Trie (Prefix Tree): A Powerful Data Structure for Efficient String Operations
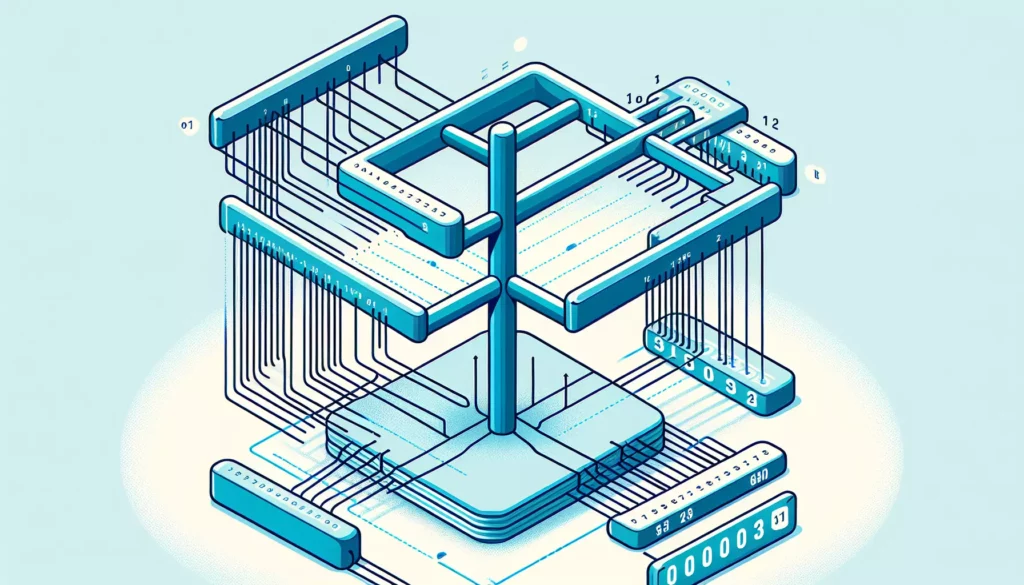
In the world of computer science and algorithmic problem-solving, efficient data structures play a crucial role in optimizing operations and improving overall performance. One such powerful data structure that often comes up in technical interviews and real-world applications is the Trie, also known as a Prefix Tree. In this comprehensive guide, we’ll dive deep into the Trie data structure, exploring its concepts, implementation, and various applications.
What is a Trie?
A Trie, derived from the word “retrieval,” is a tree-like data structure used to store and search strings efficiently. It is particularly useful for tasks involving prefix matching, autocomplete functionality, and dictionary operations. The Trie structure allows for quick insertion, deletion, and lookup of strings, making it an excellent choice for many string-related problems.
The key characteristics of a Trie include:
- Each node in the Trie represents a single character of a string.
- The root node is typically empty.
- Each path from the root to a node represents a prefix of one or more strings.
- Leaf nodes or specially marked nodes indicate the end of a complete word.
Basic Structure of a Trie Node
Before we dive into the implementation details, let’s understand the basic structure of a Trie node. A typical Trie node consists of:
- A boolean flag to indicate if the node represents the end of a word
- An array or map to store child nodes, typically with a size equal to the alphabet size
Here’s a simple representation of a Trie node in Python:
class TrieNode:
def __init__(self):
self.is_end_of_word = False
self.children = {} # Using a dictionary for flexibility
Implementing a Trie in Python
Now that we understand the basic structure, let’s implement a Trie class with essential operations like insertion, search, and prefix search. We’ll use Python for this implementation, but the concepts can be easily adapted to other programming languages.
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end_of_word = True
def search(self, word):
node = self.root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_end_of_word
def starts_with(self, prefix):
node = self.root
for char in prefix:
if char not in node.children:
return False
node = node.children[char]
return True
Let’s break down each method:
1. Insertion (insert)
The insert method adds a new word to the Trie. It iterates through each character of the word, creating new nodes if they don’t exist, and marks the last node as the end of a word.
2. Search (search)
The search method checks if a word exists in the Trie. It traverses the Trie following the characters of the word and returns True if the last node is marked as the end of a word.
3. Prefix Search (starts_with)
The starts_with method checks if there are any words in the Trie that start with the given prefix. It’s similar to the search method but doesn’t check for the end of word flag.
Time and Space Complexity
Understanding the time and space complexity of Trie operations is crucial for evaluating its efficiency:
Time Complexity:
- Insertion: O(m), where m is the length of the word being inserted
- Search: O(m), where m is the length of the word being searched
- Prefix Search: O(p), where p is the length of the prefix
Space Complexity:
The space complexity of a Trie depends on the number of nodes, which is influenced by the total number of characters in all inserted words and the amount of prefix overlap. In the worst case, where there’s no overlap between words, the space complexity can be O(n*m), where n is the number of words and m is the average word length.
Advantages of Using a Trie
Tries offer several advantages over other data structures for string-related operations:
- Efficient Prefix Matching: Tries excel at prefix-based operations, making them ideal for autocomplete and spell-check features.
- Fast Lookup: Searching for a word or prefix is independent of the number of words in the Trie, depending only on the word’s length.
- Space Efficiency for Common Prefixes: Tries can be space-efficient when storing words with common prefixes, as the common parts are stored only once.
- Alphabetical Ordering: Words in a Trie are naturally stored in alphabetical order, which can be useful for certain applications.
Real-World Applications of Tries
Tries find applications in various domains and scenarios:
1. Autocomplete and Search Suggestions
Tries are extensively used in implementing autocomplete features in search engines, text editors, and mobile keyboards. They allow for quick retrieval of words or phrases starting with a given prefix.
2. IP Routing Tables
In computer networking, Tries are used to implement IP routing tables, allowing for efficient longest prefix matching of IP addresses.
3. Spell Checkers
Tries can be used to implement spell-checking algorithms, where words from a dictionary are stored in a Trie for quick lookup and suggestion of corrections.
4. Dictionary Implementation
Tries provide an efficient way to implement dictionaries, especially for applications requiring prefix-based searches or autocompletion.
5. Genomic Sequence Analysis
In bioinformatics, Tries can be used to store and analyze DNA sequences, allowing for efficient pattern matching and sequence alignment.
Advanced Trie Concepts and Optimizations
As you delve deeper into Tries, you’ll encounter advanced concepts and optimizations that can further enhance their efficiency and applicability:
1. Compressed Trie (Radix Tree)
A compressed Trie, also known as a Radix Tree, is an optimization where nodes with only one child are merged with their parent. This reduces the space requirement and can improve traversal time for certain operations.
2. Ternary Search Tree
A Ternary Search Tree is a type of Trie where each node has three children (left, middle, and right) instead of potentially 26 (for lowercase English letters). This can be more space-efficient for sparse datasets.
3. Suffix Trie
A Suffix Trie is a Trie that contains all suffixes of a given string. It’s particularly useful for pattern matching problems and is often used in conjunction with suffix arrays.
4. Burst Tries
Burst Tries combine the space efficiency of BSTs with the cache efficiency of arrays, providing a good balance between memory usage and access speed.
Implementing Advanced Trie Features
Let’s enhance our basic Trie implementation with some additional features that are often useful in practice:
1. Delete Operation
Implementing a delete operation in a Trie can be tricky. We need to remove the word while ensuring we don’t delete parts of other words. Here’s an implementation:
class Trie:
# ... (previous methods)
def delete(self, word):
def _delete_helper(node, word, index):
if index == len(word):
if not node.is_end_of_word:
return False
node.is_end_of_word = False
return len(node.children) == 0
char = word[index]
if char not in node.children:
return False
should_delete_current_node = _delete_helper(node.children[char], word, index + 1)
if should_delete_current_node:
del node.children[char]
return len(node.children) == 0
return False
return _delete_helper(self.root, word, 0)
2. Autocomplete Feature
An autocomplete feature suggests words based on a given prefix. Here’s how we can implement it:
class Trie:
# ... (previous methods)
def autocomplete(self, prefix):
node = self.root
for char in prefix:
if char not in node.children:
return []
node = node.children[char]
suggestions = []
self._dfs(node, prefix, suggestions)
return suggestions
def _dfs(self, node, current_prefix, suggestions):
if node.is_end_of_word:
suggestions.append(current_prefix)
for char, child_node in node.children.items():
self._dfs(child_node, current_prefix + char, suggestions)
3. Counting Words with a Given Prefix
This feature can be useful in various applications. Let’s implement it:
class TrieNode:
def __init__(self):
self.is_end_of_word = False
self.children = {}
self.count = 0 # Count of words with this prefix
class Trie:
# ... (previous methods)
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.count += 1 # Increment count for each prefix
node.is_end_of_word = True
def count_words_with_prefix(self, prefix):
node = self.root
for char in prefix:
if char not in node.children:
return 0
node = node.children[char]
return node.count
Common Interview Questions and Problem-Solving with Tries
Tries are a popular topic in coding interviews, especially for positions at major tech companies. Here are some common problems and how to approach them using Tries:
1. Implement an Autocomplete System
This is a classic Trie problem. You can use the autocomplete method we implemented earlier as a starting point.
2. Word Search II (Leetcode 212)
Given a 2D board of characters and a list of words, find all words on the board. This problem can be efficiently solved using a Trie to store the words and then performing a DFS on the board.
3. Longest Common Prefix (Leetcode 14)
While this can be solved without a Trie, using one can make the solution more efficient, especially for a large number of strings.
4. Design Add and Search Words Data Structure (Leetcode 211)
This problem involves implementing a data structure that supports adding words and searching for words, including with wildcards. A Trie is an excellent choice for this.
5. Stream of Characters (Leetcode 1032)
This problem involves checking if a stream of characters forms a word from a given word list. A Trie can be used to efficiently store the words and check the stream.
Best Practices and Tips for Working with Tries
As you work with Tries in your projects or prepare for interviews, keep these best practices and tips in mind:
- Understand the Trade-offs: While Tries are excellent for certain operations, they might not always be the best choice. Consider the space-time trade-offs for your specific use case.
- Optimize for Space: If memory is a concern, consider using compressed Tries or other space-saving variants.
- Handle Edge Cases: Always consider edge cases like empty strings, very long strings, or characters outside your expected alphabet.
- Consider Case Sensitivity: Decide how you want to handle uppercase and lowercase letters. You might want to convert all input to lowercase for consistency.
- Use Appropriate Data Structures: Choose the right data structure for child nodes based on your alphabet size and expected data distribution.
- Implement Helper Methods: Methods like isLeaf() or hasChildren() can make your code more readable and maintainable.
- Practice Recursion: Many Trie operations are naturally recursive. Get comfortable with recursive implementations.
Conclusion
Tries are a powerful and versatile data structure that excel in string-related operations. Their efficiency in prefix-based searches and space-saving capabilities for common prefixes make them invaluable in various applications, from autocomplete systems to IP routing tables.
As you continue your journey in algorithm and data structure mastery, remember that understanding Tries is not just about memorizing their implementation. It’s about recognizing patterns in problems where Tries can provide efficient solutions. Practice implementing Tries from scratch, solve related problems, and explore their applications in real-world scenarios.
Whether you’re preparing for technical interviews at top tech companies or working on projects that involve extensive string operations, a solid grasp of Tries will undoubtedly be a valuable asset in your programming toolkit. Keep exploring, practicing, and pushing the boundaries of what you can achieve with this fascinating data structure!