Top Twitter (X) Interview Questions: Ace Your Technical Interview
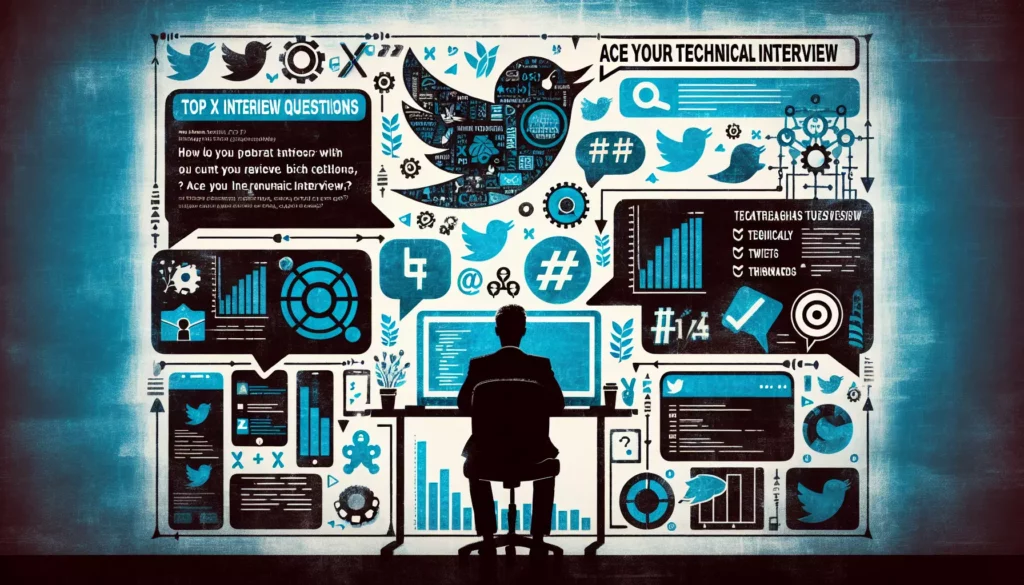
Are you gearing up for a technical interview at Twitter (now known as X)? Congratulations on making it this far in the process! Twitter, being one of the tech giants, is known for its rigorous interview process that tests candidates on various aspects of computer science and software engineering. In this comprehensive guide, we’ll walk you through some of the most common Twitter interview questions, provide insights into what the interviewers are looking for, and offer tips on how to prepare effectively.
Table of Contents
- Introduction to Twitter’s Interview Process
- Coding and Algorithm Questions
- System Design Questions
- Behavioral Questions
- Twitter-Specific Technical Questions
- Tips for Success
- How to Prepare
- Conclusion
1. Introduction to Twitter’s Interview Process
Twitter’s interview process typically consists of several rounds:
- Initial Screening: This is usually a phone call with a recruiter to discuss your background and the role.
- Technical Phone Screen: A 45-60 minute interview with an engineer, focusing on coding and problem-solving.
- On-site Interviews: A series of 4-5 interviews covering coding, system design, and behavioral questions.
- Team-Specific Interviews: Depending on the role, you might have additional interviews with the team you’d be joining.
Throughout this process, Twitter is looking for candidates who demonstrate strong problem-solving skills, a deep understanding of computer science fundamentals, and the ability to write clean, efficient code.
2. Coding and Algorithm Questions
Coding questions form the core of Twitter’s technical interviews. Here are some common types of questions you might encounter:
2.1. Array Manipulation
Question: Given an array of integers, find the contiguous subarray with the largest sum.
Solution: This is the classic Kadane’s algorithm problem. Here’s a Python implementation:
def max_subarray_sum(arr):
max_sum = current_sum = arr[0]
for num in arr[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
# Example usage
arr = [-2, 1, -3, 4, -1, 2, 1, -5, 4]
print(max_subarray_sum(arr)) # Output: 6
2.2. String Manipulation
Question: Implement a function to determine if a string has all unique characters.
Solution: Here’s a Python implementation using a set:
def has_unique_chars(s):
return len(set(s)) == len(s)
# Example usage
print(has_unique_chars("abcdefg")) # Output: True
print(has_unique_chars("abcdefga")) # Output: False
2.3. Tree and Graph Traversal
Question: Implement a function to find the lowest common ancestor of two nodes in a binary tree.
Solution: Here’s a Python implementation:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def lowest_common_ancestor(root, p, q):
if not root or root == p or root == q:
return root
left = lowest_common_ancestor(root.left, p, q)
right = lowest_common_ancestor(root.right, p, q)
if left and right:
return root
return left if left else right
# Example usage
root = TreeNode(3)
root.left = TreeNode(5)
root.right = TreeNode(1)
root.left.left = TreeNode(6)
root.left.right = TreeNode(2)
root.right.left = TreeNode(0)
root.right.right = TreeNode(8)
p = root.left
q = root.right
lca = lowest_common_ancestor(root, p, q)
print(lca.val) # Output: 3
2.4. Dynamic Programming
Question: Given a set of coin denominations and a target amount, find the minimum number of coins needed to make up that amount.
Solution: Here’s a Python implementation using dynamic programming:
def min_coins(coins, amount):
dp = [float('inf')] * (amount + 1)
dp[0] = 0
for i in range(1, amount + 1):
for coin in coins:
if coin <= i:
dp[i] = min(dp[i], dp[i - coin] + 1)
return dp[amount] if dp[amount] != float('inf') else -1
# Example usage
coins = [1, 2, 5]
amount = 11
print(min_coins(coins, amount)) # Output: 3
3. System Design Questions
System design questions are crucial for senior engineering roles at Twitter. These questions test your ability to design large-scale distributed systems. Some common Twitter system design questions include:
3.1. Design Twitter’s Tweet Feed
Question: Design a system that can handle millions of tweets per second and deliver them to users’ feeds in real-time.
Key Considerations:
- How to handle the high write load of incoming tweets
- Efficient storage and retrieval of tweets
- Real-time delivery of tweets to followers
- Scaling the system to handle millions of users
Possible Solution Outline:
- Use a distributed database like Cassandra for tweet storage
- Implement a message queue system (e.g., Kafka) for handling incoming tweets
- Use a caching layer (e.g., Redis) for frequently accessed tweets and user data
- Implement a fan-out service to deliver tweets to followers’ timelines
- Use a load balancer to distribute traffic across multiple servers
3.2. Design Twitter’s Search Functionality
Question: Design a system that allows users to search through billions of tweets efficiently.
Key Considerations:
- Indexing tweets for fast retrieval
- Handling real-time updates to the search index
- Implementing relevance ranking for search results
- Scaling the system to handle high query loads
Possible Solution Outline:
- Use an inverted index for efficient keyword-based search
- Implement a distributed search engine like Elasticsearch
- Use a message queue to handle real-time updates to the search index
- Implement caching for frequently searched terms
- Use machine learning models for relevance ranking
4. Behavioral Questions
Behavioral questions are an essential part of Twitter’s interview process. They help assess your problem-solving skills, teamwork abilities, and cultural fit. Here are some common behavioral questions you might encounter:
- Tell me about a time when you had to deal with a difficult team member.
- Describe a situation where you had to make a decision with incomplete information.
- How do you handle criticism or feedback on your work?
- Can you share an example of a project where you demonstrated leadership?
- How do you stay updated with the latest technologies and industry trends?
When answering behavioral questions, use the STAR method (Situation, Task, Action, Result) to structure your responses and provide concrete examples from your past experiences.
5. Twitter-Specific Technical Questions
Given Twitter’s unique position in the social media landscape, you might encounter questions specific to Twitter’s technology stack and challenges. Here are a few examples:
5.1. Rate Limiting
Question: How would you implement a rate limiter for Twitter’s API?
Solution Outline:
- Use a token bucket algorithm or sliding window counter
- Store rate limit data in a distributed cache like Redis
- Implement the rate limiter as a middleware in the API gateway
- Use a unique identifier (e.g., API key or user ID) to track requests
5.2. Trending Topics
Question: Design a system to identify and display trending topics on Twitter.
Solution Outline:
- Use a streaming processing system like Apache Flink to process incoming tweets
- Implement a sliding window algorithm to count hashtag occurrences
- Use a min-heap to maintain the top N trending topics
- Implement a decay factor to give more weight to recent tweets
- Use a caching layer to serve trending topics to users quickly
6. Tips for Success
To increase your chances of success in a Twitter interview, keep these tips in mind:
- Practice coding on a whiteboard: Many interviews still use whiteboards, so practice writing code without an IDE.
- Think out loud: Explain your thought process as you solve problems. This gives the interviewer insight into how you approach challenges.
- Ask clarifying questions: Don’t hesitate to ask for more information or clarification on the problem statement.
- Consider edge cases: Always think about and discuss potential edge cases in your solutions.
- Know your resume: Be prepared to discuss any project or technology mentioned in your resume in detail.
- Stay calm: If you get stuck, take a deep breath and try to break the problem down into smaller parts.
- Be familiar with Twitter’s products: Have a good understanding of Twitter’s features and recent developments.
7. How to Prepare
Preparing for a Twitter interview requires dedication and a structured approach. Here’s a recommended preparation plan:
- Review Computer Science Fundamentals: Brush up on data structures, algorithms, and system design concepts.
- Practice Coding Problems: Solve problems on platforms like LeetCode, HackerRank, or AlgoCademy. Focus on medium to hard difficulty questions.
- Study System Design: Read books like “Designing Data-Intensive Applications” by Martin Kleppmann and practice designing large-scale systems.
- Mock Interviews: Conduct mock interviews with friends or use platforms that offer mock interview services.
- Learn About Twitter: Research Twitter’s technology stack, recent projects, and company culture.
- Prepare Your Own Questions: Have thoughtful questions ready to ask your interviewers about the role and the company.
8. Conclusion
Preparing for a Twitter interview can be challenging, but with the right approach and dedication, you can significantly increase your chances of success. Remember that the interview process is not just about testing your technical skills but also about assessing your problem-solving abilities, communication skills, and cultural fit.
Focus on strengthening your understanding of core computer science concepts, practice coding regularly, and stay updated with the latest trends in technology, especially those relevant to Twitter’s ecosystem. Don’t forget to also prepare for behavioral questions and have a solid understanding of your past projects and experiences.
Lastly, remember that interviewing is a two-way process. Use this opportunity to also evaluate if Twitter is the right fit for your career goals and aspirations. Good luck with your interview!