Top Swift Interview Questions and Answers for 2023
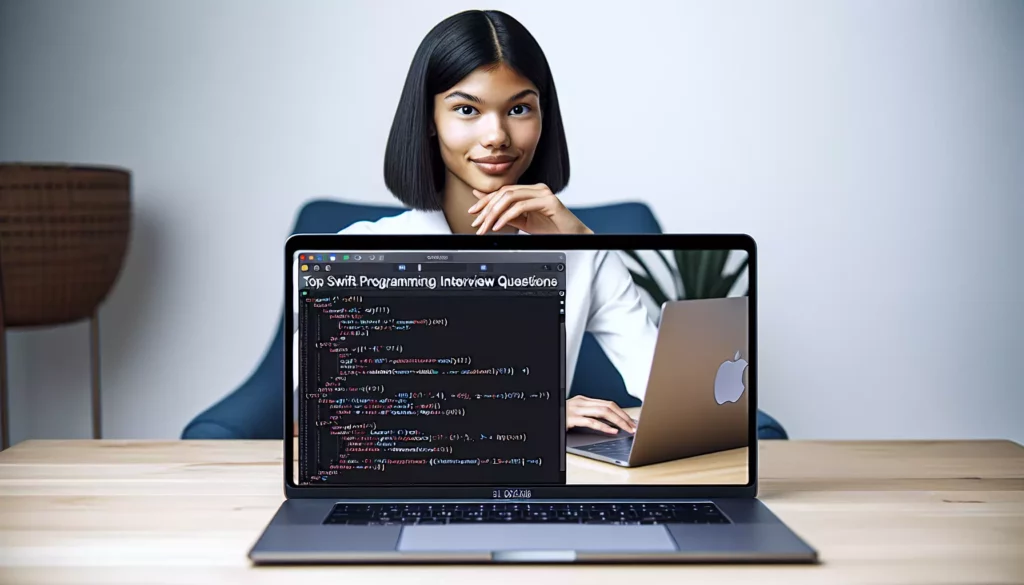
Are you preparing for a Swift developer interview? Whether you’re a seasoned professional or just starting your journey in iOS development, it’s crucial to be well-prepared for the questions that might come your way. In this comprehensive guide, we’ll explore some of the most common Swift interview questions and provide detailed answers to help you ace your next interview.
Table of Contents
- Swift Basics
- Object-Oriented Programming in Swift
- Functional Programming in Swift
- Memory Management
- Concurrency and Multithreading
- Design Patterns
- iOS-Specific Questions
- Advanced Swift Concepts
- Coding Challenges
- Best Practices and Tips
1. Swift Basics
Q1: What are the key features of Swift?
Swift is a modern programming language developed by Apple for iOS, macOS, watchOS, and tvOS development. Some key features include:
- Type safety and inference
- Optionals for handling nil values
- Protocol-oriented programming
- Functional programming support
- Generics
- Automatic Reference Counting (ARC) for memory management
- Interoperability with Objective-C
- Playground support for rapid prototyping
Q2: Explain the difference between let and var in Swift.
let
is used to declare constants, while var
is used to declare variables. The main differences are:
let
creates immutable values that cannot be changed after initializationvar
creates mutable values that can be modified after initialization
Example:
let constantValue = 10
var variableValue = 20
// This is allowed
variableValue = 30
// This will result in a compile-time error
// constantValue = 40
Q3: What are optionals in Swift and why are they useful?
Optionals are a feature in Swift that allow variables to have either a value or no value (nil). They are useful for handling situations where a value may be absent, helping to prevent runtime errors caused by unexpected nil values.
There are two ways to declare optionals:
var optionalString: String?
var implicitlyUnwrappedOptional: String!
Optionals are particularly useful for:
- Representing the absence of a value
- Handling potential errors or edge cases
- Improving code safety by forcing developers to handle nil cases explicitly
Q4: How do you unwrap an optional in Swift?
There are several ways to unwrap optionals in Swift:
- Force unwrapping (not recommended unless you’re certain the value exists):
let forcedValue = optionalValue!
- Optional binding:
if let unwrappedValue = optionalValue { print(unwrappedValue) }
- Guard statement:
guard let unwrappedValue = optionalValue else { return } print(unwrappedValue)
- Nil-coalescing operator:
let value = optionalValue ?? defaultValue
- Optional chaining:
let result = optionalValue?.property?.method()
2. Object-Oriented Programming in Swift
Q5: Explain the difference between a struct and a class in Swift.
Structs and classes are both used to define custom types in Swift, but they have some key differences:
- Structs are value types, while classes are reference types
- Structs have an automatically generated memberwise initializer, classes don’t
- Classes support inheritance, structs don’t
- Classes have deinitializers, structs don’t
- Multiple variables can reference the same instance of a class, but each struct variable has its own copy of the data
Example:
struct Point {
var x: Int
var y: Int
}
class Person {
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
}
Q6: What is inheritance in Swift and how is it implemented?
Inheritance is a fundamental concept in object-oriented programming that allows a class to inherit properties and methods from another class. In Swift, inheritance is implemented using the colon (:) syntax.
Example:
class Vehicle {
var numberOfWheels: Int
init(numberOfWheels: Int) {
self.numberOfWheels = numberOfWheels
}
func description() -> String {
return "This vehicle has \(numberOfWheels) wheels."
}
}
class Car: Vehicle {
var brand: String
init(brand: String) {
self.brand = brand
super.init(numberOfWheels: 4)
}
override func description() -> String {
return "This \(brand) car has \(numberOfWheels) wheels."
}
}
Q7: What are protocols in Swift and how are they used?
Protocols in Swift define a blueprint of methods, properties, and other requirements that suit a particular task or piece of functionality. They can be adopted by classes, structs, or enums to provide a common interface.
Example:
protocol Drawable {
func draw()
}
class Circle: Drawable {
func draw() {
print("Drawing a circle")
}
}
class Square: Drawable {
func draw() {
print("Drawing a square")
}
}
let shapes: [Drawable] = [Circle(), Square()]
for shape in shapes {
shape.draw()
}
3. Functional Programming in Swift
Q8: What are closures in Swift and how are they used?
Closures are self-contained blocks of functionality that can be passed around and used in your code. They are similar to lambda functions in other programming languages.
Example:
let numbers = [1, 2, 3, 4, 5]
let squaredNumbers = numbers.map { $0 * $0 }
print(squaredNumbers) // [1, 4, 9, 16, 25]
let evenNumbers = numbers.filter { $0 % 2 == 0 }
print(evenNumbers) // [2, 4]
let sum = numbers.reduce(0) { $0 + $1 }
print(sum) // 15
Q9: Explain the concept of higher-order functions in Swift.
Higher-order functions are functions that take other functions as parameters or return functions as results. Some common higher-order functions in Swift include map
, filter
, and reduce
.
Example of a custom higher-order function:
func performOperation(_ a: Int, _ b: Int, operation: (Int, Int) -> Int) -> Int {
return operation(a, b)
}
let result = performOperation(5, 3) { $0 + $1 }
print(result) // 8
4. Memory Management
Q10: How does Automatic Reference Counting (ARC) work in Swift?
Automatic Reference Counting (ARC) is Swift’s memory management system. It works by keeping track of strong references to instances of classes. When the number of strong references to an instance drops to zero, ARC automatically deallocates the instance.
Key points about ARC:
- It only applies to instances of classes (reference types)
- It doesn’t apply to structs or enums (value types)
- It can cause retain cycles if not managed properly
Q11: What is a retain cycle and how can it be prevented?
A retain cycle occurs when two instances hold strong references to each other, creating a cycle that prevents ARC from deallocating them. This can lead to memory leaks.
To prevent retain cycles, you can use weak or unowned references:
class Person {
let name: String
weak var apartment: Apartment?
init(name: String) {
self.name = name
}
deinit {
print("\(name) is being deinitialized")
}
}
class Apartment {
let unit: String
weak var tenant: Person?
init(unit: String) {
self.unit = unit
}
deinit {
print("Apartment \(unit) is being deinitialized")
}
}
In this example, using weak
references prevents a retain cycle between Person
and Apartment
.
5. Concurrency and Multithreading
Q12: What is Grand Central Dispatch (GCD) and how is it used in Swift?
Grand Central Dispatch (GCD) is a low-level API for managing concurrent operations. It allows you to execute code concurrently on multicore hardware by submitting work to dispatch queues managed by the system.
Example of using GCD:
DispatchQueue.global(qos: .background).async {
// Perform time-consuming task
DispatchQueue.main.async {
// Update UI on the main thread
}
}
Q13: Explain the difference between synchronous and asynchronous execution.
Synchronous execution means that the code is executed sequentially, and each operation waits for the previous one to complete before starting. Asynchronous execution allows multiple operations to be executed concurrently without waiting for each other to complete.
Example:
// Synchronous
let result = performExpensiveCalculation()
print(result)
// Asynchronous
DispatchQueue.global().async {
let result = performExpensiveCalculation()
DispatchQueue.main.async {
print(result)
}
}
6. Design Patterns
Q14: What is the Singleton pattern and how is it implemented in Swift?
The Singleton pattern ensures that a class has only one instance and provides a global point of access to that instance. In Swift, it can be implemented using a static property:
class Singleton {
static let shared = Singleton()
private init() {}
func someMethod() {
print("Singleton method called")
}
}
// Usage
Singleton.shared.someMethod()
Q15: Explain the Model-View-Controller (MVC) pattern in iOS development.
Model-View-Controller (MVC) is a design pattern commonly used in iOS development:
- Model: Represents the data and business logic
- View: Displays the user interface and sends user actions to the Controller
- Controller: Acts as an intermediary between the Model and View, handling user input and updating the Model and View accordingly
Example structure:
// Model
struct User {
let name: String
let email: String
}
// View
class UserView: UIView {
@IBOutlet weak var nameLabel: UILabel!
@IBOutlet weak var emailLabel: UILabel!
}
// Controller
class UserViewController: UIViewController {
var user: User?
@IBOutlet weak var userView: UserView!
override func viewDidLoad() {
super.viewDidLoad()
updateView()
}
func updateView() {
guard let user = user else { return }
userView.nameLabel.text = user.name
userView.emailLabel.text = user.email
}
}
7. iOS-Specific Questions
Q16: What is the App lifecycle in iOS?
The App lifecycle in iOS refers to the different states an app can be in and the transitions between these states. The main states are:
- Not running
- Inactive
- Active
- Background
- Suspended
These states are managed by the system, and you can respond to state changes in your app delegate methods.
Q17: Explain the difference between frame and bounds in UIView.
frame
and bounds
are both properties of UIView that describe its size and position, but they differ in their frame of reference:
frame
: Describes the view’s location and size in its superview’s coordinate systembounds
: Describes the view’s internal dimensions in its own coordinate system (usually starting at (0, 0))
Example:
let view = UIView(frame: CGRect(x: 50, y: 50, width: 100, height: 100))
print(view.frame) // {{50, 50}, {100, 100}}
print(view.bounds) // {{0, 0}, {100, 100}}
8. Advanced Swift Concepts
Q18: What are generics in Swift and how are they used?
Generics allow you to write flexible, reusable functions and types that can work with any type, subject to requirements that you define. They help you avoid duplication and express your intent in a clear, abstracted manner.
Example of a generic function:
func swapValues<T>(_ a: inout T, _ b: inout T) {
let temp = a
a = b
b = temp
}
var x = 5
var y = 10
swapValues(&x, &y)
print(x, y) // 10 5
var str1 = "hello"
var str2 = "world"
swapValues(&str1, &str2)
print(str1, str2) // world hello
Q19: Explain the concept of associated types in protocols.
Associated types are a powerful feature in Swift that allow you to write protocols that depend on types that are not known until the protocol is adopted. They are specified using the associatedtype
keyword.
Example:
protocol Container {
associatedtype Item
mutating func add(_ item: Item)
var count: Int { get }
}
struct Stack<Element>: Container {
var items = [Element]()
mutating func add(_ item: Element) {
items.append(item)
}
var count: Int {
return items.count
}
}
9. Coding Challenges
Q20: Write a function to reverse a string in Swift.
Here’s a simple implementation to reverse a string:
func reverseString(_ str: String) -> String {
return String(str.reversed())
}
// Test
let original = "Hello, World!"
let reversed = reverseString(original)
print(reversed) // !dlroW ,olleH
Q21: Implement a binary search algorithm in Swift.
Here’s an implementation of binary search for a sorted array:
func binarySearch<T: Comparable>(_ array: [T], _ target: T) -> Int? {
var low = 0
var high = array.count - 1
while low <= high {
let mid = (low + high) / 2
if array[mid] == target {
return mid
} else if array[mid] < target {
low = mid + 1
} else {
high = mid - 1
}
}
return nil
}
// Test
let numbers = [1, 3, 5, 7, 9, 11, 13, 15]
if let index = binarySearch(numbers, 7) {
print("Found at index: \(index)")
} else {
print("Not found")
}
10. Best Practices and Tips
Q22: What are some best practices for writing clean and maintainable Swift code?
Here are some best practices for writing clean and maintainable Swift code:
- Follow the official Swift style guide
- Use meaningful and descriptive names for variables, functions, and types
- Keep functions small and focused on a single task
- Use comments to explain complex logic or non-obvious code
- Leverage Swift’s type inference when appropriate
- Use access control to hide implementation details
- Prefer value types (structs) over reference types (classes) when possible
- Use protocol-oriented programming to create flexible and reusable code
- Write unit tests for your code
- Use version control (e.g., Git) to track changes and collaborate with others
Q23: How can you optimize Swift code for better performance?
Here are some tips for optimizing Swift code:
- Use value types (structs and enums) instead of classes when possible
- Avoid unnecessary force unwrapping of optionals
- Use lazy properties for expensive computations
- Leverage Swift’s built-in collection operations (map, filter, reduce) instead of writing custom loops
- Use appropriate data structures for your use case (e.g., Set for fast lookup)
- Minimize memory allocations by reusing objects when possible
- Use the Instruments tool to profile your app and identify performance bottlenecks
- Enable compiler optimizations in release builds
- Use dispatch queues for concurrent operations
- Cache expensive results when appropriate
Remember, premature optimization can lead to more complex and harder-to-maintain code. Always measure performance before and after optimization to ensure your changes are actually improving performance.
Conclusion
Mastering these Swift interview questions and concepts will greatly improve your chances of success in your next iOS developer interview. Remember that understanding the underlying principles is more important than memorizing specific answers. Practice coding regularly, work on personal projects, and stay up-to-date with the latest developments in Swift and iOS development to continue growing as a developer.
Good luck with your interview preparation!