Turning Tutorials Into Personal Projects: How to Make Them Yours
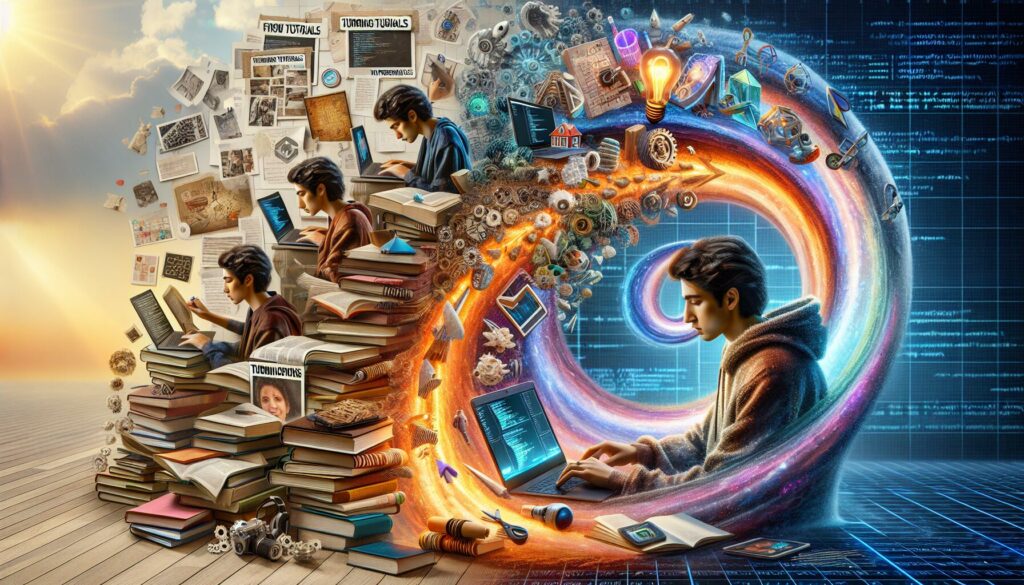
In the ever-evolving world of coding and software development, tutorials have become an invaluable resource for learners at all levels. Platforms like AlgoCademy offer a wealth of interactive coding tutorials designed to help you progress from beginner-level coding to mastering complex algorithms. However, the true power of learning comes not just from following tutorials but from transforming that knowledge into personal projects. This article will guide you through the process of turning tutorials into unique, portfolio-worthy projects that showcase your skills and creativity.
The Importance of Personal Projects
Before we dive into the how-to, let’s understand why personal projects are crucial:
- Deeper Understanding: When you apply tutorial concepts to your own projects, you gain a more profound understanding of the material.
- Portfolio Building: Personal projects are excellent additions to your coding portfolio, demonstrating your abilities to potential employers.
- Creativity and Problem-Solving: Creating your own projects encourages creative thinking and improves your problem-solving skills.
- Motivation and Engagement: Working on personal projects can be more engaging and motivating than simply following tutorials.
Step 1: Choose the Right Tutorial
The first step in turning a tutorial into a personal project is selecting the right tutorial. Here are some tips:
- Align with Your Interests: Choose tutorials that cover topics or technologies you’re genuinely interested in.
- Consider Your Skill Level: Select tutorials that match or slightly exceed your current skill level to ensure a good challenge without overwhelming yourself.
- Look for Extensibility: Opt for tutorials that teach concepts or build projects that can be easily expanded or modified.
For instance, if you’re using AlgoCademy and are interested in data structures, you might choose a tutorial on implementing a basic binary search tree. This could be a great starting point for a more complex project.
Step 2: Complete the Tutorial
Before you can transform a tutorial into a personal project, you need to understand it thoroughly. Here’s how to make the most of the tutorial:
- Follow Along Actively: Don’t just copy and paste code. Type it out yourself and try to understand each line.
- Take Notes: Jot down key concepts, functions, or methods that you find interesting or challenging.
- Experiment: As you go through the tutorial, try tweaking the code to see how it affects the outcome.
- Use Resources: If you’re using a platform like AlgoCademy, take advantage of their AI-powered assistance to clarify any doubts.
Step 3: Brainstorm Extensions and Modifications
Once you’ve completed the tutorial, it’s time to think about how you can extend or modify it to create your personal project. Here are some strategies:
- Add Features: Think about what additional functionality would make the project more useful or interesting.
- Change the Context: Apply the core concept to a different problem or domain.
- Combine Multiple Tutorials: Merge concepts from different tutorials to create a more complex project.
- Optimize Performance: Look for ways to make the code more efficient or scalable.
- Improve User Experience: If the tutorial created a basic interface, consider enhancing it for better usability.
For example, if you followed a tutorial on creating a basic to-do list app, you could extend it by adding features like due dates, priority levels, or even integrating it with a calendar API.
Step 4: Plan Your Personal Project
Before diving into coding, take some time to plan your project:
- Define Clear Goals: What do you want your project to achieve? What skills do you want to demonstrate?
- Break It Down: Divide your project into smaller, manageable tasks.
- Create a Timeline: Set realistic deadlines for each task to keep yourself accountable.
- Choose Your Tools: Decide what additional libraries, frameworks, or APIs you’ll need.
Let’s say you’re extending the binary search tree tutorial from AlgoCademy. Your plan might include tasks like implementing additional tree operations, creating a visualization of the tree structure, or applying the tree to solve a specific problem like organizing a file system.
Step 5: Start Coding
Now comes the exciting part – bringing your personal project to life! Here are some tips for the coding phase:
- Start with the Core Functionality: Begin by implementing the main features of your project.
- Reuse and Adapt: Don’t hesitate to reuse code from the tutorial, but make sure you understand and adapt it to your needs.
- Comment Your Code: Write clear comments explaining your logic, especially when you’re deviating from the tutorial.
- Commit Often: If you’re using version control (which you should), make frequent, small commits to track your progress.
- Test as You Go: Regularly test your code to catch and fix bugs early.
Here’s an example of how you might start extending the binary search tree tutorial:
class BinarySearchTree:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def insert(self, value):
if value < self.value:
if self.left is None:
self.left = BinarySearchTree(value)
else:
self.left.insert(value)
else:
if self.right is None:
self.right = BinarySearchTree(value)
else:
self.right.insert(value)
# New method: Find the minimum value in the tree
def find_min(self):
current = self
while current.left is not None:
current = current.left
return current.value
# New method: Find the maximum value in the tree
def find_max(self):
current = self
while current.right is not None:
current = current.right
return current.value
# Example usage
bst = BinarySearchTree(10)
bst.insert(5)
bst.insert(15)
bst.insert(2)
bst.insert(7)
print(f"Minimum value: {bst.find_min()}")
print(f"Maximum value: {bst.find_max()}")
In this example, we’ve extended the basic binary search tree implementation with methods to find the minimum and maximum values in the tree.
Step 6: Debug and Refine
As you work on your project, you’ll inevitably encounter bugs and areas for improvement. Here’s how to handle this phase:
- Use Debugging Tools: Familiarize yourself with debugging tools in your IDE or use print statements strategically.
- Rubber Duck Debugging: Sometimes, explaining your code out loud (even to an inanimate object) can help you spot issues.
- Seek Feedback: Share your code with peers or mentors for review and suggestions.
- Refactor: Look for opportunities to make your code more efficient or readable.
- Optimize: If you’re working on algorithmic problems, consider the time and space complexity of your solutions.
Step 7: Document Your Project
Documentation is crucial, especially if you plan to showcase your project in your portfolio. Here’s what to include:
- README File: Create a comprehensive README that explains what your project does, how to set it up, and how to use it.
- Code Comments: Ensure your code is well-commented, explaining complex logic or algorithms.
- Usage Examples: Provide clear examples of how to use your project or its main functions.
- Challenges and Solutions: Discuss any significant challenges you faced and how you overcame them.
- Future Improvements: Mention any ideas for future enhancements or features.
Here’s an example of what a section of your README might look like for the extended binary search tree project:
# Extended Binary Search Tree
This project extends the basic Binary Search Tree implementation with additional functionalities.
## Features
- Basic BST operations: insert, search
- Find minimum value in the tree
- Find maximum value in the tree
- Visualize the tree structure (ASCII art)
## Usage
```python
bst = BinarySearchTree(10)
bst.insert(5)
bst.insert(15)
bst.insert(2)
bst.insert(7)
print(f"Minimum value: {bst.find_min()}")
print(f"Maximum value: {bst.find_max()}")
bst.visualize()
```
## Challenges Overcome
One of the main challenges was implementing the tree visualization. I solved this by using a recursive approach to build a 2D array representing the tree structure, then converting it to ASCII art.
## Future Improvements
- Implement balancing algorithms (AVL or Red-Black)
- Add deletion operation
- Create a graphical user interface for tree manipulation
Step 8: Share and Showcase Your Project
Once your project is complete, it’s time to share it with the world:
- GitHub: Host your project on GitHub to make it easily accessible to potential employers and collaborators.
- Portfolio Website: Feature your project on your personal portfolio website with screenshots and descriptions.
- Blog Post: Write a blog post about your project, discussing your process and what you learned.
- Social Media: Share your project on platforms like LinkedIn or Twitter to get feedback and recognition.
- Coding Communities: Present your project in coding forums or communities to get feedback from fellow developers.
Real-World Example: From Tutorial to Job-Landing Project
Let’s look at a real-world example of how turning a tutorial into a personal project can lead to tangible career benefits.
Sarah, a self-taught programmer, followed an AlgoCademy tutorial on building a basic recommendation system using collaborative filtering. Intrigued by the concept, she decided to extend this into a personal project. Here’s how she did it:
- Extension Idea: Sarah decided to create a book recommendation system that not only used collaborative filtering but also incorporated natural language processing to analyze book descriptions.
- Data Collection: She scraped data from a popular book review website, collecting information on books, user ratings, and book descriptions.
- Algorithm Implementation: Sarah implemented the collaborative filtering algorithm from the tutorial but added a content-based filtering component using TF-IDF and cosine similarity on book descriptions.
- User Interface: She created a simple web interface using Flask where users could rate books and receive personalized recommendations.
- Testing and Refinement: Sarah tested her system with friends and fellow book lovers, refining the algorithm based on their feedback.
- Documentation: She wrote a detailed README and created a blog post explaining her process, challenges, and learnings.
- Sharing: Sarah shared her project on GitHub and posted about it on LinkedIn.
The result? A startup working on a personalized education platform saw Sarah’s project and was impressed by her initiative and the skills she demonstrated. They invited her for an interview and ultimately offered her a junior data scientist position.
This example illustrates how taking a tutorial and transforming it into a unique, well-executed personal project can showcase your skills, creativity, and problem-solving abilities to potential employers.
Conclusion: The Power of Personal Projects
Turning tutorials into personal projects is a powerful way to deepen your understanding of programming concepts, showcase your skills, and stand out in the competitive tech industry. By following the steps outlined in this article, you can transform the knowledge gained from platforms like AlgoCademy into tangible, impressive projects that demonstrate your ability to apply coding concepts to real-world problems.
Remember, the key is to not just replicate tutorials, but to use them as springboards for your own ideas and innovations. Each personal project you create is an opportunity to learn, grow, and demonstrate your unique capabilities as a developer.
So the next time you complete a coding tutorial, don’t stop there. Ask yourself: “How can I make this my own? How can I extend this concept to solve a problem I care about?” By doing so, you’ll not only become a better programmer but also build a portfolio that truly represents your skills and passion for coding.
Happy coding, and may your personal projects open doors to exciting opportunities in your programming journey!