Top SQL Interview Questions: Mastering Database Queries for Your Next Tech Interview
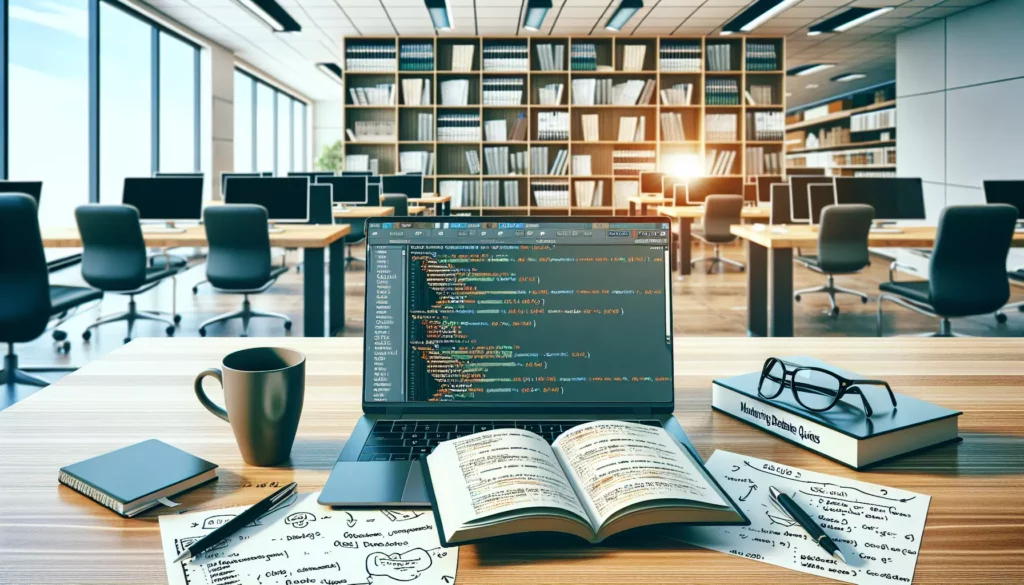
Are you preparing for a technical interview that involves SQL? Whether you’re aiming for a position at a FAANG company or any other tech firm, mastering SQL is crucial. In this comprehensive guide, we’ll explore the most common and challenging SQL interview questions, providing detailed explanations and examples to help you ace your next interview.
Table of Contents
- Introduction to SQL Interviews
- Basic SQL Interview Questions
- Intermediate SQL Interview Questions
- Advanced SQL Interview Questions
- SQL Performance Optimization Questions
- Scenario-Based SQL Questions
- Tips for Succeeding in SQL Interviews
- Conclusion
1. Introduction to SQL Interviews
SQL (Structured Query Language) is the standard language for managing and manipulating relational databases. In technical interviews, especially for roles involving data analysis, backend development, or database administration, you’re likely to encounter SQL questions. These questions assess your ability to write efficient queries, understand database design principles, and solve real-world data problems.
The questions typically range from basic syntax and operations to complex queries involving multiple joins, subqueries, and performance optimization. Let’s dive into the most common types of SQL interview questions you might encounter.
2. Basic SQL Interview Questions
These questions test your fundamental understanding of SQL syntax and basic operations.
Q1: What is SQL, and what are its main components?
Answer: SQL stands for Structured Query Language. It’s used for managing and manipulating relational databases. The main components of SQL include:
- Data Definition Language (DDL): For defining database structures (CREATE, ALTER, DROP)
- Data Manipulation Language (DML): For manipulating data (SELECT, INSERT, UPDATE, DELETE)
- Data Control Language (DCL): For controlling access to data (GRANT, REVOKE)
- Transaction Control Language (TCL): For managing transactions (COMMIT, ROLLBACK)
Q2: Write a SQL query to select all columns from a table named ’employees’.
Answer:
SELECT * FROM employees;
Q3: How do you insert a new record into a table?
Answer: You can insert a new record using the INSERT INTO statement. Here’s an example:
INSERT INTO employees (first_name, last_name, email)
VALUES ('John', 'Doe', 'john.doe@example.com');
Q4: What is the difference between CHAR and VARCHAR data types?
Answer: CHAR is a fixed-length string data type, while VARCHAR is a variable-length string data type. CHAR always uses the specified length, padding with spaces if necessary, while VARCHAR only uses as much space as needed for the actual data, up to the specified maximum length.
3. Intermediate SQL Interview Questions
These questions delve deeper into SQL concepts and test your ability to write more complex queries.
Q5: Explain the difference between INNER JOIN and LEFT JOIN.
Answer:
– INNER JOIN returns only the matching rows from both tables.
– LEFT JOIN returns all rows from the left table and the matching rows from the right table. If there’s no match, NULL values are returned for the right table’s columns.
Q6: Write a query to find the second highest salary from an ’employees’ table.
Answer: There are multiple ways to solve this. Here’s one approach:
SELECT MAX(salary) AS second_highest_salary
FROM employees
WHERE salary < (SELECT MAX(salary) FROM employees);
Q7: What is a subquery? Provide an example.
Answer: A subquery is a query nested inside another query. It can be used in various parts of an SQL statement. Here’s an example that finds employees who earn more than the average salary:
SELECT first_name, last_name, salary
FROM employees
WHERE salary > (SELECT AVG(salary) FROM employees);
Q8: Explain the purpose of the GROUP BY clause and provide an example.
Answer: The GROUP BY clause is used to group rows that have the same values in specified columns. It’s often used with aggregate functions. Here’s an example that counts the number of employees in each department:
SELECT department, COUNT(*) AS employee_count
FROM employees
GROUP BY department;
4. Advanced SQL Interview Questions
These questions test your ability to handle complex scenarios and demonstrate advanced SQL knowledge.
Q9: What are window functions in SQL? Provide an example.
Answer: Window functions perform calculations across a set of rows that are related to the current row. They are similar to aggregate functions but do not cause rows to become grouped into a single output row. Here’s an example that ranks employees by salary within each department:
SELECT
first_name,
last_name,
department,
salary,
RANK() OVER (PARTITION BY department ORDER BY salary DESC) AS salary_rank
FROM employees;
Q10: Explain the concept of a CTE (Common Table Expression) and provide an example.
Answer: A CTE is a named temporary result set that exists within the scope of a single SELECT, INSERT, UPDATE, DELETE, or MERGE statement. It’s defined using a WITH clause. Here’s an example that uses a CTE to find employees who earn more than their department’s average salary:
WITH dept_avg AS (
SELECT department, AVG(salary) AS avg_salary
FROM employees
GROUP BY department
)
SELECT e.first_name, e.last_name, e.department, e.salary
FROM employees e
JOIN dept_avg d ON e.department = d.department
WHERE e.salary > d.avg_salary;
Q11: What is a correlated subquery? How does it differ from a regular subquery?
Answer: A correlated subquery is a subquery that depends on the outer query for its values. It’s executed once for each row processed by the outer query. Unlike regular subqueries, correlated subqueries are not independent and cannot be executed on their own. Here’s an example that finds employees who earn more than the average salary in their department:
SELECT e1.first_name, e1.last_name, e1.department, e1.salary
FROM employees e1
WHERE e1.salary > (
SELECT AVG(e2.salary)
FROM employees e2
WHERE e2.department = e1.department
);
5. SQL Performance Optimization Questions
These questions focus on your ability to write efficient queries and understand database performance concepts.
Q12: What are indexes in SQL? How do they improve query performance?
Answer: Indexes are database objects that improve the speed of data retrieval operations on database tables. They work similarly to an index in a book, allowing the database engine to find data quickly without scanning the entire table. Indexes can greatly improve the performance of SELECT queries and WHERE clauses, but they may slow down INSERT, UPDATE, and DELETE operations as the index needs to be updated along with the data.
Q13: Explain the difference between a clustered and a non-clustered index.
Answer:
– Clustered Index: Determines the physical order of data in a table. There can be only one clustered index per table. The leaf nodes of a clustered index contain the actual data pages of the table.
– Non-Clustered Index: Does not determine the physical order of data. A table can have multiple non-clustered indexes. The leaf nodes of a non-clustered index contain pointers to the data rows rather than the data itself.
Q14: What is query plan optimization? How can you view the execution plan of a query?
Answer: Query plan optimization is the process by which the database engine determines the most efficient way to execute a given query. It involves choosing the best algorithms, join orders, and access methods to minimize resource usage and execution time. You can view the execution plan of a query using tools provided by your database management system. For example, in SQL Server Management Studio, you can use the “Display Estimated Execution Plan” or “Include Actual Execution Plan” options.
Q15: How can you optimize a slow-running query?
Answer: There are several strategies to optimize a slow-running query:
- Analyze the execution plan to identify bottlenecks
- Add appropriate indexes
- Rewrite the query to use more efficient joins or subqueries
- Use table partitioning for large tables
- Avoid using functions in WHERE clauses
- Use appropriate data types
- Consider denormalization for read-heavy scenarios
6. Scenario-Based SQL Questions
These questions simulate real-world scenarios and test your ability to apply SQL knowledge to solve practical problems.
Q16: Given a table of employee salaries by department, write a query to find the top 3 earners in each department.
Answer: We can use a window function with PARTITION BY to achieve this:
WITH ranked_salaries AS (
SELECT
first_name,
last_name,
department,
salary,
DENSE_RANK() OVER (PARTITION BY department ORDER BY salary DESC) AS salary_rank
FROM employees
)
SELECT first_name, last_name, department, salary
FROM ranked_salaries
WHERE salary_rank <= 3
ORDER BY department, salary DESC;
Q17: You have a table of user activities with columns user_id, activity_type, and timestamp. Write a query to find users who performed the same activity three or more times in a row.
Answer: We can use the LAG function to compare consecutive activities:
WITH consecutive_activities AS (
SELECT
user_id,
activity_type,
LAG(activity_type, 1) OVER (PARTITION BY user_id ORDER BY timestamp) AS prev_activity,
LAG(activity_type, 2) OVER (PARTITION BY user_id ORDER BY timestamp) AS prev_prev_activity
FROM user_activities
)
SELECT DISTINCT user_id
FROM consecutive_activities
WHERE activity_type = prev_activity AND activity_type = prev_prev_activity;
Q18: Given a table of orders with columns order_id, customer_id, and order_date, write a query to find customers who have made orders on three consecutive days.
Answer: We can use the LEAD function to check the next two days:
WITH consecutive_orders AS (
SELECT
customer_id,
order_date,
LEAD(order_date, 1) OVER (PARTITION BY customer_id ORDER BY order_date) AS next_day,
LEAD(order_date, 2) OVER (PARTITION BY customer_id ORDER BY order_date) AS next_next_day
FROM orders
)
SELECT DISTINCT customer_id
FROM consecutive_orders
WHERE DATEDIFF(day, order_date, next_day) = 1
AND DATEDIFF(day, next_day, next_next_day) = 1;
7. Tips for Succeeding in SQL Interviews
To excel in SQL interviews, consider the following tips:
- Practice regularly: Use online platforms like LeetCode, HackerRank, or SQL Zoo to practice SQL problems.
- Understand the basics thoroughly: Ensure you have a solid grasp of fundamental SQL concepts and syntax.
- Learn to optimize queries: Understand indexing, query execution plans, and performance optimization techniques.
- Be familiar with different database systems: While the core SQL syntax is similar, be aware of differences between major database systems like MySQL, PostgreSQL, and SQL Server.
- Explain your thought process: During the interview, verbalize your approach to solving problems. This gives the interviewer insight into your problem-solving skills.
- Ask clarifying questions: If a question is ambiguous, don’t hesitate to ask for more details or clarification.
- Review database design principles: Understand concepts like normalization, primary and foreign keys, and entity relationships.
- Practice writing complex queries: Focus on mastering joins, subqueries, and window functions.
- Stay updated: Keep abreast of new SQL features and best practices in database management.
8. Conclusion
Mastering SQL is an essential skill for many roles in the tech industry, from data analysts to backend developers. By understanding and practicing these common SQL interview questions, you’ll be well-prepared to showcase your database skills in your next technical interview.
Remember, the key to success in SQL interviews is not just memorizing syntax, but understanding the underlying concepts and being able to apply them to solve real-world problems. Continue to practice, explore more complex scenarios, and don’t be afraid to dive deep into the intricacies of database management and query optimization.
As you prepare for your interview, consider using platforms like AlgoCademy, which offer interactive coding tutorials and AI-powered assistance to help you refine your SQL skills. With dedication and practice, you’ll be well on your way to acing your SQL interview and landing your dream job in the tech industry.