Top C# Interview Questions: Ace Your Next Technical Interview
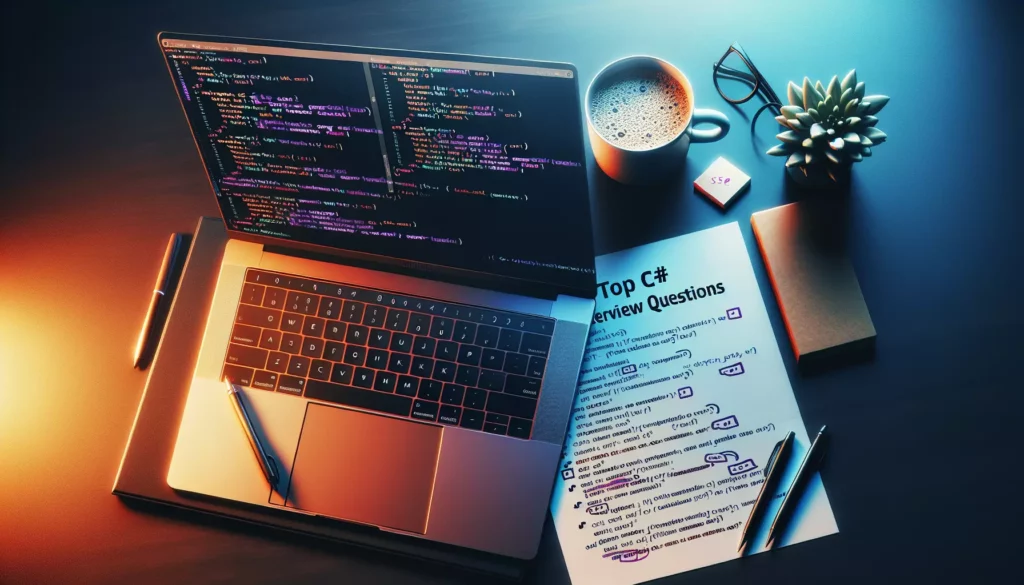
Are you preparing for a C# developer position? Whether you’re a seasoned programmer or just starting your journey in the world of .NET, being well-prepared for technical interviews is crucial. In this comprehensive guide, we’ll explore some of the most common and important C# interview questions you might encounter. We’ll cover a range of topics from basic concepts to more advanced features of C#, helping you sharpen your skills and boost your confidence for that upcoming interview.
Table of Contents
- C# Basics
- Object-Oriented Programming in C#
- Advanced C# Concepts
- .NET Framework and CLR
- Performance and Optimization
- Coding Challenges
- Best Practices and Design Patterns
- Interview Tips and Preparation Strategies
1. C# Basics
Q1: What is C#, and how does it differ from other programming languages?
C# is a modern, object-oriented programming language developed by Microsoft as part of the .NET framework. It combines the power and flexibility of C++ with the simplicity of Visual Basic. Some key differences include:
- Strong typing and type safety
- Automatic memory management through garbage collection
- Support for both managed and unmanaged code
- Extensive standard library and framework support
- Language-level support for asynchronous programming
Q2: Explain the difference between “value types” and “reference types” in C#.
In C#, data types are categorized into value types and reference types:
- Value types directly contain their data and are stored on the stack. Examples include int, float, char, and struct.
- Reference types store a reference to their data, which is allocated on the heap. Examples include classes, interfaces, delegates, and arrays.
The main differences are:
- Memory allocation: Stack vs. Heap
- Assignment behavior: Copy of value vs. Copy of reference
- Nullability: Value types cannot be null (unless using nullable types), while reference types can be null
Q3: What are the access modifiers in C#, and what do they do?
C# provides several access modifiers to control the visibility and accessibility of types and type members:
- public: Accessible from anywhere
- private: Accessible only within the same class or struct
- protected: Accessible within the same class or derived classes
- internal: Accessible within the same assembly
- protected internal: Accessible within the same assembly or derived classes in other assemblies
- private protected (C# 7.2+): Accessible within the same class or derived classes within the same assembly
Q4: What is the difference between “const” and “readonly” keywords?
Both const
and readonly
are used to create immutable fields, but they have some key differences:
- const:
- Must be initialized at declaration
- Value is determined at compile-time
- Can only be used with primitive types and strings
- Is implicitly static
- readonly:
- Can be initialized at declaration or in a constructor
- Value can be determined at runtime
- Can be used with any type
- Can be instance-level or static
2. Object-Oriented Programming in C#
Q5: Explain the four pillars of Object-Oriented Programming and how they are implemented in C#.
The four pillars of OOP are:
- Encapsulation: Hiding the internal details of an object and providing a public interface. In C#, this is achieved through access modifiers and properties.
- Inheritance: Allowing a class to inherit properties and methods from another class. C# supports single inheritance for classes using the
:
operator. - Polymorphism: The ability of objects to take on multiple forms. C# implements this through method overriding and interfaces.
- Abstraction: Representing essential features without including background details. C# provides abstract classes and interfaces for this purpose.
Q6: What is the difference between an abstract class and an interface in C#?
While both abstract classes and interfaces are used to achieve abstraction, they have some key differences:
- Abstract Class:
- Can have both abstract and non-abstract methods
- Can have fields and properties
- Can have constructors
- A class can inherit from only one abstract class
- Interface:
- Can only have abstract method signatures (prior to C# 8.0)
- Cannot have fields (can have properties from C# 8.0)
- Cannot have constructors
- A class can implement multiple interfaces
Q7: Explain method overloading and method overriding in C#.
Method Overloading: Creating multiple methods with the same name but different parameters within the same class.
public class Calculator
{
public int Add(int a, int b)
{
return a + b;
}
public double Add(double a, double b)
{
return a + b;
}
}
Method Overriding: Redefining a method in a derived class that already exists in the base class with the same signature.
public class Animal
{
public virtual void MakeSound()
{
Console.WriteLine("The animal makes a sound");
}
}
public class Dog : Animal
{
public override void MakeSound()
{
Console.WriteLine("The dog barks");
}
}
3. Advanced C# Concepts
Q8: What are delegates and events in C#? How are they related?
Delegates are type-safe function pointers in C#. They allow methods to be passed as parameters and can be used to create callback mechanisms.
public delegate void MyDelegate(string message);
public class MyClass
{
public void MyMethod(string message)
{
Console.WriteLine(message);
}
}
MyClass obj = new MyClass();
MyDelegate del = obj.MyMethod;
del("Hello, World!");
Events are a way to implement the publish-subscribe pattern using delegates. They provide a level of encapsulation and control over the delegate.
public class Publisher
{
public event EventHandler<EventArgs> MyEvent;
protected virtual void OnMyEvent()
{
MyEvent?.Invoke(this, EventArgs.Empty);
}
}
public class Subscriber
{
public void HandleEvent(object sender, EventArgs e)
{
Console.WriteLine("Event received!");
}
}
Publisher pub = new Publisher();
Subscriber sub = new Subscriber();
pub.MyEvent += sub.HandleEvent;
Q9: Explain the concept of generics in C#. What are their benefits?
Generics allow you to write code that can work with any data type while maintaining type safety. Benefits include:
- Type safety at compile-time
- Code reusability
- Better performance by avoiding boxing/unboxing
- Cleaner and more readable code
public class GenericList<T>
{
private List<T> items = new List<T>();
public void Add(T item)
{
items.Add(item);
}
public T GetItem(int index)
{
return items[index];
}
}
GenericList<int> intList = new GenericList<int>();
intList.Add(1);
int value = intList.GetItem(0);
Q10: What are extension methods, and how are they useful?
Extension methods allow you to add new methods to existing types without modifying the original type. They are particularly useful for adding functionality to types you don’t have control over, such as built-in .NET types.
public static class StringExtensions
{
public static bool IsValidEmail(this string email)
{
// Simplified email validation logic
return email.Contains("@") && email.Contains(".");
}
}
string email = "user@example.com";
bool isValid = email.IsValidEmail();
4. .NET Framework and CLR
Q11: What is the Common Language Runtime (CLR), and what role does it play in .NET applications?
The Common Language Runtime (CLR) is the virtual machine component of the .NET Framework responsible for managing the execution of .NET applications. Its key responsibilities include:
- Memory management and garbage collection
- Code execution and just-in-time (JIT) compilation
- Type safety and security enforcement
- Exception handling
- Thread management
Q12: Explain the difference between managed and unmanaged code.
Managed Code:
- Executed by the CLR
- Benefits from automatic memory management
- Has access to .NET Framework features
- Examples: C#, VB.NET, F#
Unmanaged Code:
- Executed directly by the operating system
- Requires manual memory management
- Doesn’t have direct access to .NET Framework features
- Examples: C, C++
Q13: What is the difference between .NET Framework, .NET Core, and .NET 5+?
.NET Framework:
- Original implementation of .NET
- Windows-only
- Large API surface
.NET Core:
- Cross-platform, open-source reimplementation of .NET
- Modular and lightweight
- High-performance
.NET 5+ (including .NET 6, 7, etc.):
- Unification of .NET Framework and .NET Core
- Cross-platform
- Single BCL (Base Class Library) and runtime
- Improved performance and new features
5. Performance and Optimization
Q14: What is the difference between StringBuilder and String in C#? When would you use one over the other?
String:
- Immutable – each operation creates a new string object
- Good for storing and passing around string values
- Efficient for small number of concatenations
StringBuilder:
- Mutable – allows in-place modifications
- More efficient for multiple string manipulations
- Ideal for building large strings or when doing many concatenations
// Using String
string result = "";
for (int i = 0; i < 1000; i++)
{
result += i.ToString(); // Inefficient for large loops
}
// Using StringBuilder
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++)
{
sb.Append(i); // More efficient
}
string result = sb.ToString();
Q15: What is the ‘using’ statement in C#, and how does it help with resource management?
The using
statement in C# provides a convenient syntax that ensures the correct use of IDisposable objects. It automatically calls the Dispose method on the object when it goes out of scope, even if an exception is thrown.
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
// Use the connection...
} // Connection is automatically closed and disposed here
This is equivalent to:
SqlConnection connection = new SqlConnection(connectionString);
try
{
connection.Open();
// Use the connection...
}
finally
{
if (connection != null)
((IDisposable)connection).Dispose();
}
Q16: Explain the concept of lazy loading in C#. How can it improve performance?
Lazy loading is a design pattern that defers the initialization of an object until it’s actually needed. This can improve performance by:
- Reducing initial load time
- Conserving memory
- Avoiding unnecessary database queries or expensive computations
C# provides the Lazy<T>
class to implement lazy loading:
public class ExpensiveObject
{
public ExpensiveObject()
{
// Simulate expensive creation
Thread.Sleep(2000);
}
}
public class Container
{
private Lazy<ExpensiveObject> lazyObject = new Lazy<ExpensiveObject>();
public ExpensiveObject ExpensiveObject
{
get { return lazyObject.Value; }
}
}
Container container = new Container();
// ExpensiveObject is not created yet
Console.WriteLine("Accessing ExpensiveObject...");
var obj = container.ExpensiveObject; // ExpensiveObject is created here
6. Coding Challenges
Q17: Write a C# method to reverse a string without using any built-in reverse functions.
public static string ReverseString(string input)
{
char[] charArray = input.ToCharArray();
int left = 0;
int right = charArray.Length - 1;
while (left < right)
{
// Swap characters
char temp = charArray[left];
charArray[left] = charArray[right];
charArray[right] = temp;
left++;
right--;
}
return new string(charArray);
}
// Usage
string original = "Hello, World!";
string reversed = ReverseString(original);
Console.WriteLine(reversed); // Output: !dlroW ,olleH
Q18: Implement a simple thread-safe singleton pattern in C#.
public sealed class Singleton
{
private static Singleton instance = null;
private static readonly object padlock = new object();
private Singleton()
{
}
public static Singleton Instance
{
get
{
if (instance == null)
{
lock (padlock)
{
if (instance == null)
{
instance = new Singleton();
}
}
}
return instance;
}
}
}
// Usage
Singleton s1 = Singleton.Instance;
Singleton s2 = Singleton.Instance;
Console.WriteLine(object.ReferenceEquals(s1, s2)); // Output: True
Q19: Write a C# method to find the nth Fibonacci number using recursion and memoization.
public class FibonacciCalculator
{
private Dictionary<int, long> memo = new Dictionary<int, long>();
public long Fibonacci(int n)
{
if (n <= 1)
return n;
if (memo.ContainsKey(n))
return memo[n];
long result = Fibonacci(n - 1) + Fibonacci(n - 2);
memo[n] = result;
return result;
}
}
// Usage
FibonacciCalculator calculator = new FibonacciCalculator();
Console.WriteLine(calculator.Fibonacci(10)); // Output: 55
Console.WriteLine(calculator.Fibonacci(50)); // Output: 12586269025
7. Best Practices and Design Patterns
Q20: What are SOLID principles? Can you explain each one briefly?
SOLID is an acronym for five design principles in object-oriented programming:
- Single Responsibility Principle: A class should have only one reason to change.
- Open/Closed Principle: Software entities should be open for extension but closed for modification.
- Liskov Substitution Principle: Objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program.
- Interface Segregation Principle: Many client-specific interfaces are better than one general-purpose interface.
- Dependency Inversion Principle: High-level modules should not depend on low-level modules. Both should depend on abstractions.
Q21: What is dependency injection, and how is it implemented in C#?
Dependency Injection (DI) is a design pattern that implements Inversion of Control (IoC) for resolving dependencies. It allows for loosely coupled code by injecting dependencies into a class rather than creating them inside the class.
In C#, DI can be implemented manually or using frameworks like Microsoft.Extensions.DependencyInjection:
public interface ILogger
{
void Log(string message);
}
public class ConsoleLogger : ILogger
{
public void Log(string message)
{
Console.WriteLine($"Log: {message}");
}
}
public class UserService
{
private readonly ILogger _logger;
public UserService(ILogger logger)
{
_logger = logger;
}
public void CreateUser(string username)
{
// Create user logic
_logger.Log($"User created: {username}");
}
}
// Using Microsoft.Extensions.DependencyInjection
var services = new ServiceCollection();
services.AddSingleton<ILogger, ConsoleLogger>();
services.AddTransient<UserService>();
var serviceProvider = services.BuildServiceProvider();
var userService = serviceProvider.GetService<UserService>();
userService.CreateUser("JohnDoe");
8. Interview Tips and Preparation Strategies
Practice Coding Regularly
Consistent practice is key to mastering C# and preparing for technical interviews. Consider using platforms like LeetCode, HackerRank, or CodeWars to solve coding challenges regularly. Focus on implementing common data structures and algorithms in C#.
Review C# Language Features
Stay up-to-date with the latest C# features. Review recent versions of C# (e.g., C# 9.0, 10.0) and understand new language capabilities like records, pattern matching enhancements, and top-level statements.
Understand .NET Ecosystem
Familiarize yourself with the broader .NET ecosystem, including ASP.NET Core for web development, Entity Framework Core for data access, and popular third-party libraries and tools commonly used in C# development.
Mock Interviews
Conduct mock interviews with peers or use online platforms that offer mock interview services. This will help you get comfortable with explaining your thought process and coding under pressure.
Soft Skills Matter
Don’t forget about soft skills. Practice explaining complex technical concepts clearly and concisely. Be prepared to discuss your past projects and how you’ve solved challenging problems using C# and .NET technologies.
Stay Calm and Ask Questions
During the interview, stay calm and don’t hesitate to ask for clarification if you’re unsure about a question. It’s better to ask for more information than to make incorrect assumptions.
Follow-up and Learn
After each interview, whether successful or not, reflect on the experience. Identify areas where you can improve and focus on strengthening those skills for future interviews.
Remember, preparing for C# interviews is not just about memorizing answers but about understanding core concepts and being able to apply them practically. Good luck with your interview preparation!