s Requests library:
import requests
api_url = "https://api.example.com/data"
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36"
}
response = requests.get(api_url, headers=headers)
if response.status_code == 200:
data = response.json()
print(f"API response: {data}")
else:
print(f"Failed to retrieve data from API. Status code: {response.status_code}")
2. Handling Authentication
Some websites require authentication to access certain content. To scrape these sites, you’ll need to implement authentication in your scraping algorithm. Here’s an example of handling basic authentication using Python’s Requests library:
import requests
url = "https://api.example.com/protected-data"
username = "your_username"
password = "your_password"
response = requests.get(url, auth=(username, password))
if response.status_code == 200:
data = response.json()
print(f"Protected data: {data}")
else:
print(f"Failed to retrieve protected data. Status code: {response.status_code}")
3. Implementing Rate Limiting
To be a responsible scraper and avoid overloading servers or getting banned, it’s crucial to implement rate limiting in your scraping algorithm. This involves adding delays between requests and respecting the website’s robots.txt file. Here’s an example of implementing rate limiting using Python:
import requests
import time
from urllib.robotparser import RobotFileParser
def scrape_with_rate_limit(url, delay=1):
# Check robots.txt
rp = RobotFileParser()
rp.set_url(f"{url.split('/')[0]}//{url.split('/')[2]}/robots.txt")
rp.read()
if not rp.can_fetch("*", url):
print(f"Scraping not allowed for {url} according to robots.txt")
return None
# Make the request
response = requests.get(url)
# Implement rate limiting
time.sleep(delay)
return response.text if response.status_code == 200 else None
# Example usage
urls = ["https://example.com/page1", "https://example.com/page2", "https://example.com/page3"]
for url in urls:
content = scrape_with_rate_limit(url)
if content:
print(f"Successfully scraped {url}")
# Process the content here
else:
print(f"Failed to scrape {url}")
4. Distributed Scraping
For large-scale scraping projects, you may need to implement distributed scraping to improve efficiency and speed. This involves using multiple machines or processes to scrape different parts of a website concurrently. Here’s a simple example using Python’s multiprocessing module:
import requests
from bs4 import BeautifulSoup
from multiprocessing import Pool
def scrape_page(url):
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
# Extract data from the page
title = soup.title.string if soup.title else "No title"
return f"{url}: {title}"
return f"Failed to scrape {url}"
if __name__ == "__main__":
urls = [f"https://example.com/page/{i}" for i in range(1, 101)]
# Create a pool of worker processes
with Pool(processes=4) as pool:
results = pool.map(scrape_page, urls)
for result in results:
print(result)
Ethical Considerations and Best Practices
While web scraping can be a powerful tool, it’s important to use it responsibly and ethically. Here are some best practices to keep in mind:
- Respect robots.txt: Always check and adhere to the website’s robots.txt file, which specifies which parts of the site can be scraped.
- Implement rate limiting: Don’t overwhelm servers with too many requests in a short time. Use delays between requests and consider using exponential backoff for retries.
- Identify your scraper: Use a custom User-Agent string that identifies your bot and provides contact information.
- Check terms of service: Ensure that scraping is not prohibited by the website’s terms of service.
- Use APIs when available: If a website offers an API, use it instead of scraping, as it’s usually more efficient and less likely to cause issues.
- Be mindful of personal data: If you’re scraping personal information, ensure you comply with relevant data protection regulations (e.g., GDPR).
- Cache data when appropriate: To reduce the load on the target website, consider caching scraped data and updating it periodically rather than scraping on every request.
Conclusion
Web scraping algorithms are powerful tools that enable developers to extract valuable data from the web automatically. By understanding the basics of HTTP requests, HTML parsing, and data extraction techniques, you can build robust scraping solutions for various applications.
As you progress in your web scraping journey, you’ll encounter more complex scenarios that require advanced techniques like handling dynamic content, authentication, and distributed scraping. By implementing these techniques and following best practices, you can create efficient and responsible web scraping algorithms that respect website owners and provide valuable insights from web data.
Remember that web scraping is a constantly evolving field, with websites implementing new technologies and protection measures. Stay updated with the latest tools and techniques, and always approach web scraping with an ethical mindset to ensure your projects are both successful and respectful of the websites you’re interacting with.