Top DoorDash Interview Questions: Ace Your Technical Interview
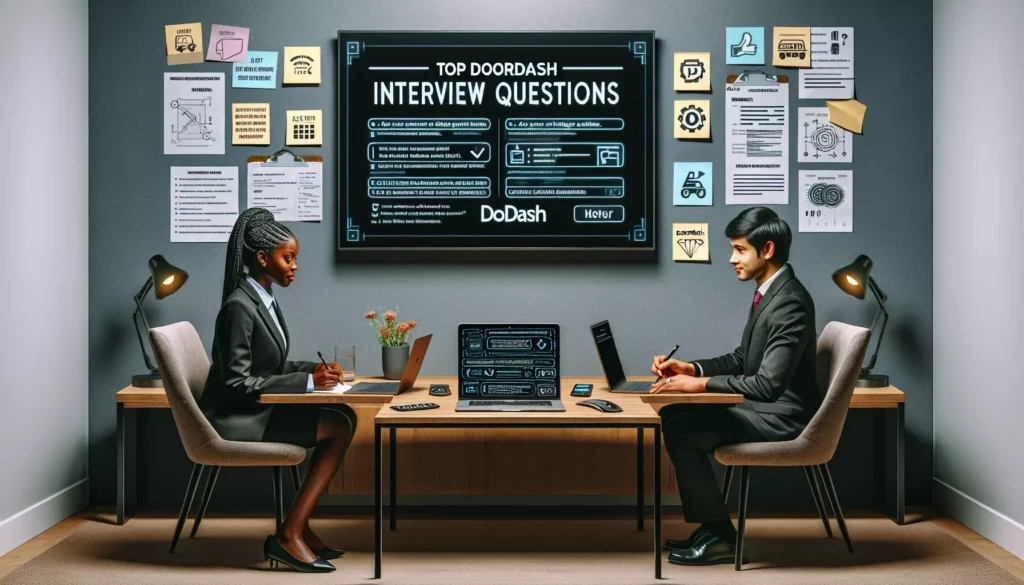
Are you gearing up for a technical interview with DoorDash? As one of the leading food delivery platforms, DoorDash is always on the lookout for talented software engineers to join their team. To help you prepare, we’ve compiled a comprehensive list of DoorDash interview questions, along with tips and strategies to ace your interview. Whether you’re a seasoned developer or a fresh graduate, this guide will give you the edge you need to succeed.
Table of Contents
- Introduction to DoorDash Interviews
- Coding and Algorithm Questions
- System Design Questions
- Behavioral Questions
- Interview Tips and Strategies
- How to Prepare for Your DoorDash Interview
- Conclusion
1. Introduction to DoorDash Interviews
DoorDash’s interview process typically consists of several rounds, including:
- Initial phone screen
- Technical phone interview
- On-site interviews (or virtual on-site interviews)
The technical interviews at DoorDash focus on assessing your problem-solving skills, coding abilities, and system design knowledge. You’ll also be evaluated on your communication skills and cultural fit within the company.
2. Coding and Algorithm Questions
DoorDash’s coding questions often focus on data structures, algorithms, and problem-solving. Here are some common types of questions you might encounter:
2.1. Array and String Manipulation
Question: Given an array of integers, find two numbers such that they add up to a specific target number.
Solution: This problem can be solved efficiently using a hash table.
def two_sum(nums, target):
seen = {}
for i, num in enumerate(nums):
complement = target - num
if complement in seen:
return [seen[complement], i]
seen[num] = i
return []
# Example usage
nums = [2, 7, 11, 15]
target = 9
result = two_sum(nums, target)
print(f"Indices: {result}") # Output: Indices: [0, 1]
2.2. Graph and Tree Traversal
Question: Implement a function to find the shortest path between two nodes in a graph.
Solution: We can use Breadth-First Search (BFS) to find the shortest path.
from collections import deque
def shortest_path(graph, start, end):
queue = deque([(start, [start])])
visited = set([start])
while queue:
(node, path) = queue.popleft()
if node == end:
return path
for neighbor in graph[node]:
if neighbor not in visited:
visited.add(neighbor)
queue.append((neighbor, path + [neighbor]))
return None
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
start = 'A'
end = 'F'
path = shortest_path(graph, start, end)
print(f"Shortest path from {start} to {end}: {path}")
# Output: Shortest path from A to F: ['A', 'C', 'F']
2.3. Dynamic Programming
Question: Given a set of food items with values and weights, and a maximum weight capacity, find the maximum value that can be carried.
Solution: This is the classic Knapsack problem, which can be solved using dynamic programming.
def knapsack(values, weights, capacity):
n = len(values)
dp = [[0 for _ in range(capacity + 1)] for _ in range(n + 1)]
for i in range(1, n + 1):
for w in range(1, capacity + 1):
if weights[i-1] <= w:
dp[i][w] = max(values[i-1] + dp[i-1][w-weights[i-1]], dp[i-1][w])
else:
dp[i][w] = dp[i-1][w]
return dp[n][capacity]
# Example usage
values = [60, 100, 120]
weights = [10, 20, 30]
capacity = 50
max_value = knapsack(values, weights, capacity)
print(f"Maximum value: {max_value}") # Output: Maximum value: 220
2.4. Sorting and Searching
Question: Implement a function to find the kth largest element in an unsorted array.
Solution: We can use the QuickSelect algorithm to solve this problem efficiently.
import random
def quick_select(nums, k):
k = len(nums) - k # Convert to kth smallest
def partition(left, right):
pivot_idx = random.randint(left, right)
pivot = nums[pivot_idx]
nums[pivot_idx], nums[right] = nums[right], nums[pivot_idx]
store_idx = left
for i in range(left, right):
if nums[i] < pivot:
nums[store_idx], nums[i] = nums[i], nums[store_idx]
store_idx += 1
nums[right], nums[store_idx] = nums[store_idx], nums[right]
return store_idx
def select(left, right):
if left == right:
return nums[left]
pivot_idx = partition(left, right)
if k == pivot_idx:
return nums[k]
elif k < pivot_idx:
return select(left, pivot_idx - 1)
else:
return select(pivot_idx + 1, right)
return select(0, len(nums) - 1)
# Example usage
nums = [3, 2, 1, 5, 6, 4]
k = 2
result = quick_select(nums, k)
print(f"{k}th largest element: {result}") # Output: 2th largest element: 5
3. System Design Questions
System design questions are crucial for senior engineering positions at DoorDash. Here are some potential questions you might face:
3.1. Design DoorDash’s Food Delivery System
Question: Design a scalable system for DoorDash’s food delivery platform.
Key points to consider:
- User management (customers, restaurants, delivery drivers)
- Order placement and tracking
- Restaurant menu management
- Real-time location tracking for drivers
- Payment processing
- Scalability and load balancing
3.2. Design a Real-time ETA System
Question: Design a system that can provide accurate estimated time of arrival (ETA) for food deliveries.
Key points to consider:
- Real-time traffic data integration
- Historical delivery time analysis
- Machine learning models for prediction
- Handling of various factors (restaurant preparation time, driver availability)
- Scalability and low-latency requirements
3.3. Design a Recommendation System
Question: Design a recommendation system for suggesting restaurants to users based on their preferences and order history.
Key points to consider:
- User profiling and preference tracking
- Collaborative filtering algorithms
- Content-based filtering
- Real-time updates and personalization
- Scalability and performance optimization
4. Behavioral Questions
DoorDash also evaluates candidates on their soft skills and cultural fit. Here are some common behavioral questions you might encounter:
- Tell me about a time when you had to deal with a difficult team member.
- Describe a situation where you had to make a decision with incomplete information.
- How do you stay updated with the latest technologies and industry trends?
- Tell me about a project you’re most proud of and why.
- How do you handle tight deadlines and pressure?
- Describe a time when you had to give constructive feedback to a colleague.
When answering behavioral questions, use the STAR method (Situation, Task, Action, Result) to structure your responses effectively.
5. Interview Tips and Strategies
To increase your chances of success in a DoorDash interview, consider the following tips:
- Practice coding on a whiteboard: Many technical interviews involve coding on a whiteboard or in a shared online editor. Practice this to become comfortable with the format.
- Think out loud: Communicate your thought process clearly as you solve problems. This gives the interviewer insight into your problem-solving approach.
- Ask clarifying questions: Don’t hesitate to ask for more information or clarification about the problem. This shows that you’re thorough and careful in your approach.
- Consider edge cases: When solving problems, always consider edge cases and potential issues. This demonstrates attention to detail and thoroughness.
- Optimize your solutions: After solving a problem, discuss potential optimizations or alternative approaches. This shows your ability to think critically about efficiency and scalability.
- Be familiar with DoorDash’s products and challenges: Research DoorDash’s business model, products, and the technical challenges they face. This knowledge can be valuable during system design discussions and behavioral interviews.
- Practice explaining technical concepts: Be prepared to explain complex technical ideas in simple terms. This is particularly important for system design questions.
6. How to Prepare for Your DoorDash Interview
To effectively prepare for your DoorDash interview, follow these steps:
- Review fundamental data structures and algorithms: Ensure you have a solid understanding of arrays, linked lists, trees, graphs, hash tables, and common algorithms like sorting and searching.
- Practice coding problems: Solve coding problems on platforms like LeetCode, HackerRank, or AlgoCademy. Focus on medium to hard difficulty problems, as DoorDash interviews can be challenging.
- Study system design: If you’re interviewing for a senior position, dedicate time to studying system design principles, scalability, and distributed systems.
- Mock interviews: Conduct mock interviews with friends or use online platforms that offer mock interview services. This will help you get comfortable with the interview format and improve your communication skills.
- Review your past projects: Be prepared to discuss your previous work experiences and projects in detail. Focus on your contributions, challenges faced, and lessons learned.
- Research DoorDash: Familiarize yourself with DoorDash’s business model, recent news, and technological challenges. This will help you align your answers with the company’s goals and values.
- Prepare questions for the interviewer: Have thoughtful questions ready to ask your interviewer about the role, team, and company. This demonstrates your genuine interest and engagement.
7. Conclusion
Preparing for a DoorDash interview requires a combination of technical skills, problem-solving abilities, and effective communication. By focusing on the areas outlined in this guide and consistently practicing, you’ll be well-equipped to tackle the challenges of the interview process.
Remember that the interview is not just about showcasing your technical prowess but also about demonstrating your ability to work in a team, think critically, and align with DoorDash’s mission and values. Stay calm, be confident in your abilities, and approach each question as an opportunity to showcase your skills and passion for technology.
Good luck with your DoorDash interview! With thorough preparation and the right mindset, you’ll be well on your way to joining one of the most innovative companies in the food delivery industry.