Top Robinhood Interview Questions: Ace Your Tech Interview
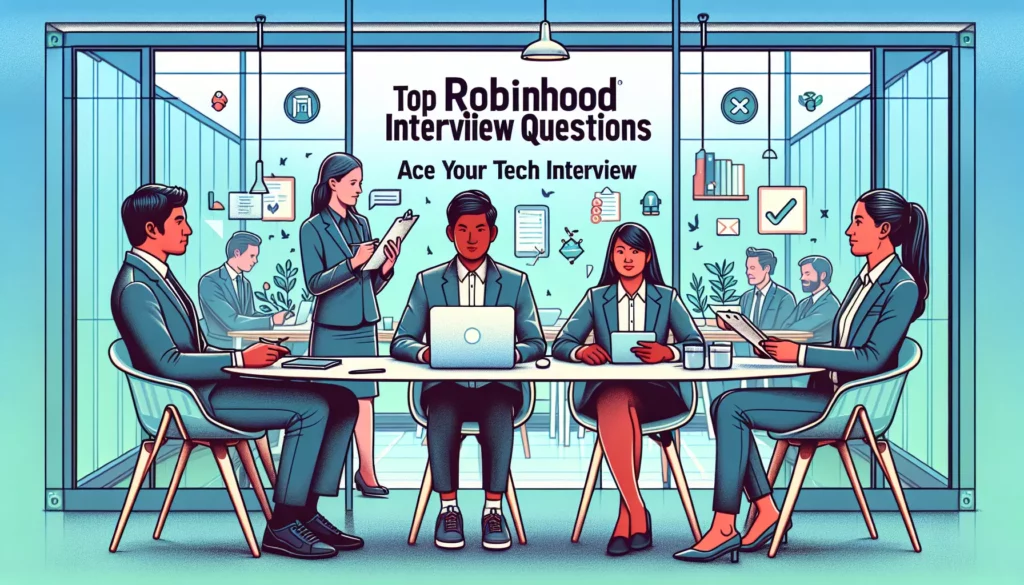
Are you preparing for a technical interview at Robinhood? You’ve come to the right place! In this comprehensive guide, we’ll explore some of the most common Robinhood interview questions, provide insights into the company’s interview process, and offer tips to help you succeed. Whether you’re a seasoned developer or just starting your career in fintech, this article will equip you with the knowledge and strategies needed to excel in your Robinhood interview.
Table of Contents
- Understanding Robinhood: Company Overview
- The Robinhood Interview Process
- Technical Interview Questions
- Behavioral Interview Questions
- System Design Questions
- Coding Challenges
- Tips for Success
- Conclusion
1. Understanding Robinhood: Company Overview
Before diving into the interview questions, it’s essential to understand Robinhood’s mission, values, and the technology stack they use. Robinhood is a financial services company that offers commission-free trading of stocks, exchange-traded funds (ETFs), options, and cryptocurrencies through a mobile app. The company’s mission is to democratize finance for all by making investing accessible to everyone.
Robinhood’s tech stack includes:
- Python for backend services
- React Native for mobile app development
- Go for high-performance systems
- PostgreSQL and Apache Cassandra for databases
- Apache Kafka for real-time data streaming
- Docker and Kubernetes for containerization and orchestration
Understanding these technologies and how they fit into Robinhood’s ecosystem can give you an edge during the interview process.
2. The Robinhood Interview Process
The Robinhood interview process typically consists of several stages:
- Initial Phone Screen: A brief conversation with a recruiter to discuss your background and the role.
- Technical Phone Interview: A coding interview conducted remotely, usually focusing on data structures and algorithms.
- On-site Interviews: A series of interviews that may include:
- Coding interviews
- System design discussions
- Behavioral interviews
- Technical deep dives related to your area of expertise
- Final Interview: Sometimes, there’s a final round with a senior leader or hiring manager.
Now, let’s explore some common questions you might encounter during these interviews.
3. Technical Interview Questions
Robinhood’s technical interviews often focus on data structures, algorithms, and problem-solving skills. Here are some example questions you might encounter:
3.1. Array and String Manipulation
Q: Given an array of integers, find two numbers such that they add up to a specific target number.
This is a classic problem that can be solved efficiently using a hash table. Here’s a Python implementation:
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
# Example usage
nums = [2, 7, 11, 15]
target = 9
result = two_sum(nums, target)
print(result) # Output: [0, 1]
3.2. Linked Lists
Q: Implement a function to reverse a singly linked list.
This problem tests your understanding of linked list operations. Here’s a Python solution:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverse_linked_list(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
# Example usage
# Create a linked list: 1 -> 2 -> 3 -> 4 -> 5
head = ListNode(1)
head.next = ListNode(2)
head.next.next = ListNode(3)
head.next.next.next = ListNode(4)
head.next.next.next.next = ListNode(5)
# Reverse the linked list
new_head = reverse_linked_list(head)
# Print the reversed list
current = new_head
while current:
print(current.val, end=" ")
current = current.next
# Output: 5 4 3 2 1
3.3. Trees and Graphs
Q: Implement a function to check if a binary tree is balanced.
This question tests your understanding of tree traversal and recursive problem-solving. Here’s a Python implementation:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def is_balanced(root):
def check_height(node):
if not node:
return 0
left_height = check_height(node.left)
if left_height == -1:
return -1
right_height = check_height(node.right)
if right_height == -1:
return -1
if abs(left_height - right_height) > 1:
return -1
return max(left_height, right_height) + 1
return check_height(root) != -1
# Example usage
# Create a balanced binary tree
# 1
# / \
# 2 3
# / \
# 4 5
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
print(is_balanced(root)) # Output: True
# Create an unbalanced binary tree
# 1
# /
# 2
# /
# 3
unbalanced_root = TreeNode(1)
unbalanced_root.left = TreeNode(2)
unbalanced_root.left.left = TreeNode(3)
print(is_balanced(unbalanced_root)) # Output: False
3.4. Dynamic Programming
Q: Given a list of stock prices, find the maximum profit you can achieve by buying and selling stocks.
This problem can be solved using dynamic programming. Here’s a Python solution:
def max_profit(prices):
if not prices:
return 0
max_profit = 0
min_price = float('inf')
for price in prices:
if price < min_price:
min_price = price
else:
max_profit = max(max_profit, price - min_price)
return max_profit
# Example usage
prices = [7, 1, 5, 3, 6, 4]
print(max_profit(prices)) # Output: 5
4. Behavioral Interview Questions
Robinhood also places a strong emphasis on cultural fit and soft skills. Here are some behavioral questions you might encounter:
- Tell me about a time when you had to work on a project with tight deadlines. How did you manage your time and priorities?
- Describe a situation where you had to collaborate with a difficult team member. How did you handle it?
- Can you share an example of a time when you had to learn a new technology quickly? How did you approach the learning process?
- Tell me about a project you’re particularly proud of. What was your role, and what were the outcomes?
- How do you stay up-to-date with the latest trends and technologies in your field?
- Describe a time when you had to make a difficult decision with limited information. How did you approach the decision-making process?
- Tell me about a time when you received constructive criticism. How did you respond, and what did you learn from it?
- How do you approach debugging complex issues in production systems?
When answering these questions, use the STAR method (Situation, Task, Action, Result) to structure your responses and provide concrete examples from your past experiences.
5. System Design Questions
For more senior positions, Robinhood may include system design questions in their interviews. These questions assess your ability to design scalable, reliable, and efficient systems. Some example questions might include:
- Design a real-time stock trading system.
- How would you design a notification system for Robinhood’s mobile app?
- Design a system to handle millions of concurrent users placing trades during market hours.
- How would you implement a feature to display real-time price charts for stocks?
- Design a system to detect and prevent fraudulent transactions.
When approaching system design questions, consider the following aspects:
- Requirements clarification
- System interface definition
- Back-of-the-envelope estimation
- Defining data model
- High-level design
- Detailed design
- Identifying and resolving bottlenecks
6. Coding Challenges
Robinhood may also present you with coding challenges during the interview process. These challenges are designed to assess your problem-solving skills, coding ability, and attention to detail. Here’s an example of a coding challenge you might encounter:
Q: Implement a simple order book for a trading system.
This challenge tests your ability to work with data structures and implement core functionality of a trading system. Here’s a Python implementation:
from collections import defaultdict
import heapq
class OrderBook:
def __init__(self):
self.buy_orders = defaultdict(list)
self.sell_orders = defaultdict(list)
self.buy_prices = []
self.sell_prices = []
def add_order(self, side, price, quantity):
if side == 'buy':
heapq.heappush(self.buy_prices, -price)
self.buy_orders[price].append(quantity)
elif side == 'sell':
heapq.heappush(self.sell_prices, price)
self.sell_orders[price].append(quantity)
def match_orders(self):
while self.buy_prices and self.sell_prices:
best_buy = -self.buy_prices[0]
best_sell = self.sell_prices[0]
if best_buy >= best_sell:
buy_quantity = self.buy_orders[best_buy].pop(0)
sell_quantity = self.sell_orders[best_sell].pop(0)
if buy_quantity > sell_quantity:
self.buy_orders[best_buy].insert(0, buy_quantity - sell_quantity)
elif sell_quantity > buy_quantity:
self.sell_orders[best_sell].insert(0, sell_quantity - buy_quantity)
if not self.buy_orders[best_buy]:
heapq.heappop(self.buy_prices)
if not self.sell_orders[best_sell]:
heapq.heappop(self.sell_prices)
print(f"Matched: {min(buy_quantity, sell_quantity)} @ {best_sell}")
else:
break
def display_order_book(self):
print("Buy Orders:")
for price in sorted(self.buy_orders.keys(), reverse=True):
for quantity in self.buy_orders[price]:
print(f" {quantity} @ {price}")
print("Sell Orders:")
for price in sorted(self.sell_orders.keys()):
for quantity in self.sell_orders[price]:
print(f" {quantity} @ {price}")
# Example usage
order_book = OrderBook()
order_book.add_order('buy', 100, 10)
order_book.add_order('buy', 99, 5)
order_book.add_order('sell', 101, 8)
order_book.add_order('sell', 102, 7)
print("Initial Order Book:")
order_book.display_order_book()
print("\nMatching orders:")
order_book.match_orders()
print("\nUpdated Order Book:")
order_book.display_order_book()
order_book.add_order('buy', 102, 6)
print("\nAfter adding a new buy order:")
order_book.display_order_book()
print("\nMatching orders:")
order_book.match_orders()
print("\nFinal Order Book:")
order_book.display_order_book()
7. Tips for Success
To increase your chances of success in a Robinhood interview, consider the following tips:
- Brush up on your fundamentals: Review core computer science concepts, data structures, and algorithms.
- Practice coding: Solve coding problems on platforms like LeetCode, HackerRank, or AlgoCademy to improve your problem-solving skills.
- Understand Robinhood’s business: Familiarize yourself with Robinhood’s products, services, and recent news.
- Be prepared to discuss your projects: Have detailed explanations ready for the projects listed on your resume.
- Ask thoughtful questions: Prepare questions about the role, team, and company to show your genuine interest.
- Communicate clearly: Practice explaining your thought process and problem-solving approach out loud.
- Stay calm under pressure: Remember that interviewers are more interested in your problem-solving approach than perfect solutions.
- Follow up: Send a thank-you note to your interviewers after the interview, reiterating your interest in the position.
8. Conclusion
Preparing for a Robinhood interview requires a combination of technical skills, problem-solving ability, and cultural fit. By familiarizing yourself with the types of questions asked, practicing coding challenges, and understanding the company’s mission and values, you’ll be well-equipped to tackle the interview process.
Remember that interviews are also an opportunity for you to evaluate whether Robinhood is the right fit for your career goals. Don’t hesitate to ask questions about the company culture, growth opportunities, and the specific team you’d be joining.
Good luck with your Robinhood interview! With thorough preparation and a positive attitude, you’ll be well on your way to joining one of the most innovative fintech companies in the industry.