Top PHP Interview Questions: Ace Your Next Technical Interview
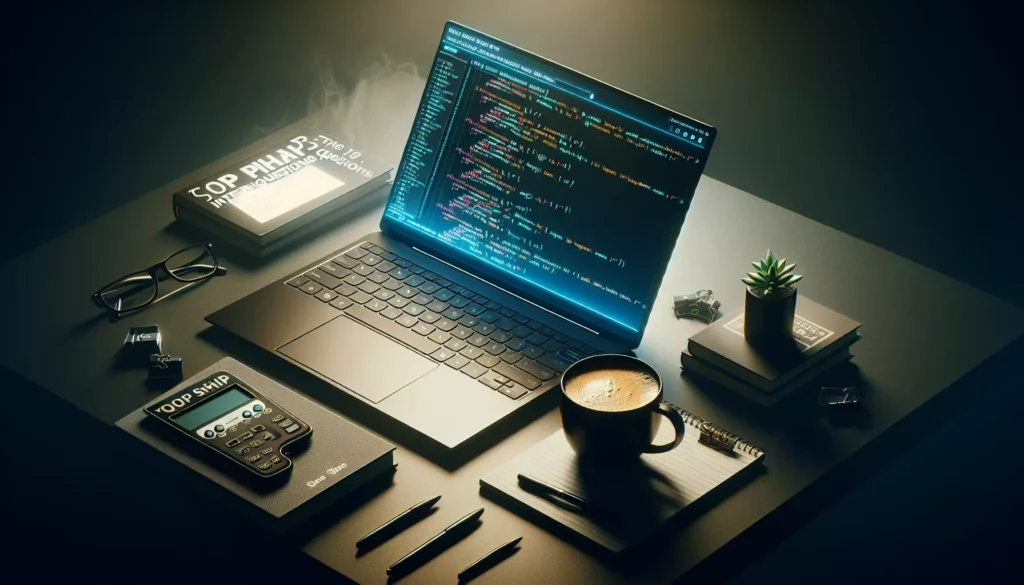
Are you preparing for a PHP developer interview? Whether you’re a seasoned pro or just starting your career in web development, being well-prepared for interview questions is crucial. In this comprehensive guide, we’ll cover a wide range of PHP interview questions, from basic concepts to advanced topics, to help you ace your next technical interview.
Table of Contents
- Basic PHP Concepts
- Object-Oriented Programming in PHP
- PHP Security
- PHP Performance Optimization
- PHP Frameworks
- Database Interaction in PHP
- PHP 7 and PHP 8 Features
- Error Handling and Debugging
- PHP Best Practices
- Practical Coding Scenarios
1. Basic PHP Concepts
Q: What is PHP, and how does it work?
A: PHP (Hypertext Preprocessor) is a server-side scripting language designed for web development. It works by embedding PHP code within HTML, which is then processed by the PHP interpreter on the web server. The interpreter executes the PHP code and generates dynamic HTML content that is sent to the client’s browser.
Q: What are the differences between echo and print in PHP?
A: Both echo and print are used to output data in PHP, but they have some differences:
- echo is slightly faster than print
- echo can take multiple parameters, while print can only take one argument
- print always returns 1, while echo has no return value
- echo is a language construct, while print is a function
Q: Explain the difference between ‘==’ and ‘===’ operators in PHP.
A: The ‘==’ operator checks for equality of values, while the ‘===’ operator checks for both equality of values and equality of types. For example:
$a = 5;
$b = "5";
if ($a == $b) {
echo "Equal using =="; // This will be printed
}
if ($a === $b) {
echo "Equal using ==="; // This will not be printed
}
Q: What are the different types of arrays in PHP?
A: PHP supports three types of arrays:
- Indexed arrays: Arrays with numeric keys
- Associative arrays: Arrays with named keys
- Multidimensional arrays: Arrays containing one or more arrays
Q: What is the difference between include and require in PHP?
A: Both include and require are used to include and evaluate files in PHP, but they differ in how they handle errors:
- include will only produce a warning (E_WARNING) if the file is not found, and the script will continue to execute
- require will produce a fatal error (E_COMPILE_ERROR) if the file is not found, and the script will stop executing
2. Object-Oriented Programming in PHP
Q: What are the main principles of Object-Oriented Programming in PHP?
A: The main principles of OOP in PHP are:
- Encapsulation: Bundling data and methods that operate on that data within a single unit (class)
- Inheritance: Allowing a class to inherit properties and methods from another class
- Polymorphism: The ability of objects of different classes to respond to the same method call in different ways
- Abstraction: Hiding complex implementation details and showing only the necessary features of an object
Q: What is the difference between an abstract class and an interface in PHP?
A: The main differences are:
- Abstract classes can have both abstract and non-abstract methods, while interfaces can only have abstract methods (prior to PHP 8.0)
- A class can implement multiple interfaces but can only extend one abstract class
- Abstract classes can have properties, while interfaces cannot (prior to PHP 8.1)
- Abstract classes can have constructors, while interfaces cannot
Q: What is method overriding in PHP?
A: Method overriding is a feature of OOP where a child class provides a specific implementation for a method that is already defined in its parent class. The overridden method in the child class must have the same name, signature, and return type as the method in the parent class.
Q: Explain the concept of namespaces in PHP.
A: Namespaces in PHP are used to organize and group related classes, interfaces, functions, and constants. They help prevent naming conflicts between different parts of a program or between different libraries. Namespaces are declared using the namespace keyword at the beginning of a file.
Q: What is the purpose of the final keyword in PHP?
A: The final keyword in PHP has two main purposes:
- When applied to a class, it prevents the class from being inherited
- When applied to a method, it prevents the method from being overridden in child classes
3. PHP Security
Q: What is SQL injection, and how can you prevent it in PHP?
A: SQL injection is a technique where malicious SQL statements are inserted into application queries to manipulate the database. To prevent SQL injection in PHP:
- Use prepared statements with parameterized queries
- Utilize PDO or MySQLi with prepared statements
- Sanitize and validate user input
- Use stored procedures
- Implement the principle of least privilege for database users
Q: How can you prevent Cross-Site Scripting (XSS) attacks in PHP?
A: To prevent XSS attacks in PHP:
- Use the htmlspecialchars() function to encode output
- Implement Content Security Policy (CSP) headers
- Validate and sanitize user input
- Use the strip_tags() function to remove HTML and PHP tags from user input
- Set the HttpOnly flag on cookies to prevent JavaScript access
Q: What is CSRF, and how can you protect against it in PHP?
A: CSRF (Cross-Site Request Forgery) is an attack that forces authenticated users to submit unwanted requests to a web application. To protect against CSRF in PHP:
- Generate and validate CSRF tokens for each form submission
- Use the SameSite attribute for cookies
- Implement proper session management
- Verify the Origin and Referer headers
Q: How can you securely store passwords in PHP?
A: To securely store passwords in PHP:
- Use the password_hash() function to create a secure hash of the password
- Store the resulting hash in the database, not the plain-text password
- Use password_verify() to compare user-provided passwords with stored hashes
- Implement password complexity requirements and enforce regular password changes
4. PHP Performance Optimization
Q: What are some ways to improve PHP performance?
A: Some ways to improve PHP performance include:
- Use a PHP accelerator like OPcache
- Implement caching mechanisms (e.g., Redis, Memcached)
- Optimize database queries and use indexes
- Minimize the use of external resources and API calls
- Use efficient coding practices and avoid unnecessary loops
- Implement proper error logging and debugging
- Utilize asynchronous processing for time-consuming tasks
Q: What is the difference between include_once and require_once?
A: Both include_once and require_once are used to include a file only once during script execution, but they differ in how they handle errors:
- include_once will produce a warning if the file is not found, and the script will continue to execute
- require_once will produce a fatal error if the file is not found, and the script will stop executing
Q: How can you optimize database queries in PHP?
A: To optimize database queries in PHP:
- Use proper indexing on frequently queried columns
- Avoid using SELECT * and only retrieve necessary columns
- Use LIMIT to restrict the number of rows returned
- Implement caching for frequently accessed data
- Use prepared statements to improve query execution speed
- Optimize JOIN operations and avoid unnecessary subqueries
5. PHP Frameworks
Q: What are some popular PHP frameworks, and what are their advantages?
A: Some popular PHP frameworks include:
- Laravel: Known for its elegant syntax and robust features
- Symfony: Modular components and high performance
- CodeIgniter: Lightweight and easy to learn
- Yii: High-performance framework with extensive caching support
- Zend Framework: Enterprise-level framework with a modular architecture
Advantages of using PHP frameworks include:
- Faster development time
- Built-in security features
- Standardized coding practices
- Easier maintenance and scalability
- Access to a large community and ecosystem of plugins
Q: What is the MVC architecture, and how is it implemented in PHP frameworks?
A: MVC (Model-View-Controller) is an architectural pattern that separates an application into three main components:
- Model: Manages data and business logic
- View: Handles the presentation layer
- Controller: Acts as an intermediary between Model and View, processing user input and managing application flow
PHP frameworks implement MVC by providing a structured directory layout and base classes for each component. This separation of concerns allows for easier maintenance, testing, and scalability of applications.
6. Database Interaction in PHP
Q: What are the different ways to connect to a MySQL database in PHP?
A: There are three main ways to connect to a MySQL database in PHP:
- MySQLi (MySQL Improved) extension
- PDO (PHP Data Objects)
- MySQL extension (deprecated since PHP 5.5)
It’s recommended to use either MySQLi or PDO for modern PHP applications due to their improved security features and object-oriented interfaces.
Q: What is the difference between MySQLi and PDO?
A: The main differences between MySQLi and PDO are:
- PDO supports multiple database systems, while MySQLi is specific to MySQL
- PDO uses a unified API for different databases, making it easier to switch between database systems
- MySQLi provides both procedural and object-oriented interfaces, while PDO is fully object-oriented
- PDO generally offers more features and flexibility compared to MySQLi
Q: How can you prevent SQL injection when using PDO?
A: To prevent SQL injection when using PDO:
- Use prepared statements with parameterized queries
- Bind parameters using the bindParam() or bindValue() methods
- Set the PDO error mode to throw exceptions (PDO::ERRMODE_EXCEPTION)
- Avoid using string concatenation to build SQL queries
7. PHP 7 and PHP 8 Features
Q: What are some key features introduced in PHP 7?
A: Some key features introduced in PHP 7 include:
- Improved performance (up to 2x faster than PHP 5.6)
- Scalar type declarations
- Return type declarations
- Null coalescing operator (??)
- Spaceship operator (<=>)
- Anonymous classes
- Constant arrays using define()
Q: What are some important features introduced in PHP 8?
A: Some important features introduced in PHP 8 include:
- Just-In-Time (JIT) compilation
- Union types
- Named arguments
- Attributes
- Match expression
- Nullsafe operator (?.)
- Constructor property promotion
Q: Explain the concept of type hinting in PHP.
A: Type hinting in PHP allows you to specify the expected data type of function parameters and return values. This helps catch type-related errors early and improves code readability. Type hinting can be used for:
- Scalar types (int, float, string, bool)
- Array and callable types
- Class and interface names
- Self and parent for late static bindings
- Union types (PHP 8+)
8. Error Handling and Debugging
Q: What are the different types of errors in PHP?
A: PHP has several types of errors:
- Parse errors: Syntax errors that prevent the script from running
- Fatal errors: Errors that cause the script to terminate
- Warning errors: Non-fatal errors that don’t stop script execution
- Notice errors: Runtime notifications for non-critical issues
- Deprecated errors: Warnings about code that will not work in future versions
Q: How can you handle exceptions in PHP?
A: Exceptions in PHP can be handled using try-catch blocks:
try {
// Code that may throw an exception
throw new Exception("An error occurred");
} catch (Exception $e) {
// Handle the exception
echo "Caught exception: " . $e->getMessage();
} finally {
// Optional block that always executes
echo "This will always execute";
}
Q: What is the purpose of the error_reporting() function in PHP?
A: The error_reporting() function in PHP is used to set which PHP errors are reported. It allows you to control the level of error reporting during development and production. For example:
// Report all errors
error_reporting(E_ALL);
// Report all errors except E_NOTICE
error_reporting(E_ALL & ~E_NOTICE);
// Turn off all error reporting
error_reporting(0);
9. PHP Best Practices
Q: What are some PHP coding best practices?
A: Some PHP coding best practices include:
- Follow a consistent coding style (e.g., PSR-1 and PSR-12)
- Use meaningful variable and function names
- Comment your code appropriately
- Implement proper error handling and logging
- Use version control (e.g., Git)
- Write unit tests for your code
- Keep your code DRY (Don’t Repeat Yourself)
- Use dependency injection to manage object creation and lifetime
- Implement proper security measures (e.g., input validation, output encoding)
Q: What is autoloading in PHP, and why is it useful?
A: Autoloading in PHP is a mechanism that automatically loads class files when they are needed, without requiring explicit include or require statements. It’s useful because:
- It reduces the number of include/require statements in your code
- It improves performance by only loading classes when they are actually used
- It simplifies code organization and maintenance
PHP provides the spl_autoload_register() function to register autoloader functions.
Q: What are PHP design patterns, and can you give an example?
A: PHP design patterns are reusable solutions to common problems in software design. They provide a structured approach to solving design issues and can improve code maintainability and scalability. An example of a design pattern is the Singleton pattern:
class Singleton {
private static $instance = null;
private function __construct() {}
public static function getInstance() {
if (self::$instance === null) {
self::$instance = new self();
}
return self::$instance;
}
// Prevent cloning of the instance
private function __clone() {}
// Prevent unserializing of the instance
public function __wakeup() {}
}
// Usage
$singleton = Singleton::getInstance();
10. Practical Coding Scenarios
Q: Write a PHP function to reverse a string without using the built-in strrev() function.
A: Here’s a PHP function to reverse a string without using strrev():
function reverseString($str) {
$length = strlen($str);
$reversed = '';
for ($i = $length - 1; $i >= 0; $i--) {
$reversed .= $str[$i];
}
return $reversed;
}
// Usage
$original = "Hello, World!";
$reversed = reverseString($original);
echo $reversed; // Output: !dlroW ,olleH
Q: Implement a simple API endpoint in PHP that returns JSON data.
A: Here’s a basic example of a PHP script that creates a simple API endpoint returning JSON data:
<?php
// Set the content type to JSON
header('Content-Type: application/json');
// Sample data
$data = [
'id' => 1,
'name' => 'John Doe',
'email' => 'john@example.com'
];
// Encode the data as JSON and output it
echo json_encode($data);
Q: Write a PHP function to check if a given string is a palindrome.
A: Here’s a PHP function to check if a string is a palindrome:
function isPalindrome($str) {
// Remove non-alphanumeric characters and convert to lowercase
$str = preg_replace('/[^a-zA-Z0-9]/', '', strtolower($str));
// Compare the string with its reverse
return $str === strrev($str);
}
// Usage
$testString1 = "A man, a plan, a canal: Panama";
$testString2 = "Hello, World!";
echo isPalindrome($testString1) ? "Is a palindrome" : "Not a palindrome"; // Output: Is a palindrome
echo "\n";
echo isPalindrome($testString2) ? "Is a palindrome" : "Not a palindrome"; // Output: Not a palindrome
These practical coding scenarios demonstrate how to apply PHP concepts in real-world situations, which is often a crucial part of technical interviews.
Conclusion
Preparing for a PHP interview involves understanding a wide range of topics, from basic syntax to advanced concepts like security, performance optimization, and design patterns. By familiarizing yourself with these common PHP interview questions and practicing your coding skills, you’ll be well-prepared to showcase your expertise and land your dream PHP developer job.
Remember that technical interviews often involve more than just answering questions. Be prepared to discuss your past projects, explain your problem-solving approach, and demonstrate your ability to write clean, efficient code. Good luck with your interview!