Top Netflix Interview Questions: Mastering the Technical Challenge
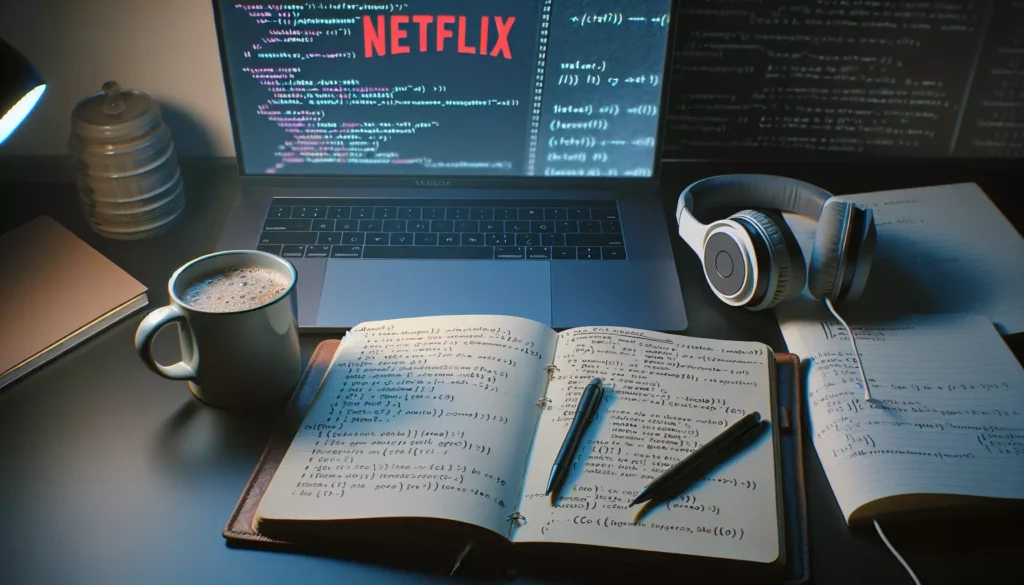
Netflix, a streaming giant that revolutionized how we consume entertainment, is not just known for its vast library of content but also for its cutting-edge technology. As one of the most sought-after employers in the tech industry, Netflix’s interview process is notoriously challenging, designed to identify top-tier talent capable of pushing the boundaries of streaming technology. In this comprehensive guide, we’ll explore some of the most common Netflix interview questions, providing insights into what the company looks for in potential hires and how you can prepare to ace your interview.
Understanding Netflix’s Interview Process
Before diving into specific questions, it’s crucial to understand Netflix’s unique interview approach. The company values cultural fit as much as technical expertise, emphasizing their famous “culture deck” principles. Netflix looks for candidates who embody qualities such as judgment, communication, impact, curiosity, innovation, courage, passion, honesty, and selflessness.
The interview process typically includes:
- Initial phone screen
- Technical phone interview
- On-site interviews (or virtual equivalent)
- Coding challenges
- System design discussions
- Behavioral interviews
Now, let’s explore some of the common technical questions you might encounter during a Netflix interview.
1. Algorithmic and Data Structure Questions
Question: Implement a Least Recently Used (LRU) Cache
This is a classic problem that tests your understanding of data structures and efficient algorithm design. Netflix might use LRU caches in various parts of their system to optimize performance.
Here’s a sample implementation in Python:
from collections import OrderedDict
class LRUCache:
def __init__(self, capacity: int):
self.cache = OrderedDict()
self.capacity = capacity
def get(self, key: int) -> int:
if key not in self.cache:
return -1
self.cache.move_to_end(key)
return self.cache[key]
def put(self, key: int, value: int) -> None:
if key in self.cache:
self.cache.move_to_end(key)
self.cache[key] = value
if len(self.cache) > self.capacity:
self.cache.popitem(last=False)
Question: Design a Movie Recommendation System
This question tests your ability to think about large-scale systems and recommendation algorithms, which are crucial for Netflix’s core business.
Key points to discuss:
- Collaborative filtering techniques
- Content-based filtering
- Hybrid approaches
- Handling cold start problems
- Scalability considerations
2. System Design Questions
Question: Design Netflix’s Video Streaming System
This question evaluates your ability to architect complex, distributed systems. You should discuss:
- Content Delivery Networks (CDNs)
- Video encoding and transcoding
- Adaptive bitrate streaming
- Load balancing
- Caching strategies
- Fault tolerance and redundancy
Here’s a high-level diagram you might draw:
[User Devices] <--> [CDN] <--> [Load Balancer] <--> [API Servers] <--> [Databases]
^
|
v
[Encoding Servers]
^
|
v
[Storage Systems]
Question: Design a System to Handle Millions of Simultaneous Video Streams
This question tests your knowledge of scalable architectures. Key points to address:
- Microservices architecture
- Horizontal scaling
- Database sharding
- Caching layers (e.g., Redis)
- Message queues for asynchronous processing
- Monitoring and auto-scaling
3. Coding Implementation Questions
Question: Implement a Rate Limiter
Rate limiting is crucial for maintaining system stability. Here’s a simple token bucket implementation in Python:
import time
class RateLimiter:
def __init__(self, capacity, refill_rate):
self.capacity = capacity
self.tokens = capacity
self.refill_rate = refill_rate
self.last_refill = time.time()
def allow_request(self):
now = time.time()
time_passed = now - self.last_refill
self.tokens = min(self.capacity, self.tokens + time_passed * self.refill_rate)
self.last_refill = now
if self.tokens >= 1:
self.tokens -= 1
return True
return False
Question: Implement a Concurrent Download Manager
This question tests your understanding of concurrency and parallel processing. Here’s a basic implementation using Python’s threading module:
import threading
import requests
class DownloadManager:
def __init__(self, urls):
self.urls = urls
self.results = {}
def download(self, url):
response = requests.get(url)
self.results[url] = response.content
def concurrent_download(self):
threads = []
for url in self.urls:
thread = threading.Thread(target=self.download, args=(url,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
return self.results
4. Database and SQL Questions
Question: Design a Schema for Netflix’s Movie and User Data
This question tests your ability to design efficient database schemas. You might create tables like:
CREATE TABLE Users (
user_id INT PRIMARY KEY,
username VARCHAR(50),
email VARCHAR(100),
signup_date DATE
);
CREATE TABLE Movies (
movie_id INT PRIMARY KEY,
title VARCHAR(100),
release_year INT,
genre VARCHAR(50),
duration INT
);
CREATE TABLE UserWatchHistory (
id INT PRIMARY KEY,
user_id INT,
movie_id INT,
watch_date DATE,
FOREIGN KEY (user_id) REFERENCES Users(user_id),
FOREIGN KEY (movie_id) REFERENCES Movies(movie_id)
);
Question: Write a SQL Query to Find the Top 10 Most Watched Movies
This tests your SQL skills. Here’s a sample query:
SELECT m.title, COUNT(*) as watch_count
FROM Movies m
JOIN UserWatchHistory uwh ON m.movie_id = uwh.movie_id
GROUP BY m.movie_id, m.title
ORDER BY watch_count DESC
LIMIT 10;
5. Behavioral Questions
Netflix places a strong emphasis on cultural fit. Some common behavioral questions include:
- Describe a time when you had to make a difficult decision with incomplete information.
- How do you stay updated with the latest technology trends?
- Tell me about a time when you disagreed with a team member. How did you resolve it?
- Describe a project where you had to learn a new technology quickly.
- How do you handle feedback, both positive and constructive?
6. Netflix-Specific Technical Questions
Question: Explain How Netflix Might Optimize Video Streaming Quality
This question tests your understanding of video streaming technologies. Key points to discuss:
- Adaptive Bitrate Streaming (ABR)
- MPEG-DASH and HLS protocols
- Video compression techniques (e.g., H.264, HEVC)
- Per-title encoding
- Quality of Experience (QoE) metrics
Question: How Would You Implement A/B Testing for New Features?
This tests your understanding of experimentation in software development. Discuss:
- Feature flagging
- User segmentation
- Statistical significance
- Metrics for success (e.g., engagement, retention)
- Gradual rollout strategies
7. Open-Ended Problem-Solving Questions
Question: How Would You Detect and Prevent Account Sharing?
This question tests your ability to think creatively about complex problems. Consider:
- IP address tracking
- Device fingerprinting
- Usage pattern analysis
- Machine learning for anomaly detection
- Balancing security with user experience
Question: Design a System to Handle Global Outages
This tests your knowledge of fault-tolerant systems. Discuss:
- Multi-region deployments
- Chaos engineering principles
- Circuit breakers and fallback mechanisms
- Real-time monitoring and alerting
- Automated recovery processes
Preparing for Your Netflix Interview
To maximize your chances of success in a Netflix interview:
- Study the Netflix Culture Deck: Understand the company’s values and how they align with your own.
- Practice System Design: Be prepared to discuss large-scale, distributed systems.
- Brush Up on Algorithms and Data Structures: Leetcode and HackerRank are great resources for practice.
- Stay Current with Technology: Netflix is at the forefront of streaming technology, so keep up with the latest trends.
- Prepare Concrete Examples: For behavioral questions, have specific situations ready to discuss.
- Understand Netflix’s Technical Stack: Familiarize yourself with technologies like Java, Python, Node.js, and AWS.
Conclusion
Preparing for a Netflix interview can be challenging, but it’s also an opportunity to showcase your skills and passion for technology. The company looks for individuals who can not only solve complex technical problems but also align with their unique culture of freedom and responsibility.
Remember, the key to success is not just about having the right answers, but also demonstrating your problem-solving process, your ability to communicate complex ideas, and your enthusiasm for tackling challenging problems in the world of streaming technology.
By thoroughly preparing for these types of questions and understanding Netflix’s culture and technical needs, you’ll be well-equipped to make a strong impression in your interview. Good luck!