Top Go Interview Questions and How to Ace Them
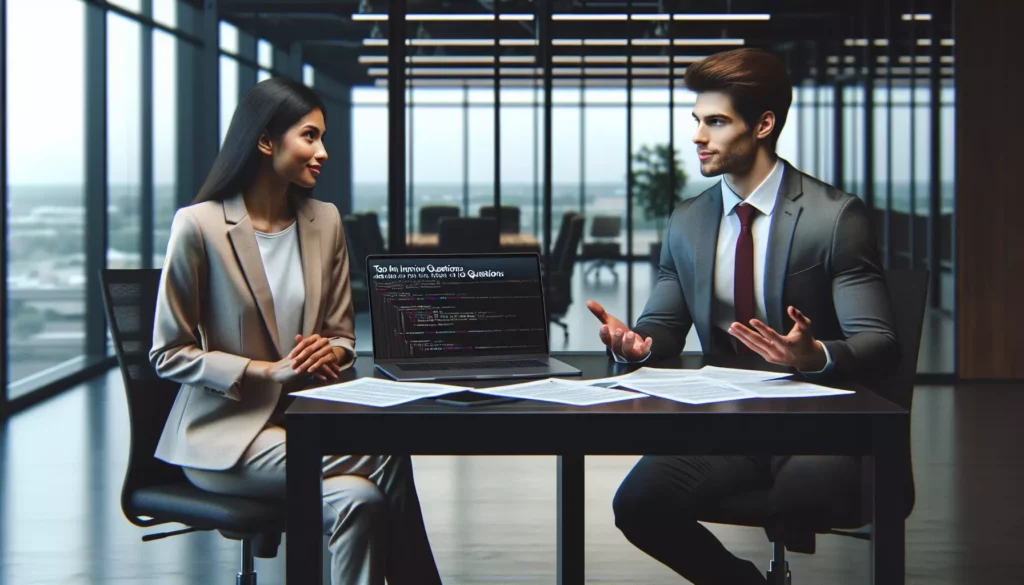
Welcome to our comprehensive guide on Go programming interview questions! Whether you’re a seasoned developer looking to brush up on your skills or a newcomer preparing for your first Go interview, this article will equip you with the knowledge and confidence to tackle common interview questions. We’ll cover a wide range of topics, from basic syntax to advanced concepts, and provide detailed explanations and example code snippets to help you understand and remember key concepts.
Table of Contents
- Introduction to Go
- Basic Concepts
- Data Structures
- Concurrency
- Error Handling
- Interfaces
- Testing
- Best Practices
- Advanced Topics
- Conclusion
1. Introduction to Go
Before diving into specific questions, let’s briefly discuss what makes Go unique and why it’s becoming increasingly popular among developers and companies.
Q: What is Go, and what are its main features?
A: Go, also known as Golang, is an open-source programming language developed by Google. Its main features include:
- Simplicity and readability
- Strong static typing
- Garbage collection
- Built-in concurrency support
- Fast compilation
- Cross-platform compatibility
- Extensive standard library
Q: Why would you choose Go over other programming languages?
A: Go offers several advantages that make it an attractive choice for many projects:
- Excellent performance, comparable to C/C++
- Easy to learn and use, with a clean and straightforward syntax
- Built-in concurrency support with goroutines and channels
- Fast compilation times, enabling rapid development cycles
- Strong standard library, reducing the need for third-party dependencies
- Great for building scalable network services and distributed systems
2. Basic Concepts
Let’s start with some fundamental concepts that are crucial for any Go developer to understand.
Q: What are the basic types in Go?
A: Go has several basic types:
- Numeric types: int, int8, int16, int32, int64, uint, uint8, uint16, uint32, uint64, float32, float64, complex64, complex128
- Boolean type: bool
- String type: string
- Error type: error
Q: How do you declare and initialize variables in Go?
A: There are several ways to declare and initialize variables in Go:
// Using var keyword
var x int = 10
var y = 20 // Type inference
// Short variable declaration
z := 30
// Multiple variable declaration
var a, b int = 1, 2
c, d := 3, 4
// Declare without initialization
var e int
Q: What is the difference between `var` and `:=` in Go?
A: The main differences are:
var
can be used both inside and outside of functions, while:=
can only be used inside functions.var
allows you to declare variables without initialization, while:=
requires an initial value.:=
uses type inference, whilevar
can be used with explicit type declarations.
Q: How do you handle unused variables in Go?
A: Go is strict about unused variables and will throw a compilation error if a declared variable is not used. To handle this, you can:
- Remove the unused variable
- Use the blank identifier
_
to explicitly ignore the variable
// Example of using blank identifier
_, err := someFunction()
if err != nil {
// Handle error
}
3. Data Structures
Understanding Go’s data structures is crucial for writing efficient and idiomatic code.
Q: What are slices in Go, and how do they differ from arrays?
A: Slices are dynamic, flexible views into arrays. The main differences are:
- Arrays have a fixed size, while slices can grow or shrink.
- Slices are reference types, while arrays are value types.
- Slices have a length and a capacity, while arrays only have a length.
// Array declaration
var arr [5]int
// Slice declaration
var slice []int
slice = make([]int, 5, 10) // length 5, capacity 10
Q: How do you implement a stack or queue in Go?
A: You can implement a stack or queue using a slice:
// Stack implementation
type Stack []int
func (s *Stack) Push(v int) {
*s = append(*s, v)
}
func (s *Stack) Pop() (int, bool) {
if len(*s) == 0 {
return 0, false
}
index := len(*s) - 1
element := (*s)[index]
*s = (*s)[:index]
return element, true
}
// Queue implementation
type Queue []int
func (q *Queue) Enqueue(v int) {
*q = append(*q, v)
}
func (q *Queue) Dequeue() (int, bool) {
if len(*q) == 0 {
return 0, false
}
element := (*q)[0]
*q = (*q)[1:]
return element, true
}
Q: What are maps in Go, and how do you use them?
A: Maps are Go’s built-in associative data type (hash tables). They store key-value pairs and provide fast lookups, insertions, and deletions.
// Declare and initialize a map
m := make(map[string]int)
// Add key-value pairs
m["apple"] = 1
m["banana"] = 2
// Retrieve a value
value, exists := m["apple"]
if exists {
fmt.Println(value) // Output: 1
}
// Delete a key-value pair
delete(m, "banana")
// Iterate over a map
for key, value := range m {
fmt.Printf("%s: %d\n", key, value)
}
4. Concurrency
Go’s built-in concurrency features are one of its strongest selling points. Understanding these concepts is crucial for any Go developer.
Q: What are goroutines, and how do they differ from traditional threads?
A: Goroutines are lightweight, user-space threads managed by the Go runtime. They differ from traditional threads in several ways:
- Goroutines are much cheaper to create and destroy than OS threads.
- They have a smaller stack size that can grow and shrink as needed.
- The Go runtime multiplexes goroutines onto a smaller number of OS threads.
- Switching between goroutines is much faster than switching between threads.
// Starting a goroutine
go func() {
// Do something concurrently
}()
Q: What are channels in Go, and how do you use them?
A: Channels are a typed conduit through which you can send and receive values with the channel operator <-
. They are used for communication and synchronization between goroutines.
// Create a channel
ch := make(chan int)
// Send a value on a channel
ch <- 42
// Receive a value from a channel
value := <-ch
// Close a channel
close(ch)
// Range over a channel
for v := range ch {
fmt.Println(v)
}
Q: What is the difference between buffered and unbuffered channels?
A: The main differences are:
- Unbuffered channels have no capacity and require both a sender and receiver to be ready at the same time for communication to take place.
- Buffered channels have a capacity and can hold a specified number of values before blocking.
// Unbuffered channel
unbuffered := make(chan int)
// Buffered channel with capacity 5
buffered := make(chan int, 5)
Q: How do you handle race conditions in Go?
A: There are several ways to handle race conditions in Go:
- Use channels for communication between goroutines
- Use the
sync
package (e.g.,Mutex
,RWMutex
) for synchronization - Use atomic operations from the
sync/atomic
package - Use the
-race
flag when testing to detect race conditions
import "sync"
var (
counter int
mutex sync.Mutex
)
func incrementCounter() {
mutex.Lock()
defer mutex.Unlock()
counter++
}
5. Error Handling
Proper error handling is crucial for writing robust and reliable Go programs.
Q: How does Go handle errors?
A: Go uses explicit error handling through return values. Functions that can fail typically return an error as their last return value.
func doSomething() (int, error) {
// Do something that might fail
if somethingWentWrong {
return 0, errors.New("something went wrong")
}
return 42, nil
}
result, err := doSomething()
if err != nil {
// Handle the error
log.Fatal(err)
}
// Use the result
Q: What is the difference between `panic` and `error` in Go?
A: The main differences are:
- Errors are used for expected failure conditions and are part of a function’s normal return values.
- Panic is used for unexpected runtime errors that should crash the program if not recovered.
- Errors are handled using conditional checks, while panics can be caught using `defer` and `recover`.
Q: How do you create custom error types in Go?
A: You can create custom error types by implementing the `error` interface:
type MyError struct {
Code int
Message string
}
func (e *MyError) Error() string {
return fmt.Sprintf("error %d: %s", e.Code, e.Message)
}
func someFunction() error {
return &MyError{Code: 404, Message: "Not Found"}
}
6. Interfaces
Interfaces are a powerful feature in Go that enables polymorphism and decoupling.
Q: What are interfaces in Go, and how do you use them?
A: Interfaces in Go are a way to specify behavior. They define a set of methods that a type must implement to satisfy the interface. Types implicitly implement interfaces by implementing the required methods.
type Writer interface {
Write([]byte) (int, error)
}
type FileWriter struct {
// ...
}
func (fw *FileWriter) Write(data []byte) (int, error) {
// Implement writing to a file
}
// FileWriter now implements the Writer interface
Q: What is an empty interface, and when would you use it?
A: An empty interface, `interface{}`, is an interface with no methods. It can hold values of any type. You might use it when:
- You need to handle values of unknown type
- You’re implementing a generic data structure
- You’re working with reflection
func printAny(v interface{}) {
fmt.Printf("Value: %v, Type: %T\n", v, v)
}
printAny(42) // Value: 42, Type: int
printAny("hello") // Value: hello, Type: string
printAny(true) // Value: true, Type: bool
7. Testing
Go has built-in support for testing, making it easy to write and run tests for your code.
Q: How do you write and run tests in Go?
A: Go uses the built-in `testing` package for writing tests. Test files are named with a `_test.go` suffix and contain functions starting with `Test`.
// main.go
package main
func Add(a, b int) int {
return a + b
}
// main_test.go
package main
import "testing"
func TestAdd(t *testing.T) {
result := Add(2, 3)
if result != 5 {
t.Errorf("Add(2, 3) = %d; want 5", result)
}
}
To run tests, use the `go test` command in your terminal:
go test
Q: What is table-driven testing, and why is it useful?
A: Table-driven testing is a technique where you define a table of test cases and iterate over them. It’s useful because:
- It allows you to easily add new test cases
- It reduces code duplication
- It makes the test structure more readable and maintainable
func TestAdd(t *testing.T) {
tests := []struct {
a, b, want int
}{
{2, 3, 5},
{0, 0, 0},
{-1, 1, 0},
{100, 200, 300},
}
for _, tt := range tests {
t.Run(fmt.Sprintf("%d+%d", tt.a, tt.b), func(t *testing.T) {
if got := Add(tt.a, tt.b); got != tt.want {
t.Errorf("Add(%d, %d) = %d; want %d", tt.a, tt.b, got, tt.want)
}
})
}
}
8. Best Practices
Following Go best practices will help you write clean, efficient, and idiomatic code.
Q: What are some Go code formatting conventions?
A: Go has official formatting guidelines enforced by the `gofmt` tool. Some key conventions include:
- Use tabs for indentation
- Use camelCase for variable and function names
- Use PascalCase for exported names (public)
- Keep lines under 80 characters when possible
- Group related declarations
Q: What is the purpose of the `init()` function in Go?
A: The `init()` function is used for package initialization. It’s called automatically before the main function and can be used to:
- Initialize package-level variables
- Register data structures
- Perform one-time computations
var globalVar int
func init() {
globalVar = 42
// Perform other initialization tasks
}
func main() {
// globalVar is already initialized
}
Q: What are some common mistakes to avoid in Go?
A: Some common mistakes include:
- Ignoring errors
- Using pointers unnecessarily
- Not using `defer` for cleanup operations
- Misusing goroutines and channels
- Not closing resources properly (e.g., files, network connections)
- Using global variables excessively
9. Advanced Topics
For more experienced Go developers, interviewers might ask about advanced topics to gauge the depth of your knowledge.
Q: What is reflection in Go, and when would you use it?
A: Reflection is the ability of a program to examine, introspect, and modify its own structure and behavior at runtime. In Go, the `reflect` package provides this functionality. You might use reflection when:
- Implementing generic algorithms
- Working with unknown types
- Marshaling and unmarshaling data
- Implementing ORM-like functionality
import "reflect"
func printFieldNames(v interface{}) {
t := reflect.TypeOf(v)
for i := 0; i < t.NumField(); i++ {
fmt.Println(t.Field(i).Name)
}
}
type Person struct {
Name string
Age int
}
printFieldNames(Person{}) // Outputs: Name, Age
Q: How does Go’s garbage collector work?
A: Go uses a concurrent, tri-color mark-and-sweep garbage collector. Key points include:
- It runs concurrently with the program, minimizing stop-the-world pauses
- It uses a write barrier to maintain consistency during concurrent collection
- It employs escape analysis to allocate some objects on the stack instead of the heap
- It can be tuned using environment variables and runtime functions
Q: What are Go modules, and how do they work?
A: Go modules are the official dependency management system for Go. They provide:
- Version control for dependencies
- Reproducible builds
- Semantic versioning support
- Ability to work outside of GOPATH
// Initialize a new module
go mod init example.com/myproject
// Add a dependency
go get github.com/some/dependency
// Update dependencies
go get -u
// Tidy up the go.mod file
go mod tidy
10. Conclusion
This comprehensive guide has covered a wide range of Go interview questions, from basic syntax to advanced concepts. By understanding these topics and practicing your coding skills, you’ll be well-prepared for your next Go interview.
Remember that interviews are not just about memorizing answers but also about demonstrating your problem-solving skills and ability to write clean, efficient code. Be prepared to explain your thought process and discuss trade-offs in your solutions.
Good luck with your interview preparation, and don’t forget to keep coding and exploring Go’s rich ecosystem!