Top Reddit Interview Questions: Ace Your Tech Interview
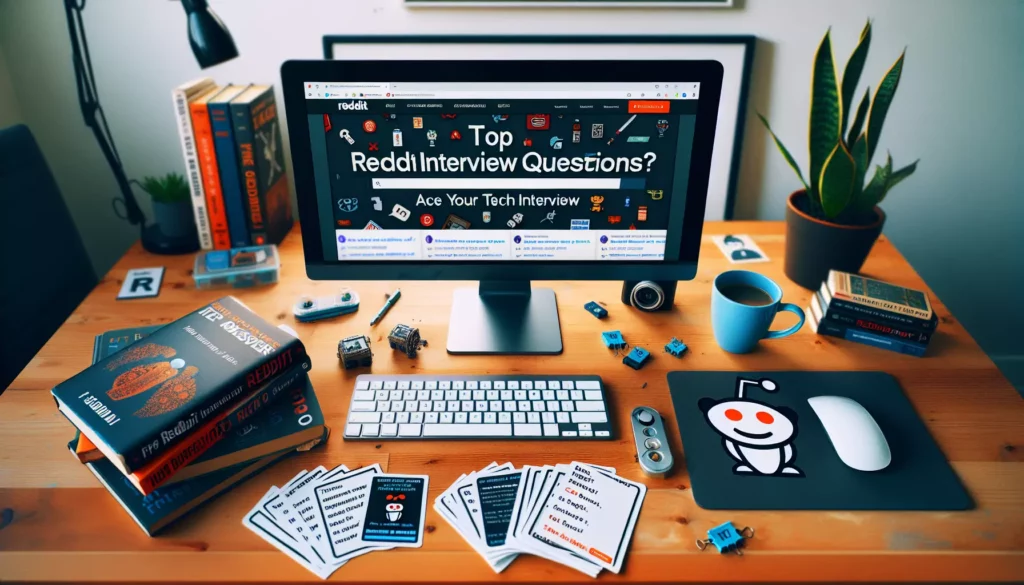
Are you gearing up for a technical interview and looking for insider information on what to expect? Look no further! In this comprehensive guide, we’ll dive deep into the most common Reddit interview questions, providing you with valuable insights and strategies to help you succeed. Whether you’re aiming for a position at a FAANG company or any other tech giant, mastering these questions will give you a significant edge in your interview preparation.
Table of Contents
- Introduction to Reddit Interview Questions
- Data Structures and Algorithms
- System Design Questions
- Coding Challenges
- Behavioral Questions
- Reddit-Specific Questions
- Preparation Tips and Resources
- The Importance of Mock Interviews
- Conclusion
1. Introduction to Reddit Interview Questions
Reddit, the popular social news and discussion website, is known for its rigorous interview process. Like many tech companies, Reddit’s interviews typically cover a range of topics, including data structures and algorithms, system design, coding challenges, and behavioral questions. Understanding the types of questions asked and the skills Reddit values can significantly improve your chances of success.
Reddit’s interview process usually consists of several rounds:
- Initial phone screen
- Technical phone interview
- On-site interviews (or virtual equivalent)
- Final round with leadership
Throughout these rounds, you’ll encounter various question types, each designed to assess different aspects of your technical knowledge and problem-solving abilities.
2. Data Structures and Algorithms
Data structures and algorithms form the backbone of many Reddit interview questions. Here are some common topics and example questions:
Arrays and Strings
Question: Given an array of integers, find two numbers such that they add up to a specific target number.
Solution: This problem can be solved efficiently using a hash table.
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
Linked Lists
Question: Reverse a linked list.
Solution: This can be done iteratively or recursively. Here’s an iterative solution:
def reverse_linked_list(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
Trees and Graphs
Question: Implement a function to check if a binary tree is balanced.
Solution: We can use a depth-first approach:
def is_balanced(root):
def check_height(node):
if not node:
return 0
left = check_height(node.left)
right = check_height(node.right)
if left == -1 or right == -1 or abs(left - right) > 1:
return -1
return max(left, right) + 1
return check_height(root) != -1
Dynamic Programming
Question: Given a set of coin denominations and a target amount, find the minimum number of coins needed to make up that amount.
Solution: This classic problem can be solved using dynamic programming:
def min_coins(coins, amount):
dp = [float('inf')] * (amount + 1)
dp[0] = 0
for i in range(1, amount + 1):
for coin in coins:
if coin
3. System Design Questions
System design questions are crucial in Reddit interviews, especially for more senior positions. These questions assess your ability to design large-scale systems and make architectural decisions. Some common Reddit system design questions include:
Design Reddit’s Comment System
Key Considerations:
- Scalability to handle millions of comments
- Nested comment structure
- Real-time updates
- Voting system
- Efficient retrieval of top comments
Possible Approach:
- Use a NoSQL database like Cassandra for horizontal scalability
- Implement a tree-like data structure for nested comments
- Use a message queue (e.g., Kafka) for real-time updates
- Implement a separate voting service
- Use caching (e.g., Redis) for frequently accessed comments
Design Reddit’s Front Page Algorithm
Key Considerations:
- Ranking posts based on various factors (upvotes, time, etc.)
- Personalization for individual users
- Handling different time zones
- Preventing manipulation and spam
Possible Approach:
- Implement a scoring algorithm (e.g., a variation of the Wilson score interval)
- Use machine learning for personalization
- Store timestamps in UTC and convert to user’s local time
- Implement rate limiting and spam detection mechanisms
4. Coding Challenges
Coding challenges are a staple of Reddit interviews. These questions test your ability to implement solutions to algorithmic problems efficiently. Here are some examples:
Implement a Rate Limiter
Problem: Design a rate limiter that allows n requests per second.
Solution: We can use the token bucket algorithm:
import time
class RateLimiter:
def __init__(self, capacity, refill_rate):
self.capacity = capacity
self.refill_rate = refill_rate
self.tokens = capacity
self.last_refill = time.time()
def allow_request(self):
now = time.time()
time_passed = now - self.last_refill
self.tokens = min(self.capacity, self.tokens + time_passed * self.refill_rate)
self.last_refill = now
if self.tokens >= 1:
self.tokens -= 1
return True
return False
Implement a Trie (Prefix Tree)
Problem: Implement a trie data structure for efficient string searches.
Solution:
class TrieNode:
def __init__(self):
self.children = {}
self.is_end = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end = True
def search(self, word):
node = self.root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_end
def starts_with(self, prefix):
node = self.root
for char in prefix:
if char not in node.children:
return False
node = node.children[char]
return True
5. Behavioral Questions
Behavioral questions are an essential part of Reddit interviews. They help assess your soft skills, problem-solving approach, and cultural fit. Some common behavioral questions include:
- Tell me about a time when you had to deal with a difficult team member.
- Describe a situation where you had to make a decision with incomplete information.
- How do you handle criticism or feedback on your work?
- Can you share an example of a project you’re particularly proud of?
- How do you stay updated with the latest technologies and industry trends?
When answering behavioral questions, use the STAR method (Situation, Task, Action, Result) to structure your responses effectively.
6. Reddit-Specific Questions
To stand out in a Reddit interview, it’s crucial to demonstrate knowledge of the platform and its unique challenges. Here are some Reddit-specific questions you might encounter:
Content Moderation
Question: How would you design a system to efficiently moderate user-generated content on Reddit?
Possible Answer: A comprehensive content moderation system for Reddit could include:
- Automated filtering using machine learning models to detect spam and offensive content
- A distributed queue system to handle moderation tasks
- A tiered moderation approach involving community moderators and Reddit employees
- A reporting system for users to flag inappropriate content
- An appeals process for users whose content was removed
Subreddit Recommendation
Question: Design an algorithm to recommend subreddits to users based on their activity and interests.
Possible Approach:
- Collect user data: subscribed subreddits, upvoted posts, commenting activity
- Use collaborative filtering to find similar users
- Implement content-based filtering using topic modeling on subreddit descriptions and posts
- Combine these approaches using a hybrid recommendation system
- Continuously update recommendations based on user feedback and new activity
7. Preparation Tips and Resources
To excel in your Reddit interview, consider the following preparation tips:
- Master the fundamentals: Ensure you have a solid understanding of data structures, algorithms, and system design principles.
- Practice coding: Solve problems on platforms like LeetCode, HackerRank, or AlgoCademy to improve your coding skills.
- Study system design: Read books like “Designing Data-Intensive Applications” by Martin Kleppmann and practice designing large-scale systems.
- Mock interviews: Conduct mock interviews with friends or use platforms like Pramp to get comfortable with the interview process.
- Learn about Reddit: Familiarize yourself with Reddit’s features, challenges, and recent developments.
- Improve your communication: Practice explaining your thought process clearly and concisely.
- Prepare questions: Have thoughtful questions ready to ask your interviewers about Reddit and the role.
Useful resources for preparation:
- LeetCode’s interview preparation section
- AlgoCademy’s interactive coding tutorials and interview prep materials
- System Design Primer on GitHub
- Reddit Engineering Blog
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
8. The Importance of Mock Interviews
Mock interviews are crucial for success in your Reddit interview. They help you:
- Get comfortable with the interview format
- Practice explaining your thought process out loud
- Receive feedback on your communication and problem-solving skills
- Identify areas for improvement
- Reduce interview anxiety
Consider using platforms like AlgoCademy, which offer AI-powered mock interviews tailored to Reddit’s interview process. These can provide valuable practice and feedback to help you refine your skills.
9. Conclusion
Preparing for a Reddit interview can be challenging, but with the right approach and resources, you can significantly increase your chances of success. Focus on mastering data structures and algorithms, practicing system design, and honing your problem-solving skills. Don’t forget to familiarize yourself with Reddit’s unique challenges and culture.
Remember, the key to success in any technical interview is not just knowing the answers, but being able to communicate your thought process effectively. Practice explaining your solutions out loud, and don’t be afraid to ask clarifying questions during the interview.
By thoroughly preparing and staying calm during the interview, you’ll be well-equipped to tackle any question that comes your way. Good luck with your Reddit interview!