Understanding and Applying Greedy Algorithms: A Comprehensive Guide
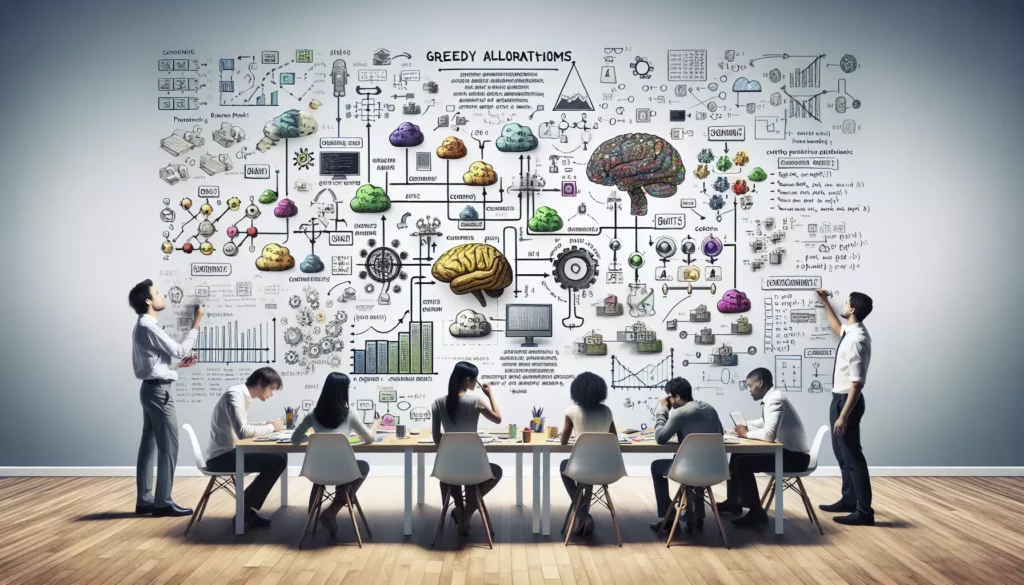
In the world of computer science and programming, efficiency is key. As developers, we’re constantly seeking ways to optimize our code and solve complex problems with the least amount of computational resources. This is where algorithmic thinking comes into play, and one of the most powerful tools in our arsenal is the greedy algorithm. In this comprehensive guide, we’ll dive deep into the concept of greedy algorithms, explore their applications, and learn how to implement them effectively.
What are Greedy Algorithms?
Greedy algorithms are a class of algorithms that follow the problem-solving heuristic of making the locally optimal choice at each step, with the hope of finding a global optimum. In other words, a greedy algorithm always makes the choice that seems best at the moment, without considering the bigger picture or potential future consequences.
The key characteristics of greedy algorithms include:
- Making locally optimal choices at each step
- Never reconsidering previous choices
- Aiming to find an approximate global optimum
- Often providing fast and efficient solutions
While greedy algorithms don’t always yield the best possible solution, they often provide a good approximation and are typically much faster than other approaches, such as dynamic programming or brute force methods.
When to Use Greedy Algorithms
Greedy algorithms are particularly useful in optimization problems where we need to maximize or minimize a certain value. They work well when the problem has the following properties:
- Greedy choice property: A globally optimal solution can be reached by making locally optimal choices.
- Optimal substructure: An optimal solution to the problem contains optimal solutions to subproblems.
Some common scenarios where greedy algorithms are applicable include:
- Huffman coding for data compression
- Dijkstra’s algorithm for finding the shortest path in a graph
- Kruskal’s algorithm for finding the minimum spanning tree
- Activity selection problems
- Fractional knapsack problem
Advantages and Disadvantages of Greedy Algorithms
Like any algorithmic approach, greedy algorithms have their strengths and weaknesses. Let’s explore them:
Advantages:
- Simplicity: Greedy algorithms are often straightforward to understand and implement.
- Efficiency: They typically have lower time complexity compared to other approaches.
- Quick solutions: Greedy algorithms can provide fast approximations for complex problems.
Disadvantages:
- Suboptimal solutions: Greedy algorithms may not always find the globally optimal solution.
- Limited applicability: They work well only for problems with specific properties.
- Lack of foresight: Greedy choices may lead to poor long-term results in some scenarios.
Examples of Greedy Algorithms
Let’s explore some classic examples of greedy algorithms to better understand their application:
1. Coin Change Problem
Problem: Given a set of coin denominations and a target amount, find the minimum number of coins needed to make up that amount.
Greedy Approach: Start with the largest denomination and use as many of those coins as possible, then move to the next largest denomination, and so on.
def coin_change(denominations, target):
denominations.sort(reverse=True)
coins_used = 0
for coin in denominations:
while target >= coin:
target -= coin
coins_used += 1
return coins_used if target == 0 else -1
# Example usage
denominations = [1, 5, 10, 25]
target = 67
result = coin_change(denominations, target)
print(f"Minimum coins needed: {result}")
In this example, the greedy algorithm works well for most common coin systems, but it may not always provide the optimal solution for all possible denomination sets.
2. Activity Selection Problem
Problem: Given a set of activities with start and finish times, select the maximum number of non-overlapping activities.
Greedy Approach: Sort activities by finish time and select activities that don’t overlap with previously selected activities.
def activity_selection(activities):
# Sort activities by finish time
activities.sort(key=lambda x: x[1])
selected = [activities[0]]
last_finish = activities[0][1]
for activity in activities[1:]:
if activity[0] >= last_finish:
selected.append(activity)
last_finish = activity[1]
return selected
# Example usage
activities = [(1, 4), (3, 5), (0, 6), (5, 7), (3, 8), (5, 9), (6, 10), (8, 11), (8, 12), (2, 13), (12, 14)]
result = activity_selection(activities)
print(f"Selected activities: {result}")
This greedy approach guarantees an optimal solution for the activity selection problem.
3. Huffman Coding
Huffman coding is a popular data compression technique that uses a greedy approach to assign variable-length codes to characters based on their frequency of occurrence.
Here’s a simplified implementation of Huffman coding:
import heapq
from collections import defaultdict
class Node:
def __init__(self, char, freq):
self.char = char
self.freq = freq
self.left = None
self.right = None
def __lt__(self, other):
return self.freq < other.freq
def build_huffman_tree(text):
# Count frequency of each character
frequency = defaultdict(int)
for char in text:
frequency[char] += 1
# Create a priority queue of nodes
heap = [Node(char, freq) for char, freq in frequency.items()]
heapq.heapify(heap)
# Build the Huffman tree
while len(heap) > 1:
left = heapq.heappop(heap)
right = heapq.heappop(heap)
merged = Node(None, left.freq + right.freq)
merged.left = left
merged.right = right
heapq.heappush(heap, merged)
return heap[0]
def generate_codes(root, current_code, codes):
if root is None:
return
if root.char:
codes[root.char] = current_code
return
generate_codes(root.left, current_code + "0", codes)
generate_codes(root.right, current_code + "1", codes)
def huffman_encoding(text):
root = build_huffman_tree(text)
codes = {}
generate_codes(root, "", codes)
encoded_text = ""
for char in text:
encoded_text += codes[char]
return encoded_text, codes
# Example usage
text = "this is an example for huffman encoding"
encoded_text, codes = huffman_encoding(text)
print(f"Encoded text: {encoded_text}")
print(f"Huffman codes: {codes}")
Huffman coding is a prime example of how greedy algorithms can be used to solve real-world problems efficiently.
Implementing Greedy Algorithms: Best Practices
When implementing greedy algorithms, consider the following best practices:
- Identify the optimal substructure: Ensure that the problem can be broken down into smaller subproblems that can be solved independently.
- Define the greedy choice: Clearly articulate the locally optimal choice at each step.
- Prove the greedy choice is safe: Verify that making the greedy choice at each step leads to a globally optimal solution.
- Implement efficiently: Use appropriate data structures (e.g., heaps, priority queues) to optimize the algorithm’s performance.
- Test with various inputs: Verify the algorithm’s correctness and efficiency with different input sizes and edge cases.
- Consider the limitations: Be aware of scenarios where the greedy approach may not yield the optimal solution.
Common Pitfalls and How to Avoid Them
When working with greedy algorithms, be mindful of these common pitfalls:
- Assuming greedy always works: Not all problems can be solved optimally with a greedy approach. Always verify that the greedy choice property holds for your problem.
- Overlooking edge cases: Greedy algorithms may fail for certain input configurations. Test your implementation thoroughly with various inputs.
- Ignoring the proof of correctness: While greedy algorithms often seem intuitive, it’s crucial to prove their correctness mathematically.
- Inefficient implementation: Greedy algorithms can become slow if not implemented efficiently. Choose appropriate data structures and optimize your code.
- Neglecting the big picture: Sometimes, a series of locally optimal choices may not lead to a globally optimal solution. Consider the overall problem context.
Greedy Algorithms in Technical Interviews
Greedy algorithms are a popular topic in technical interviews, especially for positions at major tech companies. Here are some tips for tackling greedy algorithm problems in interviews:
- Recognize the pattern: Learn to identify problems that can be solved using a greedy approach.
- Articulate your thought process: Clearly explain why you believe a greedy approach is suitable for the given problem.
- Consider alternatives: Discuss other potential approaches and why greedy might be more efficient.
- Prove correctness: Be prepared to provide a brief proof or explanation of why your greedy algorithm works.
- Analyze time and space complexity: Discuss the efficiency of your solution in terms of big O notation.
- Implement cleanly: Write clear, well-structured code that implements your greedy algorithm.
- Test your solution: Walk through your code with sample inputs and edge cases to demonstrate its correctness.
Advanced Topics in Greedy Algorithms
As you become more comfortable with basic greedy algorithms, consider exploring these advanced topics:
- Greedy algorithms in graph theory: Study advanced applications like Prim’s algorithm, Kruskal’s algorithm, and Dijkstra’s algorithm.
- Approximation algorithms: Learn how greedy approaches can be used to approximate solutions for NP-hard problems.
- Online algorithms: Explore greedy strategies for problems where input is received incrementally.
- Matroids and greedy algorithms: Understand the connection between matroid theory and greedy algorithm optimality.
- Competitive analysis: Study how to analyze the performance of greedy algorithms against optimal solutions.
Conclusion
Greedy algorithms are a powerful tool in a programmer’s toolkit, offering efficient solutions to a wide range of optimization problems. While they may not always provide the globally optimal solution, their simplicity and speed make them an attractive choice for many scenarios.
As you continue your journey in algorithm design and problem-solving, remember that mastering greedy algorithms is just one step towards becoming a well-rounded developer. Practice implementing greedy solutions, analyze their performance, and always be ready to consider alternative approaches when necessary.
By understanding the principles behind greedy algorithms and knowing when to apply them, you’ll be better equipped to tackle complex problems efficiently, whether in your day-to-day coding tasks or during technical interviews at top tech companies.
Keep practicing, stay curious, and never stop learning. Happy coding!