Top K Elements: Mastering Heap and Quickselect Algorithms
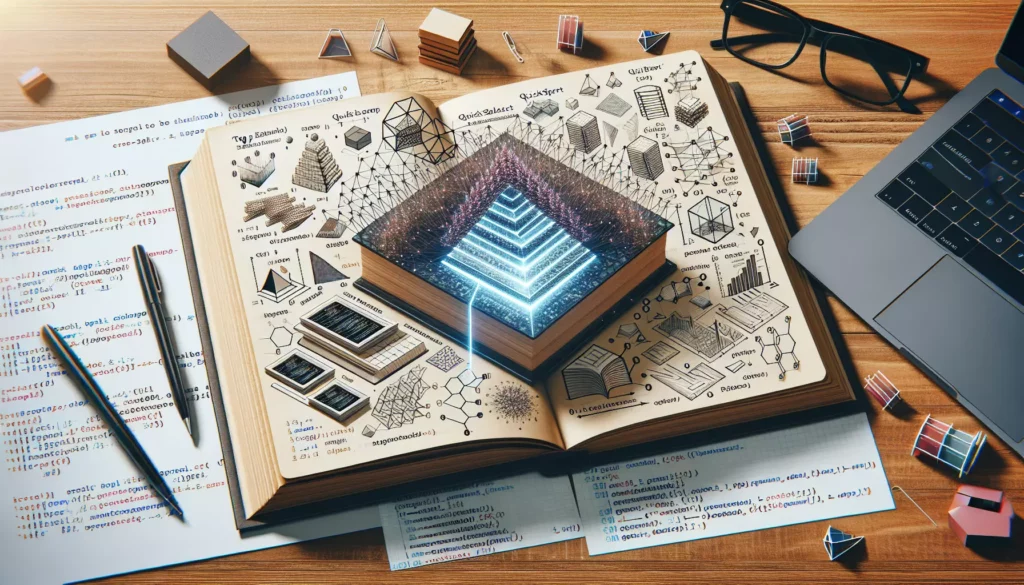
In the world of competitive programming and technical interviews, particularly for prestigious tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), certain algorithmic problems consistently appear. One such problem is finding the top K elements from a given set of data. This problem not only tests a programmer’s ability to work with data structures but also challenges their understanding of efficient algorithms. In this comprehensive guide, we’ll dive deep into two powerful approaches to solve the Top K Elements problem: using Heap and Quickselect algorithms.
Understanding the Top K Elements Problem
Before we delve into the solutions, let’s clearly define the problem at hand. The Top K Elements problem typically asks us to find the K largest (or smallest) elements from an unsorted array or stream of data. This could be applied in various real-world scenarios, such as:
- Finding the top K most frequent words in a document
- Identifying the K closest points to a given location
- Determining the K most popular items in an e-commerce platform
- Selecting the K highest-scoring students in a class
The challenge lies in solving this problem efficiently, especially when dealing with large datasets. A naive approach of sorting the entire array and then selecting the top K elements would work, but it’s not the most efficient solution, particularly for large values of N (the size of the input) and small values of K.
Solution 1: Using Heap Data Structure
A Heap is a specialized tree-based data structure that satisfies the heap property. In a max-heap, for any given node, the value of the node is greater than or equal to the values of its children. Conversely, in a min-heap, the value of the node is less than or equal to the values of its children.
For the Top K Elements problem, we can use a min-heap to efficiently keep track of the K largest elements. Here’s how the algorithm works:
- Create a min-heap of size K
- Iterate through the array:
- If the heap size is less than K, add the current element to the heap
- If the heap size is K and the current element is larger than the root of the heap, remove the root and add the current element
Let’s implement this solution in Python:
import heapq
def find_top_k_elements(arr, k):
if k <= 0:
return []
min_heap = []
for num in arr:
if len(min_heap) < k:
heapq.heappush(min_heap, num)
elif num > min_heap[0]:
heapq.heappop(min_heap)
heapq.heappush(min_heap, num)
return min_heap
# Example usage
arr = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
k = 3
result = find_top_k_elements(arr, k)
print(f"The top {k} elements are: {result}")
In this implementation, we use Python’s heapq module, which provides an implementation of the heap queue algorithm. The time complexity of this solution is O(N log K), where N is the number of elements in the array. This is because we perform N operations, each potentially involving a heap operation that takes log K time.
Advantages of the Heap Approach
- Efficient for large datasets: It doesn’t require sorting the entire array
- Works well for streaming data: Can process elements one by one without needing the entire dataset in memory
- Flexible: Can be easily modified to find the K smallest elements by using a max-heap instead
Disadvantages of the Heap Approach
- Requires additional space: O(K) extra space is needed for the heap
- Not the most efficient for small K: For very small K, other approaches might be faster
Solution 2: Using Quickselect Algorithm
The Quickselect algorithm is a selection algorithm to find the kth smallest element in an unordered list. It is related to the Quicksort sorting algorithm and uses a similar partitioning strategy. We can adapt this algorithm to solve the Top K Elements problem.
Here’s how the Quickselect algorithm works for finding the Kth largest element:
- Choose a pivot element from the array
- Partition the array around the pivot (elements greater than pivot on one side, smaller on the other)
- If the pivot is in the Kth position, we’ve found our element
- If the Kth position is on the left of the pivot, recurse on the left subarray
- If the Kth position is on the right of the pivot, recurse on the right subarray
To find the Top K elements, we can use Quickselect to find the Kth largest element, and then all elements greater than or equal to this element will be our answer.
Let’s implement this solution in Python:
import random
def quickselect(arr, k):
if not 1 <= k <= len(arr):
return None
def partition(left, right, pivot_idx):
pivot = arr[pivot_idx]
arr[pivot_idx], arr[right] = arr[right], arr[pivot_idx]
store_idx = left
for i in range(left, right):
if arr[i] > pivot:
arr[store_idx], arr[i] = arr[i], arr[store_idx]
store_idx += 1
arr[right], arr[store_idx] = arr[store_idx], arr[right]
return store_idx
def select(left, right):
if left == right:
return arr[left]
pivot_idx = random.randint(left, right)
pivot_idx = partition(left, right, pivot_idx)
if k == pivot_idx + 1:
return arr[pivot_idx]
elif k < pivot_idx + 1:
return select(left, pivot_idx - 1)
else:
return select(pivot_idx + 1, right)
return select(0, len(arr) - 1)
def find_top_k_elements_quickselect(arr, k):
if k <= 0:
return []
kth_largest = quickselect(arr, k)
return [num for num in arr if num >= kth_largest]
# Example usage
arr = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
k = 3
result = find_top_k_elements_quickselect(arr, k)
print(f"The top {k} elements are: {result}")
The time complexity of Quickselect is O(N) on average, making it very efficient. However, in the worst case (when we consistently choose bad pivots), it can degrade to O(N^2).
Advantages of the Quickselect Approach
- Very efficient on average: O(N) time complexity
- In-place algorithm: Doesn’t require additional space (except for the recursion stack)
- Can be faster than the heap approach for smaller K
Disadvantages of the Quickselect Approach
- Worst-case time complexity is O(N^2)
- Not suitable for streaming data: Requires the entire dataset to be available
- Modifies the original array
Comparing Heap and Quickselect Approaches
Both the Heap and Quickselect approaches have their strengths and are suitable for different scenarios. Let’s compare them across various dimensions:
Time Complexity
- Heap: O(N log K)
- Quickselect: O(N) on average, O(N^2) worst case
For large N and small K, the Heap approach is generally more consistent. For smaller datasets or when K is close to N, Quickselect might be faster.
Space Complexity
- Heap: O(K) additional space
- Quickselect: O(1) additional space (ignoring recursion stack)
Quickselect is more space-efficient, especially for large K.
Stability
- Heap: Stable (maintains order for equal elements)
- Quickselect: Not stable (may change the order of equal elements)
If maintaining the original order of elements is important, the Heap approach is preferable.
Suitability for Streaming Data
- Heap: Suitable for streaming data
- Quickselect: Not suitable for streaming data
For scenarios where data comes in a stream or the entire dataset isn’t available at once, the Heap approach is the clear winner.
Ease of Implementation
- Heap: Relatively simple, especially with built-in heap libraries
- Quickselect: More complex, requires careful implementation of partitioning
For quick implementations or when using languages with good heap support, the Heap approach might be easier to code correctly.
Real-world Applications
Understanding and implementing efficient solutions for the Top K Elements problem is crucial for various real-world applications. Let’s explore some scenarios where this problem and its solutions are directly applicable:
1. Search Engine Results
Search engines need to quickly return the most relevant results for a query. Using a heap-based approach, they can efficiently maintain the top K most relevant documents as they process a large corpus of web pages.
2. Recommendation Systems
E-commerce platforms and streaming services use recommendation systems to suggest products or content. These systems often need to find the top K items that best match a user’s preferences from a vast catalog.
3. Network Monitoring
In network management, identifying the top K IP addresses generating the most traffic or the K most frequent error codes can help in troubleshooting and optimization. The streaming nature of network data makes the heap approach particularly suitable.
4. Financial Analysis
In stock market analysis, finding the top K performing stocks or the K largest transactions can provide valuable insights. The ability to process streaming data in real-time makes heap-based solutions valuable in this domain.
5. Social Media Trending Topics
Social media platforms need to identify trending topics or hashtags. By maintaining a heap of the most frequently used terms and updating it as new posts come in, they can efficiently track trending content.
6. Geospatial Queries
Finding the K nearest points of interest to a given location is a common problem in mapping and location-based services. This is a direct application of the Top K Elements problem in two-dimensional space.
Advanced Considerations and Optimizations
As we dive deeper into the Top K Elements problem, there are several advanced considerations and optimizations worth exploring:
1. Handling Duplicates
In some applications, you might need to handle duplicate elements carefully. For example, if you’re finding the top K most frequent elements, you’ll need to count occurrences. This might involve using a hash map in conjunction with your heap or quickselect algorithm.
2. Parallel Processing
For extremely large datasets, parallel processing can significantly speed up both the Heap and Quickselect approaches. You could divide the data into chunks, process each chunk independently, and then merge the results.
3. External Memory Algorithms
When dealing with datasets too large to fit in memory, external memory algorithms become necessary. Modifications of the heap approach, like using a min-heap of size K and processing data in chunks, can be effective in these scenarios.
4. Approximate Algorithms
In some cases, an approximate solution might be acceptable. Algorithms like Count-Min Sketch or HyperLogLog can provide approximate frequencies or top K elements with significantly reduced space complexity.
5. Online vs. Offline Processing
Consider whether you need to process data online (as it arrives) or offline (with the entire dataset available). The heap approach is generally better for online processing, while quickselect can be more efficient for offline processing.
6. Custom Comparators
Both the heap and quickselect approaches can be adapted to use custom comparison functions. This is useful when dealing with complex objects or when the “top” elements are determined by multiple criteria.
Conclusion
The Top K Elements problem is a fundamental challenge in computer science and data processing, with wide-ranging applications in the real world. Mastering efficient solutions to this problem, particularly using Heap and Quickselect algorithms, is crucial for aspiring software engineers and data scientists, especially those aiming for positions at top tech companies.
Both the Heap and Quickselect approaches offer powerful solutions, each with its own strengths:
- The Heap approach excels in scenarios involving streaming data, where consistency is key, and when K is significantly smaller than N.
- The Quickselect approach shines in situations where the entire dataset is available, space is at a premium, and average-case performance is prioritized over worst-case guarantees.
As you prepare for coding interviews or tackle real-world data processing challenges, remember that the choice between these algorithms often depends on the specific requirements of your problem. Factors such as the size of your dataset, the value of K, whether you’re dealing with streaming data, and the importance of stable sorting should all influence your decision.
By understanding these algorithms deeply and recognizing their appropriate use cases, you’ll be well-equipped to tackle a wide range of data processing challenges efficiently and effectively. Keep practicing with diverse datasets and problem variations to sharpen your skills and intuition for when to apply each approach.
Remember, the journey to mastering these algorithms is ongoing. As you encounter new variations of the Top K Elements problem in your studies or work, don’t hesitate to revisit and refine your understanding. Happy coding, and may your algorithms always find the top K elements with blazing efficiency!