Twilio Technical Interview Prep: A Comprehensive Guide
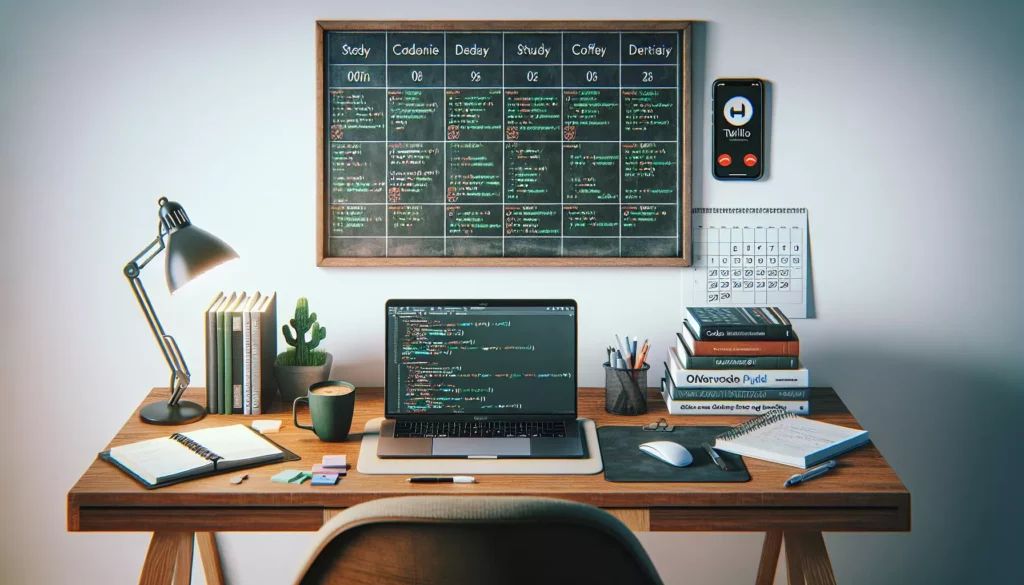
If you’re gearing up for a technical interview at Twilio, you’re in the right place. This comprehensive guide will walk you through everything you need to know to ace your Twilio technical interview. From understanding the company’s culture to mastering the specific technical skills they’re looking for, we’ve got you covered.
Table of Contents
- Understanding Twilio
- The Twilio Interview Process
- Technical Skills to Master
- Common Coding Challenges
- System Design Questions
- Behavioral Questions
- Preparation Tips
- Additional Resources
Understanding Twilio
Before diving into the technical aspects, it’s crucial to understand what Twilio does and why it’s a unique player in the tech industry. Twilio is a cloud communications platform that allows software developers to programmatically make and receive phone calls, send and receive text messages, and perform other communication functions using its web service APIs.
Key points about Twilio:
- Founded in 2008 by Jeff Lawson, Evan Cooke, and John Wolthuis
- Provides a cloud-based platform for building SMS, voice, and messaging applications
- Offers APIs for various communication channels including SMS, voice, video, and email
- Known for its developer-friendly approach and extensive documentation
Understanding Twilio’s mission and products will not only help you during the interview process but also demonstrate your genuine interest in the company.
The Twilio Interview Process
Twilio’s interview process typically consists of several stages:
- Initial Phone Screen: This is usually with a recruiter to discuss your background and interest in Twilio.
- Technical Phone Interview: A 45-60 minute call with an engineer, involving coding questions and technical discussions.
- Take-Home Coding Assignment: Some positions may require a take-home project to showcase your coding skills.
- On-Site Interviews: A series of interviews (now often conducted virtually) including:
- Coding interviews
- System design discussion
- Behavioral interviews
The entire process can take anywhere from 2-4 weeks, depending on the position and your availability.
Technical Skills to Master
Twilio values strong fundamental programming skills. Here are the key areas to focus on:
1. Programming Languages
While Twilio uses various languages, proficiency in at least one of the following is crucial:
- Python
- Java
- JavaScript/Node.js
- Ruby
- C#
2. Data Structures and Algorithms
Strong knowledge of fundamental data structures and algorithms is essential. Focus on:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Sorting and Searching algorithms
- Dynamic Programming
- Big O notation and time/space complexity analysis
3. Web Technologies
Given Twilio’s focus on web-based APIs, understanding these technologies is important:
- RESTful API design principles
- HTTP/HTTPS protocols
- JSON and XML
- WebSockets
4. Database Knowledge
Familiarity with both SQL and NoSQL databases:
- MySQL or PostgreSQL
- MongoDB
- Redis
5. Cloud and DevOps
Understanding of cloud platforms and DevOps practices:
- AWS, Google Cloud, or Azure
- Docker and Kubernetes
- CI/CD pipelines
Common Coding Challenges
During the technical interviews, you may encounter coding challenges similar to these:
1. String Manipulation
Example: Implement a function to determine if a string has all unique characters.
def has_unique_chars(s):
char_set = set()
for char in s:
if char in char_set:
return False
char_set.add(char)
return True
# Test the function
print(has_unique_chars("abcdefg")) # True
print(has_unique_chars("abcdefga")) # False
2. Array Processing
Example: Find the maximum subarray sum in an array of integers.
def max_subarray_sum(arr):
max_sum = current_sum = arr[0]
for num in arr[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
# Test the function
print(max_subarray_sum([-2, 1, -3, 4, -1, 2, 1, -5, 4])) # 6
3. Tree Traversal
Example: Implement an in-order traversal of a binary tree.
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
result = []
def inorder(node):
if node:
inorder(node.left)
result.append(node.val)
inorder(node.right)
inorder(root)
return result
# Test the function
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
print(inorder_traversal(root)) # [4, 2, 5, 1, 3]
4. Graph Algorithms
Example: Implement Breadth-First Search (BFS) on a graph.
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(vertex, end=' ')
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
# Test the function
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
bfs(graph, 'A') # Output: A B C D E F
System Design Questions
For more senior positions, you may be asked system design questions. Here are some topics to prepare for:
1. Designing a Chat Application
This is particularly relevant to Twilio’s services. Consider:
- Real-time message delivery
- Scaling to millions of users
- Handling offline users
- Message persistence
2. Designing a URL Shortener
This tests your ability to design a distributed system. Think about:
- Generating unique short URLs
- Redirecting to original URLs
- Analytics and tracking
- Scalability and load balancing
3. Designing a Notification System
Relevant to Twilio’s core business. Consider:
- Supporting multiple channels (SMS, email, push notifications)
- Handling high throughput
- Ensuring delivery and retry mechanisms
- User preferences and opt-outs
When tackling system design questions, remember to:
- Clarify requirements and constraints
- Start with a high-level design
- Dive into specific components
- Discuss trade-offs and potential improvements
Behavioral Questions
Twilio places a strong emphasis on culture fit. Be prepared to answer questions about your experiences and how you handle various situations. Some common themes include:
- Describing a challenging project you’ve worked on
- How you handle disagreements with team members
- Your approach to learning new technologies
- How you’ve dealt with failure or setbacks
- Your experience with Agile methodologies
Remember to use the STAR method (Situation, Task, Action, Result) when answering behavioral questions. This helps structure your responses and provides concrete examples of your skills and experiences.
Preparation Tips
To maximize your chances of success in the Twilio technical interview, consider these tips:
- Practice coding regularly: Use platforms like LeetCode, HackerRank, or AlgoCademy to sharpen your coding skills.
- Review Twilio’s documentation: Familiarize yourself with Twilio’s products and APIs. This shows genuine interest and initiative.
- Mock interviews: Practice with a friend or use online mock interview services to get comfortable with the interview format.
- Understand Twilio’s values: Twilio has a strong company culture. Research their values and think about how you align with them.
- Prepare questions: Have thoughtful questions ready for your interviewers about the role, team, and company.
- Stay updated: Keep up with the latest trends in cloud communications and API development.
Additional Resources
To further prepare for your Twilio technical interview, consider these resources:
- Twilio Documentation: Familiarize yourself with Twilio’s products and APIs.
- Cracking the Coding Interview by Gayle Laakmann McDowell: A comprehensive guide to technical interviews.
- Grokking the System Design Interview: An excellent resource for system design preparation.
- Twilio Blog: Stay updated with Twilio’s latest developments and technical insights.
- Glassdoor Twilio Interview Questions: Read about others’ interview experiences at Twilio.
Conclusion
Preparing for a Twilio technical interview requires a combination of strong coding skills, system design knowledge, and cultural fit. By focusing on the areas outlined in this guide and consistently practicing, you’ll be well-equipped to showcase your abilities and land that dream job at Twilio.
Remember, the key to success is not just about having the right answers, but also demonstrating your problem-solving process, communication skills, and passion for technology. Good luck with your Twilio interview!