Top Tesla Interview Questions: Ace Your Next Tech Interview
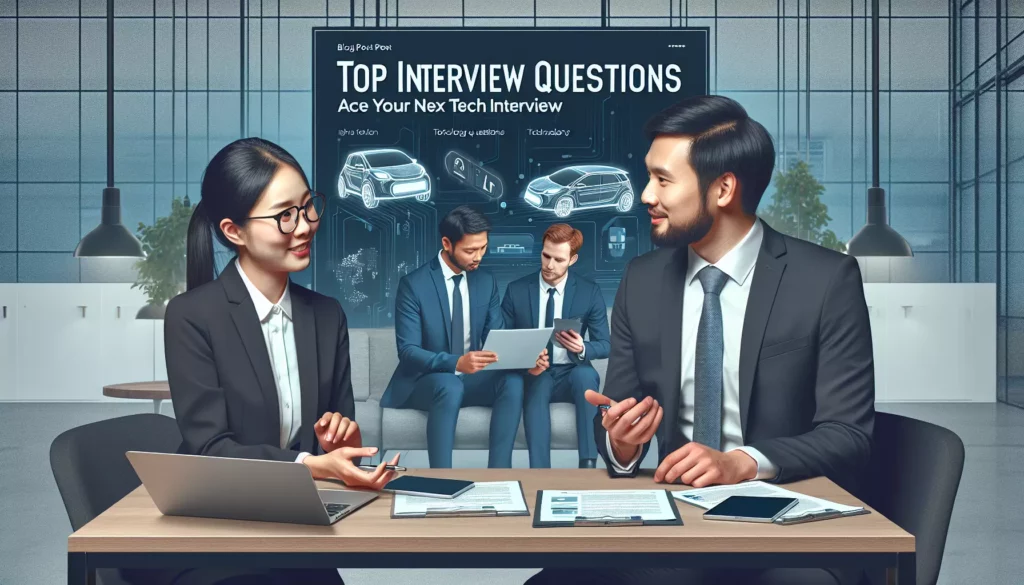
Are you gearing up for a technical interview at Tesla? As one of the most innovative companies in the automotive and energy sectors, Tesla is known for its rigorous interview process. To help you prepare, we’ve compiled a comprehensive list of common Tesla interview questions, along with tips and strategies to tackle them effectively. Whether you’re a software engineer, data scientist, or aspiring to join Tesla’s cutting-edge teams, this guide will give you the edge you need to succeed.
Table of Contents
- Introduction to Tesla’s Interview Process
- Coding and Algorithm Questions
- System Design Questions
- Behavioral and Cultural Fit Questions
- Technical Knowledge Questions
- Problem-Solving and Analytical Questions
- AI and Machine Learning Questions
- Automotive and Energy-Specific Questions
- Tips for Success in Tesla Interviews
- Conclusion
1. Introduction to Tesla’s Interview Process
Before diving into specific questions, it’s essential to understand Tesla’s interview process. Typically, it involves several stages:
- Initial phone screen with a recruiter
- Technical phone interview with an engineer
- Online coding assessment
- On-site interviews (or virtual equivalent)
- Final interview with senior management (for some positions)
The process is designed to assess not only your technical skills but also your problem-solving abilities, cultural fit, and passion for Tesla’s mission. Now, let’s explore the types of questions you might encounter during these interviews.
2. Coding and Algorithm Questions
Tesla places a strong emphasis on coding skills and algorithmic thinking. Here are some common coding questions you might face:
2.1. Reverse a Linked List
Question: Write a function to reverse a singly linked list.
Solution:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverseLinkedList(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
2.2. Implement a Queue using Stacks
Question: Implement a first in first out (FIFO) queue using only two stacks.
Solution:
class MyQueue:
def __init__(self):
self.stack1 = []
self.stack2 = []
def push(self, x: int) -> None:
self.stack1.append(x)
def pop(self) -> int:
self._transfer()
return self.stack2.pop()
def peek(self) -> int:
self._transfer()
return self.stack2[-1]
def empty(self) -> bool:
return len(self.stack1) == 0 and len(self.stack2) == 0
def _transfer(self):
if not self.stack2:
while self.stack1:
self.stack2.append(self.stack1.pop())
2.3. Find the Longest Palindromic Substring
Question: Given a string s, return the longest palindromic substring in s.
Solution:
def longestPalindrome(s: str) -> str:
if not s:
return ""
start = 0
max_length = 1
def expand_around_center(left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return right - left - 1
for i in range(len(s)):
len1 = expand_around_center(i, i)
len2 = expand_around_center(i, i + 1)
length = max(len1, len2)
if length > max_length:
start = i - (length - 1) // 2
max_length = length
return s[start:start + max_length]
These coding questions test your ability to work with fundamental data structures and algorithms. When solving these problems, make sure to discuss your thought process, consider edge cases, and analyze the time and space complexity of your solutions.
3. System Design Questions
For more senior positions, Tesla often includes system design questions to assess your ability to architect large-scale systems. Here are some examples:
3.1. Design Tesla’s Autopilot System
Question: How would you design the core components of Tesla’s Autopilot system?
Key points to consider:
- Sensor fusion (cameras, radar, ultrasonic sensors)
- Real-time data processing and decision making
- Machine learning models for object detection and prediction
- Safety mechanisms and fail-safes
- Over-the-air updates and continuous improvement
3.2. Design a Charging Station Management System
Question: Design a system to manage and optimize Tesla’s Supercharger network.
Key points to consider:
- Real-time availability tracking
- Load balancing and queue management
- Payment processing and user authentication
- Predictive maintenance
- Integration with Tesla vehicles for route planning
3.3. Design a Battery Management System
Question: How would you design a system to monitor and optimize battery performance in Tesla vehicles?
Key points to consider:
- Real-time monitoring of battery cells
- Thermal management
- State of charge and state of health estimation
- Power distribution and optimization
- Degradation prediction and mitigation
When answering system design questions, focus on high-level architecture, scalability, reliability, and how your design addresses Tesla’s specific challenges and goals.
4. Behavioral and Cultural Fit Questions
Tesla values candidates who align with their mission and culture. Be prepared for questions like:
- Why do you want to work at Tesla?
- Describe a time when you had to solve a complex problem under tight deadlines.
- How do you stay updated with the latest technologies in your field?
- Tell me about a time when you had to work with a difficult team member.
- How do you handle criticism or feedback on your work?
When answering these questions, use the STAR method (Situation, Task, Action, Result) and focus on demonstrating your problem-solving skills, adaptability, and passion for innovation.
5. Technical Knowledge Questions
Depending on the specific role you’re applying for, you might encounter questions that test your domain knowledge. Here are some examples:
5.1. Software Engineering
- Explain the difference between processes and threads.
- What are the principles of object-oriented programming?
- How does garbage collection work in languages like Java or Python?
- Describe the HTTP request/response cycle.
- What are the benefits and drawbacks of microservices architecture?
5.2. Data Science
- Explain the difference between supervised and unsupervised learning.
- What is the curse of dimensionality, and how can it be addressed?
- Describe the process of feature selection in machine learning.
- What is the difference between L1 and L2 regularization?
- How would you handle imbalanced datasets in classification problems?
5.3. Embedded Systems
- What is the difference between a microprocessor and a microcontroller?
- Explain the concept of real-time operating systems (RTOS).
- How do you optimize code for embedded systems with limited resources?
- Describe the CAN bus protocol and its applications in automotive systems.
- What are the challenges in developing firmware for safety-critical systems?
When answering technical questions, be concise but thorough. If you’re unsure about something, it’s better to admit it and explain how you would go about finding the answer.
6. Problem-Solving and Analytical Questions
Tesla values candidates who can think critically and solve complex problems. You might encounter questions like:
6.1. Estimate the Number of Electric Vehicles in a City
Question: How would you estimate the number of electric vehicles in a given city?
Approach:
- Start with the city’s population
- Estimate the number of households
- Estimate the percentage of households owning a car
- Research or estimate the market share of electric vehicles
- Calculate the final estimate
6.2. Optimize Supercharger Station Placement
Question: How would you determine the optimal locations for new Supercharger stations?
Factors to consider:
- Traffic patterns and popular routes
- Existing charging infrastructure
- Population density and Tesla ownership data
- Proximity to amenities (restaurants, shopping centers)
- Electrical grid capacity and costs
6.3. Troubleshoot a Production Line Issue
Question: A Tesla production line is experiencing unexpected delays. How would you approach troubleshooting the issue?
Steps to consider:
- Gather data on the nature and frequency of the delays
- Analyze recent changes in the production process
- Check for equipment malfunctions or maintenance issues
- Review supply chain and inventory levels
- Assess worker performance and training
- Implement and test potential solutions
When answering these questions, focus on your problem-solving approach, ability to break down complex issues, and data-driven decision-making skills.
7. AI and Machine Learning Questions
Given Tesla’s focus on autonomous driving and AI-powered systems, you might encounter questions related to artificial intelligence and machine learning:
7.1. Explain the Concept of Transfer Learning
Question: What is transfer learning, and how might it be applied in the context of autonomous driving?
Key points:
- Definition of transfer learning
- Benefits in scenarios with limited labeled data
- Application to object detection and scene understanding
- Challenges and limitations in the autonomous driving context
7.2. Describe Reinforcement Learning for Autonomous Driving
Question: How can reinforcement learning be used to improve autonomous driving capabilities?
Key points:
- Explanation of reinforcement learning concepts (agents, states, actions, rewards)
- Application to decision-making in complex driving scenarios
- Challenges in defining appropriate reward functions
- Safety considerations and the need for simulation
7.3. Discuss Ethics in AI for Autonomous Vehicles
Question: What ethical considerations should be taken into account when developing AI systems for autonomous vehicles?
Topics to address:
- Safety prioritization and decision-making in unavoidable accident scenarios
- Transparency and explainability of AI decision-making processes
- Data privacy and security concerns
- Potential biases in training data and their implications
- Regulatory compliance and liability issues
When discussing AI and ML topics, demonstrate your understanding of both the technical aspects and the broader implications of these technologies in the automotive industry.
8. Automotive and Energy-Specific Questions
Depending on the role, you might be asked questions specific to the automotive or energy sectors:
8.1. Explain Regenerative Braking
Question: How does regenerative braking work in electric vehicles, and what are its benefits?
Key points:
- Conversion of kinetic energy to electrical energy
- Role of the electric motor as a generator
- Energy efficiency improvements
- Impact on brake wear and maintenance
8.2. Discuss Battery Technology Trends
Question: What are the current trends in electric vehicle battery technology, and how might they impact the future of EVs?
Topics to cover:
- Improvements in energy density and charging speeds
- Solid-state batteries and their potential advantages
- Sustainability and recycling considerations
- Cost reduction strategies
8.3. Explain Vehicle-to-Grid (V2G) Technology
Question: What is vehicle-to-grid technology, and how could it benefit both EV owners and the power grid?
Key points:
- Bidirectional power flow between EVs and the grid
- Load balancing and grid stability benefits
- Potential for EV owners to earn money by selling power back to the grid
- Challenges in implementation and adoption
When answering these questions, demonstrate your understanding of the technologies involved and their broader implications for the automotive and energy industries.
9. Tips for Success in Tesla Interviews
To increase your chances of success in Tesla interviews, keep these tips in mind:
- Do your research: Familiarize yourself with Tesla’s products, technologies, and recent news.
- Practice coding: Use platforms like AlgoCademy to sharpen your coding skills and practice common interview questions.
- Think out loud: During problem-solving questions, explain your thought process clearly.
- Ask questions: Show your interest by asking thoughtful questions about the role and the company.
- Demonstrate passion: Convey your enthusiasm for Tesla’s mission and innovative technologies.
- Be prepared for technical depth: Tesla interviewers often dive deep into technical topics, so be ready to discuss your expertise in detail.
- Highlight relevant projects: Prepare examples of projects or experiences that demonstrate your skills and problem-solving abilities.
- Stay up-to-date: Keep abreast of the latest developments in automotive technology, renewable energy, and AI/ML.
- Practice system design: For senior roles, practice designing large-scale systems and articulating your design decisions.
- Be adaptable: Tesla values candidates who can adapt to rapidly changing environments and technologies.
10. Conclusion
Preparing for a Tesla interview can be challenging, but with the right approach and mindset, you can significantly increase your chances of success. By familiarizing yourself with the types of questions asked, practicing your coding and problem-solving skills, and demonstrating your passion for Tesla’s mission, you’ll be well-equipped to tackle the interview process.
Remember that Tesla is looking for candidates who not only have strong technical skills but also align with their innovative culture and mission to accelerate the world’s transition to sustainable energy. Show them how you can contribute to this vision, and you’ll be one step closer to joining one of the most exciting companies in the tech and automotive industries.
Good luck with your Tesla interview! And don’t forget to leverage resources like AlgoCademy to continue honing your coding and problem-solving skills as you prepare for this exciting opportunity.