Top Strategies for Effective Coding Interview Preparation
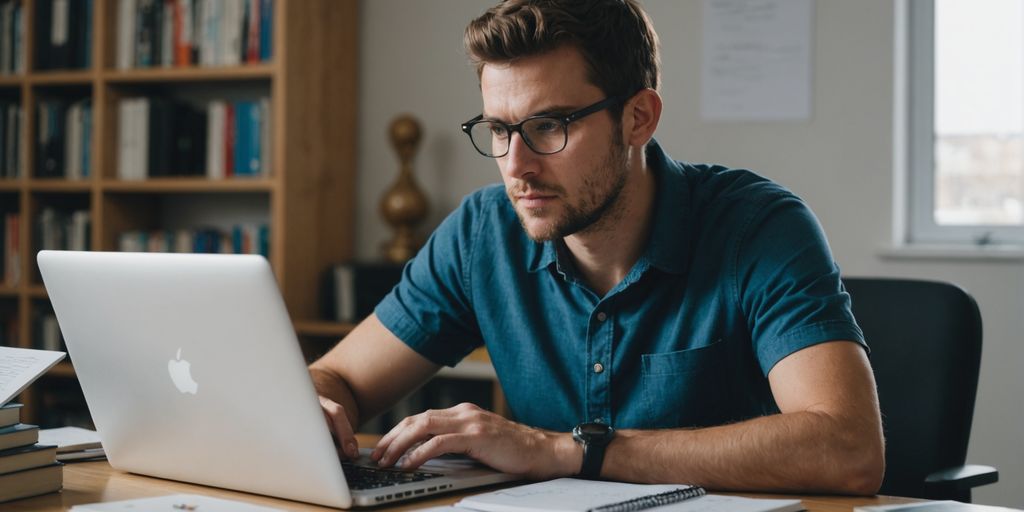
Preparing for a coding interview can be a daunting task, but with the right strategies, you can set yourself up for success. Whether you’re a seasoned programmer or just getting started, these tips will help you navigate the interview process with confidence. Let’s dive into the top strategies for effective coding interview preparation.
Key Takeaways
- Practice mock interviews with friends to improve both technical and communication skills.
- Focus on understanding data structures and algorithms, as they are fundamental to solving coding problems.
- Use pseudocode to plan your solutions before diving into actual coding, which helps in organizing thoughts.
- Engage with community forums like GitHub and Stack Overflow to gain insights and tips from other developers.
- Participate in coding challenges and hackathons to sharpen your skills and gain real-world coding experience.
1. Mock Interviews With Friends
Mock interviews with friends are a great way to prepare for the real thing. Don’t let your first interview be the real one. Practicing with friends can help you get comfortable with the interview format and reduce anxiety.
Here are some tips for effective mock interviews:
- Partner Up: Find a friend or peer who is also preparing for coding interviews. Take turns being the interviewer and the interviewee.
- Use Real Questions: Use actual coding interview questions to make the practice sessions as realistic as possible.
- Give Feedback: After each session, provide constructive feedback to each other. Focus on areas like problem-solving, communication, and time management.
- Track Progress: Write down the feedback you receive and use it to create action items for improvement.
The more mistakes you make and address in mock interviews, the fewer mistakes you’ll make when it counts.
If you can’t find a friend to practice with, consider using platforms like interviewing.io, which connects you with industry engineers for mock interviews. This can be a valuable resource for getting unbiased and helpful feedback.
2. Data Structures and Algorithms
Understanding data structures and algorithms is crucial for coding interviews. They form the backbone of most technical questions. Here’s a breakdown of what you need to know:
- Arrays: These are list-like structures that store elements at specific indexes. They are fundamental and often appear in tricky interview questions.
- Strings: A sequence of characters, usually implemented as an array of bytes. Strings are common in many coding problems.
- Linked Lists: Unlike arrays, linked lists store elements in nodes that point to the next node. This allows for dynamic resizing.
- Stacks and Queues: These are linear data structures used for storing data temporarily. Stacks follow Last In First Out (LIFO) and queues follow First In First Out (FIFO).
- Heaps: A tree-based structure that acts as a priority queue. Elements are dequeued based on priority rather than order of insertion.
Algorithms are equally important. Here are some key ones:
- Depth-First Search (DFS): Used for traversing trees and graphs by exploring as far as possible along each branch before backtracking.
- Breadth-First Search (BFS): Another traversal method for trees and graphs, visiting all nodes at the present depth level before moving on to nodes at the next depth level.
- Binary Search: A fast search algorithm that works on sorted arrays by repeatedly dividing the search interval in half.
Mastering these data structures and algorithms will give you a solid foundation for tackling any coding interview question.
For more practice, consider looking at the top 100 DSA interview questions, which are often segregated based on the data structure or algorithm used to solve them.
3. Use Pseudocode
Using pseudocode is a powerful strategy for coding interview preparation. Pseudocode is an informal way of writing a program for better human understanding. It helps you outline your plan and show your work to the interviewer.
- Clarify Your Thoughts: Writing pseudocode allows you to clarify your thoughts before diving into actual coding. This can help you avoid mistakes and ensure you understand the problem fully.
- Simplify Complex Problems: Breaking down complex problems into smaller, manageable steps using pseudocode can make them easier to solve.
- Communicate Effectively: Pseudocode helps you communicate your approach clearly to the interviewer, showcasing your problem-solving skills.
Pseudocode in C is an informal way of writing a program for better human understanding. This tutorial will help you learn more about pseudocode in C.
By practicing pseudocode, you can improve your ability to think through problems and develop efficient solutions.
4. Practice Coding by Hand
Practicing coding by hand is a crucial step in preparing for cracking any coding interview. It helps you understand the logic and structure of your code without relying on an IDE’s auto-complete and error-checking features. This method sharpens your problem-solving skills and ensures you truly grasp the concepts.
When you code by hand, you need to be meticulous and precise. This practice can be done on paper or a whiteboard, mimicking the environment of many technical interviews. Here are some benefits and tips for coding by hand:
- Improves Syntax Memory: Writing code by hand helps you remember the syntax better since you can’t rely on auto-complete.
- Enhances Problem-Solving Skills: It forces you to think through each step of the problem, improving your logical thinking.
- Prepares for Whiteboard Interviews: Many companies still use whiteboard interviews, so this practice is directly applicable.
- Reduces Dependency on Tools: You become less dependent on coding tools and more confident in your coding abilities.
Practicing coding by hand is a great way to prepare for the real challenges of a coding interview. It builds confidence and ensures you are ready to tackle problems without any digital aids.
To get started, pick a problem and try to solve it on paper. Once you have a solution, type it into your computer to check for errors. This process will help you identify any mistakes and understand where you need to improve.
5. Engage with Community Forums
Engaging with community forums like GitHub and Stack Overflow is a goldmine for coding interview preparation. These platforms are more than just Q&A sites; they are vibrant communities where real-world problems, solutions, and discussions converge. Here, you can deep-dive into threads discussing the latest in tech trends, common interview pitfalls, and even specific interview questions that others have faced.
Benefits of Community Forums
- Collective Wisdom: Tap into the knowledge of experienced developers who share their insights and solutions.
- Real-World Problems: Learn from real-world coding issues and how they were resolved.
- Interview Questions: Find threads that discuss specific interview questions and strategies to tackle them.
How to Get Started
- Create an Account: Sign up on platforms like GitHub and Stack Overflow.
- Join Discussions: Participate in ongoing discussions or start your own thread.
- Ask Questions: Don’t hesitate to ask for help or clarification on topics you find challenging.
- Share Your Knowledge: Contribute by answering questions and sharing your own experiences.
Engaging with community forums not only helps you prepare for coding interviews but also allows you to become a part of a supportive and knowledgeable community.
By actively participating in these forums, you can stay updated on the latest trends and best practices in coding, making your preparation more effective.
6. Participate in Coding Challenges and Hackathons
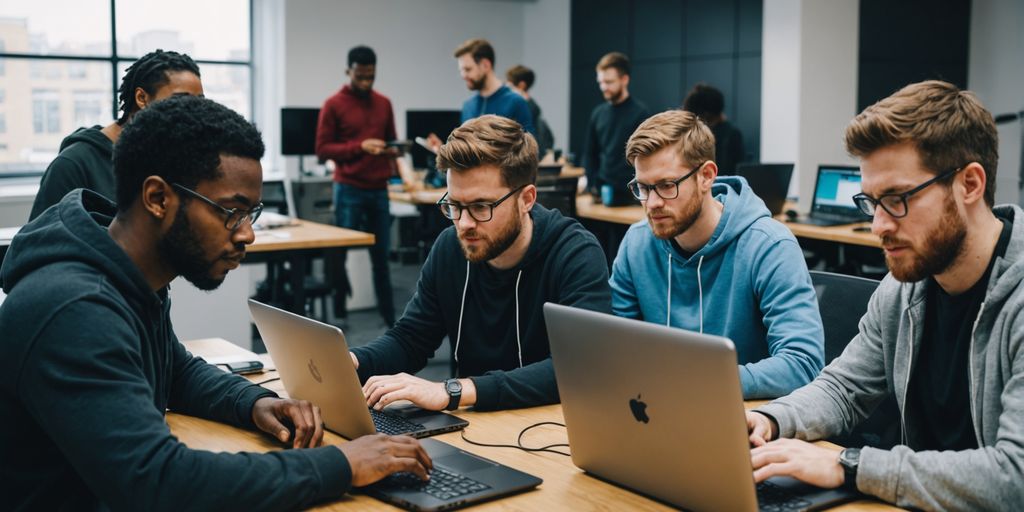
Engaging in coding challenges and hackathons, whether online or in-person, can significantly bolster your coding skills and your professional network. These events are not only a great way to practice coding under time constraints but also an opportunity to meet like-minded individuals, showcase your skills to potential employers, and even win prizes.
Benefits of Coding Challenges and Hackathons
- Skill Improvement: Coding challenges push you to solve problems quickly and efficiently, enhancing your problem-solving abilities.
- Networking: These events are excellent for meeting other coders and potential employers.
- Real-World Experience: Hackathons often simulate real-world projects, giving you a taste of what it’s like to work on a team under deadlines.
- Recognition: Winning or even participating in these events can add a significant boost to your resume.
Top Websites for Coding Challenges
Here are some popular websites where you can participate in coding challenges:
Website | Description |
---|---|
GeeksforGeeks | A comprehensive resource for coding challenges. |
TopCoder | Known for its competitive programming contests. |
HackerRank | Offers a variety of coding challenges and tracks. |
Participating in coding challenges and hackathons can be a game-changer in your coding interview preparation. It not only hones your skills but also provides invaluable networking opportunities.
Tips for Success
- Start Small: Begin with easier challenges and gradually move to more difficult ones.
- Be Consistent: Regular practice is key to improvement.
- Collaborate: Work with others to gain different perspectives and solutions.
- Review and Reflect: After each challenge, review your solutions and learn from any mistakes.
By actively participating in these events, you’ll be better prepared for the coding interview process and more confident in your abilities.
7. Review Potential Questions
One of the best ways to prepare for a coding interview is to review potential questions that might be asked. This helps you get familiar with the types of problems you might encounter and the best ways to solve them.
Types of Questions
When reviewing questions, it’s helpful to categorize them by topic. Here are some common categories:
- Arrays: Questions about arrays often involve tasks like finding the maximum sum subarray or rotating an array.
- Strings: String questions might ask you to reverse a string or find the first non-repeating character.
- Linked Lists: These questions can include finding the middle of a linked list or detecting a cycle.
- Stacks and Queues: You might be asked to implement a stack using queues or vice versa.
- Graphs: Graph questions often involve traversals like depth-first search or breadth-first search.
- Trees: Common tree questions include finding the height of a tree or checking if a tree is balanced.
- Heaps: You might need to implement a min-heap or solve problems related to priority queues.
Difficulty Levels
It’s also useful to review questions based on their difficulty level. This way, you can gradually increase the complexity of the problems you tackle.
Difficulty | Example Questions |
---|---|
Easy | Find the maximum element in an array. |
Medium | Reverse a linked list. |
Hard | Implement a LRU cache. |
Practice Resources
There are many resources available where you can find lists of potential questions. Some popular ones include:
- Online coding platforms like LeetCode, HackerRank, and CodeSignal.
- Books such as "Cracking the Coding Interview".
- Community forums and study groups.
Reviewing potential questions not only helps you get familiar with common problems but also boosts your confidence for the actual interview.
By categorizing questions and practicing them based on difficulty, you can systematically improve your problem-solving skills and be better prepared for your coding interview.
8. Books and Courses
When preparing for coding interviews, books and courses can be invaluable resources. They provide structured learning paths and cover a wide range of topics essential for success.
Recommended Books
- Cracking the Coding Interview by Gayle Laakmann McDowell: This book is highly recommended because it includes 189 programming questions across 24 categories, offering a comprehensive guide to coding interviews.
- Elements of Programming Interviews by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash: This book provides a collection of problems and solutions to help you practice and improve your coding skills.
- Introduction to Algorithms by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein: A fundamental book for understanding algorithms and data structures.
Online Courses
- Data Structures and Algorithms in Python: This course covers the basics of data structures and algorithms using Python, making it easier to understand and implement complex concepts.
- Full Stack Development with React & Node JS (Live): A comprehensive course that teaches you how to build full-stack applications using React and Node JS.
- Master Competitive Programming (Live): This course is designed to help you excel in competitive programming by covering advanced topics and problem-solving techniques.
- Placement Preparation Course: Aimed at students, this course covers everything you need to know to prepare for placement interviews, including coding, aptitude, and soft skills.
Investing time in the right books and courses can significantly boost your confidence and performance in coding interviews.
9. Research the Company
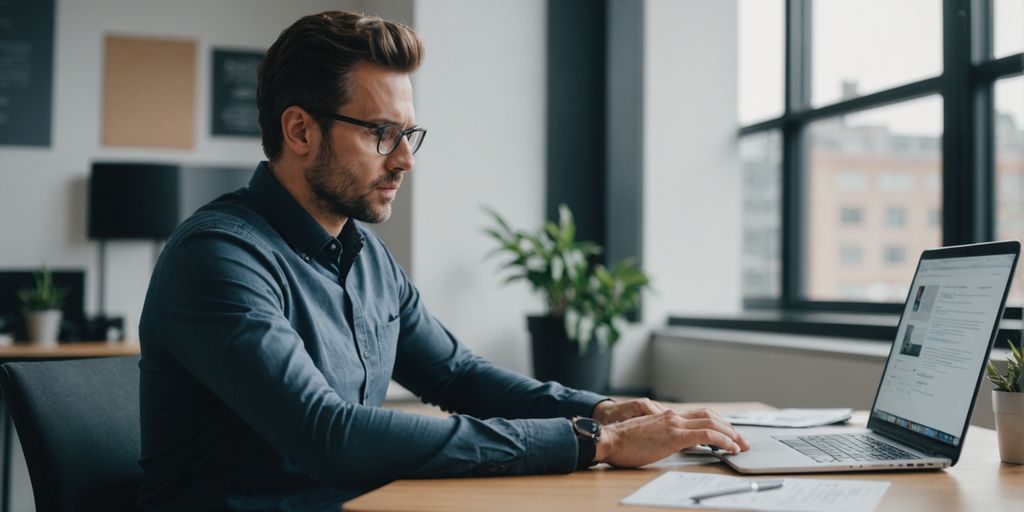
Before you step into the interview room, it’s crucial to research the company you’re applying to. This preparation can set you apart from other candidates.
Understand the Company’s Mission and Values
Start by visiting the company’s website. Look for sections like "About Us" or "Our Mission." Understanding their core values and mission can help you align your answers with what the company stands for.
Review Recent News and Projects
Check out recent news articles or press releases about the company. Knowing their latest projects or achievements can give you talking points during your interview.
Study the Company’s Tech Stack
Each company has a preferred set of technologies they use. Familiarize yourself with these tools and languages to show your readiness to hit the ground running.
Look Up Interview Experiences
Websites like Glassdoor and Reddit can provide insights into the interview process. Search for "[company name] interview questions" to find out what others have experienced.
Prepare Questions for the Interviewer
Interviewers often allocate time for you to ask questions. Prepare thoughtful questions about the company or the role to show your genuine interest.
Taking the time to research the company not only prepares you for potential questions but also shows your enthusiasm and dedication to the role.
10. Practice Mock Coding Interviews
Mock coding interviews are a crucial part of preparing for the real thing. They help you get comfortable with the interview format and reduce anxiety. Here are some tips to make the most out of your mock interviews:
- Partner with Friends or Peers: Practicing with friends or peers can be very helpful. You can take turns being the interviewer and the interviewee. This way, you get to experience both sides of the interview process.
- Use Online Platforms: Websites like interviewing.io and Pramp offer great resources for mock interviews. On these platforms, you can practice with peers or even with experienced interviewers from top tech companies.
- Get Feedback: After each mock interview, make sure to get feedback. Write down the feedback and use it to improve. The more mistakes you make and fix in mock interviews, the fewer you’ll make in the real ones.
- Simulate Real Conditions: Try to make your mock interviews as close to the real thing as possible. This means timing yourself, using a whiteboard, and even dressing up if it helps you get into the right mindset.
Practicing mock interviews with peers can be a game-changer. It helps you get used to the interview format and reduces anxiety.
By following these tips, you’ll be well-prepared for your coding interviews.
Conclusion
Preparing for a coding interview can seem like a huge task, but with the right strategies, you can make it manageable and even enjoyable. Remember to start by understanding the basics and gradually move on to more complex topics. Practice regularly, whether it’s through mock interviews, coding challenges, or community forums. Don’t forget to review your work and learn from your mistakes. Stay calm and confident, and use each interview as a learning experience. With dedication and the right approach, you’ll be well on your way to acing your coding interviews and landing your dream job.
Frequently Asked Questions
What are some key topics I should focus on for coding interviews?
You should focus on data structures like linked lists, trees, graphs, stacks, and queues. Also, algorithms such as binary search, merge sort, and quick sort are important. Concepts like recursion, dynamic programming, and Big O notation are also crucial.
How can mock interviews help in my preparation?
Mock interviews can help you practice coding under pressure, improve your communication skills, and get familiar with the interview format. They can also provide valuable feedback on your performance.
Why is it important to use pseudocode during an interview?
Using pseudocode helps you organize your thoughts and explain your approach clearly to the interviewer. It shows your problem-solving process and makes it easier to translate your plan into actual code.
What are the benefits of practicing coding by hand?
Practicing coding by hand helps you understand the logic and structure of your code better. It also prepares you for whiteboard interviews, where you might need to write code without a computer.
How can participating in coding challenges and hackathons help me?
Coding challenges and hackathons provide real-world problems to solve, which can improve your coding skills. They also offer a chance to work under time constraints and collaborate with others, simulating actual interview conditions.
What should I do if I get stuck on a problem during an interview?
If you get stuck, try to stay calm and explain your thought process to the interviewer. They may give you hints or guide you in the right direction. Showing that you can work through challenges is often as important as solving the problem.