Understanding and Implementing Trie Data Structures
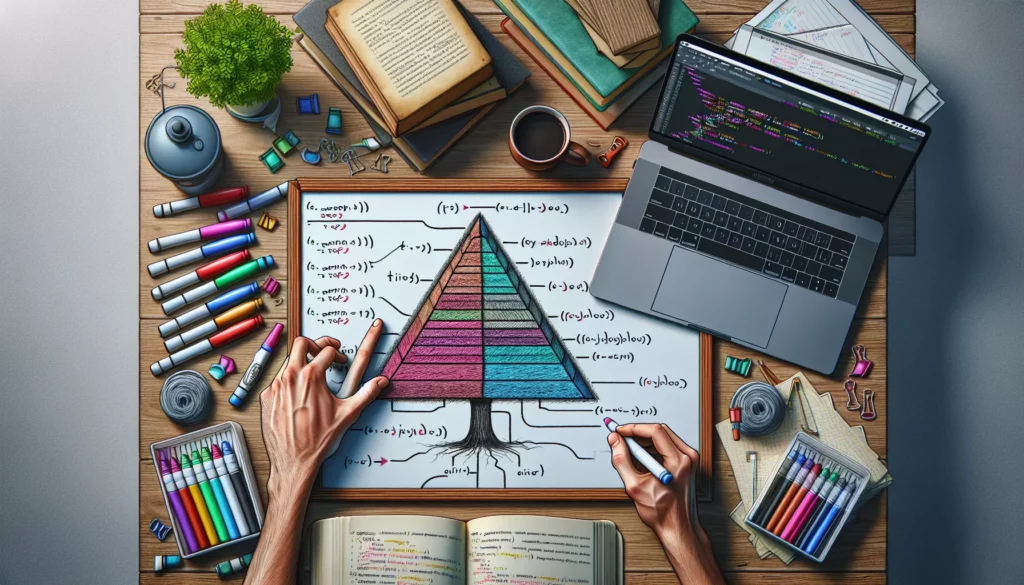
In the world of computer science and algorithmic problem-solving, efficient data structures play a crucial role in optimizing the performance of various applications. One such powerful yet often underutilized data structure is the Trie, also known as a prefix tree. In this comprehensive guide, we’ll dive deep into the concept of Trie data structures, explore their implementation, and discuss their practical applications in solving real-world problems.
What is a Trie?
A Trie, derived from the word “retrieval,” is a tree-like data structure used to store and retrieve strings in a space-efficient manner. It is particularly useful for tasks involving string searches, prefix matching, and autocomplete functionality. The structure of a Trie allows for quick lookups and insertions, making it an excellent choice for applications that require fast string manipulation and searching.
Unlike binary trees or hash tables, Tries store characters of strings in nodes, with each node potentially having multiple children. The root node of a Trie is typically empty, and each path from the root to a node represents a prefix of one or more strings stored in the Trie.
Key Features of Trie Data Structures
- Prefix-based Storage: Tries store strings character by character, with common prefixes shared among multiple strings.
- Efficient Lookup: Searching for a string in a Trie has a time complexity of O(m), where m is the length of the string.
- Space Efficiency: Tries can be more space-efficient than hash tables for certain types of data, especially when storing many strings with common prefixes.
- Prefix Matching: Tries excel at finding all strings with a given prefix, making them ideal for autocomplete and spell-checking applications.
- Ordered Traversal: Tries naturally maintain lexicographic ordering of stored strings.
Basic Structure of a Trie Node
Before we dive into the implementation, let’s understand the basic structure of a Trie node. In its simplest form, a Trie node typically contains:
- An array or map to store child nodes (usually representing the alphabet)
- A boolean flag to indicate if the node represents the end of a word
Here’s a basic representation of a Trie node in Python:
class TrieNode:
def __init__(self):
self.children = {} # A dictionary to store child nodes
self.is_end_of_word = False # Flag to mark the end of a word
Implementing a Trie in Python
Now that we understand the basic structure, let’s implement a Trie class with essential operations like insertion, search, and prefix search.
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end_of_word = True
def search(self, word):
node = self.root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_end_of_word
def starts_with(self, prefix):
node = self.root
for char in prefix:
if char not in node.children:
return False
node = node.children[char]
return True
Let’s break down each method:
1. Insertion (insert method)
The insert method adds a new word to the Trie. It iterates through each character of the word, creating new nodes as necessary. After inserting all characters, it marks the last node as the end of a word.
2. Search (search method)
The search method checks if a given word exists in the Trie. It traverses the Trie following the characters of the word. If at any point a character is not found, it returns False. If all characters are found and the last node is marked as the end of a word, it returns True.
3. Prefix Search (starts_with method)
The starts_with method checks if there are any words in the Trie that start with the given prefix. It’s similar to the search method but doesn’t check the is_end_of_word flag.
Time and Space Complexity Analysis
Understanding the time and space complexity of Trie operations is crucial for evaluating its performance in different scenarios.
Time Complexity:
- Insertion: O(m), where m is the length of the word being inserted.
- Search: O(m), where m is the length of the word being searched.
- Prefix Search: O(p), where p is the length of the prefix.
Space Complexity:
The space complexity of a Trie depends on the number of unique prefixes among all inserted words. In the worst case, where there are no common prefixes, the space complexity can be O(n*m), where n is the number of words and m is the average length of the words.
Practical Applications of Tries
Tries find applications in various domains due to their efficient string manipulation capabilities. Here are some common use cases:
1. Autocomplete and Predictive Text
Tries are excellent for implementing autocomplete features in search engines, text editors, and mobile keyboards. By traversing the Trie based on user input, you can quickly find all words that start with the given prefix.
2. Spell Checkers
Tries can be used to implement efficient spell-checking algorithms. By storing a dictionary of correct words in a Trie, you can quickly verify if a given word exists or find similar words for suggestions.
3. IP Routing Tables
In computer networking, Tries are used to implement IP routing tables. The IP addresses are treated as strings, and the Trie structure allows for efficient longest prefix matching.
4. Dictionary Implementation
Tries can serve as an efficient data structure for implementing dictionaries, especially when dealing with a large number of words with common prefixes.
5. Genome Analysis
In bioinformatics, Tries are used for storing and analyzing DNA sequences, allowing for quick pattern matching and substring searches.
Advanced Trie Concepts
As you become more comfortable with basic Trie operations, you can explore more advanced concepts and optimizations:
1. Compressed Tries (Radix Trees)
Compressed Tries, also known as Radix Trees, optimize space usage by merging nodes with only one child. This can significantly reduce the memory footprint of the Trie.
2. Ternary Search Trees
Ternary Search Trees (TSTs) are a variation of Tries that combine the space efficiency of binary search trees with the speed of Tries. Each node in a TST has three children: left, middle, and right.
3. Suffix Trees
Suffix Trees are a compressed Trie of all suffixes of a given string. They are particularly useful for complex string matching problems and are widely used in bioinformatics.
4. Trie with Wildcard Matching
Implementing wildcard matching in a Trie allows for more flexible string searches, useful in scenarios like file system path matching or regular expression-like functionality.
Implementing an Autocomplete Feature Using Trie
To demonstrate a practical application of Tries, let’s implement a simple autocomplete feature. This feature will suggest words based on a given prefix.
class Trie:
def __init__(self):
self.root = TrieNode()
# ... (previous methods remain the same)
def autocomplete(self, prefix):
node = self.root
for char in prefix:
if char not in node.children:
return []
node = node.children[char]
return self._dfs(node, prefix)
def _dfs(self, node, prefix):
results = []
if node.is_end_of_word:
results.append(prefix)
for char, child_node in node.children.items():
results.extend(self._dfs(child_node, prefix + char))
return results
# Example usage
trie = Trie()
words = ["apple", "app", "application", "append", "banana", "ball", "cat"]
for word in words:
trie.insert(word)
print(trie.autocomplete("app")) # Output: ['app', 'apple', 'append', 'application']
print(trie.autocomplete("ba")) # Output: ['banana', 'ball']
print(trie.autocomplete("c")) # Output: ['cat']
In this implementation, the autocomplete method first navigates to the node representing the end of the given prefix. Then, it performs a depth-first search (DFS) from that node to collect all words that start with the prefix.
Optimizing Trie Performance
While Tries are generally efficient, there are several ways to optimize their performance for specific use cases:
1. Memory Optimization
For large datasets, memory usage can be a concern. Consider using bit vectors or arrays instead of hash maps for child nodes, especially if the alphabet size is known and limited.
2. Lazy Loading
In scenarios where the entire dataset doesn’t need to be loaded upfront, implement lazy loading of Trie nodes. This can significantly reduce initial memory usage and startup time.
3. Caching
For frequently accessed prefixes or words, implement a caching mechanism to store results and avoid repeated Trie traversals.
4. Parallel Processing
For very large datasets or high-throughput requirements, consider implementing parallel processing for Trie operations, especially for bulk insertions or searches.
Comparing Tries with Other Data Structures
To fully appreciate the strengths of Tries, it’s helpful to compare them with other data structures commonly used for string operations:
Tries vs. Hash Tables
- Advantages of Tries:
- More efficient for prefix-based operations
- Can be more space-efficient for strings with common prefixes
- Maintain lexicographic ordering
- Advantages of Hash Tables:
- Generally faster for simple key-value lookups
- More flexible (can store any type of key-value pair)
Tries vs. Binary Search Trees (BSTs)
- Advantages of Tries:
- Faster for string operations (O(m) vs. O(m log n) for BSTs)
- Better for prefix matching and autocomplete
- Advantages of BSTs:
- More memory-efficient for diverse datasets
- Can handle a wider range of data types
Real-world Examples of Trie Usage
To further illustrate the practical importance of Tries, let’s look at some real-world examples where major tech companies leverage this data structure:
1. Google Search Autocomplete
Google’s search engine uses Trie-like structures to provide fast and relevant autocomplete suggestions as users type their queries.
2. Smartphone Keyboards
Mobile keyboard applications, like SwiftKey or Gboard, use Tries to implement predictive text and autocorrect features, improving typing speed and accuracy.
3. Redis
The popular in-memory data structure store Redis uses a variation of Tries called Radix Trees for certain operations, enhancing performance for specific use cases.
4. Elasticsearch
Elasticsearch, a distributed search and analytics engine, uses Tries in its completion suggester feature for implementing autocomplete functionality.
Challenges and Limitations of Tries
While Tries are powerful, they’re not without challenges:
1. Memory Overhead
For small datasets or strings with few common prefixes, Tries can consume more memory compared to other data structures.
2. Complexity in Implementation
Implementing and maintaining a Trie, especially with advanced features, can be more complex than simpler data structures like arrays or hash tables.
3. Limited to String-like Data
Tries are primarily designed for string operations and may not be suitable for other types of data without significant modifications.
Conclusion
Trie data structures offer a powerful and efficient solution for various string-related problems, particularly in areas like autocomplete, spell-checking, and prefix matching. Their unique structure allows for fast lookups and insertions, making them invaluable in many real-world applications.
As you continue your journey in algorithmic problem-solving and software development, keep Tries in your toolkit. They may not be the go-to solution for every problem, but in scenarios involving string manipulation and searching, they can provide significant performance improvements and elegant solutions.
Remember, the key to mastering data structures like Tries is practice. Implement them from scratch, experiment with different variations, and apply them to real-world problems. As you gain experience, you’ll develop an intuition for when and how to use Tries effectively in your projects.
Happy coding, and may your Tries always be efficient and your autocompletes lightning-fast!