Uber Technical Interview Prep: A Comprehensive Guide
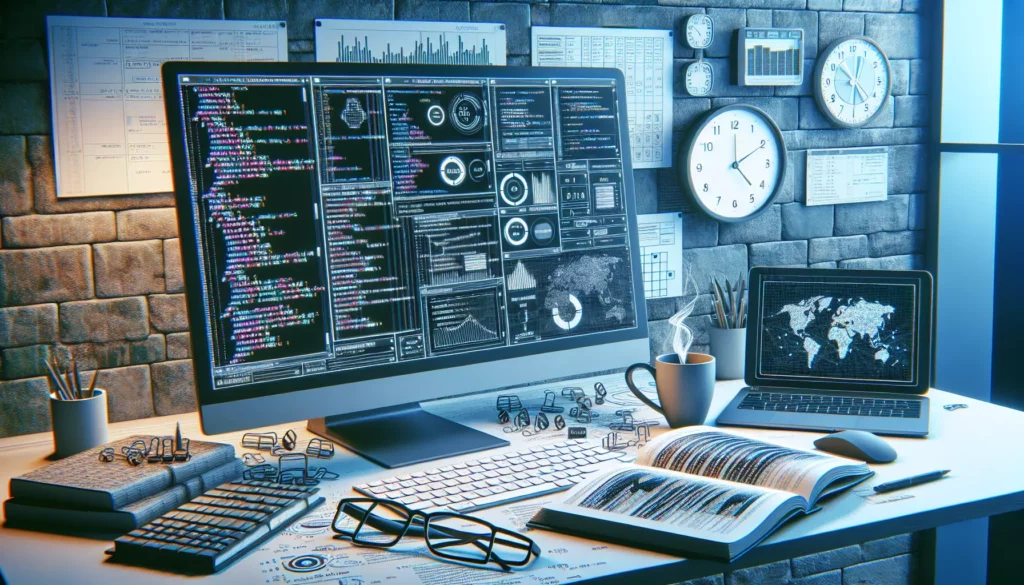
Preparing for a technical interview at Uber can be an exciting yet challenging experience. As one of the leading tech companies in the world, Uber sets high standards for its engineering talent. This comprehensive guide will walk you through the essential steps and key areas to focus on as you prepare for your Uber technical interview.
Table of Contents
- Understanding Uber’s Technical Interview Process
- Core Concepts to Master
- Sharpening Your Coding Skills
- System Design and Architecture
- Behavioral Questions and Soft Skills
- Practice Resources and Mock Interviews
- The Interview Day: What to Expect
- Post-Interview: Follow-up and Next Steps
1. Understanding Uber’s Technical Interview Process
Before diving into the specifics of preparation, it’s crucial to understand the structure of Uber’s technical interview process. Typically, it consists of several stages:
- Initial Phone Screen: A brief conversation with a recruiter to assess your background and interest.
- Technical Phone Interview: A coding interview conducted remotely, usually focusing on algorithmic problems.
- On-site Interviews: A series of in-person (or virtual) interviews covering coding, system design, and behavioral aspects.
- Final Decision: The hiring committee reviews all feedback to make a decision.
Understanding this process helps you tailor your preparation to each stage and know what to expect as you progress.
2. Core Concepts to Master
To excel in Uber’s technical interviews, you need a strong foundation in computer science fundamentals. Focus on mastering these core concepts:
Data Structures
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
Algorithms
- Sorting (Quick Sort, Merge Sort, Heap Sort)
- Searching (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Graph Algorithms (Dijkstra’s, Bellman-Ford)
Time and Space Complexity Analysis
Understanding Big O notation and being able to analyze the efficiency of your solutions is crucial. Practice explaining the time and space complexity of your code.
Example: Implementing a Binary Search Tree
Here’s a basic implementation of a Binary Search Tree in Python to illustrate the importance of understanding data structures:
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = Node(value)
else:
self._insert_recursive(self.root, value)
def _insert_recursive(self, node, value):
if value < node.value:
if node.left is None:
node.left = Node(value)
else:
self._insert_recursive(node.left, value)
else:
if node.right is None:
node.right = Node(value)
else:
self._insert_recursive(node.right, value)
def search(self, value):
return self._search_recursive(self.root, value)
def _search_recursive(self, node, value):
if node is None or node.value == value:
return node
if value < node.value:
return self._search_recursive(node.left, value)
return self._search_recursive(node.right, value)
Understanding how to implement and use data structures like this is crucial for success in technical interviews.
3. Sharpening Your Coding Skills
Uber’s coding interviews are designed to assess your problem-solving skills and coding proficiency. Here are some tips to sharpen your coding skills:
Practice Coding Problems Regularly
Aim to solve at least one coding problem daily. Platforms like LeetCode, HackerRank, and CodeSignal offer a wide range of problems similar to those you might encounter in interviews.
Focus on Problem-Solving Strategies
- Break down complex problems into smaller, manageable parts.
- Start with a brute force solution, then optimize.
- Think aloud and communicate your thought process clearly.
Write Clean and Efficient Code
Practice writing code that is not only correct but also clean, readable, and efficient. Pay attention to naming conventions, code organization, and comments.
Example: Solving the Two Sum Problem
Let’s look at how to approach and solve a common coding interview question, the Two Sum problem:
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
# Test the function
print(two_sum([2, 7, 11, 15], 9)) # Output: [0, 1]
This solution demonstrates efficient use of a hash table to solve the problem in O(n) time complexity.
4. System Design and Architecture
For more senior positions, Uber places significant emphasis on system design questions. These assess your ability to design large-scale distributed systems. Key areas to focus on include:
- Scalability and Performance
- Reliability and Fault Tolerance
- Data Storage and Management
- Microservices Architecture
- Load Balancing and Caching
- API Design
Preparing for System Design Questions
- Study existing large-scale systems and their architectures.
- Practice drawing system diagrams and explaining your design choices.
- Understand trade-offs between different architectural decisions.
- Be familiar with real-world constraints and how to work within them.
Example: Designing a Ride-Sharing System
Here’s a high-level overview of how you might approach designing a ride-sharing system like Uber:
- Requirements Gathering: Identify key features (user registration, ride booking, driver matching, etc.)
- High-Level Design: Sketch out main components (user service, driver service, matching service, etc.)
- Data Model: Design database schema for users, drivers, rides, etc.
- API Design: Define key APIs for user and driver interactions
- Scalability Considerations: Discuss how to handle millions of users and concurrent ride requests
- Real-time Aspects: Address how to handle real-time location updates and matching
Remember to discuss trade-offs and justify your design decisions during the interview.
5. Behavioral Questions and Soft Skills
While technical skills are crucial, Uber also values soft skills and cultural fit. Prepare for behavioral questions that assess your:
- Problem-solving approach
- Teamwork and collaboration skills
- Ability to handle conflicts
- Leadership potential
- Passion for technology and Uber’s mission
Preparing for Behavioral Questions
- Use the STAR method (Situation, Task, Action, Result) to structure your responses.
- Prepare specific examples from your past experiences.
- Practice articulating your thoughts clearly and concisely.
- Research Uber’s culture and values to align your responses.
Example Behavioral Questions
- “Describe a time when you had to work on a challenging project with a tight deadline.”
- “How do you handle disagreements with team members?”
- “Tell me about a time you had to learn a new technology quickly.”
6. Practice Resources and Mock Interviews
To maximize your chances of success, make use of various practice resources:
Online Coding Platforms
- LeetCode
- HackerRank
- CodeSignal
- AlgoExpert
System Design Resources
- “Designing Data-Intensive Applications” by Martin Kleppmann
- System Design Primer (GitHub repository)
- Grokking the System Design Interview (course)
Mock Interviews
Practice with mock interviews to simulate the real experience:
- Pramp: Offers free peer-to-peer mock interviews
- interviewing.io: Provides anonymous practice interviews with engineers from top companies
- Ask friends or colleagues to conduct mock interviews
7. The Interview Day: What to Expect
On the day of your Uber technical interview, keep these tips in mind:
Before the Interview
- Get a good night’s sleep and eat a healthy meal.
- Test your equipment if it’s a virtual interview.
- Have a pen and paper ready for notes.
- Review your prepared examples and key concepts.
During the Interview
- Listen carefully to the questions and ask for clarification if needed.
- Think out loud to show your problem-solving process.
- Stay calm and composed, even if you encounter difficult questions.
- Be honest about what you know and don’t know.
Coding Interview Tips
- Start with a brute force solution, then optimize.
- Discuss time and space complexity of your solutions.
- Test your code with various inputs, including edge cases.
System Design Interview Tips
- Clarify requirements and constraints before diving into the design.
- Start with a high-level design, then drill down into specific components.
- Discuss trade-offs and justify your design decisions.
8. Post-Interview: Follow-up and Next Steps
After your Uber technical interview:
Immediate Actions
- Send a thank-you email to your interviewers or recruiter.
- Reflect on the interview experience and note areas for improvement.
- Follow up with the recruiter if you haven’t heard back within the specified timeframe.
If You Receive an Offer
- Carefully review the offer details.
- Ask questions about team placement, project details, and growth opportunities.
- Negotiate the offer if necessary, considering total compensation and benefits.
If You Don’t Receive an Offer
- Ask for feedback to understand areas for improvement.
- Continue practicing and improving your skills.
- Consider reapplying after gaining more experience (usually after 6-12 months).
Conclusion
Preparing for an Uber technical interview requires dedication, practice, and a well-rounded approach. By focusing on core computer science concepts, sharpening your coding skills, understanding system design principles, and developing your soft skills, you’ll be well-equipped to tackle the challenges of the interview process.
Remember that the interview is not just about showcasing your technical skills, but also about demonstrating your problem-solving approach, communication abilities, and passion for technology. Stay calm, be yourself, and let your skills and enthusiasm shine through.
Good luck with your Uber technical interview preparation! With the right mindset and thorough preparation, you’ll be well on your way to joining one of the most innovative tech companies in the world.