Tree Traversal: Mastering the Art of Navigating Data Structures
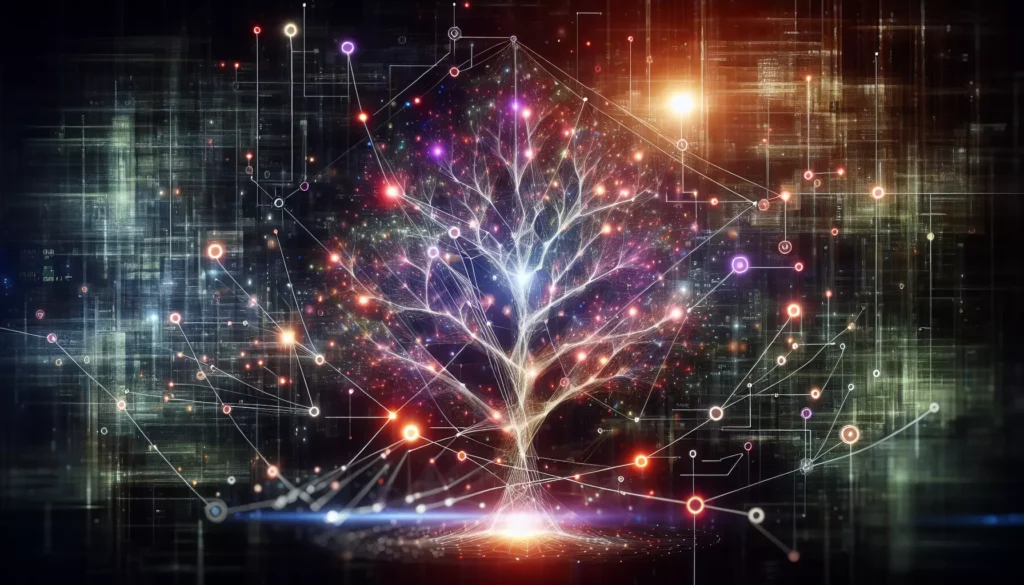
In the world of computer science and programming, understanding data structures is crucial for developing efficient algorithms and solving complex problems. Among these structures, trees stand out as versatile and powerful tools for organizing and representing hierarchical data. One of the fundamental operations performed on trees is traversal – the process of visiting each node in a specific order. In this comprehensive guide, we’ll dive deep into the concept of tree traversal, exploring various techniques, their implementations, and real-world applications.
What is Tree Traversal?
Tree traversal, also known as tree search, is the process of visiting (checking or updating) each node in a tree data structure exactly once. Unlike linear data structures such as arrays or linked lists, trees can be traversed in various ways. The specific traversal method chosen depends on the problem at hand and the desired outcome.
Before we delve into the different traversal techniques, let’s quickly review the basic structure of a tree:
- Node: The fundamental unit of a tree, containing data and references to its child nodes.
- Root: The topmost node of the tree, which has no parent.
- Child: A node directly connected to another node when moving away from the root.
- Parent: The converse notion of a child.
- Leaf: A node with no children.
Types of Tree Traversal
There are three main types of tree traversal for binary trees:
- In-order Traversal
- Pre-order Traversal
- Post-order Traversal
Additionally, there’s a fourth type called Level-order Traversal, which is commonly used for both binary and non-binary trees.
1. In-order Traversal
In-order traversal is defined as follows:
- Traverse the left subtree
- Visit the root
- Traverse the right subtree
This method is commonly used with binary search trees (BST) as it visits the nodes in ascending order.
Here’s a simple implementation of in-order traversal in Python:
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def in_order_traversal(root):
if root:
in_order_traversal(root.left)
print(root.value, end=' ')
in_order_traversal(root.right)
# Example usage
root = Node(1)
root.left = Node(2)
root.right = Node(3)
root.left.left = Node(4)
root.left.right = Node(5)
print("In-order traversal:")
in_order_traversal(root)
# Output: 4 2 5 1 3
2. Pre-order Traversal
Pre-order traversal follows this sequence:
- Visit the root
- Traverse the left subtree
- Traverse the right subtree
This method is useful for creating a copy of the tree or generating a prefix expression from an expression tree.
Here’s a Python implementation of pre-order traversal:
def pre_order_traversal(root):
if root:
print(root.value, end=' ')
pre_order_traversal(root.left)
pre_order_traversal(root.right)
# Example usage
print("\nPre-order traversal:")
pre_order_traversal(root)
# Output: 1 2 4 5 3
3. Post-order Traversal
Post-order traversal follows this sequence:
- Traverse the left subtree
- Traverse the right subtree
- Visit the root
This method is often used when deleting nodes from a tree or evaluating postfix expressions.
Here’s a Python implementation of post-order traversal:
def post_order_traversal(root):
if root:
post_order_traversal(root.left)
post_order_traversal(root.right)
print(root.value, end=' ')
# Example usage
print("\nPost-order traversal:")
post_order_traversal(root)
# Output: 4 5 2 3 1
4. Level-order Traversal
Level-order traversal, also known as breadth-first search (BFS), visits nodes level by level from top to bottom and left to right. This method is particularly useful for problems that require processing nodes closest to the root first.
Here’s a Python implementation of level-order traversal using a queue:
from collections import deque
def level_order_traversal(root):
if not root:
return
queue = deque([root])
while queue:
node = queue.popleft()
print(node.value, end=' ')
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
# Example usage
print("\nLevel-order traversal:")
level_order_traversal(root)
# Output: 1 2 3 4 5
Iterative vs. Recursive Implementations
While we’ve seen recursive implementations of tree traversals, it’s also possible to implement them iteratively. Iterative implementations can be more efficient in terms of memory usage, especially for large trees, as they don’t rely on the call stack.
Here’s an example of an iterative in-order traversal:
def iterative_in_order_traversal(root):
stack = []
current = root
while current or stack:
while current:
stack.append(current)
current = current.left
current = stack.pop()
print(current.value, end=' ')
current = current.right
# Example usage
print("\nIterative in-order traversal:")
iterative_in_order_traversal(root)
# Output: 4 2 5 1 3
Time and Space Complexity
For all the traversal methods discussed (in-order, pre-order, post-order, and level-order), the time complexity is O(n), where n is the number of nodes in the tree. This is because each node is visited exactly once.
The space complexity varies:
- For recursive implementations: O(h) where h is the height of the tree. In the worst case (a skewed tree), this can be O(n).
- For iterative implementations: O(h) for depth-first traversals (in-order, pre-order, post-order) and O(w) for breadth-first traversal (level-order), where w is the maximum width of the tree.
Applications of Tree Traversal
Tree traversal algorithms have numerous practical applications in computer science and software development:
- Expression Evaluation: In-order traversal of expression trees can generate infix expressions, while post-order traversal can evaluate expressions.
- File System Navigation: Pre-order traversal is often used to display file and directory structures.
- XML Parsing: Various traversal methods are used to parse and process XML documents, which have a tree-like structure.
- Compiler Design: Abstract Syntax Trees (ASTs) are traversed during various phases of compilation.
- Game AI: Decision trees in game AI often use different traversal methods to determine the best move.
- HTML DOM Manipulation: Web browsers use tree traversal to render and manipulate the Document Object Model (DOM).
Advanced Tree Traversal Techniques
Morris Traversal
Morris Traversal is an advanced technique that allows for in-order traversal of a binary tree using O(1) extra space. This method temporarily modifies the tree structure to traverse it without using a stack or recursion.
Here’s a Python implementation of Morris Traversal:
def morris_traversal(root):
current = root
while current:
if not current.left:
print(current.value, end=' ')
current = current.right
else:
pre = current.left
while pre.right and pre.right != current:
pre = pre.right
if not pre.right:
pre.right = current
current = current.left
else:
pre.right = None
print(current.value, end=' ')
current = current.right
# Example usage
print("\nMorris Traversal:")
morris_traversal(root)
# Output: 4 2 5 1 3
Threaded Binary Trees
Threaded binary trees are a variation of binary trees where “null” right pointers are replaced by pointers to the in-order successor of the node (if it exists). This allows for more efficient traversal without using a stack or recursion.
Challenges and Interview Questions
Tree traversal is a common topic in coding interviews. Here are some popular interview questions related to tree traversal:
- Binary Tree Zigzag Level Order Traversal: Given a binary tree, return the zigzag level order traversal of its nodes’ values.
- Construct Binary Tree from Preorder and Inorder Traversal: Given two integer arrays preorder and inorder where preorder is the preorder traversal of a binary tree and inorder is the inorder traversal of the same tree, construct and return the binary tree.
- Serialize and Deserialize Binary Tree: Design an algorithm to serialize and deserialize a binary tree.
- Boundary of Binary Tree: Given a binary tree, return the values of its boundary in anti-clockwise direction starting from the root.
- Vertical Order Traversal of a Binary Tree: Given a binary tree, return the vertical order traversal of its nodes values.
Best Practices and Tips
When working with tree traversals, keep these best practices in mind:
- Choose the Right Traversal: Select the traversal method that best suits your problem. For example, use in-order for BST operations, level-order for nearest neighbor problems, etc.
- Consider Space Complexity: For large trees, consider iterative implementations or advanced techniques like Morris Traversal to optimize space usage.
- Understand the Trade-offs: Recursive implementations are often more intuitive but may lead to stack overflow for very deep trees. Iterative implementations can be more complex but are generally more efficient.
- Practice with Different Tree Types: Don’t limit yourself to binary trees. Practice traversals on n-ary trees, threaded trees, and other variations.
- Combine Traversals: Some problems may require combining different traversal techniques or performing multiple passes over the tree.
Conclusion
Tree traversal is a fundamental concept in computer science and a crucial skill for any programmer. Whether you’re working on algorithm design, parsing data structures, or preparing for technical interviews, a solid understanding of tree traversal techniques is invaluable.
By mastering the various traversal methods – in-order, pre-order, post-order, and level-order – and understanding their implementations and applications, you’ll be well-equipped to tackle a wide range of programming challenges. Remember to practice regularly, explore advanced techniques, and always consider the specific requirements of your problem when choosing a traversal method.
As you continue your journey in programming and computer science, you’ll find that tree traversal is not just an academic concept, but a practical tool that you’ll use time and time again in real-world software development. Keep exploring, keep practicing, and watch as your ability to navigate and manipulate complex data structures grows!