Top C++ Interview Questions: Master Your Next Technical Interview
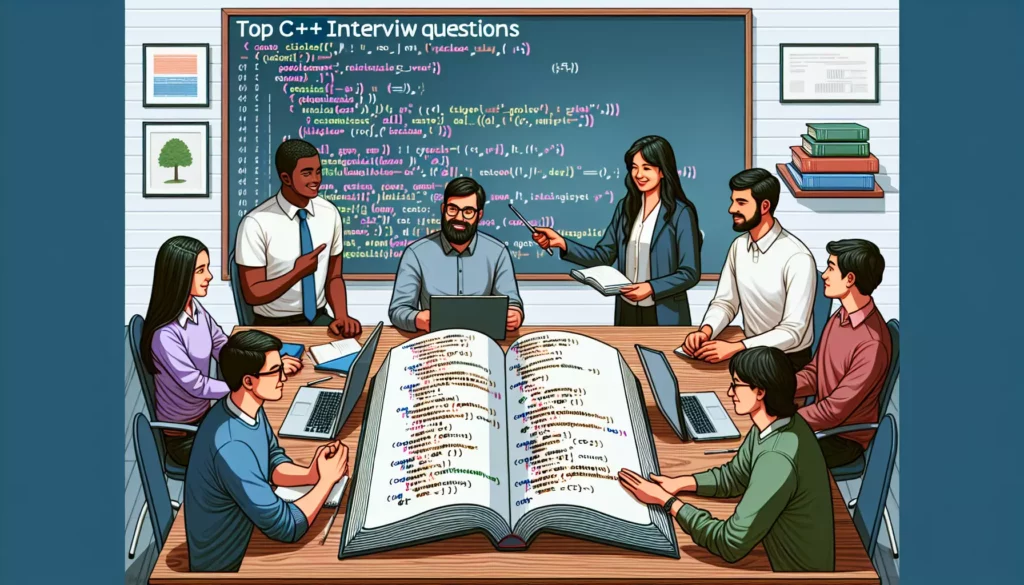
Are you preparing for a C++ interview and feeling overwhelmed? Don’t worry! We’ve got you covered with this comprehensive guide to the most common C++ interview questions. Whether you’re a fresh graduate or an experienced developer looking to switch jobs, this article will help you brush up on your C++ knowledge and boost your confidence for your next technical interview.
At AlgoCademy, we understand the importance of being well-prepared for coding interviews, especially when aiming for positions at top tech companies. That’s why we’ve compiled this extensive list of C++ interview questions, complete with detailed explanations and sample code snippets. Let’s dive in!
1. What are the key features of C++?
This is often one of the first questions you’ll encounter in a C++ interview. It’s essential to have a solid understanding of the language’s core features.
Key features of C++ include:
- Object-Oriented Programming (OOP)
- Generic Programming
- Multi-paradigm language (supports procedural, object-oriented, and generic programming)
- Strong typing
- Compile-time polymorphism (function overloading and templates)
- Runtime polymorphism (virtual functions)
- Memory management
- Exception handling
- Standard Template Library (STL)
Be prepared to explain these features and provide examples of how they’re used in C++ programming.
2. What is the difference between C and C++?
This question tests your understanding of C++’s evolution from C and the key differences between the two languages.
Main differences include:
- C++ supports OOP, while C is a procedural programming language
- C++ has built-in support for classes and objects
- C++ supports function overloading, while C doesn’t
- C++ has a richer set of library functions (STL)
- C++ supports exception handling, which C doesn’t
- C++ allows for user-defined operators (operator overloading)
- C++ has built-in support for references, while C doesn’t
3. Explain the concept of object-oriented programming in C++
Object-Oriented Programming (OOP) is a fundamental concept in C++. Be prepared to discuss the four main principles of OOP:
- Encapsulation: Bundling data and methods that operate on that data within a single unit (class)
- Inheritance: Allowing a class to inherit properties and methods from another class
- Polymorphism: The ability of objects of different classes to respond to the same method call
- Abstraction: Hiding complex implementation details and showing only the necessary features of an object
Here’s a simple example demonstrating these concepts:
class Shape {
protected:
int width;
int height;
public:
virtual int area() = 0;
void setWidth(int w) { width = w; }
void setHeight(int h) { height = h; }
};
class Rectangle : public Shape {
public:
int area() override {
return (width * height);
}
};
class Triangle : public Shape {
public:
int area() override {
return (width * height / 2);
}
};
In this example, Shape
is an abstract base class, and Rectangle
and Triangle
are derived classes that inherit from Shape
. The area()
function demonstrates polymorphism, as it’s implemented differently in each derived class.
4. What is a constructor? What are the different types of constructors in C++?
A constructor is a special member function that is automatically called when an object of a class is created. It’s used to initialize the object’s data members.
Types of constructors in C++:
- Default constructor: Takes no parameters and is automatically provided by the compiler if no constructor is defined
- Parameterized constructor: Takes one or more parameters to initialize object members
- Copy constructor: Creates a new object as a copy of an existing object
- Move constructor: Transfers ownership of resources from one object to another (C++11 and later)
Here’s an example demonstrating different types of constructors:
class MyClass {
private:
int x;
std::string str;
public:
// Default constructor
MyClass() : x(0), str("") {}
// Parameterized constructor
MyClass(int val, std::string s) : x(val), str(s) {}
// Copy constructor
MyClass(const MyClass& other) : x(other.x), str(other.str) {}
// Move constructor
MyClass(MyClass&& other) noexcept : x(std::move(other.x)), str(std::move(other.str)) {}
};
5. What is the difference between stack and heap memory allocation?
Understanding memory management is crucial in C++. Be prepared to explain the differences between stack and heap memory allocation:
- Stack:
- Faster allocation and deallocation
- Memory is automatically managed
- Limited in size
- Used for local variables and function calls
- Heap:
- Slower allocation and deallocation
- Manual memory management (using new and delete)
- Larger memory size available
- Used for dynamic memory allocation
Example of stack and heap allocation:
void exampleFunction() {
// Stack allocation
int stackVar = 5;
// Heap allocation
int* heapVar = new int(10);
// Use the variables
std::cout << "Stack variable: " << stackVar << std::endl;
std::cout << "Heap variable: " << *heapVar << std::endl;
// Clean up heap memory
delete heapVar;
} // stackVar is automatically deallocated when the function ends
6. What is a virtual function? How does it work?
Virtual functions are a key concept in C++ polymorphism. They allow a base class to declare a function that can be overridden by derived classes.
Key points about virtual functions:
- Declared using the
virtual
keyword in the base class - Enable runtime polymorphism
- Use a virtual function table (vtable) for implementation
- Allow for correct function calls when using base class pointers or references to derived class objects
Here’s an example of virtual functions in action:
class Animal {
public:
virtual void makeSound() {
std::cout << "The animal makes a sound" << std::endl;
}
};
class Dog : public Animal {
public:
void makeSound() override {
std::cout << "The dog barks" << std::endl;
}
};
class Cat : public Animal {
public:
void makeSound() override {
std::cout << "The cat meows" << std::endl;
}
};
int main() {
Animal* animal1 = new Dog();
Animal* animal2 = new Cat();
animal1->makeSound(); // Output: The dog barks
animal2->makeSound(); // Output: The cat meows
delete animal1;
delete animal2;
return 0;
}
7. What is the difference between references and pointers in C++?
Both references and pointers are used to indirectly access variables, but they have some key differences:
- References:
- Must be initialized when declared
- Cannot be null
- Cannot be reassigned to refer to a different object
- Safer and easier to use
- Pointers:
- Can be declared without initialization
- Can be null
- Can be reassigned to point to different objects
- More flexible but require careful management
Example demonstrating the differences:
int main() {
int x = 5;
int y = 10;
// Reference
int& ref = x;
ref = 7; // x is now 7
// Pointer
int* ptr = &x;
*ptr = 8; // x is now 8
ptr = &y; // ptr now points to y
*ptr = 12; // y is now 12
return 0;
}
8. Explain the concept of function overloading in C++
Function overloading is a feature of C++ that allows multiple functions with the same name but different parameter lists to coexist in the same scope. The compiler determines which function to call based on the number, types, and order of the arguments passed.
Key points about function overloading:
- Functions must have different parameter lists (number, type, or order of parameters)
- Return type alone is not sufficient for overloading
- Enables more intuitive function naming
- Resolved at compile-time (static polymorphism)
Example of function overloading:
class Calculator {
public:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
int add(int a, int b, int c) {
return a + b + c;
}
};
int main() {
Calculator calc;
std::cout << calc.add(5, 3) << std::endl; // Calls int add(int, int)
std::cout << calc.add(3.14, 2.86) << std::endl; // Calls double add(double, double)
std::cout << calc.add(1, 2, 3) << std::endl; // Calls int add(int, int, int)
return 0;
}
9. What is the purpose of the ‘const’ keyword in C++?
The const
keyword in C++ is used to declare constants and to specify that a variable or object cannot be modified. It’s an important tool for writing safer and more efficient code.
Uses of const
:
- Declaring constants
- Const pointers and references
- Const member functions
- Const objects
Examples of const
usage:
// Constant variable
const int MAX_SIZE = 100;
// Const pointer to non-const int
int value = 5;
int* const ptr = &value;
*ptr = 10; // OK
// ptr = &other_value; // Error: ptr is const
// Const pointer to const int
const int* const ptr2 = &value;
// *ptr2 = 15; // Error: *ptr2 is const
// ptr2 = &other_value; // Error: ptr2 is const
class MyClass {
public:
// Const member function
int getValue() const {
return value;
}
private:
int value;
};
// Using const objects
const MyClass obj;
int val = obj.getValue(); // OK
// obj.setValue(10); // Error: obj is const
10. What is the Standard Template Library (STL) in C++?
The Standard Template Library (STL) is a powerful set of C++ template classes to provide general-purpose classes and functions with templates that implement many popular and commonly used algorithms and data structures.
Key components of the STL:
- Containers: Data structures like vector, list, map, set, etc.
- Algorithms: Functions for searching, sorting, counting, etc.
- Iterators: Objects that provide a way to access elements in containers
- Function objects: Objects that can be used as function arguments
Example using STL components:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 2, 8, 1, 9};
// Sort the vector
std::sort(numbers.begin(), numbers.end());
// Print sorted vector
for (const auto& num : numbers) {
std::cout << num << " ";
}
std::cout << std::endl;
// Find an element
auto it = std::find(numbers.begin(), numbers.end(), 8);
if (it != numbers.end()) {
std::cout << "Found 8 at position: " << std::distance(numbers.begin(), it) << std::endl;
}
return 0;
}
11. What is a smart pointer? What types of smart pointers are available in C++?
Smart pointers are objects that act like pointers but provide additional features such as automatic memory management and ownership semantics. They help prevent common problems like memory leaks and dangling pointers.
Types of smart pointers in C++:
std::unique_ptr
: Exclusive ownership, automatically deletes the object it points to when it goes out of scopestd::shared_ptr
: Shared ownership, uses reference counting to manage the lifetime of the pointed-to objectstd::weak_ptr
: Non-owning reference to an object managed bystd::shared_ptr
, helps break circular references
Example using smart pointers:
#include <iostream>
#include <memory>
class MyClass {
public:
MyClass(int val) : value(val) {
std::cout << "MyClass constructor called" << std::endl;
}
~MyClass() {
std::cout << "MyClass destructor called" << std::endl;
}
int getValue() const { return value; }
private:
int value;
};
int main() {
// unique_ptr example
std::unique_ptr<MyClass> uniquePtr = std::make_unique<MyClass>(42);
std::cout << "uniquePtr value: " << uniquePtr->getValue() << std::endl;
// shared_ptr example
std::shared_ptr<MyClass> sharedPtr1 = std::make_shared<MyClass>(10);
std::shared_ptr<MyClass> sharedPtr2 = sharedPtr1;
std::cout << "sharedPtr1 value: " << sharedPtr1->getValue() << std::endl;
std::cout << "sharedPtr2 value: " << sharedPtr2->getValue() << std::endl;
// weak_ptr example
std::weak_ptr<MyClass> weakPtr = sharedPtr1;
if (auto sharedFromWeak = weakPtr.lock()) {
std::cout << "weakPtr value: " << sharedFromWeak->getValue() << std::endl;
}
return 0;
} // All smart pointers are automatically deleted here
12. What is the difference between a struct and a class in C++?
In C++, structs and classes are very similar, with only a few key differences:
- Default access specifier:
- struct: public by default
- class: private by default
- Inheritance:
- struct: public inheritance by default
- class: private inheritance by default
Other than these differences, structs and classes can have member functions, constructors, destructors, and can use inheritance and polymorphism.
Example demonstrating the differences:
struct MyStruct {
int x; // public by default
void print() { std::cout << x << std::endl; }
};
class MyClass {
int x; // private by default
public:
void print() { std::cout << x << std::endl; }
};
int main() {
MyStruct s;
s.x = 5; // OK, x is public
s.print();
MyClass c;
// c.x = 5; // Error, x is private
c.print();
return 0;
}
13. Explain the concept of exception handling in C++
Exception handling is a mechanism in C++ for dealing with runtime errors or exceptional situations that may occur during program execution. It allows you to separate error-handling code from normal code, making your programs more robust and easier to maintain.
Key components of exception handling:
try
: Encloses the code that might throw an exceptionthrow
: Used to throw an exception when a problem occurscatch
: Catches and handles the exception
Example of exception handling:
#include <iostream>
#include <stdexcept>
double divide(double a, double b) {
if (b == 0) {
throw std::runtime_error("Division by zero!");
}
return a / b;
}
int main() {
try {
std::cout << divide(10, 2) << std::endl; // OK
std::cout << divide(10, 0) << std::endl; // Throws exception
}
catch (const std::exception& e) {
std::cerr << "Caught exception: " << e.what() << std::endl;
}
return 0;
}
14. What is a lambda expression in C++?
Lambda expressions, introduced in C++11, allow you to create anonymous function objects. They provide a concise way to define small, inline functions that can be used in place of function objects or function pointers.
Syntax of a lambda expression:
[capture clause](parameters) -> return_type { function body }
Example using lambda expressions:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
// Using a lambda to print each number
std::for_each(numbers.begin(), numbers.end(), [](int n) {
std::cout << n << " ";
});
std::cout << std::endl;
// Using a lambda to transform the vector
std::transform(numbers.begin(), numbers.end(), numbers.begin(),
[](int n) { return n * 2; });
// Print the transformed vector
for (int n : numbers) {
std::cout << n << " ";
}
std::cout << std::endl;
return 0;
}
15. What is the difference between compile-time and runtime polymorphism?
Polymorphism in C++ can be achieved in two ways: compile-time (static) polymorphism and runtime (dynamic) polymorphism.
Compile-time polymorphism:
- Achieved through function overloading and templates
- Resolved at compile-time
- More efficient as there’s no runtime overhead
- Example: Function overloading, operator overloading
Runtime polymorphism:
- Achieved through virtual functions and inheritance
- Resolved at runtime
- Allows for more flexibility and extensibility
- Example: Virtual functions in base and derived classes
Example demonstrating both types of polymorphism:
// Compile-time polymorphism (function overloading)
class MathOperations {
public:
int add(int a, int b) { return a + b; }
double add(double a, double b) { return a + b; }
};
// Runtime polymorphism (virtual functions)
class Shape {
public:
virtual double area() = 0;
};
class Rectangle : public Shape {
private:
double width, height;
public:
Rectangle(double w, double h) : width(w), height(h) {}
double area() override { return width * height; }
};
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double area() override { return 3.14159 * radius * radius; }
};
int main() {
MathOperations math;
std::cout << math.add(5, 3) << std::endl; // Calls int add(int, int)
std::cout << math.add(3.14, 2.86) << std::endl; // Calls double add(double, double)
Shape* shape1 = new Rectangle(5, 3);
Shape* shape2 = new Circle(2);
std::cout << shape1->area() << std::endl; // Calls Rectangle::area()
std::cout << shape2->area() << std::endl; // Calls Circle::area()
delete shape1;
delete shape2;
return 0;
}
Conclusion
Mastering these C++ interview questions will significantly boost your chances of success in your next technical interview. Remember, the key to acing these interviews is not just memorizing answers, but understanding the underlying concepts and being able to apply them in practical scenarios.
At AlgoCademy, we’re committed to helping you develop your programming skills and prepare for technical interviews. Our platform offers interactive coding tutorials, AI-powered assistance, and a wealth of resources to help you progress from beginner-level coding to tackling complex algorithmic problems.
Keep practicing, stay curious, and don’t hesitate to dive deeper into any topics that interest you or challenge you. With dedication and the right resources, you’ll be well-prepared to tackle any C++ interview question that comes your way. Good luck with your interview preparation!