Two Sigma Technical Interview Prep: A Comprehensive Guide
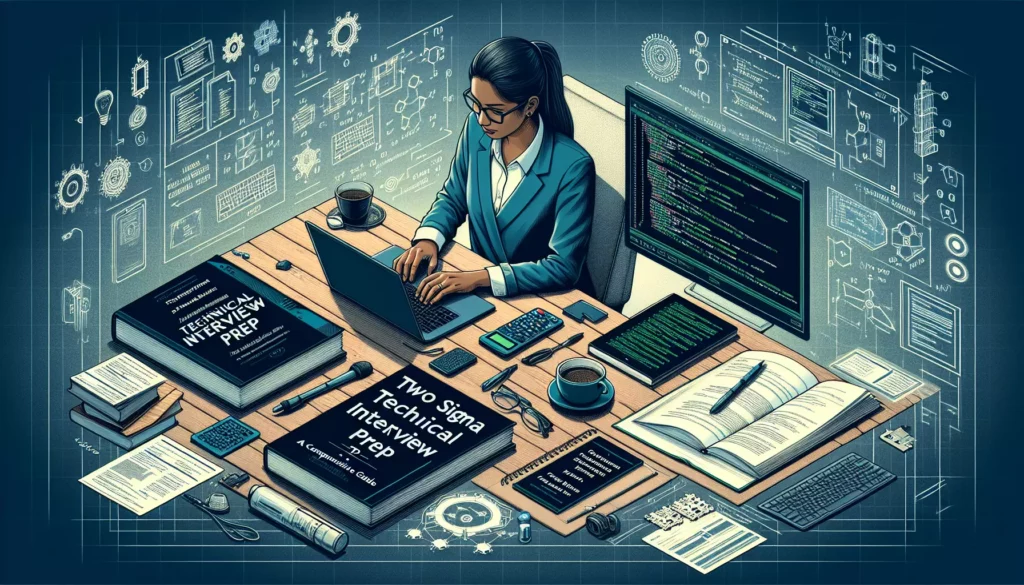
Are you aspiring to land a coveted position at Two Sigma, one of the world’s leading quantitative hedge funds? If so, you’re in the right place. This comprehensive guide will walk you through everything you need to know to ace your Two Sigma technical interview. From understanding the company’s unique culture to mastering the essential skills and topics, we’ve got you covered.
Table of Contents
- Understanding Two Sigma
- The Two Sigma Interview Process
- Essential Technical Skills
- Coding Languages to Focus On
- Data Structures and Algorithms
- System Design and Architecture
- Machine Learning and AI
- Quantitative Finance Concepts
- Behavioral Questions and Soft Skills
- Practice Resources and Mock Interviews
- Tips for Interview Day
- Conclusion
1. Understanding Two Sigma
Before diving into the technical aspects of your interview prep, it’s crucial to understand what makes Two Sigma unique. Founded in 2001, Two Sigma is a systematic investment manager that uses cutting-edge technology and data science to drive its strategies. The company is known for its innovative approach to quantitative trading and its emphasis on technology.
Key points to remember about Two Sigma:
- Focus on data-driven decision making
- Strong emphasis on technology and innovation
- Collaborative and intellectually stimulating work environment
- Commitment to open source and community involvement
Understanding these aspects of Two Sigma’s culture and approach will help you align your responses and demonstrate your fit during the interview process.
2. The Two Sigma Interview Process
The interview process at Two Sigma is known to be rigorous and comprehensive. While it may vary depending on the specific role and level you’re applying for, here’s a general overview of what you can expect:
- Initial Phone Screen: This is usually a brief conversation with a recruiter to assess your background and interest in the role.
- Technical Phone Interview: This involves solving coding problems and discussing your technical background with an engineer.
- Take-Home Assignment: Some positions may require a take-home coding challenge or project.
- On-Site Interviews: This typically consists of multiple rounds, including:
- Coding interviews
- System design discussions
- Algorithm and data structure problems
- Behavioral interviews
- Final Interview: This may involve meeting with senior team members or executives.
Each stage of the process is designed to evaluate different aspects of your skills, knowledge, and cultural fit.
3. Essential Technical Skills
To succeed in a Two Sigma technical interview, you’ll need to demonstrate proficiency in a range of technical skills. Here are some key areas to focus on:
- Strong programming fundamentals
- Proficiency in at least one high-level programming language
- In-depth understanding of data structures and algorithms
- Knowledge of system design and scalability concepts
- Familiarity with machine learning and AI principles
- Understanding of distributed systems
- Experience with big data technologies
- Solid grasp of software engineering best practices
Let’s dive deeper into some of these areas in the following sections.
4. Coding Languages to Focus On
While Two Sigma uses a variety of programming languages, some are more prevalent than others. Here are the languages you should consider focusing on:
- Python: Widely used for data analysis, machine learning, and general-purpose programming.
- Java: Used for building robust, scalable systems.
- C++: Preferred for performance-critical components and low-latency systems.
- R: Commonly used for statistical analysis and data visualization.
- Scala: Used in conjunction with Apache Spark for big data processing.
It’s not necessary to be an expert in all of these languages, but proficiency in at least one or two, along with the ability to pick up new languages quickly, will be beneficial. Here’s a simple example of a function to calculate factorial in Python:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n-1)
# Test the function
print(factorial(5)) # Output: 120
5. Data Structures and Algorithms
A solid understanding of data structures and algorithms is crucial for succeeding in Two Sigma’s technical interviews. You should be comfortable with:
Data Structures:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees (Binary Trees, Binary Search Trees, Balanced Trees)
- Graphs
- Hash Tables
- Heaps
Algorithms:
- Sorting (Quick Sort, Merge Sort, Heap Sort)
- Searching (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Graph Algorithms (Shortest Path, Minimum Spanning Tree)
- Recursion and Backtracking
Here’s an example of implementing a binary search in Python:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Test the function
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
print(binary_search(sorted_array, 7)) # Output: 3
print(binary_search(sorted_array, 6)) # Output: -1
6. System Design and Architecture
Two Sigma places a strong emphasis on building scalable and efficient systems. You should be prepared to discuss system design concepts such as:
- Distributed systems
- Load balancing
- Caching strategies
- Database design and optimization
- Microservices architecture
- Fault tolerance and redundancy
- Data consistency models
- Scalability patterns
During system design interviews, you might be asked to design a high-level architecture for a given problem. For example, you might be asked to design a distributed log aggregation system or a real-time trading platform.
Here’s a simple example of how you might approach designing a basic URL shortener service:
1. Requirements:
- Shorten long URLs
- Redirect users to original URL when accessing shortened URL
- Handle high traffic
2. Components:
- Web server (e.g., Nginx)
- Application server (e.g., Django or Flask)
- Database (e.g., PostgreSQL)
- Cache (e.g., Redis)
3. Data Model:
- Table: shortened_urls
- id: auto-incrementing primary key
- original_url: string
- short_code: string (unique)
- created_at: timestamp
4. Algorithm for generating short codes:
- Use base62 encoding (A-Z, a-z, 0-9) of the auto-incrementing ID
5. API Endpoints:
- POST /shorten
- Input: long URL
- Output: shortened URL
- GET /{short_code}
- Redirect to original URL
6. Scalability Considerations:
- Use a load balancer to distribute traffic
- Implement caching to reduce database load
- Consider using a NoSQL database for better scalability
- Implement rate limiting to prevent abuse
7. Machine Learning and AI
Given Two Sigma’s focus on data-driven decision making, a strong understanding of machine learning and AI concepts is valuable. Key areas to focus on include:
- Supervised and unsupervised learning algorithms
- Feature engineering and selection
- Model evaluation and validation techniques
- Deep learning and neural networks
- Natural Language Processing (NLP)
- Time series analysis and forecasting
- Reinforcement learning
Here’s a simple example of implementing a basic linear regression model using Python’s scikit-learn library:
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import train_test_split
import numpy as np
# Generate sample data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([2, 4, 5, 4, 5])
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train the model
model = LinearRegression()
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Print the model's coefficients and intercept
print(f"Coefficient: {model.coef_[0]}")
print(f"Intercept: {model.intercept_}")
# Evaluate the model
from sklearn.metrics import mean_squared_error, r2_score
print(f"Mean squared error: {mean_squared_error(y_test, y_pred)}")
print(f"R-squared score: {r2_score(y_test, y_pred)}")
8. Quantitative Finance Concepts
While not all roles at Two Sigma require deep knowledge of finance, having a basic understanding of quantitative finance concepts can be beneficial. Some areas to familiarize yourself with include:
- Basic financial instruments (stocks, bonds, derivatives)
- Portfolio theory and risk management
- Time series analysis
- Statistical arbitrage
- Option pricing models (e.g., Black-Scholes)
- Market microstructure
Here’s a simple example of calculating the Sharpe ratio, a common measure of risk-adjusted return in finance:
import numpy as np
def sharpe_ratio(returns, risk_free_rate):
excess_returns = returns - risk_free_rate
return np.mean(excess_returns) / np.std(excess_returns)
# Example usage
portfolio_returns = np.array([0.05, 0.03, 0.08, -0.02, 0.06])
risk_free_rate = 0.02
sharpe = sharpe_ratio(portfolio_returns, risk_free_rate)
print(f"Sharpe Ratio: {sharpe}")
9. Behavioral Questions and Soft Skills
In addition to technical skills, Two Sigma values candidates who can work well in a collaborative environment and align with the company’s culture. Be prepared to answer behavioral questions that assess your:
- Problem-solving approach
- Teamwork and collaboration skills
- Ability to handle ambiguity
- Passion for technology and continuous learning
- Communication skills
- Leadership potential
Some example behavioral questions you might encounter:
- Describe a challenging project you worked on and how you overcame obstacles.
- How do you stay updated with the latest developments in technology?
- Tell me about a time when you had to explain a complex technical concept to a non-technical audience.
- How do you approach learning a new programming language or technology?
When answering these questions, use the STAR method (Situation, Task, Action, Result) to structure your responses and provide concrete examples from your past experiences.
10. Practice Resources and Mock Interviews
To prepare for your Two Sigma technical interview, make use of various practice resources:
- LeetCode: Practice coding problems, especially those tagged as medium to hard difficulty.
- HackerRank: Offers a wide range of coding challenges and competitions.
- System Design Primer: A GitHub repository with comprehensive system design resources.
- Cracking the Coding Interview: A book with a wealth of programming interview questions and strategies.
- Mock Interviews: Practice with peers or use platforms like Pramp or interviewing.io for realistic interview simulations.
Consider creating a study schedule that covers all the key areas we’ve discussed. Here’s an example of how you might structure your preparation:
Week 1-2: Focus on data structures and algorithms
Week 3-4: Deep dive into system design and architecture
Week 5: Machine learning and AI concepts
Week 6: Quantitative finance basics
Week 7-8: Practice coding problems and mock interviews
Throughout: Work on behavioral question responses and soft skills
11. Tips for Interview Day
As your interview day approaches, keep these tips in mind:
- Get a good night’s sleep and eat a healthy meal before the interview.
- Arrive early or ensure your virtual setup is ready well in advance.
- Bring necessary items (ID, notepad, pen) for on-site interviews.
- Listen carefully to the questions and ask for clarification if needed.
- Think out loud during problem-solving to show your thought process.
- Stay calm and composed, even if you encounter difficult questions.
- Be prepared to discuss your resume in detail.
- Have thoughtful questions ready to ask your interviewers about Two Sigma and the role.
12. Conclusion
Preparing for a technical interview at Two Sigma requires dedication, thorough knowledge of computer science fundamentals, and the ability to apply this knowledge to complex problems. By focusing on the areas we’ve covered in this guide – from data structures and algorithms to system design and machine learning – you’ll be well-equipped to tackle the challenges of the interview process.
Remember that Two Sigma values not just technical skills, but also creativity, collaboration, and a passion for solving complex problems. Showcase these qualities throughout your interview process, and you’ll be well on your way to landing that dream job at Two Sigma.
Good luck with your preparation, and may your journey to Two Sigma be a successful one!