Top Techniques for Debugging and Error Handling: A Comprehensive Guide
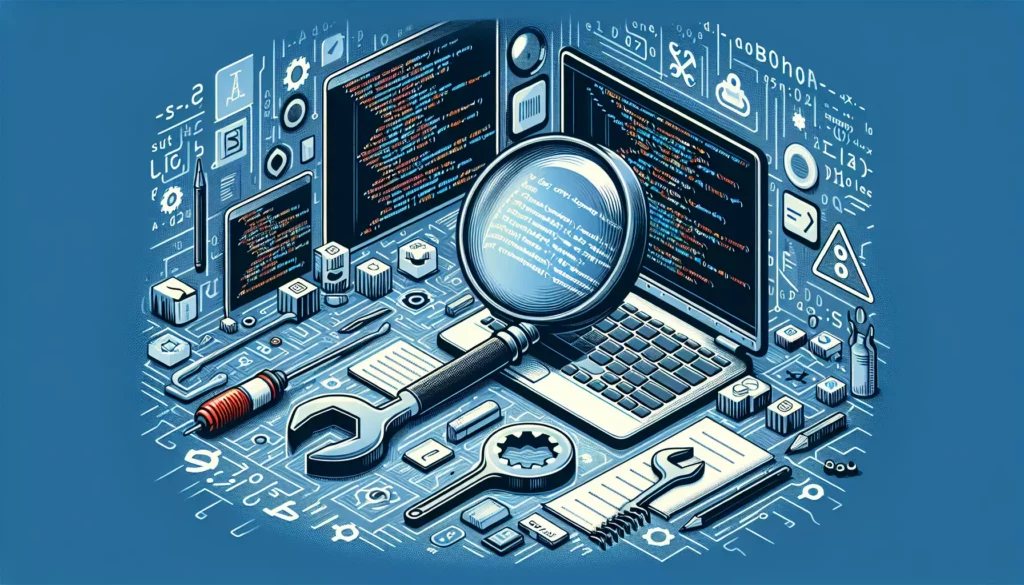
As a programmer, encountering errors and bugs is an inevitable part of the development process. The ability to effectively debug code and handle errors is a crucial skill that separates novice developers from seasoned professionals. In this comprehensive guide, we’ll explore the top techniques for debugging and error handling, equipping you with the tools and knowledge to tackle even the most challenging coding issues.
Table of Contents
- Understanding Debugging
- Common Debugging Techniques
- Advanced Debugging Strategies
- Error Handling Best Practices
- Debugging Tools and IDEs
- Language-Specific Debugging Tips
- Debugging in Production Environments
- Preventing Bugs and Errors
- Conclusion
1. Understanding Debugging
Debugging is the process of identifying, isolating, and fixing errors or bugs in software code. It’s a critical skill for any programmer, as it helps ensure the reliability and functionality of applications. Before diving into specific techniques, it’s essential to understand the different types of errors you might encounter:
- Syntax Errors: These occur when the code violates the rules of the programming language.
- Runtime Errors: These happen during program execution and can cause the program to crash.
- Logical Errors: These are the trickiest to spot, as the program runs without crashing but produces incorrect results.
Effective debugging requires a systematic approach and a deep understanding of how your code works. Let’s explore some common techniques that can help you tackle these errors efficiently.
2. Common Debugging Techniques
2.1 Print Statements
One of the simplest yet effective debugging techniques is using print statements to output variable values and program flow. This method allows you to track the execution of your code and identify where things might be going wrong.
def calculate_sum(a, b):
print(f"Calculating sum of {a} and {b}")
result = a + b
print(f"Result: {result}")
return result
total = calculate_sum(5, 3)
print(f"Total: {total}")
While this technique is straightforward, it can become cumbersome for larger codebases and should be used judiciously.
2.2 Rubber Duck Debugging
This technique involves explaining your code line-by-line to an inanimate object (like a rubber duck) or a fellow programmer. The process of articulating your code often helps you spot errors or logical flaws that you might have overlooked.
2.3 Divide and Conquer
When dealing with complex issues, breaking down your code into smaller, manageable pieces can help isolate the problem. This method involves systematically testing different parts of your code to pinpoint where the error occurs.
2.4 Using Debuggers
Most modern Integrated Development Environments (IDEs) come with built-in debuggers. These tools allow you to set breakpoints, step through code line-by-line, and inspect variable values in real-time. Learning to use a debugger effectively can significantly speed up your debugging process.
3. Advanced Debugging Strategies
3.1 Log Analysis
For larger applications, especially those running in production environments, log analysis is crucial. Implementing comprehensive logging throughout your application can help you trace errors and understand the state of your program at different points in time.
import logging
logging.basicConfig(level=logging.DEBUG)
logger = logging.getLogger(__name__)
def complex_operation(data):
logger.info(f"Starting complex operation with data: {data}")
try:
result = process_data(data)
logger.debug(f"Data processed successfully: {result}")
return result
except Exception as e:
logger.error(f"Error occurred during data processing: {str(e)}")
raise
3.2 Unit Testing
While not strictly a debugging technique, implementing comprehensive unit tests can help catch bugs early and make it easier to isolate issues when they do occur. Writing tests for individual components of your code can help ensure that each part works as expected.
import unittest
def add_numbers(a, b):
return a + b
class TestAddNumbers(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add_numbers(2, 3), 5)
def test_add_negative_numbers(self):
self.assertEqual(add_numbers(-1, -1), -2)
if __name__ == '__main__':
unittest.main()
3.3 Code Profiling
Profiling your code can help identify performance bottlenecks and potential areas for optimization. Many programming languages have built-in profiling tools or third-party libraries that can provide detailed insights into your code’s execution time and resource usage.
3.4 Remote Debugging
For applications running on remote servers or in cloud environments, remote debugging techniques allow you to connect to the running process and debug it in real-time. This can be particularly useful for issues that only occur in specific environments.
4. Error Handling Best Practices
Proper error handling is crucial for creating robust and maintainable code. Here are some best practices to consider:
4.1 Use Try-Except Blocks
Wrap potentially error-prone code in try-except blocks to catch and handle exceptions gracefully.
try:
result = complex_calculation()
except ValueError as e:
print(f"Error: Invalid value - {str(e)}")
except ZeroDivisionError:
print("Error: Division by zero")
except Exception as e:
print(f"An unexpected error occurred: {str(e)}")
else:
print(f"Calculation successful. Result: {result}")
finally:
print("Calculation attempt completed.")
4.2 Raise Custom Exceptions
Create custom exception classes for specific error scenarios in your application. This can make error handling more precise and informative.
class InvalidInputError(Exception):
pass
def process_user_input(input_string):
if not input_string.isalpha():
raise InvalidInputError("Input must contain only alphabetic characters")
# Process the input
return input_string.upper()
4.3 Provide Meaningful Error Messages
Ensure that your error messages are clear, concise, and provide useful information for debugging or user feedback.
4.4 Log Errors
Implement a logging system to record errors and exceptions. This is particularly important for applications running in production environments where direct access to the console may not be available.
5. Debugging Tools and IDEs
Familiarizing yourself with powerful debugging tools can significantly enhance your ability to find and fix bugs quickly. Here are some popular options:
5.1 Integrated Development Environments (IDEs)
- PyCharm: A powerful IDE for Python development with advanced debugging features.
- Visual Studio Code: A versatile, lightweight code editor with excellent debugging capabilities for multiple languages.
- IntelliJ IDEA: A robust IDE for Java development with comprehensive debugging tools.
5.2 Standalone Debugging Tools
- GDB (GNU Debugger): A powerful command-line debugger for C, C++, and other languages.
- Chrome DevTools: Built-in developer tools in the Chrome browser for debugging JavaScript, HTML, and CSS.
- Valgrind: A tool for memory debugging, memory leak detection, and profiling.
5.3 Browser Developer Tools
For web development, browser developer tools are invaluable for debugging JavaScript, inspecting DOM elements, and analyzing network requests.
6. Language-Specific Debugging Tips
Different programming languages often have unique debugging challenges and tools. Here are some language-specific tips:
6.1 Python
- Use the built-in
pdb
module for interactive debugging. - Leverage the
logging
module for flexible logging capabilities. - Utilize the
traceback
module to get detailed stack traces.
import pdb
def complex_function(x, y):
result = x / y
pdb.set_trace() # This will start the debugger
return result * 2
complex_function(10, 0)
6.2 JavaScript
- Use
console.log()
,console.warn()
, andconsole.error()
for different levels of logging. - Leverage the
debugger
statement to create breakpoints in your code. - Utilize browser developer tools for step-by-step debugging and variable inspection.
function complexCalculation(a, b) {
console.log(`Starting calculation with ${a} and ${b}`);
let result = a * b;
debugger; // This will pause execution in browser dev tools
return result / 2;
}
complexCalculation(5, 10);
6.3 Java
- Use the
assert
keyword for debugging and testing. - Leverage Java’s built-in logging framework (
java.util.logging
) or popular alternatives like Log4j. - Utilize IDEs like IntelliJ IDEA or Eclipse for powerful Java-specific debugging features.
public class DebugExample {
public static void main(String[] args) {
int x = 5;
int y = 0;
assert y != 0 : "Division by zero!";
int result = x / y;
System.out.println(result);
}
}
7. Debugging in Production Environments
Debugging issues in production environments presents unique challenges. Here are some strategies to consider:
7.1 Implement Robust Logging
Ensure your application logs contain sufficient information to diagnose issues without compromising security or performance.
7.2 Use Error Monitoring Tools
Implement error monitoring services like Sentry, Rollbar, or New Relic to automatically capture and report errors in real-time.
7.3 Feature Flags
Use feature flags to selectively enable or disable features in production. This can help isolate issues and roll back problematic changes quickly.
7.4 Reproduce in Staging
Whenever possible, try to reproduce production issues in a staging environment where you have more debugging capabilities.
8. Preventing Bugs and Errors
While debugging skills are crucial, preventing bugs in the first place is even more valuable. Here are some strategies to minimize the occurrence of bugs:
8.1 Code Reviews
Implement a thorough code review process. Having other developers examine your code can help catch potential issues before they make it to production.
8.2 Static Code Analysis
Use static code analysis tools to automatically detect potential bugs, code smells, and style violations.
8.3 Continuous Integration and Testing
Implement a CI/CD pipeline that includes automated testing. This helps catch bugs early in the development process.
8.4 Defensive Programming
Anticipate potential issues and handle them gracefully in your code. This includes input validation, error checking, and proper exception handling.
def divide_numbers(a, b):
if not isinstance(a, (int, float)) or not isinstance(b, (int, float)):
raise TypeError("Both arguments must be numbers")
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
try:
result = divide_numbers(10, "2")
except TypeError as e:
print(f"Error: {str(e)}")
except ValueError as e:
print(f"Error: {str(e)}")
except Exception as e:
print(f"An unexpected error occurred: {str(e)}")
else:
print(f"Result: {result}")
9. Conclusion
Debugging and error handling are essential skills for any programmer. By mastering these techniques, you’ll be better equipped to tackle complex coding challenges and create more robust, reliable software. Remember that debugging is often an iterative process that requires patience and persistence.
As you continue to develop your programming skills, make debugging and error handling an integral part of your coding practice. Regularly review and refine your approach, stay updated with the latest tools and techniques, and always strive to write code that is not only functional but also easy to debug and maintain.
By incorporating these top techniques for debugging and error handling into your development workflow, you’ll be well on your way to becoming a more effective and efficient programmer. Happy coding, and may your bugs be few and far between!