Trees and Graphs: Fundamental Data Structures for Coders
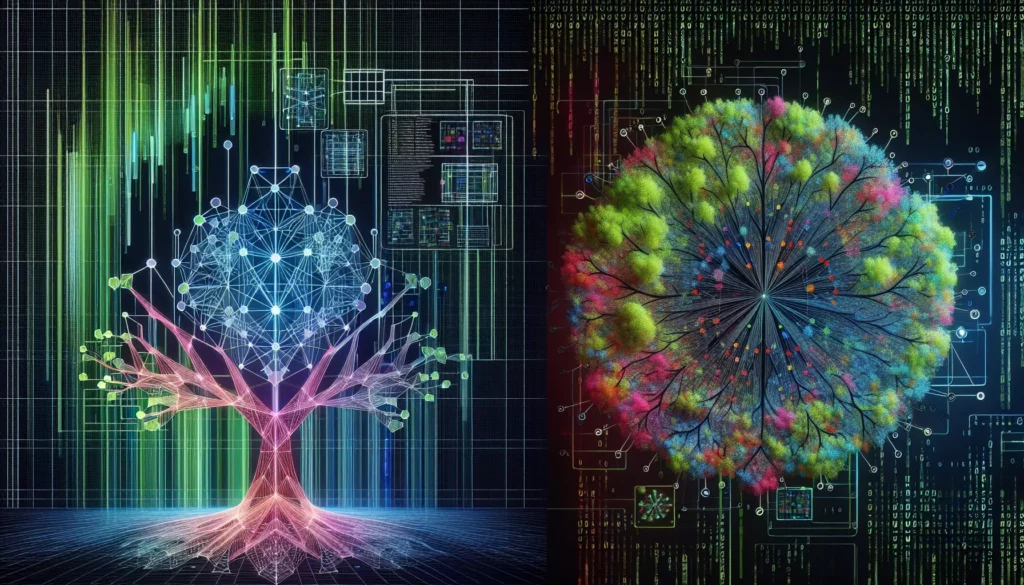
In the world of computer science and programming, data structures play a crucial role in organizing and managing information efficiently. Among these structures, trees and graphs stand out as fundamental concepts that every coder should master. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at major tech companies, understanding trees and graphs is essential. In this comprehensive guide, we’ll explore these powerful data structures, their applications, and how they can enhance your problem-solving skills.
1. Introduction to Trees
Trees are hierarchical data structures that consist of nodes connected by edges. They are widely used in computer science due to their ability to represent hierarchical relationships and organize data in a way that allows for efficient searching and sorting.
1.1 Basic Tree Terminology
- Root: The topmost node of the tree.
- Parent: A node that has child nodes.
- Child: A node directly connected to another node when moving away from the root.
- Leaf: A node with no children.
- Subtree: A tree structure formed by a node and its descendants.
- Depth: The number of edges from the root to a particular node.
- Height: The maximum depth of any node in the tree.
1.2 Types of Trees
There are several types of trees, each with its own characteristics and use cases:
1.2.1 Binary Trees
A binary tree is a tree data structure where each node has at most two children, referred to as the left child and the right child. Binary trees are widely used in various applications and serve as the foundation for more complex tree structures.
1.2.2 Binary Search Trees (BST)
A binary search tree is a special type of binary tree that maintains a specific ordering property: for each node, all elements in its left subtree are less than the node’s value, and all elements in its right subtree are greater than the node’s value. This property allows for efficient searching, insertion, and deletion operations.
1.2.3 AVL Trees
AVL trees are self-balancing binary search trees. They maintain a balance factor for each node, ensuring that the heights of the left and right subtrees differ by at most one. This balance property guarantees efficient operations with a time complexity of O(log n) for search, insertion, and deletion.
1.2.4 Red-Black Trees
Red-black trees are another type of self-balancing binary search tree. They use color properties (red and black) and a set of rules to maintain balance. Red-black trees provide efficient worst-case guarantees for insertion, deletion, and searching operations.
1.2.5 B-Trees
B-trees are self-balancing search trees designed to work efficiently with secondary storage devices. They can have more than two children per node and are commonly used in database systems and file systems to manage large amounts of data.
2. Tree Traversal Algorithms
Tree traversal is the process of visiting all the nodes in a tree data structure. There are several common traversal algorithms:
2.1 Depth-First Search (DFS)
DFS explores as far as possible along each branch before backtracking. There are three main types of DFS traversals:
- Pre-order: Visit the root, then traverse the left subtree, and finally traverse the right subtree.
- In-order: Traverse the left subtree, visit the root, and then traverse the right subtree.
- Post-order: Traverse the left subtree, traverse the right subtree, and finally visit the root.
Here’s an example implementation of DFS traversals in Python:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def preorder_traversal(root):
if root:
print(root.val, end=' ')
preorder_traversal(root.left)
preorder_traversal(root.right)
def inorder_traversal(root):
if root:
inorder_traversal(root.left)
print(root.val, end=' ')
inorder_traversal(root.right)
def postorder_traversal(root):
if root:
postorder_traversal(root.left)
postorder_traversal(root.right)
print(root.val, end=' ')
2.2 Breadth-First Search (BFS)
BFS explores all the nodes at the present depth before moving on to the nodes at the next depth level. This is typically implemented using a queue data structure.
Here’s an example implementation of BFS traversal in Python:
from collections import deque
def bfs_traversal(root):
if not root:
return
queue = deque([root])
while queue:
node = queue.popleft()
print(node.val, end=' ')
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
3. Introduction to Graphs
Graphs are versatile data structures that consist of a set of vertices (also called nodes) connected by edges. They are used to represent relationships between various entities and are fundamental in solving many real-world problems.
3.1 Basic Graph Terminology
- Vertex: A fundamental unit of a graph, representing an entity.
- Edge: A connection between two vertices.
- Directed Graph: A graph where edges have a direction.
- Undirected Graph: A graph where edges have no direction.
- Weighted Graph: A graph where edges have associated weights or costs.
- Path: A sequence of vertices connected by edges.
- Cycle: A path that starts and ends at the same vertex.
3.2 Graph Representations
There are two common ways to represent graphs in code:
3.2.1 Adjacency Matrix
An adjacency matrix is a 2D array where the cell at position (i, j) represents the edge between vertices i and j. This representation is efficient for dense graphs and allows for quick edge lookup.
class Graph:
def __init__(self, vertices):
self.V = vertices
self.graph = [[0 for _ in range(vertices)] for _ in range(vertices)]
def add_edge(self, u, v):
self.graph[u][v] = 1
self.graph[v][u] = 1 # For undirected graph
3.2.2 Adjacency List
An adjacency list uses a list or dictionary to store the neighbors of each vertex. This representation is more space-efficient for sparse graphs and is better for traversing all edges of a vertex.
from collections import defaultdict
class Graph:
def __init__(self):
self.graph = defaultdict(list)
def add_edge(self, u, v):
self.graph[u].append(v)
self.graph[v].append(u) # For undirected graph
4. Graph Traversal Algorithms
Graph traversal algorithms are used to visit all the vertices in a graph. The two main approaches are:
4.1 Depth-First Search (DFS) for Graphs
DFS explores as far as possible along each branch before backtracking. It can be implemented using recursion or a stack.
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start, end=' ')
for neighbor in graph[start]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
4.2 Breadth-First Search (BFS) for Graphs
BFS explores all the vertices at the present depth before moving on to the vertices at the next depth level. It is typically implemented using a queue.
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(vertex, end=' ')
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
5. Common Tree and Graph Algorithms
Trees and graphs are the foundation for many important algorithms in computer science. Here are some common algorithms that every coder should be familiar with:
5.1 Dijkstra’s Algorithm
Dijkstra’s algorithm is used to find the shortest path between nodes in a graph. It works on both directed and undirected graphs with non-negative edge weights.
import heapq
def dijkstra(graph, start):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
pq = [(0, start)]
while pq:
current_distance, current_node = heapq.heappop(pq)
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return distances
5.2 Prim’s Algorithm
Prim’s algorithm is used to find the minimum spanning tree of a weighted, undirected graph. It grows the spanning tree from a starting position.
import heapq
def prim(graph):
start_vertex = next(iter(graph))
mst = []
visited = set([start_vertex])
edges = [(cost, start_vertex, to) for to, cost in graph[start_vertex].items()]
heapq.heapify(edges)
while edges:
cost, frm, to = heapq.heappop(edges)
if to not in visited:
visited.add(to)
mst.append((frm, to, cost))
for next_to, next_cost in graph[to].items():
if next_to not in visited:
heapq.heappush(edges, (next_cost, to, next_to))
return mst
5.3 Kruskal’s Algorithm
Kruskal’s algorithm is another method for finding the minimum spanning tree of a weighted, undirected graph. It works by sorting all the edges and then adding them one by one to the spanning tree if they don’t create a cycle.
class UnionFind:
def __init__(self, vertices):
self.parent = {v: v for v in vertices}
self.rank = {v: 0 for v in vertices}
def find(self, item):
if self.parent[item] != item:
self.parent[item] = self.find(self.parent[item])
return self.parent[item]
def union(self, x, y):
xroot = self.find(x)
yroot = self.find(y)
if self.rank[xroot] < self.rank[yroot]:
self.parent[xroot] = yroot
elif self.rank[xroot] > self.rank[yroot]:
self.parent[yroot] = xroot
else:
self.parent[yroot] = xroot
self.rank[xroot] += 1
def kruskal(graph):
edges = [(cost, u, v) for u in graph for v, cost in graph[u].items()]
edges.sort()
vertices = list(graph.keys())
uf = UnionFind(vertices)
mst = []
for cost, u, v in edges:
if uf.find(u) != uf.find(v):
uf.union(u, v)
mst.append((u, v, cost))
return mst
5.4 Topological Sorting
Topological sorting is used to linearly order the vertices of a directed acyclic graph (DAG) such that for every directed edge (u, v), vertex u comes before v in the ordering. This algorithm is particularly useful in scheduling tasks with dependencies.
from collections import defaultdict
def topological_sort(graph):
def dfs(node):
visited.add(node)
for neighbor in graph[node]:
if neighbor not in visited:
dfs(neighbor)
stack.append(node)
visited = set()
stack = []
for node in graph:
if node not in visited:
dfs(node)
return stack[::-1]
# Example usage
graph = defaultdict(list)
graph[0].extend([1, 2])
graph[1].append(3)
graph[2].append(3)
print(topological_sort(graph)) # Output: [0, 2, 1, 3]
6. Advanced Tree and Graph Concepts
As you progress in your coding journey, you’ll encounter more advanced concepts related to trees and graphs. Here are a few important ones:
6.1 Trie (Prefix Tree)
A trie is an efficient tree-like data structure used for storing and searching strings. It’s particularly useful for tasks like autocomplete, spell checking, and IP routing tables.
class TrieNode:
def __init__(self):
self.children = {}
self.is_end_of_word = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end_of_word = True
def search(self, word):
node = self.root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_end_of_word
def starts_with(self, prefix):
node = self.root
for char in prefix:
if char not in node.children:
return False
node = node.children[char]
return True
6.2 Segment Tree
A segment tree is a tree data structure used for storing information about intervals or segments. It allows for efficient query operations over an array or list of values.
class SegmentTree:
def __init__(self, arr):
self.n = len(arr)
self.tree = [0] * (4 * self.n)
self.build(arr, 0, 0, self.n - 1)
def build(self, arr, node, start, end):
if start == end:
self.tree[node] = arr[start]
else:
mid = (start + end) // 2
self.build(arr, 2*node+1, start, mid)
self.build(arr, 2*node+2, mid+1, end)
self.tree[node] = self.tree[2*node+1] + self.tree[2*node+2]
def query(self, node, start, end, l, r):
if r < start or end < l:
return 0
if l <= start and end <= r:
return self.tree[node]
mid = (start + end) // 2
p1 = self.query(2*node+1, start, mid, l, r)
p2 = self.query(2*node+2, mid+1, end, l, r)
return p1 + p2
def update(self, node, start, end, idx, val):
if start == end:
self.tree[node] = val
else:
mid = (start + end) // 2
if start <= idx <= mid:
self.update(2*node+1, start, mid, idx, val)
else:
self.update(2*node+2, mid+1, end, idx, val)
self.tree[node] = self.tree[2*node+1] + self.tree[2*node+2]
6.3 Disjoint Set (Union-Find)
A disjoint set data structure, also known as a union-find data structure, is used to efficiently keep track of a partition of a set into disjoint subsets. It’s commonly used in Kruskal’s algorithm and for finding connected components in graphs.
class DisjointSet:
def __init__(self, vertices):
self.parent = {v: v for v in vertices}
self.rank = {v: 0 for v in vertices}
def find(self, item):
if self.parent[item] != item:
self.parent[item] = self.find(self.parent[item])
return self.parent[item]
def union(self, x, y):
xroot = self.find(x)
yroot = self.find(y)
if self.rank[xroot] < self.rank[yroot]:
self.parent[xroot] = yroot
elif self.rank[xroot] > self.rank[yroot]:
self.parent[yroot] = xroot
else:
self.parent[yroot] = xroot
self.rank[xroot] += 1
7. Practical Applications of Trees and Graphs
Trees and graphs are not just theoretical concepts; they have numerous practical applications in various fields of computer science and software development:
7.1 File Systems
File systems in operating systems are typically represented as tree structures, with directories as internal nodes and files as leaf nodes. This hierarchical organization allows for efficient file management and traversal.
7.2 Database Indexing
B-trees and their variants are commonly used in database systems for indexing. They allow for fast search, insertion, and deletion operations, which are crucial for database performance.
7.3 Network Routing
Graph algorithms like Dijkstra’s algorithm are used in network routing protocols to find the shortest path between nodes in a network. This is essential for efficient data transmission in computer networks and the internet.
7.4 Social Networks
Social networks are naturally represented as graphs, where users are vertices and connections (friendships, follows, etc.) are edges. Graph algorithms are used to analyze social connections, recommend friends, and detect communities.
7.5 Compiler Design
Abstract Syntax Trees (ASTs) are used in compiler design to represent the structure of program code. These trees are crucial for code analysis, optimization, and translation to machine code.
7.6 Machine Learning
Decision trees and their ensembles (like Random Forests) are popular machine learning models used for both classification and regression tasks. They provide interpretable results and can handle both numerical and categorical data.
8. Tips for Mastering Trees and Graphs
To become proficient in working with trees and graphs, consider the following tips:
- Practice Implementation: Implement various tree and graph data structures from scratch to understand their inner workings.
- Solve Problems: Practice solving tree and graph problems on coding platforms like LeetCode, HackerRank, or CodeForces.
- Visualize: Use visualization tools or draw diagrams to better understand complex tree and graph structures and algorithms.
- Understand Time and Space Complexity: Analyze the time and space complexity of tree and graph algorithms to choose the most efficient solution for a given problem.
- Learn Advanced Concepts: Once you’re comfortable with the basics, explore advanced concepts like balanced trees, graph coloring, and network flow algorithms.
- Apply to Real-World Problems: Try to identify and solve real-world problems using tree and graph concepts to reinforce your understanding.
9. Conclusion
Trees and graphs are fundamental data structures that form the backbone of many algorithms and applications in computer science. By mastering these concepts, you’ll significantly enhance your problem-solving skills and become a more versatile programmer. Whether you’re preparing for technical interviews at major tech companies or building complex software systems, a solid understanding of trees and graphs will serve you well throughout your coding career.
Remember that learning these concepts is an ongoing process. Start with the basics, practice regularly, and gradually tackle more complex problems and algorithms. With dedication and persistence, you’ll develop a deep understanding of trees and graphs, enabling you to solve a wide range of computational problems efficiently and elegantly.
As you continue your journey in coding education and programming skills development, keep exploring new applications of trees and graphs. They are not only essential for passing technical interviews but also for developing efficient and scalable solutions in real-world software engineering. Happy coding!