Top Rust Interview Questions: Mastering the Language for Your Next Tech Interview
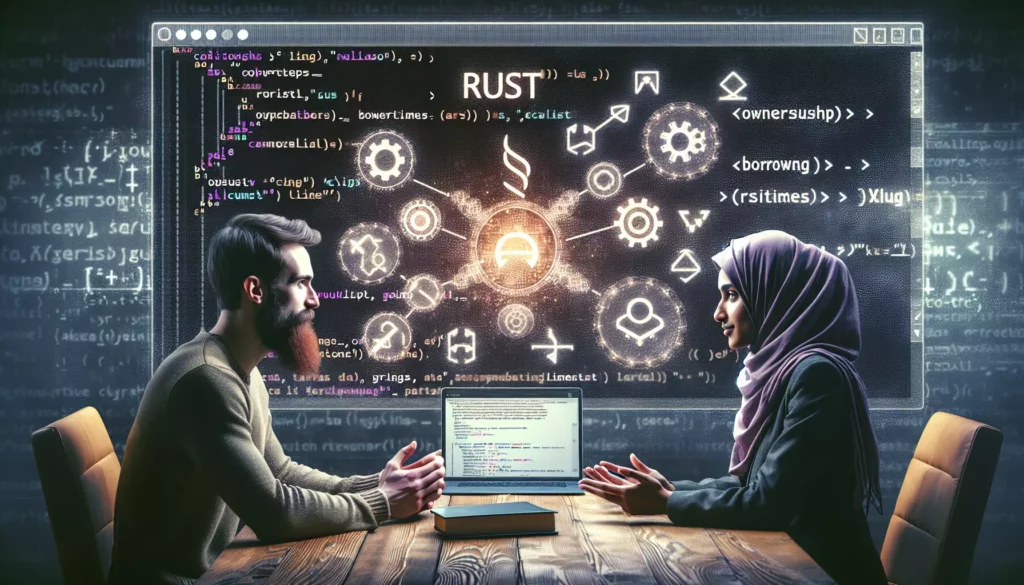
As the demand for Rust developers continues to grow, it’s crucial to be well-prepared for interviews in this powerful systems programming language. Whether you’re a seasoned Rust developer or just starting your journey, this comprehensive guide will help you tackle common Rust interview questions with confidence. We’ll cover a wide range of topics, from basic syntax to advanced concepts, ensuring you’re ready to showcase your Rust expertise in your next tech interview.
1. What is Rust, and what are its main features?
Rust is a systems programming language that focuses on safety, concurrency, and performance. Its main features include:
- Memory safety without garbage collection
- Concurrency without data races
- Zero-cost abstractions
- Pattern matching
- Type inference
- Minimal runtime
- Efficient C bindings
Rust’s unique ownership system and borrowing rules ensure memory safety and prevent common programming errors like null or dangling pointer references, buffer overflows, and data races.
2. Explain Rust’s ownership system.
Rust’s ownership system is a central feature that ensures memory safety without the need for a garbage collector. The key principles of ownership are:
- Each value in Rust has an owner (a variable).
- There can only be one owner at a time.
- When the owner goes out of scope, the value is dropped (memory is freed).
Here’s an example demonstrating ownership:
fn main() {
let s1 = String::from("hello");
let s2 = s1; // s1 is moved to s2, s1 is no longer valid
// println!("{}", s1); // This would cause a compilation error
println!("{}", s2); // This is valid
}
In this example, the ownership of the String is transferred from s1 to s2. After the move, s1 is no longer valid, and attempting to use it would result in a compilation error.
3. What are references and borrowing in Rust?
References and borrowing are concepts in Rust that allow you to refer to a value without taking ownership of it. There are two types of references:
- Shared references (&T): Allow multiple read-only borrows
- Mutable references (&mut T): Allow a single mutable borrow
Here’s an example demonstrating references and borrowing:
fn main() {
let mut s = String::from("hello");
let r1 = &s; // Shared reference
let r2 = &s; // Multiple shared references are allowed
println!("{} and {}", r1, r2);
let r3 = &mut s; // Mutable reference
r3.push_str(", world");
println!("{}", r3);
// println!("{}", r1); // This would cause a compilation error
}
In this example, we create shared references (r1 and r2) and a mutable reference (r3) to the String s. Note that you cannot have both shared and mutable references to the same value at the same time.
4. How does Rust handle null or nil values?
Rust does not have null or nil values. Instead, it uses the Option<T> enum to represent the presence or absence of a value. The Option<T> enum has two variants:
- Some(T): Represents the presence of a value of type T
- None: Represents the absence of a value
Here’s an example of using Option<T>:
fn divide(numerator: f64, denominator: f64) -> Option<f64> {
if denominator == 0.0 {
None
} else {
Some(numerator / denominator)
}
}
fn main() {
let result = divide(10.0, 2.0);
match result {
Some(x) => println!("Result: {}", x),
None => println!("Cannot divide by zero"),
}
}
In this example, the divide function returns an Option<f64>. If the denominator is zero, it returns None; otherwise, it returns Some with the result of the division.
5. What are traits in Rust, and how are they used?
Traits in Rust are similar to interfaces in other languages. They define a set of methods that types can implement. Traits allow for abstraction and code reuse. Here’s an example of defining and implementing a trait:
trait Printable {
fn print(&self);
}
struct Person {
name: String,
age: u32,
}
impl Printable for Person {
fn print(&self) {
println!("Name: {}, Age: {}", self.name, self.age);
}
}
fn main() {
let person = Person {
name: String::from("Alice"),
age: 30,
};
person.print();
}
In this example, we define a Printable trait and implement it for the Person struct. Traits can also have default implementations and can be used as bounds on generic types.
6. Explain the difference between Stack and Heap memory in Rust.
In Rust, memory management is handled through the stack and heap:
- Stack: Fast memory allocation for fixed-size, known at compile-time data. Variables stored on the stack must have a known, fixed size.
- Heap: Dynamic memory allocation for data with a size unknown at compile time or that might change. Heap allocations are slower and managed through smart pointers like Box<T>.
Here’s an example illustrating the difference:
fn main() {
// Stack allocation
let x = 5; // Integer stored on the stack
// Heap allocation
let y = Box::new(5); // Integer stored on the heap
println!("x = {}, y = {}", x, *y);
}
In this example, x is stored on the stack, while y is a Box<i32> that stores the integer on the heap and keeps a pointer to it on the stack.
7. What are lifetimes in Rust, and why are they important?
Lifetimes in Rust are a way to express the scope for which references are valid. They are part of Rust’s borrow checker and help prevent dangling references. Lifetimes are usually implicit, but sometimes need to be explicitly annotated.
Here’s an example demonstrating lifetimes:
fn longest<'a>(x: &'a str, y: &'a str) -> &'a str {
if x.len() > y.len() {
x
} else {
y
}
}
fn main() {
let s1 = String::from("short");
let s2 = String::from("longer");
let result = longest(&s1, &s2);
println!("Longest string: {}", result);
}
In this example, the ‘a lifetime annotation ensures that the returned reference will be valid for as long as both input references are valid.
8. How does Rust handle error handling?
Rust uses the Result<T, E> enum for error handling. It has two variants:
- Ok(T): Represents a successful result containing a value of type T
- Err(E): Represents an error containing an error value of type E
Here’s an example of error handling in Rust:
use std::fs::File;
use std::io::Read;
fn read_file_contents(path: &str) -> Result<String, std::io::Error> {
let mut file = File::open(path)?;
let mut contents = String::new();
file.read_to_string(&mut contents)?;
Ok(contents)
}
fn main() {
match read_file_contents("example.txt") {
Ok(contents) => println!("File contents: {}", contents),
Err(error) => println!("Error reading file: {}", error),
}
}
In this example, the read_file_contents function returns a Result. The ? operator is used for error propagation, automatically returning an Err if an operation fails.
9. What are closures in Rust?
Closures in Rust are anonymous functions that can capture values from their environment. They are similar to lambdas in other languages. Closures can be used as arguments to functions or stored in variables.
Here’s an example of using closures:
fn main() {
let x = 5;
let add_x = |y| x + y;
println!("Result: {}", add_x(3)); // Prints: Result: 8
let numbers = vec![1, 2, 3, 4, 5];
let doubled: Vec<i32> = numbers.iter().map(|&n| n * 2).collect();
println!("Doubled numbers: {:?}", doubled);
}
In this example, we define a closure add_x that captures the value of x from its environment. We also use a closure with the map function to double each number in a vector.
10. How does Rust support concurrency and parallelism?
Rust provides several mechanisms for concurrency and parallelism:
- Threads: Rust’s standard library includes support for OS-level threads.
- Channels: Rust provides channels for communication between threads.
- Mutex and Arc: For shared state between threads.
- Async/Await: For asynchronous programming.
Here’s an example of using threads and channels:
use std::thread;
use std::sync::mpsc;
fn main() {
let (tx, rx) = mpsc::channel();
thread::spawn(move || {
let val = String::from("hello");
tx.send(val).unwrap();
});
let received = rx.recv().unwrap();
println!("Got: {}", received);
}
In this example, we create a new thread that sends a message through a channel to the main thread.
11. What are smart pointers in Rust?
Smart pointers in Rust are data structures that act like pointers but also have additional metadata and capabilities. Common smart pointers in Rust include:
- Box<T>: For allocating values on the heap
- Rc<T>: A reference-counted smart pointer for shared ownership
- Arc<T>: An atomically reference-counted smart pointer for thread-safe shared ownership
- RefCell<T>: For interior mutability
Here’s an example using Box<T>:
enum List {
Cons(i32, Box<List>),
Nil,
}
use List::{Cons, Nil};
fn main() {
let list = Cons(1, Box::new(Cons(2, Box::new(Cons(3, Box::new(Nil))))));
}
In this example, we use Box<T> to create a recursive data structure (a linked list) that would otherwise have an infinite size.
12. How does Rust handle generics?
Rust supports generics, allowing you to write code that works with multiple types. Generics can be used with functions, structs, enums, and traits. Here’s an example of a generic function:
fn largest<T: PartialOrd>(list: &[T]) -> &T {
let mut largest = &list[0];
for item in list.iter() {
if item > largest {
largest = item;
}
}
largest
}
fn main() {
let numbers = vec![34, 50, 25, 100, 65];
let result = largest(&numbers);
println!("The largest number is {}", result);
let chars = vec!['y', 'm', 'a', 'q'];
let result = largest(&chars);
println!("The largest char is {}", result);
}
In this example, the largest function works with any type T that implements the PartialOrd trait, allowing it to be used with both numbers and characters.
13. What is the #[derive] attribute in Rust?
The #[derive] attribute in Rust allows you to automatically implement certain traits for custom types. Common traits that can be derived include:
- Debug: For formatting debug output
- Clone: For creating a deep copy of a value
- Copy: For types that can be copied bit-for-bit
- PartialEq and Eq: For equality comparisons
- PartialOrd and Ord: For ordering comparisons
Here’s an example:
#[derive(Debug, Clone, PartialEq)]
struct Point {
x: f64,
y: f64,
}
fn main() {
let p1 = Point { x: 1.0, y: 2.0 };
let p2 = p1.clone();
println!("p1: {:?}", p1);
println!("p1 == p2: {}", p1 == p2);
}
In this example, we derive Debug, Clone, and PartialEq for the Point struct, allowing us to print debug output, clone instances, and compare them for equality.
14. How does Rust handle string manipulation?
Rust has two main string types:
- String: A growable, heap-allocated string
- &str: A string slice, often used as a view into a String or a string literal
Here’s an example demonstrating string manipulation:
fn main() {
// Creating a String
let mut s = String::from("Hello");
// Appending to a String
s.push_str(", world!");
// Converting a String to a str slice
let slice = &s[..];
// String concatenation
let s1 = String::from("Hello, ");
let s2 = String::from("world!");
let s3 = s1 + &s2; // Note: s1 is moved here and can no longer be used
println!("s: {}", s);
println!("slice: {}", slice);
println!("s3: {}", s3);
}
This example shows creating, modifying, and concatenating strings, as well as creating string slices.
15. What are macros in Rust, and how are they used?
Macros in Rust are a way to write code that writes other code, known as metaprogramming. They allow you to reduce repetition in your code and create domain-specific languages. There are two types of macros in Rust:
- Declarative macros: Defined using macro_rules!
- Procedural macros: More powerful, can be used to generate code from attributes
Here’s an example of a simple declarative macro:
macro_rules! say_hello {
() => {
println!("Hello!");
};
($name:expr) => {
println!("Hello, {}!", $name);
};
}
fn main() {
say_hello!(); // Prints: Hello!
say_hello!("Alice"); // Prints: Hello, Alice!
}
In this example, we define a macro say_hello that can be called with or without an argument.
Conclusion
This comprehensive guide covers many of the key concepts and features of Rust that you’re likely to encounter in a technical interview. By understanding these topics and practicing with the provided examples, you’ll be well-prepared to showcase your Rust knowledge and skills.
Remember that Rust is a complex language with many nuances, so it’s essential to continue exploring and practicing beyond these interview questions. Some additional areas to focus on include:
- Unsafe Rust and when to use it
- Advanced trait techniques (trait objects, associated types)
- Asynchronous programming with async/await
- The Rust module system
- Writing and running tests in Rust
- Rust’s standard library and common crates
As you continue your Rust journey, make sure to refer to the official Rust documentation, participate in the Rust community, and work on practical projects to deepen your understanding of the language. Good luck with your Rust interviews!