Top Square (Block) Interview Questions for Software Engineers
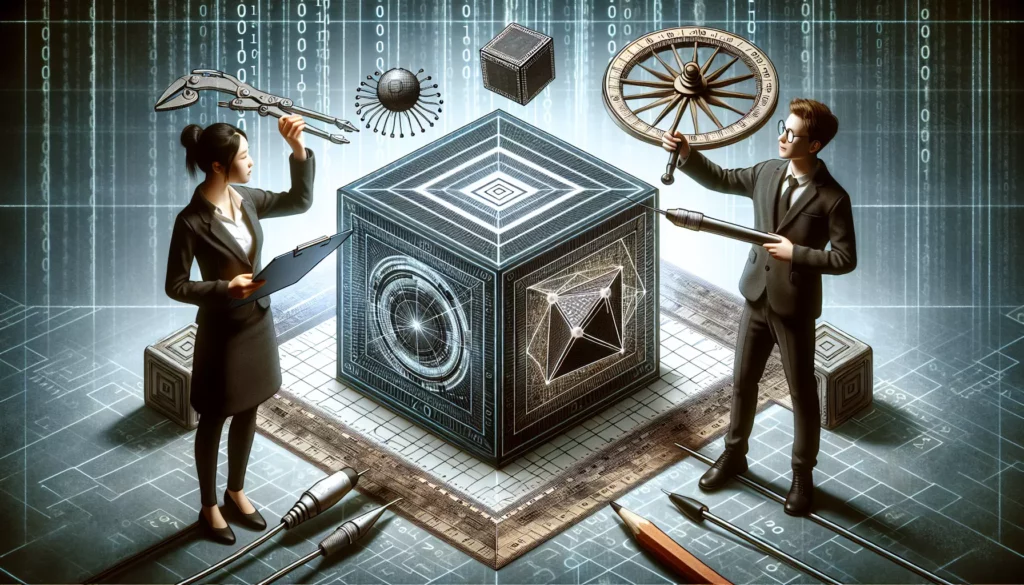
Are you preparing for a software engineering interview at Square (now known as Block)? You’re in the right place! In this comprehensive guide, we’ll explore some of the most common and challenging interview questions you might encounter during the Square hiring process. We’ll cover a range of topics, from technical questions to behavioral inquiries, and provide insights on how to approach each one effectively.
Table of Contents
- Introduction to Square (Block) Interviews
- Technical Interview Questions
- Coding Challenges and Algorithm Questions
- System Design Questions
- Behavioral Interview Questions
- Tips for Success in Square Interviews
- Conclusion
1. Introduction to Square (Block) Interviews
Square, now part of Block, is a leading financial services and digital payments company. Known for its innovative approach to technology and finance, Square attracts top talent in the software engineering field. Their interview process is designed to assess not only your technical skills but also your problem-solving abilities, cultural fit, and potential for growth within the company.
The typical interview process at Square may include:
- Initial phone screen or online assessment
- Technical phone interview
- On-site interviews (or virtual equivalent) consisting of multiple rounds
- Coding challenges
- System design discussions
- Behavioral interviews
Now, let’s dive into the types of questions you might encounter during these interviews.
2. Technical Interview Questions
Technical questions at Square are designed to assess your knowledge of fundamental computer science concepts, programming languages, and software development practices. Here are some examples:
2.1. What is the difference between a stack and a queue?
Answer: A stack and a queue are both linear data structures, but they differ in how elements are added and removed:
- Stack: Follows the Last-In-First-Out (LIFO) principle. Elements are added and removed from the same end, typically called the “top” of the stack. Think of it like a stack of plates – you add and remove from the top.
- Queue: Follows the First-In-First-Out (FIFO) principle. Elements are added at one end (rear) and removed from the other end (front). It’s similar to a line of people waiting – the first person to join the line is the first to leave.
2.2. Explain the concept of RESTful APIs.
Answer: RESTful APIs (Representational State Transfer) are a set of architectural constraints for designing networked applications. Key principles include:
- Statelessness: Each request from client to server must contain all the information needed to understand and process the request.
- Client-Server: The client and server are separated, allowing them to evolve independently.
- Cacheable: Responses must define themselves as cacheable or non-cacheable.
- Uniform Interface: A uniform way of interacting with a given server irrespective of device or type of application.
- Layered System: A client cannot ordinarily tell whether it is connected directly to the end server or an intermediary along the way.
2.3. What is the time complexity of binary search, and how does it work?
Answer: Binary search has a time complexity of O(log n), where n is the number of elements in the sorted array. It works as follows:
- Start with the middle element of the sorted array.
- If the target value is equal to the middle element, we’re done.
- If the target value is less than the middle element, repeat the search on the left half of the array.
- If the target value is greater than the middle element, repeat the search on the right half of the array.
- Repeat steps 2-4 until the element is found or it is clear the element is not present.
Here’s a simple implementation in Python:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
3. Coding Challenges and Algorithm Questions
Square’s coding challenges are designed to test your problem-solving skills, coding proficiency, and ability to optimize solutions. Here are some example questions you might encounter:
3.1. Implement a function to reverse a linked list.
Problem: Given the head of a singly linked list, reverse the list and return the reversed list.
Solution:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverseList(head):
prev = None
current = head
while current:
next_temp = current.next
current.next = prev
prev = current
current = next_temp
return prev
Explanation: This solution uses three pointers (prev, current, and next_temp) to reverse the links between nodes. We iterate through the list, changing each node’s next pointer to point to the previous node instead of the next node.
3.2. Find the longest substring without repeating characters.
Problem: Given a string s, find the length of the longest substring without repeating characters.
Solution:
def lengthOfLongestSubstring(s):
char_index = {}
max_length = 0
start = 0
for i, char in enumerate(s):
if char in char_index and char_index[char] >= start:
start = char_index[char] + 1
else:
max_length = max(max_length, i - start + 1)
char_index[char] = i
return max_length
Explanation: This solution uses a sliding window approach with a dictionary to keep track of character indices. We update the start of the window when we encounter a repeating character and calculate the maximum length of the non-repeating substring as we go.
3.3. Implement a function to check if a binary tree is balanced.
Problem: Given a binary tree, determine if it is height-balanced. A height-balanced binary tree is defined as a binary tree in which the depth of the two subtrees of every node never differ by more than 1.
Solution:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def isBalanced(root):
def check_balance(node):
if not node:
return 0
left = check_balance(node.left)
if left == -1:
return -1
right = check_balance(node.right)
if right == -1:
return -1
if abs(left - right) > 1:
return -1
return max(left, right) + 1
return check_balance(root) != -1
Explanation: This solution uses a recursive approach to check the balance of each subtree. If at any point we find an unbalanced subtree (height difference > 1), we return -1 to indicate an unbalanced tree. Otherwise, we return the height of the subtree.
4. System Design Questions
System design questions are crucial in Square interviews, especially for more senior positions. These questions assess your ability to design scalable, reliable, and efficient systems. Here are some examples:
4.1. Design a distributed key-value store.
Approach:
- Clarify requirements: consistency, availability, partition tolerance, read/write throughput, latency expectations, etc.
- Discuss data partitioning strategies (e.g., consistent hashing).
- Consider replication for fault tolerance and improved read performance.
- Address consistency models (e.g., eventual consistency, strong consistency).
- Discuss data versioning and conflict resolution mechanisms.
- Consider caching strategies to improve read performance.
- Address scalability concerns and how to handle system growth.
4.2. Design a URL shortening service like bit.ly.
Approach:
- Clarify requirements: expected traffic, storage needs, URL lifespan, etc.
- Discuss the URL shortening algorithm (e.g., base62 encoding of an incrementing ID).
- Design the database schema for storing long and short URLs.
- Consider caching strategies to improve lookup performance.
- Discuss how to handle redirects efficiently.
- Address potential issues like collision handling and security concerns.
- Consider analytics features and how to implement them.
4.3. Design a real-time chat application.
Approach:
- Clarify requirements: one-on-one chats, group chats, media sharing, etc.
- Discuss the choice of protocol for real-time communication (e.g., WebSockets).
- Design the database schema for users, messages, and conversations.
- Consider how to handle online/offline status and message delivery status.
- Discuss scalability concerns and how to handle a large number of concurrent connections.
- Address security concerns like end-to-end encryption.
- Consider additional features like push notifications and message persistence.
5. Behavioral Interview Questions
Behavioral questions are an essential part of the Square interview process. They help assess your soft skills, cultural fit, and past experiences. Here are some common behavioral questions you might encounter:
5.1. Tell me about a time when you had to deal with a difficult team member.
Approach: Use the STAR method (Situation, Task, Action, Result) to structure your answer. Focus on how you approached the situation professionally, the steps you took to improve the working relationship, and the positive outcome you achieved.
5.2. Describe a project where you had to learn a new technology quickly.
Approach: Highlight your ability to adapt and learn quickly. Discuss the strategies you used to get up to speed, any challenges you faced, and how you successfully applied the new technology to complete the project.
5.3. How do you handle disagreements with your manager?
Approach: Emphasize your communication skills and your ability to handle conflicts professionally. Discuss how you approach such situations with respect, open-mindedness, and a focus on finding the best solution for the team and the company.
5.4. Tell me about a time when you had to make a difficult decision with incomplete information.
Approach: Focus on your decision-making process, how you gathered and analyzed available information, and how you balanced the need to act with the risks of incomplete data. Discuss the outcome and any lessons learned.
5.5. Describe a situation where you went above and beyond your job responsibilities.
Approach: Highlight your initiative, work ethic, and willingness to take on additional responsibilities. Discuss the impact of your actions on the team or project, and any recognition or learning that resulted from the experience.
6. Tips for Success in Square Interviews
To increase your chances of success in Square interviews, consider the following tips:
- Practice coding regularly: Use platforms like LeetCode, HackerRank, or AlgoCademy to sharpen your coding skills and practice solving algorithmic problems.
- Review computer science fundamentals: Ensure you have a solid understanding of data structures, algorithms, and system design principles.
- Stay updated on industry trends: Square is at the forefront of fintech, so be aware of current trends in mobile payments, blockchain, and financial technology.
- Prepare your behavioral responses: Reflect on your past experiences and prepare concise, impactful stories that demonstrate your skills and problem-solving abilities.
- Research Square’s products and culture: Familiarize yourself with Square’s product lineup and company values to show genuine interest and alignment with the company’s mission.
- Ask thoughtful questions: Prepare insightful questions about the role, team, and company to demonstrate your engagement and curiosity.
- Practice explaining your thought process: During coding and system design questions, clearly communicate your approach and reasoning.
- Be ready to optimize: After solving a problem, think about ways to improve your solution in terms of time and space complexity.
- Stay calm and positive: Maintain a positive attitude throughout the interview process, even when faced with challenging questions.
- Follow up: Send a thank-you note after your interview, reiterating your interest in the position and the company.
7. Conclusion
Preparing for a Square (Block) interview requires a combination of technical proficiency, problem-solving skills, and strong communication abilities. By focusing on the areas we’ve covered in this guide – from coding challenges to system design questions and behavioral interviews – you’ll be well-equipped to showcase your talents and make a strong impression.
Remember that the interview process is not just about demonstrating your current skills, but also about showing your potential for growth and your fit within Square’s innovative culture. Stay curious, be ready to learn, and approach each question as an opportunity to showcase your unique strengths.
Good luck with your Square interview! With thorough preparation and the right mindset, you’ll be well on your way to joining one of the most exciting companies in the fintech space.