Two Sigma Interview Questions: Mastering the Technical Challenge
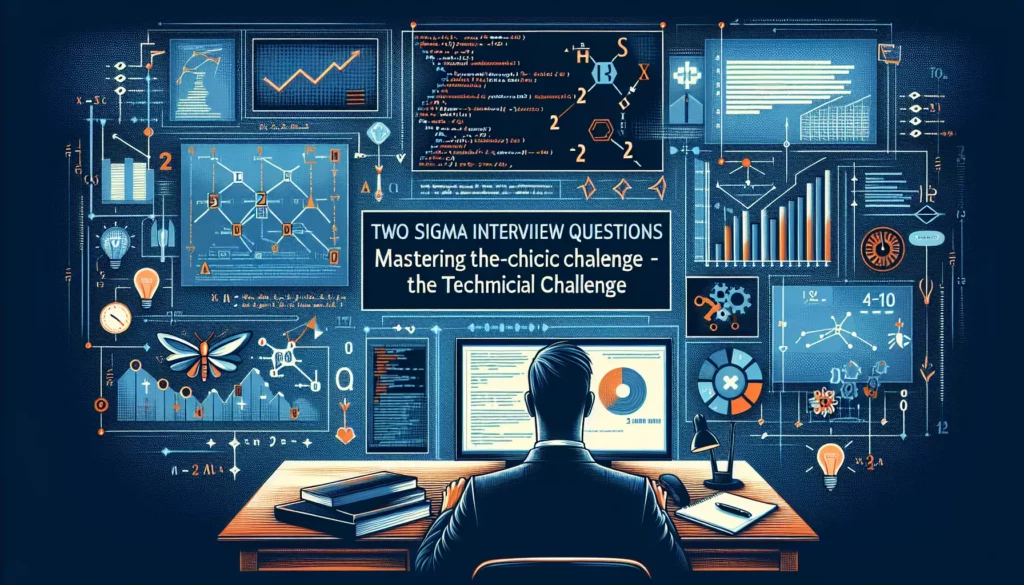
Are you gearing up for a technical interview at Two Sigma? This prestigious quantitative hedge fund is known for its rigorous hiring process, especially when it comes to evaluating candidates’ coding and problem-solving skills. In this comprehensive guide, we’ll dive deep into the types of questions you might encounter, strategies to tackle them, and how to best prepare for your Two Sigma interview.
Understanding Two Sigma’s Interview Process
Before we delve into specific questions, it’s crucial to understand the overall structure of Two Sigma’s interview process. Typically, it consists of several stages:
- Initial phone screen or online assessment
- Technical phone interview
- On-site interviews (multiple rounds)
The technical interviews, both phone and on-site, are where you’ll face the bulk of the challenging coding and problem-solving questions. Two Sigma is known for its focus on algorithmic thinking, data structures, and quantitative problem-solving skills.
Common Types of Two Sigma Interview Questions
Two Sigma’s interview questions often fall into the following categories:
1. Algorithm and Data Structure Questions
These questions test your ability to design efficient algorithms and use appropriate data structures. Examples include:
- Implement a hash table from scratch
- Design an algorithm to find the kth largest element in an unsorted array
- Implement a trie (prefix tree) and its basic operations
2. Mathematical and Probability Problems
Given Two Sigma’s focus on quantitative trading, you might encounter questions that test your mathematical and probabilistic reasoning:
- Calculate the probability of getting a full house in poker
- Solve a complex probability puzzle involving coin flips or dice rolls
- Implement a function to generate random numbers with a specific distribution
3. System Design Questions
For more senior positions, you might be asked to design large-scale systems:
- Design a distributed cache system
- Create a high-frequency trading system architecture
- Design a real-time analytics dashboard for financial data
4. Coding Implementation Questions
These questions test your ability to translate algorithms into working code:
- Implement a function to perform matrix multiplication
- Write a program to find all prime numbers up to a given limit
- Implement a basic machine learning algorithm like linear regression
Sample Two Sigma Interview Questions and Solutions
Let’s look at a few sample questions you might encounter in a Two Sigma interview, along with approaches to solve them:
Question 1: Implement a LRU (Least Recently Used) Cache
This is a classic question that tests your understanding of data structures and algorithm design.
Problem: Implement a data structure for a Least Recently Used (LRU) cache. It should support the following operations: get and put.
- get(key) – Get the value (will always be positive) of the key if the key exists in the cache, otherwise return -1.
- put(key, value) – Set or insert the value if the key is not already present. When the cache reached its capacity, it should invalidate the least recently used item before inserting a new item.
The cache is initialized with a positive capacity.
Solution:
class LRUCache {
private:
int capacity;
list<pair<int, int>> cache;
unordered_map<int, list<pair<int, int>>::iterator> map;
public:
LRUCache(int capacity) : capacity(capacity) {}
int get(int key) {
if (map.find(key) == map.end()) {
return -1;
}
cache.splice(cache.begin(), cache, map[key]);
return map[key]->second;
}
void put(int key, int value) {
if (get(key) != -1) {
map[key]->second = value;
return;
}
if (cache.size() == capacity) {
int lru_key = cache.back().first;
cache.pop_back();
map.erase(lru_key);
}
cache.push_front({key, value});
map[key] = cache.begin();
}
};
This implementation uses a combination of a doubly-linked list and a hash map to achieve O(1) time complexity for both get and put operations.
Question 2: Find the Median of Two Sorted Arrays
This question tests your ability to work with sorted arrays and implement an efficient algorithm.
Problem: Given two sorted arrays nums1 and nums2 of size m and n respectively, return the median of the two sorted arrays.
Solution:
class Solution {
public:
double findMedianSortedArrays(vector<int>& nums1, vector<int>& nums2) {
if (nums1.size() > nums2.size()) {
return findMedianSortedArrays(nums2, nums1);
}
int x = nums1.size();
int y = nums2.size();
int low = 0;
int high = x;
while (low <= high) {
int partitionX = (low + high) / 2;
int partitionY = (x + y + 1) / 2 - partitionX;
int maxLeftX = (partitionX == 0) ? INT_MIN : nums1[partitionX - 1];
int minRightX = (partitionX == x) ? INT_MAX : nums1[partitionX];
int maxLeftY = (partitionY == 0) ? INT_MIN : nums2[partitionY - 1];
int minRightY = (partitionY == y) ? INT_MAX : nums2[partitionY];
if (maxLeftX <= minRightY && maxLeftY <= minRightX) {
if ((x + y) % 2 == 0) {
return (max(maxLeftX, maxLeftY) + min(minRightX, minRightY)) / 2.0;
} else {
return max(maxLeftX, maxLeftY);
}
} else if (maxLeftX > minRightY) {
high = partitionX - 1;
} else {
low = partitionX + 1;
}
}
throw invalid_argument("Input arrays are not sorted.");
}
};
This solution uses a binary search approach to find the partition point in the smaller array, which indirectly determines the partition in the larger array. The time complexity is O(log(min(m,n))), where m and n are the lengths of the input arrays.
Question 3: Design a Time-Based Key-Value Store
This question tests your ability to design a more complex data structure with specific time-based requirements.
Problem: Design a time-based key-value data structure that can store multiple values for the same key at different time stamps and retrieve the key’s value at a certain timestamp.
Solution:
class TimeMap {
private:
unordered_map<string, vector<pair<int, string>>> m;
public:
TimeMap() {}
void set(string key, string value, int timestamp) {
m[key].push_back({timestamp, value});
}
string get(string key, int timestamp) {
if (m.find(key) == m.end()) {
return "";
}
int left = 0, right = m[key].size() - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (m[key][mid].first <= timestamp) {
if (mid == m[key].size() - 1 || m[key][mid + 1].first > timestamp) {
return m[key][mid].second;
}
left = mid + 1;
} else {
right = mid - 1;
}
}
return "";
}
};
This implementation uses a hash map where each key maps to a vector of timestamp-value pairs. The get operation uses binary search to find the most recent value before or at the given timestamp.
Strategies for Tackling Two Sigma Interview Questions
When approaching Two Sigma interview questions, keep these strategies in mind:
1. Clarify the Problem
Before diving into a solution, make sure you fully understand the problem. Ask clarifying questions about input formats, edge cases, and expected outputs.
2. Think Aloud
Verbalize your thought process as you work through the problem. This gives the interviewer insight into your problem-solving approach and allows them to provide hints if needed.
3. Consider Multiple Approaches
Don’t jump to the first solution that comes to mind. Consider multiple approaches and discuss their trade-offs in terms of time and space complexity.
4. Optimize Your Solution
After coming up with an initial solution, think about ways to optimize it. Can you reduce the time or space complexity? Are there any clever tricks or data structures you can use?
5. Test Your Code
Before declaring your solution complete, walk through it with a few test cases, including edge cases. This demonstrates attention to detail and thoroughness.
Preparing for Your Two Sigma Interview
To maximize your chances of success in a Two Sigma interview, consider the following preparation strategies:
1. Master the Fundamentals
Ensure you have a solid grasp of fundamental data structures (arrays, linked lists, trees, graphs, hash tables) and algorithms (sorting, searching, dynamic programming, graph algorithms).
2. Practice Coding Problems
Regularly solve coding problems on platforms like LeetCode, HackerRank, or CodeSignal. Focus on medium to hard difficulty problems, as Two Sigma interviews tend to be challenging.
3. Study Quantitative Topics
Brush up on probability, statistics, and mathematical problem-solving. Two Sigma may ask questions that require quantitative reasoning.
4. Learn About Financial Markets
While not strictly necessary for the coding interview, having some knowledge about financial markets and quantitative trading can be beneficial, especially for behavioral questions.
5. Mock Interviews
Practice with mock interviews, either with friends or through platforms that offer this service. This helps you get comfortable with the interview format and improves your ability to communicate your thoughts clearly.
6. Review System Design
For more senior positions, be prepared to discuss large-scale system design. Study topics like distributed systems, caching strategies, and database design.
Common Mistakes to Avoid in Two Sigma Interviews
Be aware of these common pitfalls during your Two Sigma interview:
1. Rushing to Code
Don’t start coding immediately. Take time to think through the problem and discuss your approach with the interviewer.
2. Ignoring Time and Space Complexity
Always consider and discuss the time and space complexity of your solutions. Two Sigma places a high value on efficient algorithms.
3. Neglecting Edge Cases
Make sure to consider and handle edge cases in your solutions. This demonstrates thoroughness and attention to detail.
4. Poor Communication
Clear communication is crucial. Explain your thought process clearly and ask for clarification when needed.
5. Not Testing Your Code
Always test your code with a few examples, including edge cases, before declaring it complete.
Conclusion
Preparing for a Two Sigma interview can be challenging, but with the right approach and thorough preparation, you can significantly increase your chances of success. Focus on strengthening your algorithmic thinking, problem-solving skills, and coding abilities. Remember that the interview is not just about finding the correct solution, but also about demonstrating your thought process, communication skills, and ability to work through complex problems.
Two Sigma values candidates who can think critically, optimize solutions, and communicate effectively. By mastering the types of questions discussed in this guide and following the preparation strategies outlined, you’ll be well-equipped to tackle the Two Sigma interview process.
Remember, each interview experience is unique, and the specific questions you encounter may vary. However, the core skills and problem-solving approaches discussed here will serve you well regardless of the exact questions asked. Good luck with your Two Sigma interview!