Top Programming Languages in 2023: A Comprehensive Guide for Aspiring Developers
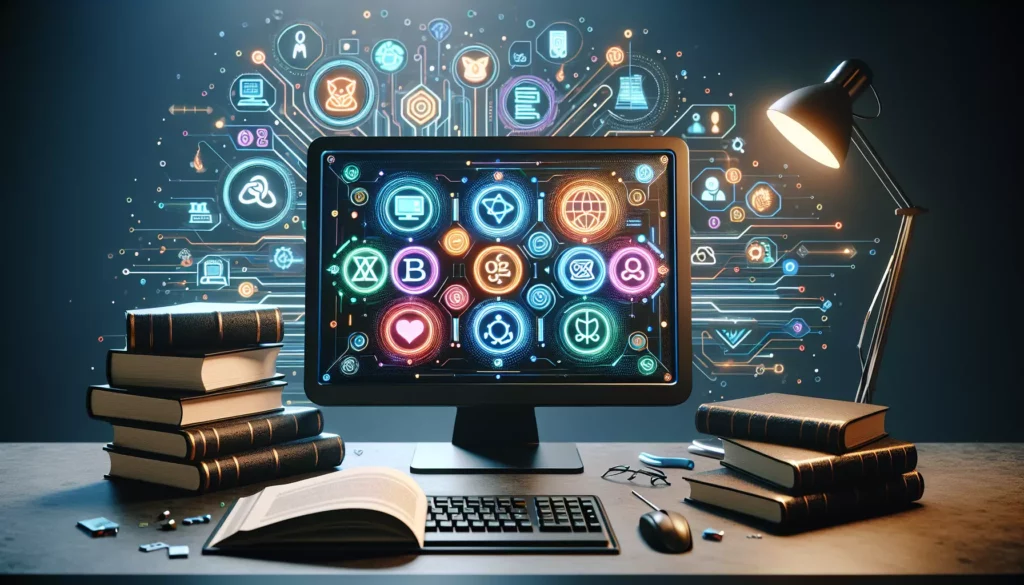
In the ever-evolving world of technology, staying up-to-date with the most in-demand programming languages is crucial for both aspiring and seasoned developers. As we navigate through 2023, the landscape of programming languages continues to shift, with some languages maintaining their stronghold while others emerge as rising stars. This comprehensive guide will explore the top programming languages of 2023, their applications, and why they’re essential for developers looking to advance their careers or break into the tech industry.
1. Python: The Versatile Powerhouse
Python continues to dominate the programming world in 2023, and for good reason. Its simplicity, readability, and versatility make it an excellent choice for beginners and experts alike.
Key Features:
- Easy to learn and read
- Extensive libraries and frameworks
- Strong community support
- Versatile applications (web development, data science, AI, machine learning)
Python’s popularity in data science and machine learning has skyrocketed in recent years. Libraries like NumPy, Pandas, and TensorFlow have made it the go-to language for AI and data analysis projects. Here’s a simple example of how you can use Python for data analysis:
import pandas as pd
import matplotlib.pyplot as plt
# Load data
data = pd.read_csv("sales_data.csv")
# Analyze data
monthly_sales = data.groupby("Month")["Sales"].sum()
# Visualize data
plt.figure(figsize=(10, 6))
monthly_sales.plot(kind="bar")
plt.title("Monthly Sales Performance")
plt.xlabel("Month")
plt.ylabel("Total Sales")
plt.show()
This simple script demonstrates how easily you can load, analyze, and visualize data using Python and its popular libraries.
2. JavaScript: The Web Development Essential
JavaScript remains an indispensable language for web development in 2023. With the rise of full-stack development and the continued popularity of front-end frameworks, JavaScript’s importance in the programming world is undeniable.
Key Features:
- Essential for front-end web development
- Powerful frameworks and libraries (React, Angular, Vue.js)
- Full-stack development capabilities with Node.js
- Asynchronous programming support
The advent of frameworks like React, Angular, and Vue.js has revolutionized front-end development, making JavaScript even more crucial for modern web applications. Here’s a simple React component example:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
export default Counter;
This example showcases how easily you can create interactive components using React and JavaScript.
3. Java: The Enterprise Standard
Despite being one of the older languages on this list, Java continues to be a cornerstone in enterprise software development. Its “write once, run anywhere” philosophy and robust ecosystem make it a reliable choice for large-scale applications.
Key Features:
- Platform independence
- Strong typing and object-oriented programming
- Extensive libraries and frameworks
- Ideal for Android app development
Java’s use in Android development ensures its continued relevance in the mobile app development sphere. Here’s a simple Java program demonstrating object-oriented programming:
public class Car {
private String brand;
private String model;
private int year;
public Car(String brand, String model, int year) {
this.brand = brand;
this.model = model;
this.year = year;
}
public void startEngine() {
System.out.println(brand + " " + model + " engine started.");
}
public static void main(String[] args) {
Car myCar = new Car("Toyota", "Corolla", 2023);
myCar.startEngine();
}
}
This example shows how Java’s object-oriented nature allows for the creation of structured and reusable code.
4. C#: The Microsoft Ecosystem Champion
C# (C-sharp) continues to be a popular choice in 2023, especially for developers working within the Microsoft ecosystem. Its versatility in developing Windows applications, game development with Unity, and web applications using .NET Core makes it a valuable language to learn.
Key Features:
- Strong integration with Microsoft technologies
- Ideal for Windows application development
- Game development with Unity
- Web development with ASP.NET Core
C#’s use in game development with Unity has contributed significantly to its popularity. Here’s a simple C# script that could be used in Unity:
using UnityEngine;
public class PlayerController : MonoBehaviour
{
public float moveSpeed = 5f;
void Update()
{
float horizontalInput = Input.GetAxis("Horizontal");
float verticalInput = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(horizontalInput, 0f, verticalInput);
transform.Translate(movement * moveSpeed * Time.deltaTime);
}
}
This script demonstrates how C# can be used to control player movement in a Unity game.
5. Go: The Efficient and Scalable Language
Go, also known as Golang, has been gaining traction in recent years and continues to be a popular choice in 2023. Developed by Google, Go is designed for efficiency, simplicity, and excellent performance in concurrent systems.
Key Features:
- Fast compilation and execution
- Built-in concurrency support
- Simplicity and ease of learning
- Excellent for cloud and network services
Go’s simplicity and efficiency make it an excellent choice for building microservices and cloud applications. Here’s a simple Go program demonstrating a concurrent operation:
package main
import (
"fmt"
"time"
)
func worker(id int, jobs <-chan int, results chan<- int) {
for j := range jobs {
fmt.Println("worker", id, "started job", j)
time.Sleep(time.Second)
fmt.Println("worker", id, "finished job", j)
results <- j * 2
}
}
func main() {
jobs := make(chan int, 100)
results := make(chan int, 100)
for w := 1; w <= 3; w++ {
go worker(w, jobs, results)
}
for j := 1; j <= 5; j++ {
jobs <- j
}
close(jobs)
for a := 1; a <= 5; a++ {
<-results
}
}
This example showcases Go’s concurrency features, demonstrating how easily you can implement parallel processing.
6. Rust: The New Systems Programming Favorite
Rust has been steadily climbing the ranks of popular programming languages, and in 2023, it’s considered one of the most loved languages by developers. Known for its focus on safety and performance, Rust is increasingly being adopted for systems programming and other performance-critical applications.
Key Features:
- Memory safety without garbage collection
- Concurrency without data races
- Zero-cost abstractions
- Interoperability with C
Rust’s emphasis on safety and performance makes it an excellent choice for systems programming. Here’s a simple Rust program demonstrating ownership and borrowing concepts:
fn main() {
let s1 = String::from("hello");
let len = calculate_length(&s1);
println!("The length of '{}' is {}.", s1, len);
}
fn calculate_length(s: &String) -> usize {
s.len()
}
This example shows how Rust’s borrowing rules allow for safe and efficient memory management without the need for garbage collection.
7. TypeScript: JavaScript with Added Safety
TypeScript, a superset of JavaScript, has been gaining popularity among developers who appreciate its static typing and object-oriented programming features. In 2023, it continues to be a preferred choice for large-scale JavaScript projects.
Key Features:
- Static typing
- Enhanced IDE support and tooling
- Object-oriented programming features
- Compatibility with JavaScript
TypeScript’s static typing can help catch errors early in the development process. Here’s an example of a TypeScript interface and class:
interface Shape {
area(): number;
}
class Circle implements Shape {
constructor(private radius: number) {}
area(): number {
return Math.PI * this.radius ** 2;
}
}
class Rectangle implements Shape {
constructor(private width: number, private height: number) {}
area(): number {
return this.width * this.height;
}
}
function printArea(shape: Shape) {
console.log(`The area is: ${shape.area()}`);
}
const circle = new Circle(5);
const rectangle = new Rectangle(4, 6);
printArea(circle); // Output: The area is: 78.53981633974483
printArea(rectangle); // Output: The area is: 24
This example demonstrates how TypeScript’s interfaces and classes can be used to create more structured and type-safe code.
8. Swift: The Apple Ecosystem Language
Swift, developed by Apple, remains the go-to language for iOS and macOS development in 2023. Its modern features and performance improvements over its predecessor, Objective-C, make it an essential language for anyone interested in Apple platform development.
Key Features:
- Fast and efficient performance
- Safe by design
- Easy to read and maintain
- Interoperability with Objective-C
Swift’s syntax is designed to be concise yet expressive. Here’s a simple Swift program demonstrating some of its features:
enum CompassPoint {
case north, south, east, west
}
struct City {
let name: String
let latitude: Double
let longitude: Double
}
func describe(city: City) -> String {
return "\(city.name) is located at (\(city.latitude), \(city.longitude))"
}
let london = City(name: "London", latitude: 51.507222, longitude: -0.1275)
print(describe(city: london))
let direction = CompassPoint.west
switch direction {
case .north:
print("Heading north")
case .south:
print("Heading south")
case .east:
print("Heading east")
case .west:
print("Heading west")
}
This example showcases Swift’s enums, structs, and string interpolation features, demonstrating its modern and expressive syntax.
9. Kotlin: The Modern Java Alternative
Kotlin has been steadily growing in popularity, especially in Android development. In 2023, it continues to be a preferred language for many developers due to its modern features and interoperability with Java.
Key Features:
- Full interoperability with Java
- Concise and expressive syntax
- Null safety
- Coroutines for asynchronous programming
Kotlin’s null safety and concise syntax make it an attractive alternative to Java. Here’s a simple Kotlin program demonstrating some of its features:
data class Person(val name: String, val age: Int)
fun main() {
val people = listOf(
Person("Alice", 29),
Person("Bob", 31),
Person("Charlie", 25)
)
val names = people.map { it.name }
println("Names: $names")
val averageAge = people.map { it.age }.average()
println("Average age: $averageAge")
val oldest = people.maxByOrNull { it.age }
oldest?.let { println("Oldest person: ${it.name}, ${it.age} years old") }
}
This example demonstrates Kotlin’s data classes, functional programming capabilities, and null safety features.
10. R: The Statistical Computing Powerhouse
While not as general-purpose as some other languages on this list, R continues to be a crucial language in 2023 for data analysis, statistical computing, and machine learning applications.
Key Features:
- Extensive statistical and graphical techniques
- Large collection of packages for various analyses
- Strong data visualization capabilities
- Active community in academia and industry
R’s powerful data analysis and visualization capabilities make it indispensable in fields like data science and bioinformatics. Here’s a simple R script demonstrating data analysis and visualization:
# Load necessary libraries
library(ggplot2)
# Create a sample dataset
data <- data.frame(
category = c("A", "B", "C", "D"),
value = c(3, 7, 9, 4)
)
# Calculate summary statistics
summary_stats <- summary(data$value)
print(summary_stats)
# Create a bar plot
ggplot(data, aes(x = category, y = value)) +
geom_bar(stat = "identity", fill = "steelblue") +
labs(title = "Value by Category", x = "Category", y = "Value") +
theme_minimal()
This example shows how R can be used to perform basic statistical analysis and create visualizations with just a few lines of code.
Conclusion: Choosing the Right Language for Your Journey
As we’ve explored the top programming languages of 2023, it’s clear that each language has its strengths and ideal use cases. The choice of which language to learn or use depends on various factors, including your career goals, the type of projects you want to work on, and the industry you’re interested in.
For those just starting their coding journey, Python remains an excellent choice due to its simplicity and versatility. JavaScript is essential for anyone interested in web development, while Java and C# are solid options for enterprise software development. Go and Rust are great choices for those interested in systems programming and high-performance applications.
Remember, the world of programming is vast and ever-changing. While mastering one language is valuable, being familiar with multiple languages can make you a more versatile and adaptable developer. As you progress in your coding journey, don’t be afraid to explore different languages and paradigms.
At AlgoCademy, we understand the importance of staying current with the latest programming trends and technologies. That’s why we offer a wide range of interactive coding tutorials and resources covering many of these top programming languages. Whether you’re a beginner looking to start your coding journey or an experienced developer aiming to expand your skill set, AlgoCademy provides the tools and guidance you need to succeed in the ever-evolving world of programming.
As you continue to develop your programming skills, remember that practice and real-world application are key. Take on projects, contribute to open-source initiatives, and never stop learning. The programming landscape of 2023 offers exciting opportunities for those willing to put in the effort to master these powerful languages.