Top Microsoft Interview Questions: Mastering Technical Interviews for Success
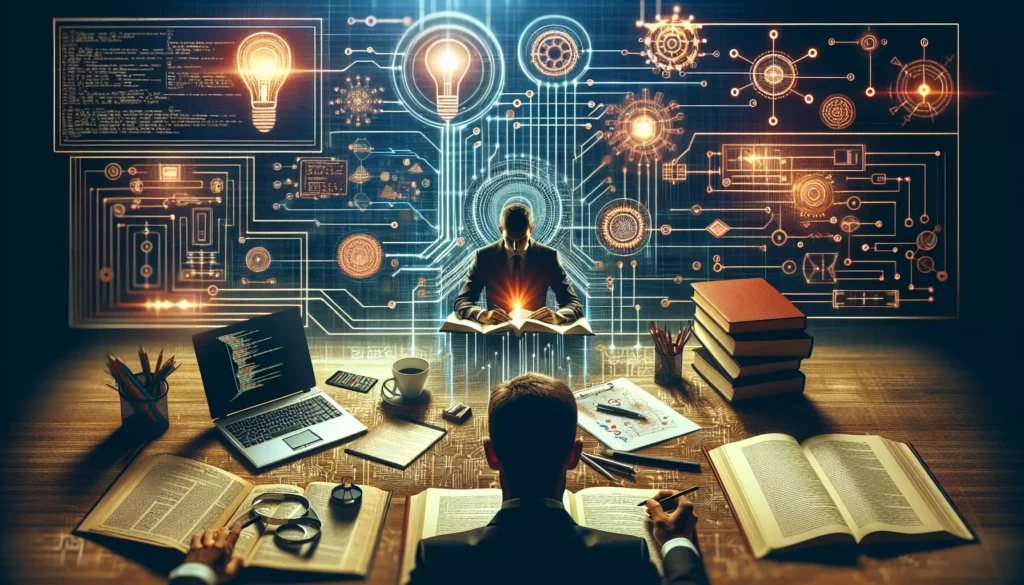
Are you gearing up for a technical interview at Microsoft? You’re in the right place! Microsoft, one of the world’s leading technology companies, is known for its rigorous interview process. This comprehensive guide will walk you through common Microsoft interview questions, provide insights into their interview structure, and offer tips to help you succeed. Whether you’re a seasoned professional or a fresh graduate, this article will equip you with the knowledge and strategies needed to ace your Microsoft interview.
Table of Contents
- Understanding Microsoft Interviews
- Technical Questions
- Behavioral Questions
- Coding Challenges
- System Design Questions
- Microsoft-Specific Questions
- Interview Tips
- Preparation Strategies
- Conclusion
1. Understanding Microsoft Interviews
Before diving into specific questions, it’s crucial to understand the structure of Microsoft interviews. Typically, the process consists of multiple rounds:
- Initial Screening: This may be a phone interview or an online assessment to evaluate basic skills.
- Technical Interviews: Usually 3-4 rounds focusing on coding, algorithms, and problem-solving skills.
- Behavioral Interview: To assess your soft skills and cultural fit.
- System Design Interview: For more experienced candidates, focusing on large-scale system architecture.
- “As Appropriate” Interview: An additional round if needed, often with a senior team member or manager.
Now, let’s explore the types of questions you might encounter in each of these stages.
2. Technical Questions
Technical questions form the core of Microsoft interviews. They assess your problem-solving skills, coding ability, and understanding of fundamental computer science concepts. Here are some common areas and example questions:
Data Structures and Algorithms
- Binary Trees: “Implement a function to check if a binary tree is balanced.”
- Linked Lists: “Reverse a linked list in-place.”
- Arrays and Strings: “Find the longest palindromic substring in a given string.”
- Stacks and Queues: “Implement a queue using two stacks.”
- Graphs: “Implement Dijkstra’s algorithm for finding the shortest path in a graph.”
Example: Reversing a Linked List
Here’s a Python implementation to reverse a linked list in-place:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverseLinkedList(head):
prev = None
current = head
while current is not None:
next_temp = current.next
current.next = prev
prev = current
current = next_temp
return prev
Time and Space Complexity
Be prepared to analyze the time and space complexity of your solutions. For example:
Q: “What’s the time and space complexity of the reverseLinkedList function?”
A: The time complexity is O(n), where n is the number of nodes in the linked list, as we traverse the list once. The space complexity is O(1) because we only use a constant amount of extra space regardless of the input size.
Optimization Problems
- “Given an array of integers, find two numbers such that they add up to a specific target number.”
- “Implement an LRU (Least Recently Used) cache.”
- “Design a data structure that supports insert, delete, getRandom in O(1) time.”
3. Behavioral Questions
Microsoft places a high value on teamwork, leadership, and problem-solving abilities. Behavioral questions help assess these qualities. Here are some examples:
- “Describe a time when you had to work with a difficult team member. How did you handle it?”
- “Tell me about a project where you had to meet a tight deadline. How did you manage your time?”
- “Can you share an example of when you had to explain a complex technical concept to a non-technical person?”
- “Describe a situation where you failed. What did you learn from it?”
- “How do you stay updated with the latest technology trends?”
When answering behavioral questions, use the STAR method:
- Situation: Set the context for your story.
- Task: Describe your responsibility in that situation.
- Action: Explain the steps you took to address it.
- Result: Share the outcomes of your actions.
4. Coding Challenges
Microsoft often includes live coding challenges during the interview process. These assess your ability to write clean, efficient code under pressure. Here are some tips and example challenges:
Tips for Coding Challenges
- Think out loud: Explain your thought process as you code.
- Start with a brute force solution, then optimize.
- Consider edge cases and handle them appropriately.
- Write clean, readable code with proper naming conventions.
- Test your code with sample inputs.
Example Coding Challenge: Merge Intervals
Problem: Given an array of intervals where intervals[i] = [starti, endi], merge all overlapping intervals and return an array of the non-overlapping intervals that cover all the intervals in the input.
Here’s a Python solution:
def mergeIntervals(intervals):
if not intervals:
return []
# Sort intervals based on start time
intervals.sort(key=lambda x: x[0])
merged = [intervals[0]]
for interval in intervals[1:]:
# If current interval overlaps with the previous, merge them
if interval[0] <= merged[-1][1]:
merged[-1][1] = max(merged[-1][1], interval[1])
else:
merged.append(interval)
return merged
# Test the function
intervals = [[1,3],[2,6],[8,10],[15,18]]
print(mergeIntervals(intervals)) # Output: [[1,6],[8,10],[15,18]]
5. System Design Questions
For more senior positions, Microsoft often includes system design questions. These assess your ability to design large-scale systems and make architectural decisions. Some example questions include:
- “Design a URL shortening service like bit.ly.”
- “How would you design Twitter’s trending topics feature?”
- “Design a distributed key-value store.”
- “How would you implement a real-time chat system?”
- “Design a system for a parking garage.”
Approaching System Design Questions
- Clarify Requirements: Ask questions to understand the scope and constraints.
- Define System Interface: Outline the API endpoints or user interactions.
- Estimate Scale: Consider factors like data volume, traffic, and storage requirements.
- Design Core Components: Sketch out the main system components and their interactions.
- Identify Key Issues: Address potential bottlenecks, single points of failure, etc.
- Scale the Design: Discuss how to handle growth and improve performance.
Example: Designing a URL Shortener
Here’s a high-level design for a URL shortening service:
- API:
- POST /shorten – Create a short URL
- GET /{short_code} – Redirect to the original URL
- Components:
- Load Balancer
- Application Servers
- Database (NoSQL for quick lookups)
- Cache (e.g., Redis for frequently accessed URLs)
- URL Encoding: Use base62 encoding (a-z, A-Z, 0-9) for short codes
- Data Model:
- short_code (primary key)
- original_url
- creation_date
- expiration_date (optional)
- Scalability: Implement sharding based on the first character of the short code
6. Microsoft-Specific Questions
Microsoft interviewers often ask questions related to their products, technologies, or company culture. Being familiar with these can give you an edge:
- “Which Microsoft product do you use the most? How would you improve it?”
- “How do you see cloud computing (Azure) impacting the future of software development?”
- “What do you think about Microsoft’s recent focus on open-source technologies?”
- “How would you explain Microsoft’s ‘Growth Mindset’ philosophy?”
- “What excites you most about working at Microsoft?”
7. Interview Tips
To increase your chances of success in a Microsoft interview, consider these tips:
- Practice Whiteboarding: Many interviews involve coding on a whiteboard or shared document. Practice this to get comfortable.
- Communicate Clearly: Explain your thought process throughout the interview. Microsoft values clear communication.
- Ask Questions: Don’t hesitate to ask for clarification or additional information about the problems presented.
- Show Enthusiasm: Demonstrate your passion for technology and your interest in Microsoft’s mission.
- Be Prepared for Follow-ups: Interviewers often ask follow-up questions to test the depth of your knowledge.
- Discuss Trade-offs: When proposing solutions, discuss the pros and cons of different approaches.
- Stay Calm: If you get stuck, take a deep breath and approach the problem step-by-step.
8. Preparation Strategies
To effectively prepare for your Microsoft interview, consider the following strategies:
- Review Computer Science Fundamentals: Brush up on data structures, algorithms, and system design principles.
- Practice Coding: Use platforms like LeetCode, HackerRank, or CodeSignal to practice coding problems.
- Mock Interviews: Conduct mock interviews with friends or use services like Pramp for realistic practice.
- Study Microsoft’s Products and Culture: Familiarize yourself with Microsoft’s products, recent news, and company values.
- Prepare Your Own Questions: Have thoughtful questions ready to ask your interviewers about the role and company.
- Review Your Past Projects: Be ready to discuss your previous work in detail, highlighting your contributions and learnings.
Recommended Resources
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “System Design Interview” by Alex Xu
- Microsoft’s official career site and blog posts about their interview process
- Online courses on data structures, algorithms, and system design
9. Conclusion
Preparing for a Microsoft interview can be challenging, but with the right approach and mindset, it’s an achievable goal. Remember that the interview process is not just about testing your technical skills, but also about assessing your problem-solving approach, communication abilities, and cultural fit.
Focus on strengthening your foundational knowledge, practicing coding problems, and developing your system design skills. Don’t forget to showcase your passion for technology and your alignment with Microsoft’s mission and values.
Lastly, remember that interviewing is a skill that improves with practice. Each interview, regardless of the outcome, is an opportunity to learn and grow. Stay persistent, keep learning, and approach each interview with confidence and enthusiasm.
Good luck with your Microsoft interview! With thorough preparation and the right mindset, you’ll be well-equipped to showcase your skills and land that dream job at one of the world’s leading technology companies.