Troubleshooting Common Coding Errors: A Comprehensive Guide for Beginners
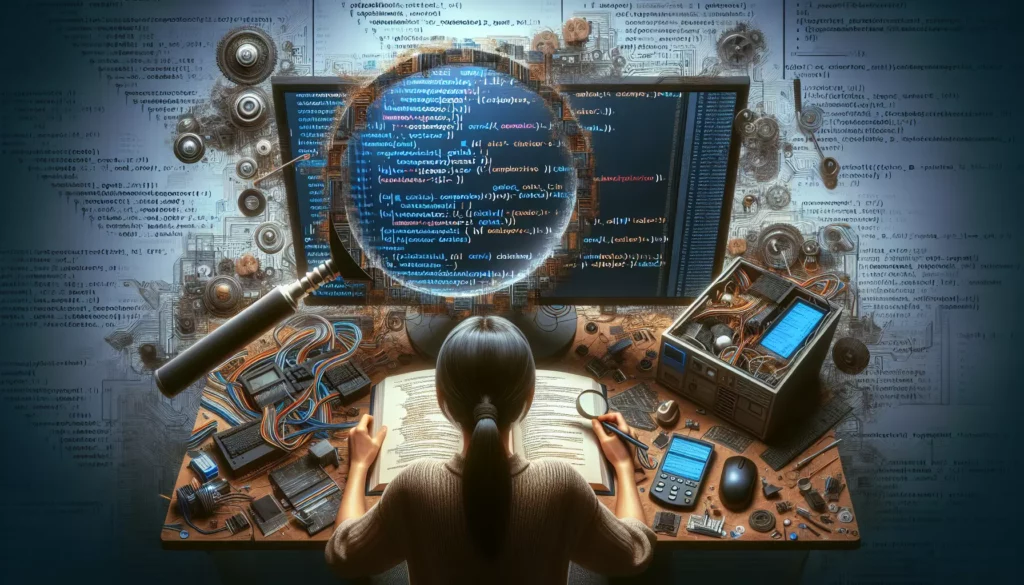
As you embark on your coding journey, you’ll quickly realize that encountering errors is an integral part of the learning process. Whether you’re a complete novice or preparing for technical interviews at major tech companies, understanding how to troubleshoot common coding errors is a crucial skill. In this comprehensive guide, we’ll explore various types of errors you might encounter, strategies for identifying and fixing them, and tips to help you become a more efficient problem-solver.
1. Understanding Different Types of Errors
Before diving into specific troubleshooting techniques, it’s essential to understand the main categories of errors you might encounter:
1.1 Syntax Errors
Syntax errors occur when you violate the rules of the programming language you’re using. These are often caught by the compiler or interpreter and prevent your code from running. Common syntax errors include:
- Missing semicolons
- Unmatched parentheses, brackets, or braces
- Misspelled keywords
- Incorrect indentation (in languages like Python)
1.2 Runtime Errors
Runtime errors occur while your program is executing. These can cause your program to crash or produce unexpected results. Examples include:
- Division by zero
- Accessing an array index out of bounds
- Trying to use an undefined variable
- Stack overflow due to infinite recursion
1.3 Logical Errors
Logical errors are the trickiest to identify because they don’t necessarily cause your program to crash. Instead, they result in incorrect output or unexpected behavior. These errors stem from flaws in your algorithm or logic. Examples include:
- Using the wrong comparison operator (e.g., > instead of <)
- Off-by-one errors in loops
- Incorrect order of operations
- Misunderstanding the problem requirements
2. General Troubleshooting Strategies
Now that we’ve covered the main types of errors, let’s explore some general strategies for troubleshooting:
2.1 Read the Error Message
This might seem obvious, but many beginners overlook the importance of carefully reading error messages. Most programming languages provide detailed information about what went wrong and where. Pay attention to:
- The type of error
- The line number where the error occurred
- Any specific details or suggestions provided in the message
2.2 Use Print Statements or Logging
When dealing with logical errors or trying to understand the flow of your program, adding print statements can be incredibly helpful. This technique, often called “printf debugging,” allows you to see the values of variables at different points in your code. For example:
def calculate_average(numbers):
total = sum(numbers)
count = len(numbers)
print(f"Total: {total}, Count: {count}") # Debug print
average = total / count
return average
result = calculate_average([1, 2, 3, 4, 5])
print(f"Average: {result}")
2.3 Use a Debugger
While print statements are useful, learning to use a debugger is a more powerful and efficient way to troubleshoot your code. Debuggers allow you to:
- Set breakpoints to pause execution at specific lines
- Step through your code line by line
- Inspect variable values at runtime
- Evaluate expressions in the current context
Most modern Integrated Development Environments (IDEs) come with built-in debuggers, so take some time to familiarize yourself with this tool.
2.4 Rubber Duck Debugging
This technique involves explaining your code, line by line, to an inanimate object (like a rubber duck). The act of verbalizing your thought process often helps you spot errors or inconsistencies in your logic. If you don’t have a rubber duck handy, explaining your code to a fellow programmer or even writing it out can be just as effective.
2.5 Divide and Conquer
When dealing with a complex problem or a large codebase, try to isolate the issue by breaking your code into smaller, testable parts. This approach can help you pinpoint where the error is occurring more quickly.
3. Troubleshooting Specific Types of Errors
Now, let’s look at some specific strategies for each type of error we discussed earlier:
3.1 Dealing with Syntax Errors
Syntax errors are often the easiest to fix, as they’re usually caught by your compiler or interpreter. Here are some tips:
- Check for missing semicolons: In languages that require semicolons (like JavaScript or Java), forgetting to add them is a common mistake.
- Ensure proper indentation: In Python, incorrect indentation can lead to syntax errors. Use spaces or tabs consistently.
- Match parentheses and braces: Use your IDE’s bracket matching feature to ensure all opening brackets have a corresponding closing bracket.
- Verify keyword spelling: Double-check the spelling of language keywords and function names.
Example of a syntax error in Python:
def calculate_sum(a, b)
return a + b # Missing colon after function definition
print(calculate_sum(5, 3))
Corrected version:
def calculate_sum(a, b): # Added colon
return a + b
print(calculate_sum(5, 3))
3.2 Addressing Runtime Errors
Runtime errors can be trickier to diagnose, as they occur during program execution. Here are some common runtime errors and how to address them:
3.2.1 Division by Zero
Always check if a divisor could potentially be zero before performing division:
def safe_divide(a, b):
if b == 0:
return "Error: Division by zero"
return a / b
print(safe_divide(10, 2)) # Output: 5.0
print(safe_divide(10, 0)) # Output: Error: Division by zero
3.2.2 Index Out of Range
When working with arrays or lists, ensure that you’re not trying to access an index that doesn’t exist:
def get_element(arr, index):
if 0 <= index < len(arr):
return arr[index]
return "Error: Index out of range"
my_list = [1, 2, 3, 4, 5]
print(get_element(my_list, 2)) # Output: 3
print(get_element(my_list, 10)) # Output: Error: Index out of range
3.2.3 Undefined Variable
Always initialize variables before using them. In some languages, you can use try-except blocks to handle potential NameError exceptions:
def print_variable(var_name):
try:
print(f"{var_name} = {eval(var_name)}")
except NameError:
print(f"Error: {var_name} is not defined")
x = 10
print_variable("x") # Output: x = 10
print_variable("y") # Output: Error: y is not defined
3.3 Tackling Logical Errors
Logical errors are often the most challenging to identify and fix. Here are some strategies to help you tackle them:
3.4.1 Use Assertions
Assertions can help you catch logical errors by verifying that certain conditions are met at specific points in your code:
def calculate_average(numbers):
assert len(numbers) > 0, "List cannot be empty"
total = sum(numbers)
average = total / len(numbers)
assert 0 <= average <= max(numbers), "Average out of expected range"
return average
print(calculate_average([1, 2, 3, 4, 5])) # Output: 3.0
# print(calculate_average([])) # Raises AssertionError: List cannot be empty
3.4.2 Write Unit Tests
Developing a habit of writing unit tests for your functions can help you catch logical errors early. Here’s a simple example using Python’s unittest module:
import unittest
def is_prime(n):
if n < 2:
return False
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
return False
return True
class TestPrime(unittest.TestCase):
def test_prime_numbers(self):
self.assertTrue(is_prime(2))
self.assertTrue(is_prime(3))
self.assertTrue(is_prime(5))
self.assertTrue(is_prime(17))
def test_non_prime_numbers(self):
self.assertFalse(is_prime(1))
self.assertFalse(is_prime(4))
self.assertFalse(is_prime(15))
self.assertFalse(is_prime(100))
if __name__ == "__main__":
unittest.main()
3.4.3 Use Code Reviews
Having another programmer review your code can often help identify logical errors that you might have missed. Fresh eyes can bring new perspectives and catch flaws in your reasoning.
4. Common Pitfalls and How to Avoid Them
As you progress in your coding journey, you’ll encounter some common pitfalls. Being aware of these can help you avoid them or recognize them quickly when they occur:
4.1 Off-by-One Errors
These errors occur when you’re iterating over a sequence and either miss the first/last element or go one step too far. To avoid them:
- Be clear about whether your ranges are inclusive or exclusive
- Use < instead of <= when appropriate
- Pay attention to zero-based vs. one-based indexing
Example:
def print_list_items(lst):
for i in range(len(lst)): # Correct: range(len(lst)) goes from 0 to len(lst)-1
print(lst[i])
my_list = ["a", "b", "c"]
print_list_items(my_list)
4.2 Infinite Loops
Infinite loops occur when the loop condition never becomes false. To avoid them:
- Ensure your loop has a clear termination condition
- Make sure the loop variable is being updated correctly
- Consider using a ‘break’ statement as a safeguard
Example of preventing an infinite loop:
def find_element(lst, target, max_iterations=1000):
i = 0
while i < len(lst):
if lst[i] == target:
return i
i += 1
if i >= max_iterations:
print("Warning: Max iterations reached")
break
return -1
print(find_element([1, 2, 3, 4, 5], 3)) # Output: 2
print(find_element([1, 2, 3, 4, 5], 6)) # Output: -1 (with warning if list is very large)
4.3 Mutable Default Arguments
In Python, using mutable objects (like lists or dictionaries) as default arguments can lead to unexpected behavior. To avoid this:
- Use None as the default value and create the mutable object inside the function
Example of the problem and solution:
# Problematic code
def add_item(item, lst=[]):
lst.append(item)
return lst
print(add_item(1)) # Output: [1]
print(add_item(2)) # Output: [1, 2] (Unexpected!)
# Corrected code
def add_item_safe(item, lst=None):
if lst is None:
lst = []
lst.append(item)
return lst
print(add_item_safe(1)) # Output: [1]
print(add_item_safe(2)) # Output: [2]
5. Advanced Debugging Techniques
As you tackle more complex projects, you may need to employ more advanced debugging techniques:
5.1 Logging
While print statements are useful for quick debugging, logging provides a more structured and flexible way to track what’s happening in your code. Most programming languages have built-in logging modules. Here’s an example using Python’s logging module:
import logging
logging.basicConfig(level=logging.DEBUG, format='%(asctime)s - %(levelname)s - %(message)s')
def complex_function(x, y):
logging.info(f"Starting complex_function with x={x}, y={y}")
result = 0
try:
result = x / y
logging.debug(f"Division result: {result}")
except ZeroDivisionError:
logging.error("Division by zero attempted")
return None
if result > 100:
logging.warning("Result is unusually large")
logging.info("complex_function completed successfully")
return result
print(complex_function(10, 2))
print(complex_function(100, 0))
print(complex_function(1000, 2))
5.2 Profiling
When dealing with performance issues, profiling your code can help identify bottlenecks. Many languages offer profiling tools. Here’s a simple example using Python’s cProfile module:
import cProfile
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
cProfile.run('fibonacci(30)')
5.3 Memory Leak Detection
In languages without automatic garbage collection, or when dealing with resource management, memory leaks can be a serious issue. Tools like Valgrind for C/C++ or memory_profiler for Python can help identify these problems.
5.4 Remote Debugging
When debugging applications running on remote servers or in different environments, remote debugging can be invaluable. Most IDEs support remote debugging, allowing you to step through code running on a different machine.
6. Developing a Debugging Mindset
Becoming proficient at troubleshooting isn’t just about knowing specific techniques; it’s also about developing the right mindset:
6.1 Stay Calm and Logical
When faced with a stubborn bug, it’s easy to get frustrated. Remember that every bug has a logical explanation, even if it’s not immediately apparent. Take a deep breath and approach the problem systematically.
6.2 Don’t Make Assumptions
Always verify your assumptions. Just because a piece of code worked before doesn’t mean it’s not the source of your current problem. Be willing to question every part of your code.
6.3 Learn from Your Mistakes
Keep a “bug journal” where you document the errors you encounter, how you solved them, and what you learned. This can be an invaluable resource as you progress in your coding journey.
6.4 Embrace the Process
Debugging is not just about fixing errors; it’s an opportunity to deepen your understanding of your code, the programming language, and computer science concepts in general. Embrace it as a learning experience.
7. Conclusion
Troubleshooting coding errors is an essential skill for any programmer, from beginners to those preparing for technical interviews at major tech companies. By understanding different types of errors, employing effective debugging strategies, and developing the right mindset, you can become more efficient at identifying and fixing issues in your code.
Remember, the ability to troubleshoot effectively is often what separates good programmers from great ones. It’s not about never making mistakes; it’s about having the tools and knowledge to find and fix those mistakes quickly and effectively.
As you continue your coding journey, whether you’re using platforms like AlgoCademy or working on personal projects, make debugging a fundamental part of your learning process. Embrace the challenges, stay curious, and keep coding!