Top Shopify Interview Questions: Ace Your Next Technical Interview
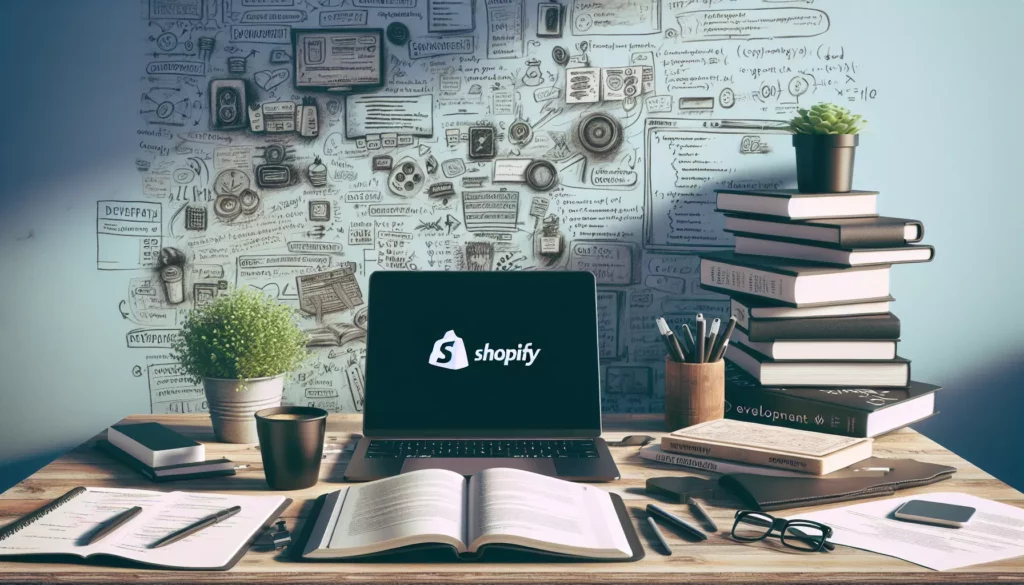
Are you preparing for a Shopify interview? Whether you’re aiming for a developer, designer, or product management role, it’s crucial to be well-prepared. In this comprehensive guide, we’ll walk you through some of the most common Shopify interview questions, provide insightful answers, and offer tips to help you succeed in your interview.
Table of Contents
- Introduction to Shopify Interviews
- Technical Questions
- Coding Challenges
- Design Questions
- Product Management Questions
- Behavioral Questions
- Tips for Success
- Conclusion
1. Introduction to Shopify Interviews
Shopify, a leading e-commerce platform, is known for its rigorous interview process. The company seeks candidates who not only possess technical skills but also demonstrate creativity, problem-solving abilities, and a passion for e-commerce. Interviews at Shopify typically involve a mix of technical questions, coding challenges, design problems, and behavioral inquiries.
Before diving into specific questions, it’s essential to understand that Shopify values candidates who are familiar with their platform, have a customer-centric mindset, and can contribute to their mission of making commerce better for everyone.
2. Technical Questions
Technical questions at Shopify can vary depending on the role you’re applying for. Here are some common questions you might encounter:
Q1: What is the difference between Ruby and Ruby on Rails?
Answer: Ruby is a programming language, while Ruby on Rails (often just called Rails) is a web application framework written in Ruby. Ruby is a general-purpose, object-oriented language, whereas Rails is a collection of tools and libraries that provide a structure for building web applications using Ruby.
Q2: Explain the MVC architecture.
Answer: MVC stands for Model-View-Controller. It’s an architectural pattern used in software design:
- Model: Represents the data and business logic of the application.
- View: Handles the presentation layer, i.e., what the user sees.
- Controller: Acts as an intermediary between the Model and View, processing user input and managing data flow.
This separation of concerns allows for more maintainable and scalable code.
Q3: What is a RESTful API?
Answer: REST (Representational State Transfer) is an architectural style for designing networked applications. A RESTful API is an interface that uses HTTP requests to GET, PUT, POST, and DELETE data. It’s stateless, meaning each request from client to server must contain all the information needed to understand and process the request.
Q4: Explain the concept of database indexing.
Answer: Database indexing is a technique used to improve the speed of data retrieval operations on a database table. It creates a data structure (index) that allows the database engine to find and access specific rows much faster, without having to search every row in a table every time it’s accessed.
Q5: What are the benefits of using GraphQL over REST?
Answer: GraphQL offers several advantages over REST:
- Flexible data fetching: Clients can request exactly what they need.
- Single endpoint: All data can be accessed through a single API endpoint.
- Strongly typed: The schema defines what’s possible, reducing errors.
- Efficient: Reduces over-fetching and under-fetching of data.
- Versioning: Easier to add new fields and types without versioning the API.
3. Coding Challenges
Shopify often includes coding challenges in their interview process. These challenges are designed to assess your problem-solving skills, coding ability, and thought process. Here are a few examples:
Challenge 1: Implement a function to reverse a string
Problem: Write a function that takes a string as input and returns the reverse of that string.
Solution (in Ruby):
def reverse_string(str)
str.reverse
end
# Alternatively, without using the built-in reverse method:
def reverse_string(str)
str.chars.reduce("") { |reversed, char| char + reversed }
end
# Test the function
puts reverse_string("Hello, Shopify!") # Output: "!yfipohS ,olleH"
Challenge 2: Find the most frequent element in an array
Problem: Write a function that takes an array of integers and returns the most frequent element in the array.
Solution (in Ruby):
def most_frequent(arr)
frequency = Hash.new(0)
arr.each { |num| frequency[num] += 1 }
frequency.max_by { |k, v| v }[0]
end
# Test the function
puts most_frequent([1, 2, 3, 2, 1, 3, 2, 2, 4, 2]) # Output: 2
Challenge 3: Implement a basic cart functionality
Problem: Create a simple shopping cart class that allows adding items, removing items, and calculating the total price.
Solution (in Ruby):
class ShoppingCart
def initialize
@items = {}
end
def add_item(item, price, quantity = 1)
@items[item] = { price: price, quantity: @items[item] ? @items[item][:quantity] + quantity : quantity }
end
def remove_item(item, quantity = 1)
return unless @items[item]
@items[item][:quantity] -= quantity
@items.delete(item) if @items[item][:quantity] <= 0
end
def total
@items.sum { |item, data| data[:price] * data[:quantity] }
end
end
# Test the shopping cart
cart = ShoppingCart.new
cart.add_item("Apple", 0.5, 3)
cart.add_item("Banana", 0.3, 2)
cart.remove_item("Apple", 1)
puts cart.total # Output: 1.6
4. Design Questions
For roles involving front-end development or UI/UX design, you might encounter questions related to design principles and user experience. Here are some examples:
Q1: How would you approach designing a new feature for Shopify’s admin interface?
Answer: When designing a new feature for Shopify’s admin interface, I would follow these steps:
- Understand the problem: Gather requirements and user needs.
- Research: Look at existing solutions and competitor approaches.
- Ideation: Brainstorm multiple solutions.
- Wireframing: Create low-fidelity mockups to visualize the layout.
- Prototyping: Develop interactive prototypes to test user flow.
- User testing: Gather feedback from actual users.
- Iteration: Refine the design based on feedback.
- Visual design: Apply Shopify’s design system to ensure consistency.
- Handoff: Prepare design assets and documentation for developers.
Q2: What are the key principles of responsive design?
Answer: The key principles of responsive design include:
- Fluid grids: Using relative units like percentages instead of fixed pixels.
- Flexible images: Ensuring images scale properly on different screen sizes.
- Media queries: Applying different styles based on device characteristics.
- Mobile-first approach: Designing for mobile devices first, then scaling up.
- Content prioritization: Displaying the most important content prominently on smaller screens.
- Touch-friendly interfaces: Designing for both mouse and touch interactions.
- Performance optimization: Ensuring fast load times across all devices.
Q3: How would you improve the checkout process for a Shopify store?
Answer: To improve the checkout process, I would consider the following:
- Simplify the form: Minimize required fields and use autofill where possible.
- Progress indicator: Show users where they are in the checkout process.
- Guest checkout option: Allow users to check out without creating an account.
- Multiple payment options: Offer various payment methods to cater to different preferences.
- Clear error messages: Provide helpful, specific error messages for form validation.
- Mobile optimization: Ensure the checkout process is smooth on mobile devices.
- Trust signals: Display security badges and customer reviews to build trust.
- One-page checkout: Consider a single-page checkout to reduce perceived complexity.
- Order summary: Provide a clear summary of the order before final confirmation.
5. Product Management Questions
For product management roles, Shopify may ask questions to assess your strategic thinking, user empathy, and ability to drive product development. Here are some examples:
Q1: How would you prioritize features for a new Shopify app?
Answer: To prioritize features for a new Shopify app, I would use the following approach:
- Conduct user research to understand merchant needs and pain points.
- Analyze competitor offerings and identify gaps in the market.
- Use a prioritization framework like RICE (Reach, Impact, Confidence, Effort) or MoSCoW (Must have, Should have, Could have, Won’t have).
- Consider the strategic alignment with Shopify’s overall goals.
- Evaluate the technical feasibility and resource requirements for each feature.
- Create a roadmap that balances quick wins with long-term value.
- Gather feedback from stakeholders and iterate on the prioritization.
Q2: How would you measure the success of a new feature?
Answer: To measure the success of a new feature, I would:
- Define clear objectives and key results (OKRs) for the feature.
- Establish relevant metrics, such as:
- Adoption rate
- User engagement (e.g., time spent, frequency of use)
- Impact on key business metrics (e.g., conversion rate, average order value)
- User satisfaction (through surveys or Net Promoter Score)
- Set up analytics tools to track these metrics.
- Conduct A/B testing to compare performance against the old version or a control group.
- Gather qualitative feedback through user interviews and support tickets.
- Monitor performance over time and compare against predetermined benchmarks.
- Analyze the data to identify areas for improvement and iterate on the feature.
Q3: How would you handle a situation where a feature you launched isn’t performing as expected?
Answer: If a launched feature isn’t performing as expected, I would take the following steps:
- Gather data: Analyze relevant metrics and user feedback to understand the problem.
- Identify root causes: Investigate potential reasons for underperformance (e.g., usability issues, lack of awareness, misalignment with user needs).
- Develop hypotheses: Form theories about why the feature isn’t meeting expectations.
- Create an action plan: Develop strategies to address the identified issues, which might include:
- Improving the user interface or experience
- Enhancing feature education and onboarding
- Adjusting the feature based on user feedback
- Increasing marketing efforts to drive adoption
- Implement changes: Roll out improvements in a phased approach.
- Monitor and iterate: Continuously track performance and make further adjustments as needed.
- Communicate transparently: Keep stakeholders informed about the situation and the steps being taken to address it.
- Learn and document: Capture lessons learned to improve future feature launches.
6. Behavioral Questions
Behavioral questions are an essential part of Shopify interviews, as they help assess your cultural fit and how you handle various situations. Here are some common behavioral questions you might encounter:
Q1: Tell me about a time when you had to deal with a difficult customer or client.
Answer approach: Use the STAR method (Situation, Task, Action, Result) to structure your response. Describe the situation, explain your role, detail the actions you took to resolve the issue, and share the positive outcome. Emphasize your communication skills, empathy, and problem-solving abilities.
Q2: Describe a project where you had to work with a team to solve a complex problem.
Answer approach: Choose a relevant project and explain the challenge you faced. Highlight your role in the team, how you collaborated with others, and the problem-solving techniques you used. Discuss the outcome and what you learned from the experience.
Q3: How do you stay up-to-date with the latest trends and technologies in your field?
Answer approach: Mention specific resources you use, such as industry blogs, podcasts, online courses, or conferences. Discuss any personal projects or contributions to open-source that demonstrate your commitment to continuous learning.
Q4: Tell me about a time when you had to adapt to a significant change at work.
Answer approach: Describe the change and its impact on your work. Explain how you adjusted your approach, learned new skills if necessary, and maintained a positive attitude. Highlight the results of your adaptability.
Q5: How do you handle constructive criticism?
Answer approach: Emphasize your openness to feedback and your view of criticism as an opportunity for growth. Provide an example of how you’ve used constructive criticism to improve your skills or performance.
7. Tips for Success
To increase your chances of success in a Shopify interview, consider the following tips:
- Research the company: Familiarize yourself with Shopify’s products, values, and recent news.
- Practice coding challenges: Use platforms like LeetCode or HackerRank to sharpen your problem-solving skills.
- Understand e-commerce: Gain a solid understanding of e-commerce concepts and challenges.
- Prepare your own questions: Have thoughtful questions ready to ask your interviewers.
- Showcase your projects: Be ready to discuss relevant projects you’ve worked on, emphasizing your role and the impact.
- Demonstrate your passion: Show enthusiasm for technology, commerce, and Shopify’s mission.
- Practice explaining technical concepts: Be prepared to explain complex ideas in simple terms.
- Be ready for remote interviews: Ensure your technology is working and you have a quiet, professional environment.
- Follow up: Send a thank-you note after the interview, reiterating your interest in the position.
8. Conclusion
Preparing for a Shopify interview requires a combination of technical knowledge, problem-solving skills, and cultural fit. By familiarizing yourself with common questions, practicing coding challenges, and understanding Shopify’s values, you’ll be well-equipped to showcase your abilities and stand out as a strong candidate.
Remember that the interview process is also an opportunity for you to evaluate if Shopify is the right fit for your career goals. Don’t hesitate to ask questions about the company culture, growth opportunities, and the specific team you’d be joining.
Good luck with your Shopify interview! With thorough preparation and a positive attitude, you’ll be well on your way to joining one of the most innovative companies in the e-commerce space.