Top Salesforce Interview Questions: Ace Your Next Tech Interview
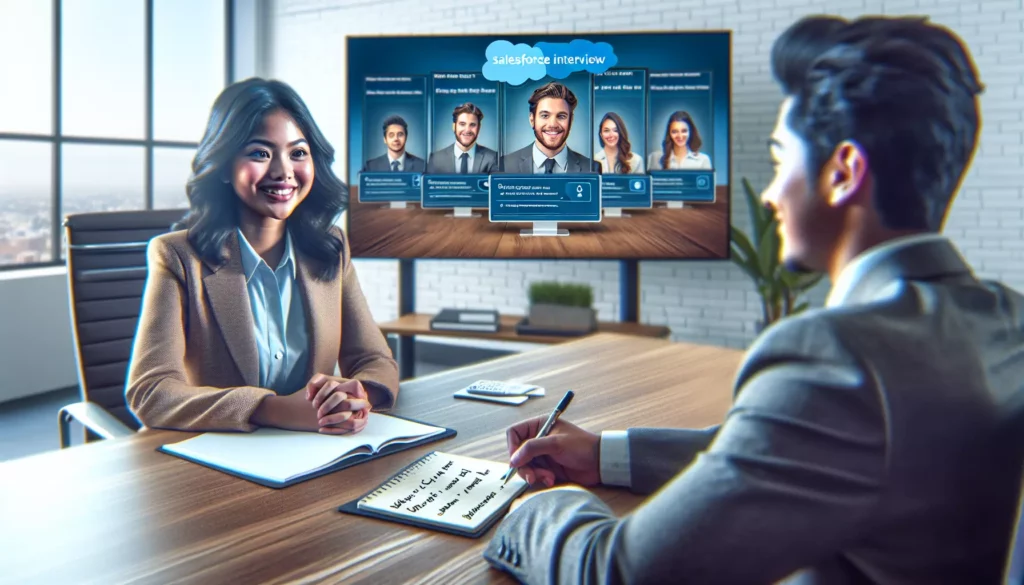
As the world’s leading customer relationship management (CRM) platform, Salesforce continues to be in high demand across industries. Whether you’re a seasoned Salesforce professional or just starting your journey in this ecosystem, being well-prepared for a Salesforce interview is crucial. In this comprehensive guide, we’ll explore the most common Salesforce interview questions, provide detailed answers, and offer tips to help you shine in your next interview.
Table of Contents
- Salesforce Basics
- Salesforce Administration
- Salesforce Development
- Apex Programming
- Lightning Components
- Integration and APIs
- Security and Sharing
- Scenario-Based Questions
- Interview Tips and Preparation Strategies
1. Salesforce Basics
Q: What is Salesforce, and what are its main components?
A: Salesforce is a cloud-based customer relationship management (CRM) platform that helps businesses manage their sales, customer service, marketing automation, analytics, and application development. The main components of Salesforce include:
- Sales Cloud: For managing sales processes and customer relationships
- Service Cloud: For customer service and support management
- Marketing Cloud: For creating and managing marketing campaigns
- Commerce Cloud: For e-commerce and online retail solutions
- Community Cloud: For building online communities and portals
- Analytics Cloud (Einstein Analytics): For data analysis and visualization
- App Cloud: For custom application development
Q: Explain the difference between Salesforce Classic and Lightning Experience.
A: Salesforce Classic is the traditional user interface of Salesforce, while Lightning Experience is the modern, more intuitive interface introduced in 2015. Key differences include:
- User Interface: Lightning has a more modern, responsive design
- Performance: Lightning is generally faster and more efficient
- Features: Lightning offers more advanced features and customization options
- App Development: Lightning Component Framework allows for faster, more flexible app development
- AI Integration: Einstein AI is more deeply integrated into Lightning Experience
Q: What are standard objects in Salesforce?
A: Standard objects are pre-built, out-of-the-box objects provided by Salesforce. Some common standard objects include:
- Account: Represents a company or organization
- Contact: Represents an individual person, typically associated with an Account
- Lead: Represents a potential sales opportunity
- Opportunity: Represents a potential deal or sale
- Case: Represents a customer issue or support ticket
- User: Represents a Salesforce user
2. Salesforce Administration
Q: What is a custom object in Salesforce, and how do you create one?
A: A custom object is a database table that allows you to store company-specific information that doesn’t fit into the standard objects. To create a custom object:
- Go to Setup
- In the Object Manager, click “Create” > “Custom Object”
- Fill in the required fields (Label, Plural Label, Object Name)
- Choose the desired options (e.g., Allow Reports, Allow Activities)
- Click “Save”
Q: Explain the difference between Workflow Rules and Process Builder.
A: Both Workflow Rules and Process Builder are automation tools in Salesforce, but they have some key differences:
- Complexity: Workflow Rules are simpler and easier to set up, while Process Builder offers more complex, multi-step processes
- Actions: Workflow Rules are limited to field updates, email alerts, tasks, and outbound messages. Process Builder can do all of these plus create records, submit for approval, and more
- Triggers: Workflow Rules can only trigger on record creation or update. Process Builder can also trigger when a record is deleted or when a platform event occurs
- Evaluation Criteria: Process Builder offers more flexibility in defining when a process should run
Q: What is a roll-up summary field, and when would you use it?
A: A roll-up summary field is a field type that automatically calculates values from related child records. It’s used in master-detail relationships to display aggregate information on the parent record. Common use cases include:
- Summing the total amount of all related opportunities on an account
- Counting the number of contacts associated with an account
- Finding the maximum or minimum value among child records
3. Salesforce Development
Q: What is Apex, and how does it differ from other programming languages?
A: Apex is Salesforce’s proprietary programming language used for customizing and extending Salesforce functionality. Key characteristics of Apex include:
- Strongly typed, object-oriented language
- Syntax similar to Java
- Designed to work with Salesforce data and features
- Includes built-in support for DML (Data Manipulation Language) operations
- Runs in a multi-tenant environment, so it has governor limits to ensure fair resource usage
Q: Explain the concept of Governor Limits in Salesforce.
A: Governor Limits are restrictions imposed by Salesforce to ensure that resources are shared fairly in the multi-tenant environment. These limits prevent any single Apex transaction from monopolizing shared resources. Some examples of Governor Limits include:
- Maximum number of SOQL queries per transaction: 100
- Maximum number of DML statements per transaction: 150
- Maximum CPU time per transaction: 10,000 milliseconds
- Maximum heap size: 6 MB
Q: What is a trigger in Salesforce, and what are the different types?
A: A trigger is Apex code that executes before or after specific data manipulation language (DML) events occur, such as insertions, updates, or deletions of Salesforce records. Types of triggers include:
- Before Insert
- After Insert
- Before Update
- After Update
- Before Delete
- After Delete
- After Undelete
4. Apex Programming
Q: Write a simple Apex trigger that updates a custom field on the Account object when a related Opportunity is closed won.
A: Here’s an example of an Apex trigger that updates a custom field “Latest_Closed_Won_Date__c” on the Account object when a related Opportunity is closed won:
trigger UpdateAccountOnOpportunityClosedWon on Opportunity (after update) {
Set<Id> accountIds = new Set<Id>();
for (Opportunity opp : Trigger.new) {
Opportunity oldOpp = Trigger.oldMap.get(opp.Id);
if (opp.IsWon && !oldOpp.IsWon && opp.AccountId != null) {
accountIds.add(opp.AccountId);
}
}
if (!accountIds.isEmpty()) {
List<Account> accountsToUpdate = new List<Account>();
for (Id accId : accountIds) {
accountsToUpdate.add(new Account(
Id = accId,
Latest_Closed_Won_Date__c = Date.today()
));
}
update accountsToUpdate;
}
}
Q: Explain the difference between SOQL and SOSL.
A: SOQL (Salesforce Object Query Language) and SOSL (Salesforce Object Search Language) are both used to retrieve data from Salesforce, but they have different purposes:
- SOQL:
- Used for retrieving records from a single object or related objects
- Similar to SQL, but designed for Salesforce data structure
- Can use relationships to query related objects
- Returns a list of sObjects
- SOSL:
- Used for text-based searches across multiple objects
- Can search text, email, and phone fields
- Faster for text searches across multiple objects
- Returns a list of lists, where each inner list contains search results for a specific object
Q: What are Apex test classes, and why are they important?
A: Apex test classes are classes specifically written to test the functionality of Apex code. They are crucial for several reasons:
- Code Coverage: Salesforce requires at least 75% code coverage for deploying Apex to production
- Quality Assurance: They help ensure that your code works as expected and handles edge cases
- Regression Testing: They allow you to quickly verify that changes haven’t broken existing functionality
- Best Practices: Writing tests encourages better code structure and modularity
5. Lightning Components
Q: What are Lightning Components, and how do they differ from Visualforce pages?
A: Lightning Components are reusable, self-contained units of an application’s user interface. Key differences from Visualforce pages include:
- Architecture: Lightning Components use a component-based architecture, while Visualforce uses a page-based approach
- Performance: Lightning Components are generally faster and more responsive
- Mobile-readiness: Lightning Components are designed to be mobile-friendly out of the box
- Reusability: Lightning Components are more easily reusable across different parts of an application
- Development Model: Lightning Components use a client-side model, while Visualforce is server-side
Q: Explain the structure of a basic Lightning Component.
A: A basic Lightning Component consists of several parts:
- Component Bundle: A collection of resources that define the component
- Component File (.cmp): The main markup file that defines the structure of the component
- Controller (.js): JavaScript file that handles the component’s client-side logic
- Helper (.js): Optional JavaScript file for sharing functions across components
- Style (.css): Optional CSS file for component-specific styling
- Documentation (.auradoc): Optional file for component documentation
- Renderer (.js): Optional JavaScript file for custom rendering
Q: How do you handle events in Lightning Components?
A: Events in Lightning Components can be handled in several ways:
- Component Events: Use
component.getEvent()
to create and fire events within a component - Application Events: Use
$A.get("e.c:eventName")
to create and fire events across the entire application - Event Handlers: Define methods in the component’s controller to handle events
- Event Attributes: Use attributes like
onclick="{!c.handleClick}"
to bind events to handler methods
6. Integration and APIs
Q: What is the Salesforce REST API, and how is it used?
A: The Salesforce REST API is a web service that allows external applications to interact with Salesforce data and functionality. It’s used for:
- Integrating Salesforce with external systems
- Building mobile and web applications that interact with Salesforce
- Performing CRUD (Create, Read, Update, Delete) operations on Salesforce records
- Executing SOQL and SOSL queries
- Accessing metadata about your Salesforce org
Q: Explain the difference between Outbound and Inbound integration in Salesforce.
A: Outbound and Inbound integration refer to the direction of data flow between Salesforce and external systems:
- Outbound Integration:
- Data flows from Salesforce to an external system
- Examples: Outbound Messages, Apex Callouts, Platform Events
- Use cases: Sending Salesforce data to external applications or databases
- Inbound Integration:
- Data flows from an external system into Salesforce
- Examples: Web-to-Lead, Email-to-Case, REST API, SOAP API
- Use cases: Importing data from external sources, creating records from web forms
Q: What are Connected Apps in Salesforce?
A: Connected Apps are applications that integrate with Salesforce using APIs. They allow external applications to authenticate with Salesforce and access Salesforce data. Key aspects of Connected Apps include:
- OAuth 2.0 authentication
- Single Sign-On (SSO) capabilities
- Mobile app integration
- API access control
- Custom attributes for sharing user data
7. Security and Sharing
Q: Explain the difference between Profiles and Permission Sets in Salesforce.
A: Profiles and Permission Sets are both used to control user access in Salesforce, but they have some key differences:
- Profiles:
- Every user must have exactly one profile
- Provide a baseline of permissions for users
- Control object permissions, field-level security, and app visibility
- Can be used to control login hours and IP restrictions
- Permission Sets:
- Users can have multiple permission sets
- Used to grant additional permissions beyond what’s in a user’s profile
- More flexible and easier to maintain than profiles
- Can be assigned temporarily for specific tasks or projects
Q: What is Role Hierarchy in Salesforce, and how does it affect record sharing?
A: Role Hierarchy in Salesforce is a tree-like structure that represents the organizational hierarchy of a company. It affects record sharing in the following ways:
- Users higher in the hierarchy can access records owned by users below them
- Sharing rules can be based on roles or role hierarchies
- It allows for automatic upward sharing of records
- It doesn’t automatically grant edit access; that’s controlled by sharing settings
Q: Describe the different types of sharing rules in Salesforce.
A: Salesforce offers several types of sharing rules to control record access:
- Owner-Based Sharing Rules: Share records based on the record owner’s role, territory, or public group
- Criteria-Based Sharing Rules: Share records that meet specific field criteria
- Guest User Sharing Rules: Control record access for unauthenticated guest users in communities
- Manual Sharing: Allows record owners to share individual records with specific users or groups
- Apex Managed Sharing: Programmatically control sharing using Apex code
8. Scenario-Based Questions
Q: A client wants to automatically create a follow-up task whenever a high-value opportunity is closed. How would you implement this?
A: To implement this requirement, you could use one of the following approaches:
- Process Builder:
- Create a new Process on the Opportunity object
- Set the process to trigger when a record is created or edited
- Add criteria to check if the opportunity is closed and meets the high-value threshold
- Add an action to create a new Task record with the required details
- Apex Trigger:
- Create a trigger on the Opportunity object
- In the trigger, check if the opportunity is being closed and meets the high-value criteria
- If conditions are met, create a new Task record and insert it
Q: Your organization wants to track the total value of all closed-won opportunities for each account in the current fiscal year. How would you approach this?
A: To implement this requirement, you could:
- Create a custom field on the Account object (e.g., “Total_Closed_Won_This_FY__c”)
- Use a Roll-Up Summary field if the relationship between Account and Opportunity is Master-Detail
- If using a Lookup relationship, create an Apex trigger on the Opportunity object:
- Trigger should fire on insert, update, and delete of Opportunities
- Query all closed-won Opportunities for the related Account in the current fiscal year
- Sum the values and update the custom field on the Account
- Optionally, create a scheduled Apex job to recalculate the values periodically to ensure accuracy
Q: A sales team wants to be notified when a lead hasn’t been contacted in 30 days. How would you implement this automation?
A: To implement this automation, you could use the following approach:
- Create a custom Date field on the Lead object to track the last contact date
- Use Process Builder or Flow to update this field whenever a task or event related to the lead is completed
- Create a scheduled Apex class that runs daily to:
- Query for Leads where the last contact date is more than 30 days ago
- Send an email notification to the Lead owner or a specified group
- Optionally, create a task for the Lead owner to follow up
- Schedule the Apex class to run daily using the Salesforce Scheduler
9. Interview Tips and Preparation Strategies
General Tips for Salesforce Interviews
- Stay updated with the latest Salesforce releases and features
- Practice explaining complex concepts in simple terms
- Be prepared to discuss real-world projects you’ve worked on
- Familiarize yourself with Salesforce best practices and design patterns
- Be ready to write code or pseudocode during the interview
- Understand the company and the specific role you’re applying for
Preparation Strategies
- Review Salesforce documentation and Trailhead modules
- Practice coding problems on platforms like LeetCode or HackerRank
- Participate in Salesforce community forums and discussions
- Work on personal projects to gain hands-on experience
- Prepare a portfolio of your Salesforce projects and certifications
- Practice mock interviews with peers or mentors
Key Areas to Focus On
- Salesforce fundamentals and architecture
- Apex programming and best practices
- Lightning Component development
- Integration patterns and API usage
- Security models and data sharing
- Performance optimization and best practices
- Salesforce platform limitations and how to work around them
Remember, Salesforce interviews often assess not just your technical knowledge but also your problem-solving skills and ability to apply Salesforce concepts to real-world scenarios. Be prepared to discuss your experiences and how you’ve overcome challenges in your Salesforce projects.
By thoroughly preparing for these common Salesforce interview questions and following the tips provided, you’ll be well-equipped to showcase your expertise and land your dream Salesforce role. Good luck with your interview!