Top Python Coding Interview Questions: Mastering the Art of Technical Interviews
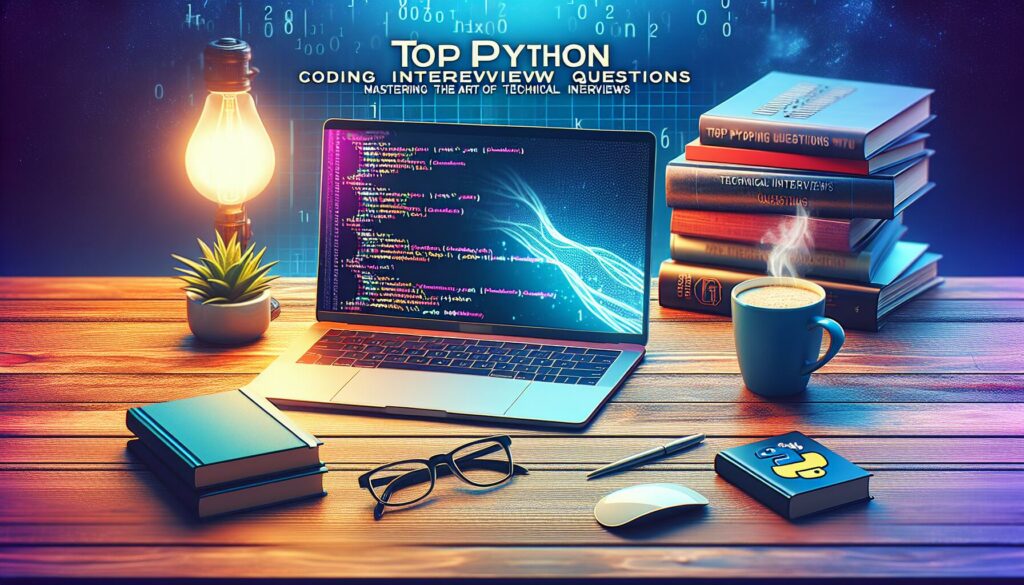
In today’s competitive tech landscape, mastering Python coding interview questions is crucial for aspiring developers aiming to land coveted positions at top companies. Whether you’re a seasoned programmer or just starting your journey in the world of coding, being well-prepared for technical interviews can make all the difference. In this comprehensive guide, we’ll explore a wide range of Python coding interview questions, from basic concepts to advanced algorithms, helping you sharpen your skills and boost your confidence for your next big interview.
Table of Contents
- Introduction to Python Coding Interviews
- Basic Python Concepts
- Data Structures in Python
- Algorithms and Problem-Solving
- Object-Oriented Programming (OOP) in Python
- Advanced Python Topics
- Common Coding Challenges
- Best Practices and Tips
- Additional Resources for Interview Preparation
- Conclusion
1. Introduction to Python Coding Interviews
Python coding interviews are designed to assess your problem-solving skills, coding proficiency, and understanding of fundamental programming concepts. These interviews often involve a combination of theoretical questions and hands-on coding challenges. To excel in these interviews, you need to have a strong grasp of Python syntax, data structures, algorithms, and best practices.
Before diving into specific questions, it’s essential to understand the typical structure of a Python coding interview:
- Technical assessment: This may include online coding tests or take-home assignments.
- Phone or video screening: A preliminary interview to assess your background and basic technical knowledge.
- On-site interviews: Multiple rounds of face-to-face or virtual interviews, often including whiteboard coding sessions.
- System design discussions: For more senior positions, you may be asked to design large-scale systems.
- Behavioral questions: To evaluate your soft skills and cultural fit.
Now, let’s explore some of the most common Python coding interview questions across various categories.
2. Basic Python Concepts
Mastering the basics is crucial for any Python developer. Here are some fundamental concepts that often come up in interviews:
Q1: What are the key features of Python?
Answer: Python’s key features include:
- Interpreted language
- Dynamically typed
- Object-oriented programming support
- High-level language with simple, easy-to-learn syntax
- Extensive standard library
- Support for multiple programming paradigms (procedural, object-oriented, functional)
- Cross-platform compatibility
- Strong community support and a wide range of third-party packages
Q2: Explain the difference between lists and tuples in Python.
Answer: The main differences between lists and tuples are:
- Mutability: Lists are mutable (can be modified after creation), while tuples are immutable (cannot be changed after creation).
- Syntax: Lists use square brackets [], tuples use parentheses ().
- Performance: Tuples are slightly faster and consume less memory than lists.
- Use cases: Lists are used for collections of similar items that may change, while tuples are used for collections of heterogeneous data that should remain constant.
Q3: What is the difference between ‘==’ and ‘is’ operators?
Answer: The ‘==’ operator compares the values of two objects, while the ‘is’ operator checks if two variables refer to the same object in memory. For example:
a = [1, 2, 3]
b = [1, 2, 3]
c = a
print(a == b) # True (same values)
print(a is b) # False (different objects)
print(a is c) # True (same object)
Q4: How do you handle exceptions in Python?
Answer: Exceptions in Python are handled using try-except blocks. Here’s a basic structure:
try:
# Code that may raise an exception
result = 10 / 0
except ZeroDivisionError:
# Handle specific exception
print("Cannot divide by zero!")
except Exception as e:
# Handle any other exception
print(f"An error occurred: {e}")
else:
# Executed if no exception occurs
print("Division successful")
finally:
# Always executed, regardless of exceptions
print("Execution completed")
3. Data Structures in Python
Understanding and implementing various data structures is crucial for efficient problem-solving. Here are some common data structure-related questions:
Q5: Implement a stack using a list in Python.
Answer: Here’s a simple implementation of a stack using a Python list:
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
return None
def peek(self):
if not self.is_empty():
return self.items[-1]
return None
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
# Usage
stack = Stack()
stack.push(1)
stack.push(2)
stack.push(3)
print(stack.pop()) # Output: 3
print(stack.peek()) # Output: 2
print(stack.size()) # Output: 2
Q6: Explain the difference between a list and a dictionary in Python.
Answer: The main differences are:
- Structure: Lists are ordered collections of items, while dictionaries are unordered collections of key-value pairs.
- Indexing: Lists use integer indices, dictionaries use keys (which can be of various immutable types).
- Use cases: Lists are used for sequential data, dictionaries for mapping relationships between keys and values.
- Performance: Dictionaries generally provide faster lookup times for large datasets.
Q7: How would you implement a queue in Python?
Answer: While you can use a list to implement a queue, it’s more efficient to use the `collections.deque` class for better performance:
from collections import deque
class Queue:
def __init__(self):
self.items = deque()
def enqueue(self, item):
self.items.append(item)
def dequeue(self):
if not self.is_empty():
return self.items.popleft()
return None
def front(self):
if not self.is_empty():
return self.items[0]
return None
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
# Usage
queue = Queue()
queue.enqueue(1)
queue.enqueue(2)
queue.enqueue(3)
print(queue.dequeue()) # Output: 1
print(queue.front()) # Output: 2
print(queue.size()) # Output: 2
4. Algorithms and Problem-Solving
Algorithmic thinking and problem-solving skills are at the core of coding interviews. Here are some common algorithm-related questions:
Q8: Implement a binary search algorithm in Python.
Answer: Here’s an implementation of binary search:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
print(binary_search(sorted_array, 7)) # Output: 3
print(binary_search(sorted_array, 10)) # Output: -1
Q9: Write a function to check if a string is a palindrome.
Answer: Here’s a simple function to check for palindromes:
def is_palindrome(s):
# Remove non-alphanumeric characters and convert to lowercase
s = ''.join(c.lower() for c in s if c.isalnum())
return s == s[::-1]
# Usage
print(is_palindrome("A man, a plan, a canal: Panama")) # Output: True
print(is_palindrome("race a car")) # Output: False
Q10: Implement a function to find the first non-repeating character in a string.
Answer: Here’s an efficient solution using a dictionary:
from collections import Counter
def first_non_repeating_char(s):
char_counts = Counter(s)
for char in s:
if char_counts[char] == 1:
return char
return None # No non-repeating character found
# Usage
print(first_non_repeating_char("leetcode")) # Output: 'l'
print(first_non_repeating_char("aabbcc")) # Output: None
5. Object-Oriented Programming (OOP) in Python
Object-Oriented Programming is a fundamental paradigm in Python. Here are some OOP-related questions you might encounter:
Q11: Explain the concept of inheritance in Python with an example.
Answer: Inheritance is a mechanism where a class can inherit attributes and methods from another class. Here’s an example:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
pass
class Dog(Animal):
def speak(self):
return f"{self.name} says Woof!"
class Cat(Animal):
def speak(self):
return f"{self.name} says Meow!"
# Usage
dog = Dog("Buddy")
cat = Cat("Whiskers")
print(dog.speak()) # Output: Buddy says Woof!
print(cat.speak()) # Output: Whiskers says Meow!
Q12: What is the difference between a class method and a static method?
Answer: The main differences are:
- Class methods receive the class as an implicit first argument, while static methods don’t receive any implicit arguments.
- Class methods can access and modify class state, while static methods can’t access the class state.
- Class methods are decorated with @classmethod, static methods with @staticmethod.
Here’s an example illustrating both:
class MyClass:
class_variable = 0
@classmethod
def class_method(cls):
cls.class_variable += 1
return cls.class_variable
@staticmethod
def static_method(x, y):
return x + y
# Usage
print(MyClass.class_method()) # Output: 1
print(MyClass.class_method()) # Output: 2
print(MyClass.static_method(5, 3)) # Output: 8
Q13: Explain the concept of encapsulation in Python.
Answer: Encapsulation is the bundling of data and the methods that operate on that data within a single unit (class). It restricts direct access to some of an object’s components, which is a means of preventing accidental interference and misuse of the methods and data. In Python, encapsulation is achieved using private and protected members:
class BankAccount:
def __init__(self, balance):
self.__balance = balance # Private attribute
def deposit(self, amount):
if amount > 0:
self.__balance += amount
return True
return False
def withdraw(self, amount):
if 0 < amount <= self.__balance:
self.__balance -= amount
return True
return False
def get_balance(self):
return self.__balance
# Usage
account = BankAccount(1000)
print(account.get_balance()) # Output: 1000
account.deposit(500)
print(account.get_balance()) # Output: 1500
account.withdraw(200)
print(account.get_balance()) # Output: 1300
# print(account.__balance) # This would raise an AttributeError
6. Advanced Python Topics
For more senior positions or specialized roles, you might encounter questions on advanced Python topics:
Q14: Explain the Global Interpreter Lock (GIL) in Python.
Answer: The Global Interpreter Lock (GIL) is a mechanism used in CPython (the reference implementation of Python) that ensures only one thread executes Python bytecode at a time. This lock is necessary because CPython’s memory management is not thread-safe. The GIL has the following implications:
- It prevents multi-core CPUs from executing multiple Python threads in parallel.
- It can become a bottleneck in CPU-bound and multi-threaded code.
- I/O-bound programs are generally not affected as the GIL is released during I/O operations.
- To achieve true parallelism, developers often use multiprocessing instead of threading for CPU-bound tasks.
Q15: What are decorators in Python? Provide an example.
Answer: Decorators are a way to modify or enhance functions or classes without directly changing their source code. They use the @decorator syntax. Here’s an example of a simple timing decorator:
import time
def timing_decorator(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"{func.__name__} took {end_time - start_time:.2f} seconds to execute.")
return result
return wrapper
@timing_decorator
def slow_function():
time.sleep(2)
print("Function executed")
# Usage
slow_function()
# Output:
# Function executed
# slow_function took 2.00 seconds to execute.
Q16: Explain the difference between deep copy and shallow copy.
Answer: In Python, assignment operations create bindings between a target and an object. For collections that are mutable or contain mutable items, there’s a difference between shallow and deep copying:
- Shallow copy: Creates a new object but references the same memory addresses as the original elements.
- Deep copy: Creates a new object and recursively copies all nested objects, creating totally independent copies.
Here’s an example illustrating the difference:
import copy
# Original list
original = [[1, 2, 3], [4, 5, 6]]
# Shallow copy
shallow = copy.copy(original)
# Deep copy
deep = copy.deepcopy(original)
# Modify the original list
original[0][0] = 9
print("Original:", original) # [[9, 2, 3], [4, 5, 6]]
print("Shallow copy:", shallow) # [[9, 2, 3], [4, 5, 6]]
print("Deep copy:", deep) # [[1, 2, 3], [4, 5, 6]]
7. Common Coding Challenges
Interviewers often present coding challenges to assess your problem-solving skills. Here are a few examples:
Q17: Write a function to reverse a linked list.
Answer: Here’s a Python implementation to reverse a linked list:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverse_linked_list(head):
prev = None
current = head
while current is not None:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
# Helper function to create a linked list from a list
def create_linked_list(arr):
if not arr:
return None
head = ListNode(arr[0])
current = head
for val in arr[1:]:
current.next = ListNode(val)
current = current.next
return head
# Helper function to convert linked list to list for printing
def linked_list_to_list(head):
result = []
current = head
while current:
result.append(current.val)
current = current.next
return result
# Usage
original_list = create_linked_list([1, 2, 3, 4, 5])
reversed_list = reverse_linked_list(original_list)
print(linked_list_to_list(reversed_list)) # Output: [5, 4, 3, 2, 1]
Q18: Implement a function to find the longest palindromic substring in a given string.
Answer: Here’s an efficient solution using dynamic programming:
def longest_palindromic_substring(s):
n = len(s)
# Create a table to store results of subproblems
dp = [[False for _ in range(n)] for _ in range(n)]
# All substrings of length 1 are palindromes
for i in range(n):
dp[i][i] = True
start = 0
max_length = 1
# Check for sub-string of length 2
for i in range(n-1):
if s[i] == s[i+1]:
dp[i][i+1] = True
start = i
max_length = 2
# Check for lengths greater than 2
for k in range(3, n+1):
for i in range(n-k+1):
j = i + k - 1
if dp[i+1][j-1] and s[i] == s[j]:
dp[i][j] = True
if k > max_length:
start = i
max_length = k
return s[start:start + max_length]
# Usage
print(longest_palindromic_substring("babad")) # Output: "bab" or "aba"
print(longest_palindromic_substring("cbbd")) # Output: "bb"
Q19: Implement a function to find the kth largest element in an unsorted array.
Answer: We can solve this efficiently using QuickSelect algorithm, which has an average time complexity of O(n):
import random
def find_kth_largest(nums, k):
def partition(left, right, pivot_index):
pivot = nums[pivot_index]
nums[pivot_index], nums[right] = nums[right], nums[pivot_index]
store_index = left
for i in range(left, right):
if nums[i] < pivot:
nums[store_index], nums[i] = nums[i], nums[store_index]
store_index += 1
nums[right], nums[store_index] = nums[store_index], nums[right]
return store_index
def select(left, right, k_smallest):
if left == right:
return nums[left]
pivot_index = random.randint(left, right)
pivot_index = partition(left, right, pivot_index)
if k_smallest == pivot_index:
return nums[k_smallest]
elif k_smallest < pivot_index:
return select(left, pivot_index - 1, k_smallest)
else:
return select(pivot_index + 1, right, k_smallest)
return select(0, len(nums) - 1, len(nums) - k)
# Usage
print(find_kth_largest([3,2,1,5,6,4], 2)) # Output: 5
print(find_kth_largest([3,2,3,1,2,4,5,5,6], 4)) # Output: 4
8. Best Practices and Tips
To excel in Python coding interviews, keep these best practices and tips in mind:
- Understand the problem: Before diving into coding, make sure you fully understand the problem. Ask clarifying questions if needed.
- Plan before coding: Outline your approach and discuss it with the interviewer before implementing.
- Write clean, readable code: Use proper indentation, meaningful variable names, and follow PEP 8 style guidelines.
- Test your code: Consider edge cases and test your solution with different inputs.
- Optimize your solution: After getting a working solution, think about ways to improve its time and space complexity.
- Communicate your thought process: Explain your reasoning as you work through the problem.
- Be familiar with Python’s built-in functions and libraries: Knowing and using them can save time and demonstrate proficiency.
- Practice regularly: Solve coding problems on platforms like LeetCode, HackerRank, or CodeSignal to build your skills.
- Learn from your mistakes: After each interview or practice session, review what you could have done better.
- Stay calm and confident: Remember that the interview process is also about assessing your problem-solving approach, not just getting the perfect solution.
9. Additional Resources for Interview Preparation
To further enhance your Python coding interview skills, consider these resources:
- Books:
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Python Crash Course” by Eric Matthes
- “Data Structures and Algorithms in Python” by Michael T. Goodrich, Roberto Tamassia, and Michael H. Goldwasser
- Online Platforms:
- LeetCode (https://leetcode.com/)
- HackerRank (https://www.hackerrank.com/)
- CodeSignal (https://codesignal.com/)
- AlgoExpert (https://www.algoexpert.io/)
- YouTube Channels:
- CS Dojo
- Back To Back SWE
- Tech With Tim
- Websites:
- GeeksforGeeks (https://www.geeksforgeeks.org/)
- Real Python (https://realpython.com/)
- Python.org Documentation (https://docs.python.org/3/)
10. Conclusion
Mastering Python coding interviews requires a combination of strong fundamental knowledge, problem-solving skills, and practical coding experience. By understanding common interview questions, practicing regularly, and staying up-to-date with Python best practices, you’ll be well-prepared to tackle even the most challenging technical interviews.
Remember that the journey to becoming a proficient Python developer is ongoing. Continuous learning and practice are key to success in the ever-evolving field of software development. As you prepare for your interviews, focus not just on memorizing solutions, but on understanding the underlying concepts and problem-solving techniques.
With dedication and the right approach, you’ll be well-equipped to showcase your Python skills and land your dream job in the tech industry. Good luck with your interview preparation!