Trees and Their Applications in Computer Science
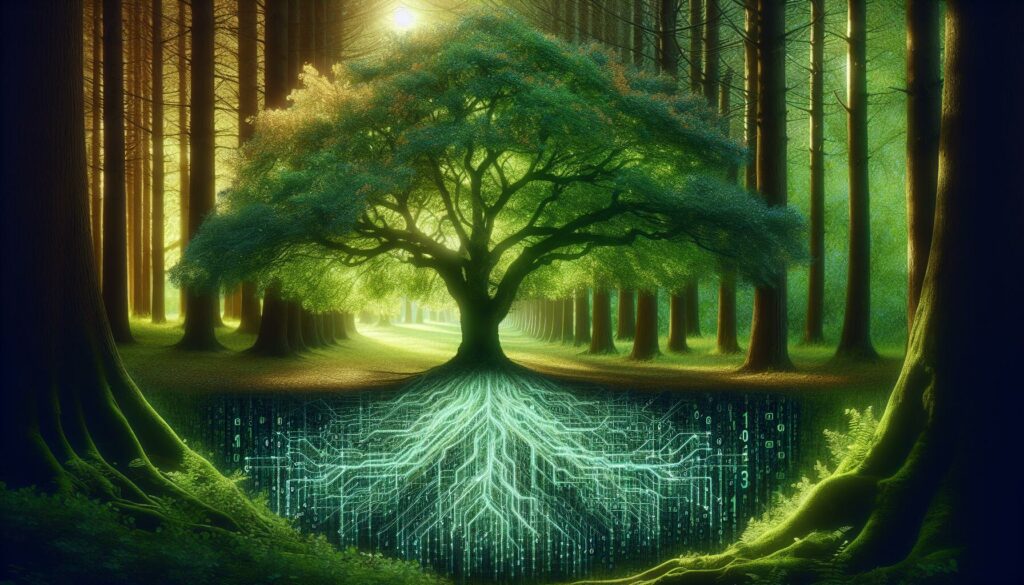
Trees are fundamental data structures in computer science, playing a crucial role in various algorithms and applications. Their hierarchical nature makes them ideal for representing and organizing data in a wide range of scenarios. In this comprehensive guide, we’ll explore the concept of trees, their types, and their practical applications in computer science and programming.
Table of Contents
- Introduction to Trees
- Types of Trees
- Applications of Trees in Computer Science
- Implementing Trees in Code
- Common Tree Algorithms
- Trees in Technical Interviews
- Advanced Tree Concepts
- Conclusion
1. Introduction to Trees
A tree is a hierarchical data structure consisting of nodes connected by edges. It’s similar to a real tree, but inverted, with the root at the top and branches extending downwards. Here are some key terms associated with trees:
- Root: The topmost node of the tree.
- Parent: A node that has child nodes.
- Child: A node directly connected to another node when moving away from the root.
- Leaf: A node with no children.
- Siblings: Nodes that share the same parent.
- Depth: The number of edges from the root to a node.
- Height: The number of edges on the longest path from a node to a leaf.
Trees are widely used in computer science due to their efficiency in organizing and retrieving data. They provide a natural way to represent hierarchical relationships and are the foundation for many algorithms and data structures.
2. Types of Trees
There are several types of trees, each with its own properties and use cases. Let’s explore some of the most common types:
2.1 Binary Trees
A binary tree is a tree data structure where each node has at most two children, referred to as the left child and the right child. Binary trees are widely used due to their simplicity and efficiency in various applications.
2.2 Binary Search Trees (BST)
A binary search tree is a special type of binary tree that maintains a specific ordering property: for each node, all elements in its left subtree are less than the node, and all elements in its right subtree are greater than the node. This property makes BSTs efficient for searching, inserting, and deleting elements.
2.3 AVL Trees
AVL trees are self-balancing binary search trees. They maintain a balance factor for each node, ensuring that the heights of the left and right subtrees differ by at most one. This balancing property guarantees O(log n) time complexity for search, insert, and delete operations.
2.4 Red-Black Trees
Red-black trees are another type of self-balancing binary search tree. They use color properties (red and black) and a set of rules to maintain balance. Red-black trees provide efficient worst-case guarantees for insertion, deletion, and search operations.
2.5 B-Trees
B-trees are self-balancing search trees designed to work efficiently with secondary storage devices. They can have more than two children per node and are commonly used in databases and file systems to organize large amounts of data.
2.6 Trie (Prefix Tree)
A trie, also called a prefix tree, is a tree-like data structure used to store and retrieve strings. Each node in a trie represents a character, and the path from the root to a node forms a prefix of the stored strings. Tries are particularly useful for tasks like autocomplete and spell-checking.
3. Applications of Trees in Computer Science
Trees have numerous applications in computer science and software development. Here are some key areas where trees play a crucial role:
3.1 File Systems
Computer file systems use tree structures to organize directories and files. The root directory serves as the tree’s root, with subdirectories and files as child nodes. This hierarchical organization allows for efficient file management and retrieval.
3.2 Database Indexing
B-trees and their variants are commonly used in database systems for indexing. They provide fast access to data on disk, allowing for efficient querying and updating of large datasets.
3.3 Expression Parsing
Abstract Syntax Trees (ASTs) are used in compilers and interpreters to represent the structure of program code. These trees help in parsing, analyzing, and evaluating expressions and statements in programming languages.
3.4 Network Routing
Routing algorithms in computer networks often use tree-like structures to determine the best path for data packets. Spanning trees, for example, are used to prevent loops in network topologies.
3.5 AI and Machine Learning
Decision trees are widely used in machine learning for classification and regression tasks. They form the basis for powerful ensemble methods like Random Forests and Gradient Boosting Machines.
3.6 Compression Algorithms
Huffman coding, a popular data compression technique, uses a binary tree to assign variable-length codes to characters based on their frequency of occurrence.
4. Implementing Trees in Code
Let’s look at how to implement a basic binary tree structure in Python:
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinaryTree:
def __init__(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = TreeNode(value)
else:
self._insert_recursive(self.root, value)
def _insert_recursive(self, node, value):
if value < node.value:
if node.left is None:
node.left = TreeNode(value)
else:
self._insert_recursive(node.left, value)
else:
if node.right is None:
node.right = TreeNode(value)
else:
self._insert_recursive(node.right, value)
def inorder_traversal(self):
return self._inorder_recursive(self.root)
def _inorder_recursive(self, node):
if node is None:
return []
return (self._inorder_recursive(node.left) +
[node.value] +
self._inorder_recursive(node.right))
# Usage example
tree = BinaryTree()
tree.insert(5)
tree.insert(3)
tree.insert(7)
tree.insert(1)
tree.insert(9)
print(tree.inorder_traversal()) # Output: [1, 3, 5, 7, 9]
This implementation creates a basic binary search tree with insertion and in-order traversal capabilities. The TreeNode
class represents individual nodes, while the BinaryTree
class manages the tree structure and operations.
5. Common Tree Algorithms
Understanding and implementing tree algorithms is crucial for working with tree data structures effectively. Here are some common tree algorithms you should be familiar with:
5.1 Tree Traversals
Tree traversals are methods for visiting all nodes in a tree. The three main types of traversals are:
- In-order traversal: Visit left subtree, root, then right subtree.
- Pre-order traversal: Visit root, left subtree, then right subtree.
- Post-order traversal: Visit left subtree, right subtree, then root.
Here’s an example of implementing all three traversals:
def inorder_traversal(node):
if node:
yield from inorder_traversal(node.left)
yield node.value
yield from inorder_traversal(node.right)
def preorder_traversal(node):
if node:
yield node.value
yield from preorder_traversal(node.left)
yield from preorder_traversal(node.right)
def postorder_traversal(node):
if node:
yield from postorder_traversal(node.left)
yield from postorder_traversal(node.right)
yield node.value
# Usage
tree = BinaryTree()
# ... insert nodes ...
print(list(inorder_traversal(tree.root)))
print(list(preorder_traversal(tree.root)))
print(list(postorder_traversal(tree.root)))
5.2 Depth-First Search (DFS) and Breadth-First Search (BFS)
DFS and BFS are two fundamental graph traversal algorithms that can be applied to trees:
- DFS: Explores as far as possible along each branch before backtracking.
- BFS: Explores all neighbors at the present depth before moving to nodes at the next depth level.
Here’s a BFS implementation for a binary tree:
from collections import deque
def bfs(root):
if not root:
return []
result = []
queue = deque([root])
while queue:
node = queue.popleft()
result.append(node.value)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
return result
# Usage
tree = BinaryTree()
# ... insert nodes ...
print(bfs(tree.root))
5.3 Finding the Lowest Common Ancestor (LCA)
The Lowest Common Ancestor of two nodes in a tree is the deepest node that has both nodes as descendants. This algorithm is useful in various applications, including computational biology and network routing.
def lowest_common_ancestor(root, p, q):
if not root or root.value == p or root.value == q:
return root
left = lowest_common_ancestor(root.left, p, q)
right = lowest_common_ancestor(root.right, p, q)
if left and right:
return root
return left if left else right
# Usage
tree = BinaryTree()
# ... insert nodes ...
lca = lowest_common_ancestor(tree.root, 3, 7)
print(f"LCA of 3 and 7 is: {lca.value}")
6. Trees in Technical Interviews
Trees are a popular topic in technical interviews, especially for positions at major tech companies. Here are some common tree-related questions you might encounter:
- Implement a binary search tree with insert, delete, and search operations.
- Check if a binary tree is balanced.
- Find the maximum depth of a binary tree.
- Determine if two binary trees are identical.
- Convert a sorted array to a balanced binary search tree.
- Implement a trie (prefix tree) with insert, search, and startsWith methods.
- Serialize and deserialize a binary tree.
- Find the kth smallest element in a BST.
- Implement a binary heap (min-heap or max-heap).
- Solve the “Lowest Common Ancestor” problem for a binary tree.
To prepare for these types of questions, practice implementing various tree algorithms and solving problems on platforms like LeetCode, HackerRank, or AlgoCademy. Focus on understanding the time and space complexity of your solutions, as well as edge cases and potential optimizations.
7. Advanced Tree Concepts
As you progress in your understanding of trees, you’ll encounter more advanced concepts and specialized tree structures. Here are some topics to explore:
7.1 Segment Trees
Segment trees are used for storing information about intervals or segments. They allow for efficient query processing on ranges, such as finding the minimum, maximum, or sum of elements in a given range.
7.2 Fenwick Trees (Binary Indexed Trees)
Fenwick trees provide an efficient way to maintain cumulative frequency tables and perform range sum queries. They are particularly useful in scenarios involving dynamic range queries and updates.
7.3 Splay Trees
Splay trees are self-adjusting binary search trees that move recently accessed elements closer to the root. This property makes them efficient for scenarios with repeated accesses to the same elements.
7.4 Treaps
Treaps combine the properties of binary search trees and heaps. Each node has both a key and a priority, maintaining the BST property for keys and the heap property for priorities.
7.5 Suffix Trees
Suffix trees are compressed trie structures used for efficient string matching and analysis. They have applications in bioinformatics, text processing, and data compression.
7.6 Merkle Trees
Merkle trees, also known as hash trees, are used in cryptography and blockchain technology. They allow efficient and secure verification of large data structures.
Understanding these advanced tree structures and their applications can significantly enhance your problem-solving skills and broaden your perspective on data structure design.
8. Conclusion
Trees are versatile and powerful data structures that form the backbone of many algorithms and applications in computer science. From organizing file systems to optimizing database queries and powering machine learning algorithms, trees play a crucial role in modern software development.
As you continue your journey in coding education and programming skills development, mastering tree concepts and algorithms will prove invaluable. Practice implementing different types of trees, solve tree-related problems, and explore their applications in real-world scenarios. This knowledge will not only prepare you for technical interviews at major tech companies but also enhance your overall problem-solving abilities as a software developer.
Remember that understanding trees is not just about memorizing algorithms; it’s about developing a intuition for hierarchical data structures and recognizing patterns in problem-solving. As you work with trees, try to visualize their structure and think about how their properties can be leveraged to solve complex problems efficiently.
Keep exploring, practicing, and building projects that incorporate tree data structures. With dedication and consistent effort, you’ll develop a strong foundation in this fundamental area of computer science, setting yourself up for success in your programming career.